Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / MS / Internal / PtsHost / PageVisual.cs / 1 / PageVisual.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: PageVisual.cs // // Description: Visual representing a PTS page. // // History: // 11/11/2003 : grzegorz - created. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Windows; using System.Windows.Controls; using System.Windows.Media; using System.Windows.Threading; namespace MS.Internal.PtsHost { // --------------------------------------------------------------------- // Visual representing a PTS page. // --------------------------------------------------------------------- internal class PageVisual : DrawingVisual, IContentHost { // ------------------------------------------------------------------ // Create a visual representing a PTS page. // ----------------------------------------------------------------- internal PageVisual(FlowDocumentPage owner) { _owner = new WeakReference(owner); } // ------------------------------------------------------------------ // Set information about background that is necessary for rendering // process. // // backgroundBrush - The background brush used for background. // renderBounds - Render bounds of the visual. // ------------------------------------------------------------------ internal void DrawBackground(Brush backgroundBrush, Rect renderBounds) { if (_backgroundBrush != backgroundBrush || _renderBounds != renderBounds) { _backgroundBrush = backgroundBrush; _renderBounds = renderBounds; // Open DrawingContext and draw background. // If background is not set, Open will clean the render data, but it // will preserve visual children. using (DrawingContext dc = RenderOpen()) { if (_backgroundBrush != null) { dc.DrawRectangle(_backgroundBrush, null, _renderBounds); } else { dc.DrawRectangle(Brushes.Transparent, null, _renderBounds); } } } } // ----------------------------------------------------------------- // Get/Set visual child. // ------------------------------------------------------------------ internal Visual Child { get { VisualCollection vc = this.Children; Debug.Assert(vc.Count <= 1); return (vc.Count == 0) ? null : vc[0]; } set { VisualCollection vc = this.Children; Debug.Assert(vc.Count <= 1); if (vc.Count == 0) { vc.Add(value); } else if (vc[0] != value) { vc[0] = value; } // else Visual child is the same as already stored; do nothing. } } // ----------------------------------------------------------------- // Clear its DrawingContext // Opening and closing a DrawingContext, clears it. // ----------------------------------------------------------------- internal void ClearDrawingContext() { DrawingContext ctx = this.RenderOpen(); if(ctx != null) ctx.Close(); } //------------------------------------------------------------------- // // IContentHost Members // //-------------------------------------------------------------------- #region IContentHost Members ////// IInputElement IContentHost.InputHitTest(Point point) { IContentHost host = _owner.Target as IContentHost; if (host != null) { return host.InputHitTest(point); } return null; } ////// /// ReadOnlyCollection/// IContentHost.GetRectangles(ContentElement child) { IContentHost host = _owner.Target as IContentHost; if (host != null) { return host.GetRectangles(child); } return new ReadOnlyCollection (new List (0)); } /// /// IEnumerator/// IContentHost.HostedElements { get { IContentHost host = _owner.Target as IContentHost; if (host != null) { return host.HostedElements; } return null; } } /// /// void IContentHost.OnChildDesiredSizeChanged(UIElement child) { IContentHost host = _owner.Target as IContentHost; if (host != null) { host.OnChildDesiredSizeChanged(child); } } #endregion IContentHost Members // ----------------------------------------------------------------- // Reference to DocumentPage that owns this visual. // ------------------------------------------------------------------ private readonly WeakReference _owner; // ------------------------------------------------------------------ // Brush used for background rendering. // ----------------------------------------------------------------- private Brush _backgroundBrush; // ------------------------------------------------------------------ // Render bounds. // ----------------------------------------------------------------- private Rect _renderBounds; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: PageVisual.cs // // Description: Visual representing a PTS page. // // History: // 11/11/2003 : grzegorz - created. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Windows; using System.Windows.Controls; using System.Windows.Media; using System.Windows.Threading; namespace MS.Internal.PtsHost { // --------------------------------------------------------------------- // Visual representing a PTS page. // --------------------------------------------------------------------- internal class PageVisual : DrawingVisual, IContentHost { // ------------------------------------------------------------------ // Create a visual representing a PTS page. // ----------------------------------------------------------------- internal PageVisual(FlowDocumentPage owner) { _owner = new WeakReference(owner); } // ------------------------------------------------------------------ // Set information about background that is necessary for rendering // process. // // backgroundBrush - The background brush used for background. // renderBounds - Render bounds of the visual. // ------------------------------------------------------------------ internal void DrawBackground(Brush