Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Controls / UIElementCollection.cs / 1 / UIElementCollection.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: UIElementCollection.cs // // Description: Contains the UIElementCollection base class. // // History: // 07/18/2003 : [....] - Added to WCP branch // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Windows.Media; using System.Windows.Threading; using System.Windows.Markup; namespace System.Windows.Controls { ////// A UIElementCollection is a ordered collection of UIElements. /// ////// A UIElementCollection has implied context affinity. It is a violation to access /// the collection from a different context than that of the owning Panel. /// ///public class UIElementCollection : IList { /// /// The colleciton is the children collection of the visualParent. The logicalParent /// is used to do logical parenting. The flags is used to invalidate /// the resource properties in the child tree, if an Application object exists. /// /// The element of whom this is a children collection /// The logicalParent of the elements of this collection. /// if overriding Panel.CreateUIElementCollection, pass the logicalParent parameter of that method here. /// public UIElementCollection(UIElement visualParent, FrameworkElement logicalParent) { if (visualParent == null) { throw new ArgumentNullException(SR.Get(SRID.Panel_NoNullVisualParent, "visualParent", this.GetType())); } _visualChildren = new VisualCollection(visualParent); _visualParent = visualParent; _logicalParent = logicalParent; } ////// Gets the number of elements in the collection. /// public virtual int Count { get { return _visualChildren.Count; } } ////// Gets a value indicating whether access to the ICollection is synchronized (thread-safe). /// For more details, see public virtual bool IsSynchronized { get { return _visualChildren.IsSynchronized; } } ////// /// For more details, see public virtual object SyncRoot { get { return _visualChildren.SyncRoot; } } ////// /// Copies the collection into the Array. /// For more details, see public virtual void CopyTo(Array array, int index) { _visualChildren.CopyTo(array, index); } ////// /// Strongly typed version of CopyTo /// Copies the collection into the Array. /// For more details, see public virtual void CopyTo(UIElement[] array, int index) { _visualChildren.CopyTo(array, index); } ////// /// For more details, see public virtual int Capacity { get { return _visualChildren.Capacity; } set { VerifyWriteAccess(); _visualChildren.Capacity = value; } } ////// /// For more details, see public virtual UIElement this[int index] { get { return _visualChildren[index] as UIElement; } set { VerifyWriteAccess(); ValidateElement(value); VisualCollection vc = _visualChildren; //if setting new element into slot or assigning null, //remove previously hooked element from the logical tree if (vc[index] != value) { UIElement e = vc[index] as UIElement; if (e != null) ClearLogicalParent(e); vc[index] = value; SetLogicalParent(value); _visualParent.InvalidateMeasure(); } } } // Warning: this method is very dangerous because it does not prevent adding children // into collection populated by generator. This may cause crashes if used incorrectly. // Don't call this unless you are deriving a panel that is populating the collection // in cooperation with the generator internal void SetInternal(int index, UIElement item) { ValidateElement(item); VisualCollection vc = _visualChildren; if(vc[index] != item) { vc[index] = null; // explicitly disconnect the existing visual; vc[index] = item; _visualParent.InvalidateMeasure(); } } ////// /// Adds the element to the UIElementCollection /// For more details, see public virtual int Add(UIElement element) { VerifyWriteAccess(); return AddInternal(element); } // Warning: this method is very dangerous because it does not prevent adding children // into collection populated by generator. This may cause crashes if used incorrectly. // Don't call this unless you are deriving a panel that is populating the collection // in cooperation with the generator internal int AddInternal(UIElement element) { ValidateElement(element); SetLogicalParent(element); int retVal = _visualChildren.Add(element); // invalidate measure on visual parent _visualParent.InvalidateMeasure(); return retVal; } ////// /// Returns the index of the element in the UIElementCollection /// For more details, see public virtual int IndexOf(UIElement element) { return _visualChildren.IndexOf(element); } ////// /// Removes the specified element from the