Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Automation / Peers / ContentElementAutomationPeer.cs / 1305600 / ContentElementAutomationPeer.cs
//---------------------------------------------------------------------------- // // File: ContentElementAutomationPeer.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Automation element for ContentElements // //--------------------------------------------------------------------------- using System; // Object using System.Collections.Generic; // Listusing System.Windows.Input; // AccessKeyManager using MS.Internal.PresentationCore; // SR using System.Windows.Automation.Provider; using System.Windows.Automation; using MS.Internal.Automation; namespace System.Windows.Automation.Peers { /// public class ContentElementAutomationPeer : AutomationPeer { /// public ContentElementAutomationPeer(ContentElement owner) { if (owner == null) { throw new ArgumentNullException("owner"); } _owner = owner; } /// public ContentElement Owner { get { return _owner; } } /// /// This static helper creates an AutomationPeer for the specified element and /// caches it - that means the created peer is going to live long and shadow the /// element for its lifetime. The peer will be used by Automation to proxy the element, and /// to fire events to the Automation when something happens with the element. /// The created peer is returned from this method and also from subsequent calls to this method /// and public static AutomationPeer CreatePeerForElement(ContentElement element) { if (element == null) { throw new ArgumentNullException("element"); } return element.CreateAutomationPeer(); } /// public static AutomationPeer FromElement(ContentElement element) { if (element == null) { throw new ArgumentNullException("element"); } return element.GetAutomationPeer(); } ///. The type of the peer is determined by the /// virtual callback. If FrameworkContentElement does not /// implement the callback, there will be no peer and this method will return 'null' (in other /// words, there is no such thing as a 'default peer'). /// /// override protected List/// GetChildrenCore() { return null; } /// override public object GetPattern(PatternInterface patternInterface) { //Support synchronized input if (patternInterface == PatternInterface.SynchronizedInput) { // Adaptor object is used here to avoid loading UIA assemblies in non-UIA scenarios. if (_synchronizedInputPattern == null) _synchronizedInputPattern = new SynchronizedInputAdaptor(_owner); return _synchronizedInputPattern; } return null; } /// /// protected override AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.Custom; } ////// /// protected override string GetAutomationIdCore() { return AutomationProperties.GetAutomationId(_owner); } ////// /// protected override string GetNameCore() { return AutomationProperties.GetName(_owner); } ////// /// protected override string GetHelpTextCore() { return AutomationProperties.GetHelpText(_owner); } ////// /// override protected Rect GetBoundingRectangleCore() { return Rect.Empty; } ////// /// override protected bool IsOffscreenCore() { return true; } ////// /// override protected AutomationOrientation GetOrientationCore() { return AutomationOrientation.None; } ////// /// override protected string GetItemTypeCore() { return string.Empty; } ////// /// override protected string GetClassNameCore() { return string.Empty; } ////// /// override protected string GetItemStatusCore() { return string.Empty; } ////// /// override protected bool IsRequiredForFormCore() { return false; } ////// /// override protected bool IsKeyboardFocusableCore() { return Keyboard.IsFocusable(_owner); } ////// /// override