Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / WebControls / CircleHotSpot.cs / 1 / CircleHotSpot.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.ComponentModel; using System.Globalization; using System.Web.UI; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class CircleHotSpot : HotSpot { protected internal override string MarkupName { get { return "circle"; } } ///Implements HotSpot for Circular regions. ////// [ DefaultValue(0), WebCategory("Appearance"), WebSysDescription(SR.CircleHotSpot_Radius), ] public int Radius { get { object radius = ViewState["Radius"]; return (radius == null)? 0 : (int)radius; } set { if (value < 0) { throw new ArgumentOutOfRangeException("value"); } ViewState["Radius"] = value; } } [ DefaultValue(0), WebCategory("Appearance"), WebSysDescription(SR.CircleHotSpot_X), ] public int X { get { object o = ViewState["X"]; return o != null? (int)o : 0; } set { ViewState["X"] = value; } } [ DefaultValue(0), WebCategory("Appearance"), WebSysDescription(SR.CircleHotSpot_Y), ] public int Y { get { object o = ViewState["Y"]; return o != null? (int)o : 0; } set { ViewState["Y"] = value; } } public override string GetCoordinates() { return X + "," + Y + "," + Radius; } } }Gets or sets the radius of the MapCircle. ///
Link Menu
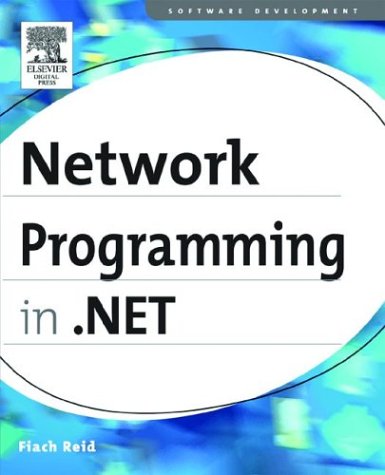
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AutomationElement.cs
- SyntaxCheck.cs
- NativeMethods.cs
- DesignerLabelAdapter.cs
- WindowInteropHelper.cs
- SerializationAttributes.cs
- LineServicesCallbacks.cs
- KeyConverter.cs
- WorkflowDesignerColors.cs
- LightweightEntityWrapper.cs
- GridLengthConverter.cs
- RedBlackList.cs
- Monitor.cs
- ProfilePropertySettings.cs
- ServiceDebugElement.cs
- XPathNodeIterator.cs
- TextBoxView.cs
- ManualResetEventSlim.cs
- COMException.cs
- ObjectStateEntryDbUpdatableDataRecord.cs
- ClosableStream.cs
- Delay.cs
- WebPartDisplayMode.cs
- WS2007FederationHttpBindingElement.cs
- ManifestSignedXml.cs
- KeyMatchBuilder.cs
- RelationshipManager.cs
- StrokeNodeEnumerator.cs
- XmlQueryType.cs
- TextComposition.cs
- JsonEncodingStreamWrapper.cs
- SQLStringStorage.cs
- ThrowHelper.cs
- TextSpanModifier.cs
- XmlSchemaAnnotation.cs
- XmlLinkedNode.cs
- SystemResourceHost.cs
- RegexWorker.cs
- DoubleCollectionValueSerializer.cs
- FilteredAttributeCollection.cs
- SqlConnection.cs
- Point.cs
- SoapAttributeAttribute.cs
- VisualStyleRenderer.cs
- webclient.cs
- TextCompositionManager.cs
- EFTableProvider.cs
- TraceEventCache.cs
- BuildResultCache.cs
- SqlExpressionNullability.cs
- DivideByZeroException.cs
- SqlServer2KCompatibilityAnnotation.cs
- AmbientLight.cs
- HotSpotCollection.cs
- ImageListUtils.cs
- JoinSymbol.cs
- FunctionMappingTranslator.cs
- RegisterResponseInfo.cs
- EndpointAddress.cs
- ControlBuilder.cs
- ContextQuery.cs
- SqlFormatter.cs
- newinstructionaction.cs
- PersistChildrenAttribute.cs
- EntityProxyTypeInfo.cs
- SafeNativeMethodsOther.cs
- infer.cs
- RayHitTestParameters.cs
- Span.cs
- MailDefinitionBodyFileNameEditor.cs
- Catch.cs
- SuppressMessageAttribute.cs
- FileUtil.cs
- TableMethodGenerator.cs
- MappingSource.cs
- TriState.cs
- Popup.cs
- EndpointDispatcher.cs
- control.ime.cs
- _FtpDataStream.cs
- DataGridViewCellFormattingEventArgs.cs
- CommonGetThemePartSize.cs
- SecurityKeyUsage.cs
- Attributes.cs
- Stackframe.cs
- AuthenticationException.cs
- BitmapEffectGroup.cs
- ListViewItemSelectionChangedEvent.cs
- SecurityManager.cs
- Window.cs
- LabelEditEvent.cs
- StagingAreaInputItem.cs
- EndPoint.cs
- SQLChars.cs
- CommandSet.cs
- DoubleMinMaxAggregationOperator.cs
- XmlName.cs
- ADMembershipUser.cs
- KeyProperty.cs
- ThicknessConverter.cs