Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Objects / Internal / ObjectQueryExecutionPlan.cs / 1 / ObjectQueryExecutionPlan.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Objects.Internal { using System; using System.Collections.Generic; using System.Data.Common; using System.Data.Common.CommandTrees; using System.Data.Common.EntitySql; using System.Data.Common.QueryCache; using System.Data.EntityClient; using System.Data.Metadata.Edm; using System.Data.Objects; using System.Data.Objects.DataClasses; using System.Diagnostics; using System.Reflection; using System.Linq.Expressions; using System.Data.Common.Internal.Materialization; using System.Data.Query.InternalTrees; ////// Represents the 'compiled' form of all elements (query + result assembly) required to execute a specific internal sealed class ObjectQueryExecutionPlan { internal readonly DbCommandDefinition CommandDefinition; internal readonly ShaperFactory ResultShaperFactory; internal readonly TypeUsage ResultType; internal readonly MergeOption MergeOption; ////// If the query yields entities from a single entity set, the value is stored here. private readonly EntitySet _singleEntitySet; internal ObjectQueryExecutionPlan(DbCommandDefinition commandDefinition, ShaperFactory resultShaperFactory, TypeUsage resultType, MergeOption mergeOption, EntitySet singleEntitySet) { Debug.Assert(commandDefinition != null, "A command definition is required"); Debug.Assert(resultShaperFactory != null, "A result shaper factory is required"); Debug.Assert(resultType != null, "A result type is required"); this.CommandDefinition = commandDefinition; this.ResultShaperFactory = resultShaperFactory; this.ResultType = resultType; this.MergeOption = mergeOption; this._singleEntitySet = singleEntitySet; } internal static ObjectQueryExecutionPlan Prepare(ObjectContext context, DbQueryCommandTree tree, Type elementType, MergeOption mergeOption, Span span) { TypeUsage treeResultType = tree.Query.ResultType; // Rewrite this tree for Span? DbExpression spannedQuery = null; SpanIndex spanInfo; if (ObjectSpanRewriter.TryRewrite(tree.Query, span, mergeOption, out spannedQuery, out spanInfo)) { tree.Query = spannedQuery; } else { spanInfo = null; } DbConnection connection = context.Connection; DbCommandDefinition definition = null; // The connection is required to get to the CommandDefinition builder. if (connection == null) { throw EntityUtil.InvalidOperation(System.Data.Entity.Strings.ObjectQuery_InvalidConnection); } DbProviderServices services = DbProviderServices.GetProviderServices(connection); try { definition = services.CreateCommandDefinition(tree); } catch (EntityCommandCompilationException) { // If we're running against EntityCommand, we probably already caught the providers' // exception and wrapped it, we don't want to do that again, so we'll just rethrow // here instead. throw; } catch (Exception e) { // we should not be wrapping all exceptions if (EntityUtil.IsCatchableExceptionType(e)) { // we don't wan't folks to have to know all the various types of exceptions that can // occur, so we just rethrow a CommandDefinitionException and make whatever we caught // the inner exception of it. throw EntityUtil.CommandCompilation(System.Data.Entity.Strings.EntityClient_CommandDefinitionPreparationFailed, e); } throw; } if (definition == null) { throw EntityUtil.ProviderDoesNotSupportCommandTrees(); } EntityCommandDefinition entityDefinition = (EntityCommandDefinition)definition; QueryCacheManager cacheManager = context.Perspective.MetadataWorkspace.GetQueryCacheManager(); ShaperFactory shaperFactory = ShaperFactory.Create(elementType, cacheManager, entityDefinition.CreateColumnMap(null), context.MetadataWorkspace, spanInfo, mergeOption, false); // attempt to determine entity information for this query (e.g. which entity type and which entity set) //EntityType rootEntityType = null; EntitySet singleEntitySet = null; if (treeResultType.EdmType.BuiltInTypeKind == BuiltInTypeKind.CollectionType) { // determine if the entity