backgroundBrush, Rect renderBounds) { if (_backgroundBrush != backgroundBrush || _renderBounds != renderBounds) { _backgroundBrush = backgroundBrush; _renderBounds = renderBounds; // Open DrawingContext and draw background. // If background is not set, Open will clean the render data, but it // will preserve visual children. using (DrawingContext dc = RenderOpen()) { if (_backgroundBrush != null) { dc.DrawRectangle(_backgroundBrush, null, _renderBounds); } else { dc.DrawRectangle(Brushes.Transparent, null, _renderBounds); } } } } // ----------------------------------------------------------------- // Get/Set visual child. // ------------------------------------------------------------------ internal Visual Child { get { VisualCollection vc = this.Children; Debug.Assert(vc.Count <= 1); return (vc.Count == 0) ? null : vc[0]; } set { VisualCollection vc = this.Children; Debug.Assert(vc.Count <= 1); if (vc.Count == 0) { vc.Add(value); } else if (vc[0] != value) { vc[0] = value; } // else Visual child is the same as already stored; do nothing. } } // ----------------------------------------------------------------- // Clear its DrawingContext // Opening and closing a DrawingContext, clears it. // ----------------------------------------------------------------- internal void ClearDrawingContext() { DrawingContext ctx = this.RenderOpen(); if(ctx != null) ctx.Close(); } //------------------------------------------------------------------- // // IContentHost Members // //-------------------------------------------------------------------- #region IContentHost Members ////// /// IInputElement IContentHost.InputHitTest(Point point) { IContentHost host = _owner.Target as IContentHost; if (host != null) { return host.InputHitTest(point); } return null; } ////// /// ReadOnlyCollection/// IContentHost.GetRectangles(ContentElement child) { IContentHost host = _owner.Target as IContentHost; if (host != null) { return host.GetRectangles(child); } return new ReadOnlyCollection (new List (0)); } /// /// IEnumerator/// IContentHost.HostedElements { get { IContentHost host = _owner.Target as IContentHost; if (host != null) { return host.HostedElements; } return null; } } /// /// void IContentHost.OnChildDesiredSizeChanged(UIElement child) { IContentHost host = _owner.Target as IContentHost; if (host != null) { host.OnChildDesiredSizeChanged(child); } } #endregion IContentHost Members // ----------------------------------------------------------------- // Reference to DocumentPage that owns this visual. // ------------------------------------------------------------------ private readonly WeakReference _owner; // ------------------------------------------------------------------ // Brush used for background rendering. // ----------------------------------------------------------------- private Brush _backgroundBrush; // ------------------------------------------------------------------ // Render bounds. // ----------------------------------------------------------------- private Rect _renderBounds; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.///
Link Menu
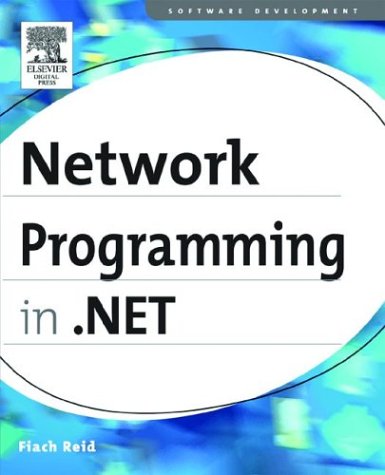
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AlgoModule.cs
- InheritedPropertyChangedEventArgs.cs
- PageCodeDomTreeGenerator.cs
- XamlFigureLengthSerializer.cs
- PartBasedPackageProperties.cs
- Bits.cs
- CellParaClient.cs
- InstanceHandle.cs
- DataGridParentRows.cs
- Debugger.cs
- QilFunction.cs
- LiteralSubsegment.cs
- RecordManager.cs
- Signature.cs
- ProcessThreadCollection.cs
- TypeUtil.cs
- ValueSerializer.cs
- KeyValueSerializer.cs
- XmlnsCache.cs
- TextPointer.cs
- ModulesEntry.cs
- DeferredReference.cs
- OrderedDictionary.cs
- _Semaphore.cs
- _HeaderInfo.cs
- Color.cs
- NamespaceDecl.cs
- FormatException.cs
- Model3DGroup.cs
- SerializerDescriptor.cs
- Stylesheet.cs
- ArrayWithOffset.cs
- ExecutionTracker.cs
- WriteableBitmap.cs
- XamlSerializer.cs
- SocketInformation.cs
- SplitContainerDesigner.cs
- ProviderIncompatibleException.cs
- CodeObject.cs
- SqlRecordBuffer.cs
- InvalidCastException.cs
- EntityDataSourceSelectingEventArgs.cs
- OdbcTransaction.cs
- MimePart.cs
- ThreadAbortException.cs
- TextElementCollectionHelper.cs
- ProtocolViolationException.cs
- WebPartAddingEventArgs.cs
- TdsValueSetter.cs
- Transform.cs
- SqlCacheDependencyDatabaseCollection.cs
- GlyphsSerializer.cs
- ExpandCollapsePattern.cs
- GroupStyle.cs
- XhtmlStyleClass.cs
- QilStrConcat.cs
- MenuCommandService.cs
- ResourceManager.cs
- OleDbError.cs
- AssemblyInfo.cs
- ServiceDescriptionSerializer.cs
- FormsAuthenticationConfiguration.cs
- CryptoApi.cs
- GridViewColumnHeader.cs
- ConstantExpression.cs
- SubclassTypeValidator.cs
- COM2PictureConverter.cs
- CharacterBufferReference.cs
- StreamInfo.cs
- SafeLibraryHandle.cs
- Accessible.cs
- ScriptRegistrationManager.cs
- FrameSecurityDescriptor.cs
- SqlProviderServices.cs
- EntryPointNotFoundException.cs
- ToolStripStatusLabel.cs
- ObjectDataSourceDisposingEventArgs.cs
- OracleRowUpdatedEventArgs.cs
- StrokeRenderer.cs
- listitem.cs
- QilTargetType.cs
- DataGridTextBoxColumn.cs
- NativeRightsManagementAPIsStructures.cs
- _TransmitFileOverlappedAsyncResult.cs
- TextDocumentView.cs
- ThrowHelper.cs
- ToolStripContentPanel.cs
- MediaContext.cs
- CodeArrayCreateExpression.cs
- RuntimeCompatibilityAttribute.cs
- DataGridViewMethods.cs
- XmlObjectSerializerReadContextComplexJson.cs
- XamlTreeBuilderBamlRecordWriter.cs
- OptimalBreakSession.cs
- EntityTypeEmitter.cs
- AuthorizationRule.cs
- ToolStripManager.cs
- ShimAsPublicXamlType.cs
- PluggableProtocol.cs
- DefaultAutoFieldGenerator.cs