Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media3D / Generated / PointLightBase.cs / 1305600 / PointLightBase.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Media3D { abstract partial class PointLightBase : Light { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new PointLightBase Clone() { return (PointLightBase)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new PointLightBase CloneCurrentValue() { return (PointLightBase)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void PositionPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { PointLightBase target = ((PointLightBase) d); target.PropertyChanged(PositionProperty); } private static void RangePropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { PointLightBase target = ((PointLightBase) d); target.PropertyChanged(RangeProperty); } private static void ConstantAttenuationPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { PointLightBase target = ((PointLightBase) d); target.PropertyChanged(ConstantAttenuationProperty); } private static void LinearAttenuationPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { PointLightBase target = ((PointLightBase) d); target.PropertyChanged(LinearAttenuationProperty); } private static void QuadraticAttenuationPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { PointLightBase target = ((PointLightBase) d); target.PropertyChanged(QuadraticAttenuationProperty); } #region Public Properties ////// Position - Point3D. Default value is new Point3D(). /// public Point3D Position { get { return (Point3D) GetValue(PositionProperty); } set { SetValueInternal(PositionProperty, value); } } ////// Range - double. Default value is Double.PositiveInfinity. /// public double Range { get { return (double) GetValue(RangeProperty); } set { SetValueInternal(RangeProperty, value); } } ////// ConstantAttenuation - double. Default value is 1.0. /// public double ConstantAttenuation { get { return (double) GetValue(ConstantAttenuationProperty); } set { SetValueInternal(ConstantAttenuationProperty, value); } } ////// LinearAttenuation - double. Default value is 0.0. /// public double LinearAttenuation { get { return (double) GetValue(LinearAttenuationProperty); } set { SetValueInternal(LinearAttenuationProperty, value); } } ////// QuadraticAttenuation - double. Default value is 0.0. /// public double QuadraticAttenuation { get { return (double) GetValue(QuadraticAttenuationProperty); } set { SetValueInternal(QuadraticAttenuationProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the PointLightBase.Position property. /// public static readonly DependencyProperty PositionProperty; ////// The DependencyProperty for the PointLightBase.Range property. /// public static readonly DependencyProperty RangeProperty; ////// The DependencyProperty for the PointLightBase.ConstantAttenuation property. /// public static readonly DependencyProperty ConstantAttenuationProperty; ////// The DependencyProperty for the PointLightBase.LinearAttenuation property. /// public static readonly DependencyProperty LinearAttenuationProperty; ////// The DependencyProperty for the PointLightBase.QuadraticAttenuation property. /// public static readonly DependencyProperty QuadraticAttenuationProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal static Point3D s_Position = new Point3D(); internal const double c_Range = Double.PositiveInfinity; internal const double c_ConstantAttenuation = 1.0; internal const double c_LinearAttenuation = 0.0; internal const double c_QuadraticAttenuation = 0.0; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static