set is unambiguous given the entity type if (null != entityDefinition.EntitySets) { foreach (EntitySet entitySet in entityDefinition.EntitySets) { if (null != entitySet) { if (entitySet.ElementType.IsAssignableFrom(((CollectionType)treeResultType.EdmType).TypeUsage.EdmType)) { if (singleEntitySet == null) { // found a single match singleEntitySet = entitySet; } else { // there's more than one matching entity set singleEntitySet = null; break; } } } } } } return new ObjectQueryExecutionPlan(definition, shaperFactory, treeResultType, mergeOption, singleEntitySet); } internal string ToTraceString() { string traceString = string.Empty; EntityCommandDefinition entityCommandDef = this.CommandDefinition as EntityCommandDefinition; if (entityCommandDef != null) { traceString = entityCommandDef.ToTraceString(); } return traceString; } internal ObjectResultExecute (ObjectContext context, ObjectParameterCollection parameterValues) { DbDataReader storeReader = null; try { // create entity command (just do this to snarf store command) EntityCommandDefinition commandDefinition = (EntityCommandDefinition)this.CommandDefinition; EntityCommand entityCommand = new EntityCommand((EntityConnection)context.Connection, commandDefinition); // pass through parameters and timeout values if (context.CommandTimeout.HasValue) { entityCommand.CommandTimeout = context.CommandTimeout.Value; } if (parameterValues != null) { foreach (ObjectParameter parameter in parameterValues) { int index = entityCommand.Parameters.IndexOf(parameter.Name); if (index != -1) { entityCommand.Parameters[index].Value = parameter.Value ?? DBNull.Value; } } } // acquire store reader storeReader = commandDefinition.ExecuteStoreCommands(entityCommand, CommandBehavior.Default); ShaperFactory shaperFactory = (ShaperFactory )this.ResultShaperFactory; Shaper shaper = shaperFactory.Create(storeReader, context, context.MetadataWorkspace, this.MergeOption); // create materializer delegate TypeUsage resultItemEdmType; if (ResultType.EdmType.BuiltInTypeKind == BuiltInTypeKind.CollectionType) { resultItemEdmType = ((CollectionType)ResultType.EdmType).TypeUsage; } else { resultItemEdmType = ResultType; } return new ObjectResult (shaper, this._singleEntitySet, resultItemEdmType); } catch (Exception) { if (null != storeReader) { // Note: The caller is responsible for disposing reader if creating // the enumerator fails. storeReader.Dispose(); } throw; } } internal static ObjectResult ExecuteCommandTree (ObjectContext context, DbQueryCommandTree query, MergeOption mergeOption) { Debug.Assert(context != null, "ObjectContext cannot be null"); Debug.Assert(query != null, "Command tree cannot be null"); ObjectQueryExecutionPlan execPlan = ObjectQueryExecutionPlan.Prepare(context, query, typeof(TResultType), mergeOption, null); return execPlan.Execute (context, null); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Objects.Internal { using System; using System.Collections.Generic; using System.Data.Common; using System.Data.Common.CommandTrees; using System.Data.Common.EntitySql; using System.Data.Common.QueryCache; using System.Data.EntityClient; using System.Data.Metadata.Edm; using System.Data.Objects; using System.Data.Objects.DataClasses; using System.Diagnostics; using System.Reflection; using System.Linq.Expressions; using System.Data.Common.Internal.Materialization; using System.Data.Query.InternalTrees; ////// Represents the 'compiled' form of all elements (query + result assembly) required to execute a specific internal sealed class ObjectQueryExecutionPlan { internal readonly DbCommandDefinition CommandDefinition; internal readonly ShaperFactory ResultShaperFactory; internal readonly TypeUsage ResultType; internal readonly MergeOption MergeOption; ////// If the query yields entities from a single entity set, the value is stored here. private readonly EntitySet _singleEntitySet; internal ObjectQueryExecutionPlan(DbCommandDefinition commandDefinition, ShaperFactory resultShaperFactory, TypeUsage resultType, MergeOption