protected bool HasKeyboardFocusCore() { return _owner.IsKeyboardFocused; } ////// /// override protected bool IsEnabledCore() { return _owner.IsEnabled; } ////// /// override protected bool IsPasswordCore() { return false; } ////// /// override protected bool IsContentElementCore() { return true; } ////// /// override protected bool IsControlElementCore() { return false; } ////// /// override protected AutomationPeer GetLabeledByCore() { return null; } ////// /// override protected string GetAcceleratorKeyCore() { return string.Empty; } ////// /// override protected string GetAccessKeyCore() { return AccessKeyManager.InternalGetAccessKeyCharacter(_owner); } ////// /// override protected Point GetClickablePointCore() { return new Point(double.NaN, double.NaN); } ////// /// override protected void SetFocusCore() { if (!_owner.Focus()) throw new InvalidOperationException(SR.Get(SRID.SetFocusFailed)); } /// internal override Rect GetVisibleBoundingRectCore() { return GetBoundingRectangle(); } private ContentElement _owner; private SynchronizedInputAdaptor _synchronizedInputPattern; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.///
Link Menu
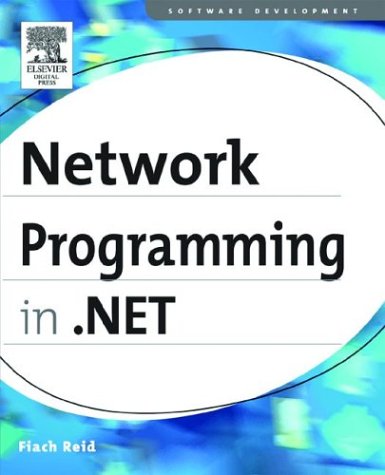
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MetafileHeaderWmf.cs
- GenericEnumConverter.cs
- ProcessInfo.cs
- WindowsClientElement.cs
- WpfGeneratedKnownProperties.cs
- AdRotatorDesigner.cs
- Registry.cs
- MetadataArtifactLoaderComposite.cs
- EncryptedData.cs
- URLIdentityPermission.cs
- PersistChildrenAttribute.cs
- storagemappingitemcollection.viewdictionary.cs
- SqlBuilder.cs
- SoundPlayerAction.cs
- SaveRecipientRequest.cs
- TextSelectionHighlightLayer.cs
- BasicViewGenerator.cs
- EqualityComparer.cs
- TdsParserStateObject.cs
- CustomErrorsSectionWrapper.cs
- DataGridViewImageCell.cs
- Light.cs
- MouseActionConverter.cs
- List.cs
- ArrayElementGridEntry.cs
- prompt.cs
- LocalizableAttribute.cs
- FixedSOMPageElement.cs
- SBCSCodePageEncoding.cs
- StorageBasedPackageProperties.cs
- CapabilitiesSection.cs
- TranslateTransform.cs
- MergeFilterQuery.cs
- TextEncodedRawTextWriter.cs
- OleDbWrapper.cs
- __FastResourceComparer.cs
- RoleManagerModule.cs
- VBCodeProvider.cs
- LogSwitch.cs
- LocatorBase.cs
- PersonalizationAdministration.cs
- ResourceDictionary.cs
- BindingExpressionUncommonField.cs
- ScriptReferenceBase.cs
- SqlDataSourceView.cs
- followingquery.cs
- PathSegmentCollection.cs
- WorkBatch.cs
- OpenTypeLayout.cs
- NonPrimarySelectionGlyph.cs
- MenuStrip.cs
- MediaElementAutomationPeer.cs
- DocumentGridPage.cs
- ParameterRetriever.cs
- Unit.cs
- TypeResolvingOptions.cs
- MaskedTextBoxDesigner.cs
- SemaphoreSecurity.cs
- SQLMoney.cs
- TripleDES.cs
- Repeater.cs
- wgx_exports.cs
- SmtpNegotiateAuthenticationModule.cs
- RelatedView.cs
- SqlConnectionString.cs
- LayoutManager.cs
- TdsParserStaticMethods.cs
- SessionSwitchEventArgs.cs
- BooleanFunctions.cs
- InvalidPrinterException.cs
- InternalBufferOverflowException.cs
- ObfuscationAttribute.cs
- RectIndependentAnimationStorage.cs
- CheckBoxRenderer.cs
- SizeValueSerializer.cs
- JsonDataContract.cs
- EventLogInternal.cs
- DeploymentSection.cs
- GridViewColumnCollection.cs
- TemplateControl.cs
- ProfileBuildProvider.cs
- KnownBoxes.cs
- Not.cs
- Codec.cs
- TextLineBreak.cs
- XmlSchemaProviderAttribute.cs
- SqlDataSourceFilteringEventArgs.cs
- NamedPipeAppDomainProtocolHandler.cs
- WaitHandleCannotBeOpenedException.cs
- LineBreakRecord.cs
- CircleHotSpot.cs
- VisualBrush.cs
- RemoteWebConfigurationHost.cs
- PermissionSetTriple.cs
- QueryCursorEventArgs.cs
- METAHEADER.cs
- httpserverutility.cs
- AssemblyCache.cs
- ColumnResult.cs
- IndicFontClient.cs