UIElementCollection. /// For more details, see public virtual void Remove(UIElement element) { VerifyWriteAccess(); _visualChildren.Remove(element); ClearLogicalParent(element); _visualParent.InvalidateMeasure(); } ////// /// Removes the specified element from the UIElementCollection. /// Used only by ItemsControl /// For more details, see internal virtual void RemoveNoVerify(UIElement element) { _visualChildren.Remove(element); } ////// /// Determines whether a element is in the UIElementCollection. /// For more details, see public virtual bool Contains(UIElement element) { return _visualChildren.Contains(element); } ////// /// Removes all elements from the UIElementCollection. /// For more details, see public virtual void Clear() { VerifyWriteAccess(); ClearInternal(); } // Warning: this method is very dangerous because it does not prevent adding children // into collection populated by generator. This may cause crashes if used incorrectly. // Don't call this unless you are deriving a panel that is populating the collection // in cooperation with the generator internal void ClearInternal() { VisualCollection vc = _visualChildren; int cnt = vc.Count; if (cnt > 0) { // copy children in VisualCollection so that we can clear the visual link first, // followed by the logical link Visual[] visuals = new Visual[cnt]; for (int i = 0; i < cnt; i++) { visuals[i] = vc[i]; } vc.Clear(); //disconnect from logical tree for (int i = 0; i < cnt; i++) { UIElement e = visuals[i] as UIElement; if (e != null) { ClearLogicalParent(e); } } _visualParent.InvalidateMeasure(); } } ////// /// Inserts an element into the UIElementCollection at the specified index. /// For more details, see public virtual void Insert(int index, UIElement element) { VerifyWriteAccess(); InsertInternal(index, element); } // Warning: this method is very dangerous because it does not prevent adding children // into collection populated by generator. This may cause crashes if used incorrectly. // Don't call this unless you are deriving a panel that is populating the collection // in cooperation with the generator internal void InsertInternal(int index, UIElement element) { ValidateElement(element); SetLogicalParent(element); _visualChildren.Insert(index, element); _visualParent.InvalidateMeasure(); } ////// /// Removes the UIElement at the specified index. /// For more details, see public virtual void RemoveAt(int index) { VerifyWriteAccess(); VisualCollection vc = _visualChildren; //disconnect from logical tree UIElement e = vc[index] as UIElement; vc.RemoveAt(index); if (e != null) ClearLogicalParent(e); _visualParent.InvalidateMeasure(); } ////// /// Removes a range of Visuals from the VisualCollection. /// For more details, see public virtual void RemoveRange(int index, int count) { VerifyWriteAccess(); RemoveRangeInternal(index, count); } // Warning: this method is very dangerous because it does not prevent adding children // into collection populated by generator. This may cause crashes if used incorrectly. // Don't call this unless you are deriving a panel that is populating the collection // in cooperation with the generator internal void RemoveRangeInternal(int index, int count) { VisualCollection vc = _visualChildren; int cnt = vc.Count; if (count > (cnt - index)) { count = cnt - index; } if (count > 0) { // copy children in VisualCollection so that we can clear the visual link first, // followed by the logical link Visual[] visuals = new Visual[count]; int i = index; for (int loop = 0; loop < count; i++, loop++) { visuals[loop] = vc[i]; } vc.RemoveRange(index, count); //disconnect from logical tree for (i = 0; i < count; i++) { UIElement e = visuals[i] as UIElement; if (e != null) { ClearLogicalParent(e); } } _visualParent.InvalidateMeasure(); } } private UIElement Cast(object value) { if (value == null) throw new System.ArgumentException(SR.Get(SRID.Collection_NoNull, "UIElementCollection")); UIElement element = value as UIElement; if (element == null) throw new System.ArgumentException(SR.Get(SRID.Collection_BadType, "UIElementCollection", value.GetType().Name, "UIElement")); return element; } #region IList Members ////// /// Adds an element to the UIElementCollection /// int IList.Add(object value) { return Add(Cast(value)); } ////// Determines whether an element is in the UIElementCollection. /// bool IList.Contains(object value) { return Contains(value as UIElement); } ////// Returns the index of the element in the UIElementCollection /// int IList.IndexOf(object value) { return IndexOf(value as UIElement); } ////// Inserts an element