mergeOption, EntitySet singleEntitySet) { Debug.Assert(commandDefinition != null, "A command definition is required"); Debug.Assert(resultShaperFactory != null, "A result shaper factory is required"); Debug.Assert(resultType != null, "A result type is required"); this.CommandDefinition = commandDefinition; this.ResultShaperFactory = resultShaperFactory; this.ResultType = resultType; this.MergeOption = mergeOption; this._singleEntitySet = singleEntitySet; } internal static ObjectQueryExecutionPlan Prepare(ObjectContext context, DbQueryCommandTree tree, Type elementType, MergeOption mergeOption, Span span) { TypeUsage treeResultType = tree.Query.ResultType; // Rewrite this tree for Span? DbExpression spannedQuery = null; SpanIndex spanInfo; if (ObjectSpanRewriter.TryRewrite(tree.Query, span, mergeOption, out spannedQuery, out spanInfo)) { tree.Query = spannedQuery; } else { spanInfo = null; } DbConnection connection = context.Connection; DbCommandDefinition definition = null; // The connection is required to get to the CommandDefinition builder. if (connection == null) { throw EntityUtil.InvalidOperation(System.Data.Entity.Strings.ObjectQuery_InvalidConnection); } DbProviderServices services = DbProviderServices.GetProviderServices(connection); try { definition = services.CreateCommandDefinition(tree); } catch (EntityCommandCompilationException) { // If we're running against EntityCommand, we probably already caught the providers' // exception and wrapped it, we don't want to do that again, so we'll just rethrow // here instead. throw; } catch (Exception e) { // we should not be wrapping all exceptions if (EntityUtil.IsCatchableExceptionType(e)) { // we don't wan't folks to have to know all the various types of exceptions that can // occur, so we just rethrow a CommandDefinitionException and make whatever we caught // the inner exception of it. throw EntityUtil.CommandCompilation(System.Data.Entity.Strings.EntityClient_CommandDefinitionPreparationFailed, e); } throw; } if (definition == null) { throw EntityUtil.ProviderDoesNotSupportCommandTrees(); } EntityCommandDefinition entityDefinition = (EntityCommandDefinition)definition; QueryCacheManager cacheManager = context.Perspective.MetadataWorkspace.GetQueryCacheManager(); ShaperFactory shaperFactory = ShaperFactory.Create(elementType, cacheManager, entityDefinition.CreateColumnMap(null), context.MetadataWorkspace, spanInfo, mergeOption, false); // attempt to determine entity information for this query (e.g. which entity type and which entity set) //EntityType rootEntityType = null; EntitySet singleEntitySet = null; if (treeResultType.EdmType.BuiltInTypeKind == BuiltInTypeKind.CollectionType) { // determine if the entity set is unambiguous given the entity type if (null != entityDefinition.EntitySets) { foreach (EntitySet entitySet in entityDefinition.EntitySets) { if (null != entitySet) { if (entitySet.ElementType.IsAssignableFrom(((CollectionType)treeResultType.EdmType).TypeUsage.EdmType)) { if (singleEntitySet == null) { // found a single match singleEntitySet = entitySet; } else { // there's more than one matching entity set singleEntitySet = null; break; } } } } } } return new ObjectQueryExecutionPlan(definition, shaperFactory, treeResultType, mergeOption, singleEntitySet); } internal string ToTraceString() { string traceString = string.Empty; EntityCommandDefinition entityCommandDef = this.CommandDefinition as EntityCommandDefinition; if (entityCommandDef != null) { traceString = entityCommandDef.ToTraceString(); } return traceString; } internal ObjectResultExecute (ObjectContext context, ObjectParameterCollection parameterValues) { DbDataReader storeReader = null; try { // create entity command (just do this to snarf store command) EntityCommandDefinition commandDefinition = (EntityCommandDefinition)this.CommandDefinition; EntityCommand entityCommand = new EntityCommand((EntityConnection)context.Connection, commandDefinition); // pass through parameters and timeout values if (context.CommandTimeout.HasValue) { entityCommand.CommandTimeout = context.CommandTimeout.Value; } if (parameterValues != null) { foreach (ObjectParameter parameter in parameterValues) { int index = entityCommand.Parameters.IndexOf(parameter.Name); if (index != -1) { entityCommand.Parameters[index].Value = parameter.Value ?? DBNull.Value; } } } // acquire store reader storeReader = commandDefinition.ExecuteStoreCommands(entityCommand, CommandBehavior.Default); ShaperFactory shaperFactory = (ShaperFactory )this.ResultShaperFactory; Shaper shaper = shaperFactory.Create(storeReader, context, context.MetadataWorkspace, this.MergeOption); // create materializer delegate TypeUsage resultItemEdmType; if (ResultType.EdmType.BuiltInTypeKind == BuiltInTypeKind.CollectionType) { resultItemEdmType = ((CollectionType)ResultType.EdmType).TypeUsage; } else { resultItemEdmType = ResultType; } return new ObjectResult (shaper, this._singleEntitySet, resultItemEdmType); } catch (Exception) { if (null != storeReader) { // Note: The caller is responsible for disposing reader if creating // the enumerator fails. storeReader.Dispose(); } throw; } } internal static ObjectResult ExecuteCommandTree (ObjectContext context, DbQueryCommandTree query, MergeOption mergeOption) { Debug.Assert(context != null, "ObjectContext cannot be null"); Debug.Assert(query != null, "Command tree cannot be null"); ObjectQueryExecutionPlan execPlan = ObjectQueryExecutionPlan.Prepare(context, query, typeof(TResultType), mergeOption, null); return execPlan.Execute (context, null); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
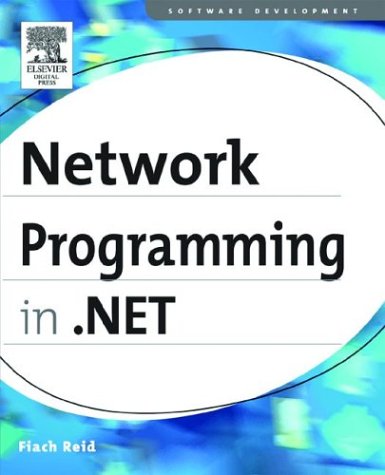
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NavigationService.cs
- TrackingStringDictionary.cs
- MetadataCacheItem.cs
- XamlClipboardData.cs
- CipherData.cs
- EntityObject.cs
- AssemblyAssociatedContentFileAttribute.cs
- FormViewDeletedEventArgs.cs
- StringStorage.cs
- CustomValidator.cs
- Configuration.cs
- InputBuffer.cs
- AutomationElementCollection.cs
- LambdaCompiler.Statements.cs
- Brush.cs
- FileLevelControlBuilderAttribute.cs
- SynchronizedDispatch.cs
- ExeContext.cs
- RoutedPropertyChangedEventArgs.cs
- HtmlControlPersistable.cs
- Win32KeyboardDevice.cs
- basevalidator.cs
- AliasExpr.cs
- ProtocolElement.cs
- PersistenceProviderBehavior.cs
- LinqDataSourceStatusEventArgs.cs
- SignedPkcs7.cs
- VirtualPath.cs
- TypeProvider.cs
- TextRunTypographyProperties.cs
- DataComponentGenerator.cs
- SafeFileHandle.cs
- SearchForVirtualItemEventArgs.cs
- PolyQuadraticBezierSegment.cs
- ExplicitDiscriminatorMap.cs
- BitmapEffectGroup.cs
- errorpatternmatcher.cs
- SelectedGridItemChangedEvent.cs
- LinqDataSource.cs
- Permission.cs
- WarningException.cs
- OuterGlowBitmapEffect.cs
- ListView.cs
- Converter.cs
- MruCache.cs
- PathFigureCollectionConverter.cs
- FontDriver.cs
- DateTimeFormatInfoScanner.cs
- StringValidatorAttribute.cs
- EventLogRecord.cs
- PagesSection.cs
- DataGrid.cs
- WebColorConverter.cs
- RowsCopiedEventArgs.cs
- ListBoxItem.cs
- Constraint.cs
- BaseValidatorDesigner.cs
- CodeDirectoryCompiler.cs
- BooleanFunctions.cs
- TextTreeInsertElementUndoUnit.cs
- LayoutDump.cs
- CryptoApi.cs
- SafeSecurityHandles.cs
- DataGridViewDataConnection.cs
- RegexParser.cs
- Configuration.cs
- SqlNodeAnnotations.cs
- ActivityUtilities.cs
- ErrorProvider.cs
- JsonFormatReaderGenerator.cs
- MachineKeySection.cs
- DeclarationUpdate.cs
- WebSysDefaultValueAttribute.cs
- XmlNodeChangedEventArgs.cs
- ResourceIDHelper.cs
- XhtmlConformanceSection.cs
- WithStatement.cs
- AnnotationMap.cs
- WebControlParameterProxy.cs
- SymmetricSecurityProtocolFactory.cs
- TextSelection.cs
- SqlMethodTransformer.cs
- BaseCollection.cs
- DataGridViewCellConverter.cs
- TailCallAnalyzer.cs
- EventMemberCodeDomSerializer.cs
- HostProtectionException.cs
- FontDialog.cs
- ControlType.cs
- recordstate.cs
- SignedPkcs7.cs
- StorageInfo.cs
- SqlDataSourceSelectingEventArgs.cs
- SafeEventHandle.cs
- SqlMethodCallConverter.cs
- HtmlInputHidden.cs
- PostBackTrigger.cs
- ToolStripDropDownMenu.cs
- ImageDrawing.cs
- GcHandle.cs