Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / HitTestFilterBehavior.cs / 1305600 / HitTestFilterBehavior.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: HitTestFilterBehavior //----------------------------------------------------------------------------- using System; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Threading; using System.Collections; using System.Diagnostics; using MS.Internal; namespace System.Windows.Media { ////// Behavior for filtering visuals while hit tesitng /// // This enum intentionally does not have a [Flags] attribute. Internally we break this enum // into flags, but the enum values already contain all legal combinations. Users should not // be combining these flags. (Windows OS #1010970) public enum HitTestFilterBehavior { ////// Hit test against current visual and not its children. /// ContinueSkipChildren = HTFBInterpreter.c_DoHitTest, ////// Do not hit test against current visual or its children. /// ContinueSkipSelfAndChildren = 0, ////// Do not hit test against current visual but hit test against children. /// ContinueSkipSelf = HTFBInterpreter.c_IncludeChidren, ////// Hit test against current visual and children. /// Continue = HTFBInterpreter.c_DoHitTest | HTFBInterpreter.c_IncludeChidren, ////// Stop any further hit testing and return. /// Stop = HTFBInterpreter.c_Stop } ////// Delegate for hit tester to control whether to test against the /// current scene graph node. /// public delegate HitTestFilterBehavior HitTestFilterCallback(DependencyObject potentialHitTestTarget); // Static helper class with methods for interpreting the HitTestFilterBehavior enum. internal static class HTFBInterpreter { internal const int c_DoHitTest = (1 << 1); internal const int c_IncludeChidren = (1 << 2); internal const int c_Stop = (1 << 3); internal static bool DoHitTest(HitTestFilterBehavior behavior) { return (((int)behavior) & c_DoHitTest) == c_DoHitTest; } internal static bool IncludeChildren(HitTestFilterBehavior behavior) { return (((int)behavior) & c_IncludeChidren) == c_IncludeChidren; } internal static bool Stop(HitTestFilterBehavior behavior) { return (((int)behavior) & c_Stop) == c_Stop; } internal static bool SkipSubgraph(HitTestFilterBehavior behavior) { return behavior == HitTestFilterBehavior.ContinueSkipSelfAndChildren; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
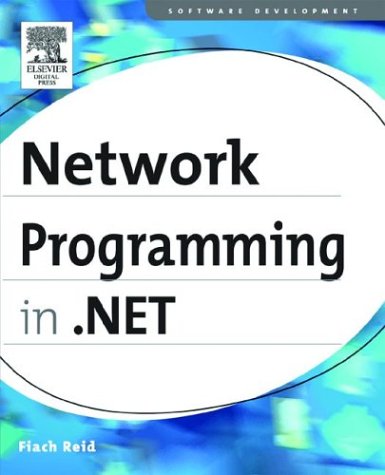
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ItemContainerPattern.cs
- BitmapDownload.cs
- DSACryptoServiceProvider.cs
- DesignerSerializationVisibilityAttribute.cs
- CacheMemory.cs
- XmlCustomFormatter.cs
- SystemIcons.cs
- SecurityHeaderTokenResolver.cs
- SqlTrackingWorkflowInstance.cs
- TdsParserSessionPool.cs
- PinnedBufferMemoryStream.cs
- ChangeInterceptorAttribute.cs
- DebugView.cs
- DescendantBaseQuery.cs
- DataContractJsonSerializerOperationBehavior.cs
- ListSortDescriptionCollection.cs
- ObjectListDesigner.cs
- StaticExtension.cs
- NativeBuffer.cs
- DbProviderFactories.cs
- Convert.cs
- EdmSchemaError.cs
- StorageBasedPackageProperties.cs
- SizeChangedEventArgs.cs
- RemotingAttributes.cs
- ViewDesigner.cs
- XmlRootAttribute.cs
- LinqDataSourceUpdateEventArgs.cs
- CapabilitiesRule.cs
- MouseButton.cs
- FormatSettings.cs
- ProxyWebPartConnectionCollection.cs
- PersonalizationDictionary.cs
- RedirectionProxy.cs
- PointUtil.cs
- TextContainerChangedEventArgs.cs
- NumberSubstitution.cs
- DecimalMinMaxAggregationOperator.cs
- SecurityContextSecurityTokenResolver.cs
- DataGridViewRowErrorTextNeededEventArgs.cs
- OleDbDataAdapter.cs
- XPathAncestorQuery.cs
- FrameworkReadOnlyPropertyMetadata.cs
- EntityDataSourceDesigner.cs
- MulticastIPAddressInformationCollection.cs
- DataSourceDesigner.cs
- InstanceDescriptor.cs
- IdentifierService.cs
- CodeDelegateCreateExpression.cs
- UTF8Encoding.cs
- WarningException.cs
- CornerRadiusConverter.cs
- InvalidOperationException.cs
- GPPOINT.cs
- XDeferredAxisSource.cs
- WebPartUtil.cs
- MultilineStringConverter.cs
- InputProcessorProfilesLoader.cs
- ListControl.cs
- SerTrace.cs
- HttpClientChannel.cs
- ResourceReferenceExpression.cs
- columnmapfactory.cs
- Encoder.cs
- CommentEmitter.cs
- FlowLayout.cs
- WsatAdminException.cs
- NaturalLanguageHyphenator.cs
- AppSettingsExpressionBuilder.cs
- EntityObject.cs
- Calendar.cs
- SecurityContextTokenCache.cs
- EventMap.cs
- DataGridItemCollection.cs
- shaper.cs
- UnsafeNativeMethods.cs
- TransportBindingElement.cs
- UserPreferenceChangedEventArgs.cs
- SiteIdentityPermission.cs
- HtmlInputFile.cs
- ZipPackagePart.cs
- WindowsTab.cs
- ExpressionBuilderContext.cs
- WebContentFormatHelper.cs
- ActivityBuilderHelper.cs
- DataKeyArray.cs
- OrderedDictionaryStateHelper.cs
- SystemIPInterfaceStatistics.cs
- ChangeNode.cs
- DataBindingExpressionBuilder.cs
- DesignerGenericWebPart.cs
- GeneralTransform3D.cs
- _DisconnectOverlappedAsyncResult.cs
- ObjectQueryExecutionPlan.cs
- EntityContainerEntitySetDefiningQuery.cs
- AsymmetricSignatureFormatter.cs
- AppDomain.cs
- AlphaSortedEnumConverter.cs
- EntitySetRetriever.cs
- XmlnsCache.cs