into the UIElementCollection /// void IList.Insert(int index, object value) { Insert(index, Cast(value)); } ////// bool IList.IsFixedSize { get { return false; } } ////// bool IList.IsReadOnly { get { return false; } } ////// Removes an element from the UIElementCollection /// void IList.Remove(object value) { Remove(value as UIElement); } ////// For more details, see object IList.this[int index] { get { return this[index]; } set { this[index] = Cast(value); } } #endregion // --------------------------------------------------------------- // IEnumerable Interface // --------------------------------------------------------------- ////// /// Returns an enumerator that can iterate through the collection. /// ///Enumerator that enumerates the collection in order. public virtual IEnumerator GetEnumerator() { return _visualChildren.GetEnumerator(); } ////// This method does logical parenting of the given element. /// /// protected void SetLogicalParent(UIElement element) { if (_logicalParent != null) { _logicalParent.AddLogicalChild(element); } } ////// This method removes logical parenting when element goes away from the collection. /// /// protected void ClearLogicalParent(UIElement element) { if (_logicalParent != null) { _logicalParent.RemoveLogicalChild(element); } } ////// Provides access to visual parent. /// internal UIElement VisualParent { get { return (_visualParent); } } // Helper function to validate element; will throw exceptions if problems are detected. private void ValidateElement(UIElement element) { if (element == null) { throw new ArgumentNullException(SR.Get(SRID.Panel_NoNullChildren, this.GetType())); } } private void VerifyWriteAccess() { Panel p = _visualParent as Panel; if (p != null && p.IsDataBound) { throw new InvalidOperationException(SR.Get(SRID.Panel_BoundPanel_NoChildren)); } } private readonly VisualCollection _visualChildren; private readonly UIElement _visualParent; private readonly FrameworkElement _logicalParent; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
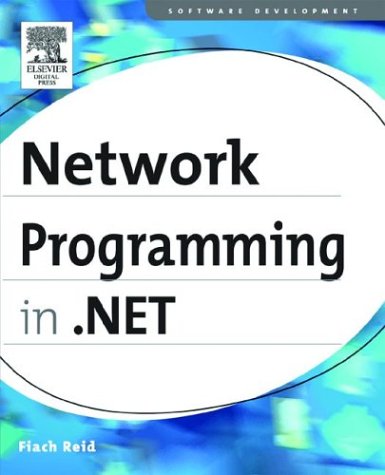
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TableLayoutColumnStyleCollection.cs
- PropertyDescriptorComparer.cs
- HyperLinkColumn.cs
- Types.cs
- XmlSchemaValidationException.cs
- ResourceLoader.cs
- _NetRes.cs
- TypeConverter.cs
- TextLineResult.cs
- ContentFilePart.cs
- CodeIdentifiers.cs
- Message.cs
- PublisherIdentityPermission.cs
- HttpResponseInternalWrapper.cs
- MaterializeFromAtom.cs
- MaterializeFromAtom.cs
- X509CertificateCollection.cs
- WindowsFormsSynchronizationContext.cs
- SqlCachedBuffer.cs
- assemblycache.cs
- TagPrefixAttribute.cs
- XmlObjectSerializerWriteContextComplexJson.cs
- ListManagerBindingsCollection.cs
- ProcessInfo.cs
- ApplicationManager.cs
- ApplicationManager.cs
- AnnotationService.cs
- PointLightBase.cs
- FacetValues.cs
- ListComponentEditorPage.cs
- PageHandlerFactory.cs
- LostFocusEventManager.cs
- MsmqTransportSecurityElement.cs
- BinaryMethodMessage.cs
- PartialTrustVisibleAssembliesSection.cs
- CookieHandler.cs
- LineUtil.cs
- OletxTransactionHeader.cs
- NamespaceList.cs
- IERequestCache.cs
- CalendarDataBindingHandler.cs
- LinqDataSourceContextData.cs
- TreeNodeMouseHoverEvent.cs
- PowerStatus.cs
- AnnotationAdorner.cs
- JournalNavigationScope.cs
- ObjectStateEntry.cs
- MimeTypeMapper.cs
- LocationEnvironment.cs
- StringSorter.cs
- MenuStrip.cs
- PropertyPathWorker.cs
- ConfigXmlComment.cs
- HitTestWithGeometryDrawingContextWalker.cs
- TableChangeProcessor.cs
- ScrollBarRenderer.cs
- PropertyOrder.cs
- InfoCard.cs
- StagingAreaInputItem.cs
- CodeMethodInvokeExpression.cs
- ECDiffieHellmanCng.cs
- GeneralTransformGroup.cs
- BlockUIContainer.cs
- ProvideValueServiceProvider.cs
- DataSpaceManager.cs
- TCEAdapterGenerator.cs
- TextDataBindingHandler.cs
- Parser.cs
- DataListItemCollection.cs
- SimpleWorkerRequest.cs
- EntityCommandExecutionException.cs
- EnvironmentPermission.cs
- WebPartAddingEventArgs.cs
- MDIControlStrip.cs
- X509ChainElement.cs
- OrderedEnumerableRowCollection.cs
- MsmqEncryptionAlgorithm.cs
- PackWebRequestFactory.cs
- LockedBorderGlyph.cs
- CalculatedColumn.cs
- DetailsViewModeEventArgs.cs
- RegexParser.cs
- TargetFrameworkUtil.cs
- SettingsContext.cs
- DataTemplateKey.cs
- PenThread.cs
- TypeViewSchema.cs
- Byte.cs
- SyntaxCheck.cs
- AlgoModule.cs
- TemplatedMailWebEventProvider.cs
- DependencyPropertyKind.cs
- ToolStripDropDownItem.cs
- EntityObject.cs
- MemberPath.cs
- PropertyMappingExceptionEventArgs.cs
- ThaiBuddhistCalendar.cs
- TableLayout.cs
- AppSettingsSection.cs
- SocketException.cs