Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / WebControls / HotSpotCollection.cs / 1305376 / HotSpotCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.Drawing.Design; using System.Web.UI; namespace System.Web.UI.WebControls { ////// [ Editor("System.Web.UI.Design.WebControls.HotSpotCollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)) ] public sealed class HotSpotCollection : StateManagedCollection { private static readonly Type[] knownTypes = new Type[] { typeof(CircleHotSpot), typeof(RectangleHotSpot), typeof(PolygonHotSpot), }; ///Collection of HotSpots. ////// public HotSpot this[int index] { get { return (HotSpot)((IList)this)[index]; } } ///Returns the HotSpot at a given index. ////// public int Add(HotSpot spot) { return ((IList)this).Add(spot); } ///Adds a HotSpot to the collection. ////// protected override object CreateKnownType(int index) { switch (index) { case 0: return new CircleHotSpot(); case 1: return new RectangleHotSpot(); case 2: return new PolygonHotSpot(); default: throw new ArgumentOutOfRangeException(SR.GetString(SR.HotSpotCollection_InvalidTypeIndex)); } } ///Creates a known type of HotSpot. ////// protected override Type[] GetKnownTypes() { return knownTypes; } ///Returns an ArrayList of known HotSpot types. ////// public void Insert(int index, HotSpot spot) { ((IList)this).Insert(index, spot); } ///Inserts a HotSpot into the collection. ////// protected override void OnValidate(object o) { base.OnValidate(o); if (!(o is HotSpot)) throw new ArgumentException(SR.GetString(SR.HotSpotCollection_InvalidType)); } ///Validates that an object is a HotSpot. ////// public void Remove(HotSpot spot) { ((IList)this).Remove(spot); } ///Removes a HotSpot from the collection. ////// public void RemoveAt(int index) { ((IList)this).RemoveAt(index); } ///Removes a HotSpot from the collection at a given index. ////// protected override void SetDirtyObject(object o) { ((HotSpot)o).SetDirty(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Marks a HotSpot as dirty so that it will record its entire state into view state. ///
Link Menu
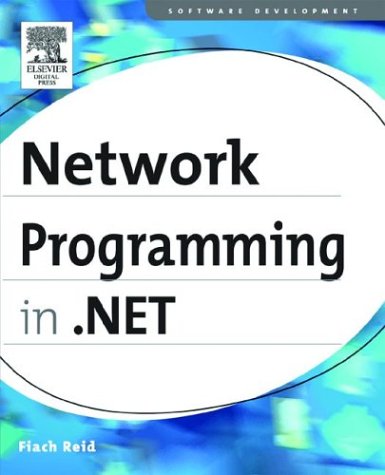
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EventLogInternal.cs
- DayRenderEvent.cs
- CompressedStack.cs
- MenuItem.cs
- SvcFileManager.cs
- JournalEntryStack.cs
- ConfigUtil.cs
- DataGridViewColumn.cs
- ParallelEnumerableWrapper.cs
- PreservationFileReader.cs
- DataSetUtil.cs
- NavigateEvent.cs
- DataViewListener.cs
- ImageBrush.cs
- Schema.cs
- TdsEnums.cs
- XmlSerializationReader.cs
- DataControlLinkButton.cs
- ExtensionWindowResizeGrip.cs
- DataSourceListEditor.cs
- DbMetaDataColumnNames.cs
- HwndAppCommandInputProvider.cs
- HwndKeyboardInputProvider.cs
- Span.cs
- DefaultAssemblyResolver.cs
- StorageMappingItemCollection.cs
- XpsInterleavingPolicy.cs
- Paragraph.cs
- CounterCreationDataCollection.cs
- MediaContextNotificationWindow.cs
- EntitySqlException.cs
- ListControlStringCollectionEditor.cs
- UIElementPropertyUndoUnit.cs
- ISO2022Encoding.cs
- Script.cs
- RuntimeArgumentHandle.cs
- C14NUtil.cs
- ScriptingAuthenticationServiceSection.cs
- UnsafeNativeMethods.cs
- UpdateProgress.cs
- ContentWrapperAttribute.cs
- Condition.cs
- HostedHttpContext.cs
- CmsUtils.cs
- DataRelationPropertyDescriptor.cs
- CatalogPartCollection.cs
- BooleanStorage.cs
- GenericAuthenticationEventArgs.cs
- Int32CAMarshaler.cs
- EdmType.cs
- RotateTransform3D.cs
- WebPartEditorOkVerb.cs
- InvokeHandlers.cs
- RightsManagementPermission.cs
- PropertyChangeTracker.cs
- NativeMethods.cs
- PnrpPermission.cs
- TextEmbeddedObject.cs
- NativeMethods.cs
- PerfCounterSection.cs
- RuntimeConfigLKG.cs
- ClientFormsAuthenticationCredentials.cs
- ObjectParameter.cs
- adornercollection.cs
- DesignerCategoryAttribute.cs
- AssemblyNameUtility.cs
- EntitySetRetriever.cs
- IncrementalReadDecoders.cs
- basenumberconverter.cs
- Page.cs
- HwndStylusInputProvider.cs
- URIFormatException.cs
- XmlSubtreeReader.cs
- ConfigXmlComment.cs
- SourceFileInfo.cs
- DataIdProcessor.cs
- ListViewUpdatedEventArgs.cs
- DataReceivedEventArgs.cs
- BorderSidesEditor.cs
- WindowsAltTab.cs
- CannotUnloadAppDomainException.cs
- SrgsText.cs
- BamlLocalizableResource.cs
- LinqDataSourceInsertEventArgs.cs
- CollectionViewProxy.cs
- TrackingAnnotationCollection.cs
- DataRow.cs
- AppAction.cs
- EventDescriptor.cs
- __ComObject.cs
- GreaterThanOrEqual.cs
- basenumberconverter.cs
- CharacterString.cs
- SchemaImporterExtensionsSection.cs
- FontInfo.cs
- InfoCardRequestException.cs
- WrappedIUnknown.cs
- X509Chain.cs
- SqlTopReducer.cs
- LinqDataSource.cs