PointLightBase() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(PointLightBase); PositionProperty = RegisterProperty("Position", typeof(Point3D), typeofThis, new Point3D(), new PropertyChangedCallback(PositionPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); RangeProperty = RegisterProperty("Range", typeof(double), typeofThis, Double.PositiveInfinity, new PropertyChangedCallback(RangePropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); ConstantAttenuationProperty = RegisterProperty("ConstantAttenuation", typeof(double), typeofThis, 1.0, new PropertyChangedCallback(ConstantAttenuationPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); LinearAttenuationProperty = RegisterProperty("LinearAttenuation", typeof(double), typeofThis, 0.0, new PropertyChangedCallback(LinearAttenuationPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); QuadraticAttenuationProperty = RegisterProperty("QuadraticAttenuation", typeof(double), typeofThis, 0.0, new PropertyChangedCallback(QuadraticAttenuationPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
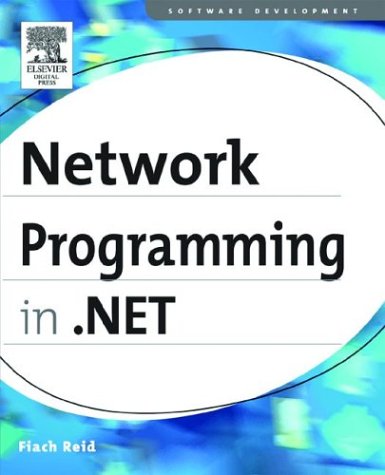
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlConnectionString.cs
- ModuleConfigurationInfo.cs
- CodeFieldReferenceExpression.cs
- ResourceDictionary.cs
- AuthorizationContext.cs
- RTTrackingProfile.cs
- FederatedMessageSecurityOverHttpElement.cs
- EmptyReadOnlyDictionaryInternal.cs
- RegexRunner.cs
- DataGridCell.cs
- FilteredXmlReader.cs
- CodeDefaultValueExpression.cs
- TransactionManager.cs
- AttributeEmitter.cs
- QueryReaderSettings.cs
- PeerApplication.cs
- NotifyParentPropertyAttribute.cs
- AgileSafeNativeMemoryHandle.cs
- XmlSchemaAnnotation.cs
- ThemeDirectoryCompiler.cs
- Pts.cs
- ProgressBarBrushConverter.cs
- NameValuePermission.cs
- HostedElements.cs
- ThousandthOfEmRealPoints.cs
- HitTestWithGeometryDrawingContextWalker.cs
- PreviewKeyDownEventArgs.cs
- StringAnimationBase.cs
- VersionUtil.cs
- TypeDescriptionProviderAttribute.cs
- ToolStripDropDown.cs
- DataGridItemAttachedStorage.cs
- shaperfactoryquerycachekey.cs
- KeyNotFoundException.cs
- PointAnimation.cs
- Int32RectConverter.cs
- OrderByQueryOptionExpression.cs
- CommandBindingCollection.cs
- SignedXml.cs
- ITextView.cs
- EntityClientCacheEntry.cs
- ConfigurationConverterBase.cs
- SerialReceived.cs
- SoapFault.cs
- RemoteArgument.cs
- x509store.cs
- MobileTextWriter.cs
- InfoCardProofToken.cs
- DoubleConverter.cs
- StatusBarItemAutomationPeer.cs
- PropertyGrid.cs
- DocumentPaginator.cs
- XmlStreamNodeWriter.cs
- BinHexEncoder.cs
- SerializationHelper.cs
- Part.cs
- SiteMapNodeItem.cs
- GenericUriParser.cs
- transactioncontext.cs
- Literal.cs
- ToolStripDesignerAvailabilityAttribute.cs
- OverflowException.cs
- DataSetMappper.cs
- ConsoleKeyInfo.cs
- IdentityHolder.cs
- DataGridViewElement.cs
- MarkupCompilePass1.cs
- SchemaImporterExtensionElementCollection.cs
- Tool.cs
- OdbcConnectionOpen.cs
- QilExpression.cs
- XmlValidatingReader.cs
- OracleFactory.cs
- WebPartMenu.cs
- SessionStateSection.cs
- TrackingSection.cs
- CustomTrackingQuery.cs
- ResetableIterator.cs
- MSHTMLHost.cs
- KeyedPriorityQueue.cs
- ButtonBase.cs
- COM2FontConverter.cs
- Utils.cs
- DetailsView.cs
- FunctionNode.cs
- SessionStateUtil.cs
- AssemblyBuilder.cs
- FixedTextPointer.cs
- InputManager.cs
- safesecurityhelperavalon.cs
- DbConnectionStringBuilder.cs
- KeyedHashAlgorithm.cs
- OleDbInfoMessageEvent.cs
- _CommandStream.cs
- CdpEqualityComparer.cs
- FormViewActionList.cs
- PageParserFilter.cs
- DataSourceControl.cs
- EpmCustomContentWriterNodeData.cs
- RSAPKCS1KeyExchangeFormatter.cs