Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / ComponentModel / COM2Interop / COM2PictureConverter.cs / 1305376 / COM2PictureConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.ComponentModel.Com2Interop { using System.Runtime.Serialization.Formatters; using System.ComponentModel; using System.Diagnostics; using System; using System.Drawing; using System.Collections; using Microsoft.Win32; using System.Runtime.Versioning; ////// /// This class maps an IPicture to a System.Drawing.Image. /// internal class Com2PictureConverter : Com2DataTypeToManagedDataTypeConverter { object lastManaged; IntPtr lastNativeHandle; WeakReference pictureRef; IntPtr lastPalette = IntPtr.Zero; Type pictureType = typeof(Bitmap); public Com2PictureConverter(Com2PropertyDescriptor pd) { if (pd.DISPID == NativeMethods.ActiveX.DISPID_MOUSEICON || pd.Name.IndexOf("Icon") != -1) { pictureType = typeof(Icon); } } ////// /// Returns the managed type that this editor maps the property type to. /// public override Type ManagedType { get { return pictureType; } } ////// /// Converts the native value into a managed value /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public override object ConvertNativeToManaged(object nativeValue, Com2PropertyDescriptor pd) { if (nativeValue == null) { return null; } Debug.Assert(nativeValue is UnsafeNativeMethods.IPicture, "nativevalue is not IPicture"); UnsafeNativeMethods.IPicture nativePicture = (UnsafeNativeMethods.IPicture)nativeValue; IntPtr handle = nativePicture.GetHandle(); if (lastManaged != null && handle == lastNativeHandle) { return lastManaged; } lastNativeHandle = handle; //lastPalette = nativePicture.GetHPal(); if (handle != IntPtr.Zero) { switch (nativePicture.GetPictureType()) { case NativeMethods.Ole.PICTYPE_ICON: pictureType = typeof(Icon); lastManaged = Icon.FromHandle(handle); break; case NativeMethods.Ole.PICTYPE_BITMAP: pictureType = typeof(Bitmap); lastManaged = Image.FromHbitmap(handle); break; default: Debug.Fail("Unknown picture type"); break; } pictureRef = new WeakReference(nativePicture); } else { lastManaged = null; pictureRef = null; } return lastManaged; } ////// /// Converts the managed value into a native value /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public override object ConvertManagedToNative(object managedValue, Com2PropertyDescriptor pd, ref bool cancelSet) { // don't cancel the set cancelSet = false; if (lastManaged != null && lastManaged.Equals(managedValue) && pictureRef != null && pictureRef.IsAlive) { return pictureRef.Target; } // we have to build an IPicture lastManaged = managedValue; if (managedValue != null) { Guid g = typeof(UnsafeNativeMethods.IPicture).GUID; NativeMethods.PICTDESC pictdesc = null; bool own = false; if (lastManaged is Icon) { pictdesc = NativeMethods.PICTDESC.CreateIconPICTDESC(((Icon)lastManaged).Handle); } else if (lastManaged is Bitmap) { pictdesc = NativeMethods.PICTDESC.CreateBitmapPICTDESC(((Bitmap)lastManaged).GetHbitmap(), lastPalette); own = true; } else { Debug.Fail("Unknown Image type: " + managedValue.GetType().Name); } UnsafeNativeMethods.IPicture pict = UnsafeNativeMethods.OleCreatePictureIndirect(pictdesc, ref g, own); lastNativeHandle = pict.GetHandle(); pictureRef = new WeakReference(pict); return pict; } else { lastManaged = null; lastNativeHandle = lastPalette = IntPtr.Zero; pictureRef = null; return null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.ComponentModel.Com2Interop { using System.Runtime.Serialization.Formatters; using System.ComponentModel; using System.Diagnostics; using System; using System.Drawing; using System.Collections; using Microsoft.Win32; using System.Runtime.Versioning; ////// /// This class maps an IPicture to a System.Drawing.Image. /// internal class Com2PictureConverter : Com2DataTypeToManagedDataTypeConverter { object lastManaged; IntPtr lastNativeHandle; WeakReference pictureRef; IntPtr lastPalette = IntPtr.Zero; Type pictureType = typeof(Bitmap); public Com2PictureConverter(Com2PropertyDescriptor pd) { if (pd.DISPID == NativeMethods.ActiveX.DISPID_MOUSEICON || pd.Name.IndexOf("Icon") != -1) { pictureType = typeof(Icon); } } ////// /// Returns the managed type that this editor maps the property type to. /// public override Type ManagedType { get { return pictureType; } } ////// /// Converts the native value into a managed value /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public override object ConvertNativeToManaged(object nativeValue, Com2PropertyDescriptor pd) { if (nativeValue == null) { return null; } Debug.Assert(nativeValue is UnsafeNativeMethods.IPicture, "nativevalue is not IPicture"); UnsafeNativeMethods.IPicture nativePicture = (UnsafeNativeMethods.IPicture)nativeValue; IntPtr handle = nativePicture.GetHandle(); if (lastManaged != null && handle == lastNativeHandle) { return lastManaged; } lastNativeHandle = handle; //lastPalette = nativePicture.GetHPal(); if (handle != IntPtr.Zero) { switch (nativePicture.GetPictureType()) { case NativeMethods.Ole.PICTYPE_ICON: pictureType = typeof(Icon); lastManaged = Icon.FromHandle(handle); break; case NativeMethods.Ole.PICTYPE_BITMAP: pictureType = typeof(Bitmap); lastManaged = Image.FromHbitmap(handle); break; default: Debug.Fail("Unknown picture type"); break; } pictureRef = new WeakReference(nativePicture); } else { lastManaged = null; pictureRef = null; } return lastManaged; } ////// /// Converts the managed value into a native value /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public override object ConvertManagedToNative(object managedValue, Com2PropertyDescriptor pd, ref bool cancelSet) { // don't cancel the set cancelSet = false; if (lastManaged != null && lastManaged.Equals(managedValue) && pictureRef != null && pictureRef.IsAlive) { return pictureRef.Target; } // we have to build an IPicture lastManaged = managedValue; if (managedValue != null) { Guid g = typeof(UnsafeNativeMethods.IPicture).GUID; NativeMethods.PICTDESC pictdesc = null; bool own = false; if (lastManaged is Icon) { pictdesc = NativeMethods.PICTDESC.CreateIconPICTDESC(((Icon)lastManaged).Handle); } else if (lastManaged is Bitmap) { pictdesc = NativeMethods.PICTDESC.CreateBitmapPICTDESC(((Bitmap)lastManaged).GetHbitmap(), lastPalette); own = true; } else { Debug.Fail("Unknown Image type: " + managedValue.GetType().Name); } UnsafeNativeMethods.IPicture pict = UnsafeNativeMethods.OleCreatePictureIndirect(pictdesc, ref g, own); lastNativeHandle = pict.GetHandle(); pictureRef = new WeakReference(pict); return pict; } else { lastManaged = null; lastNativeHandle = lastPalette = IntPtr.Zero; pictureRef = null; return null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
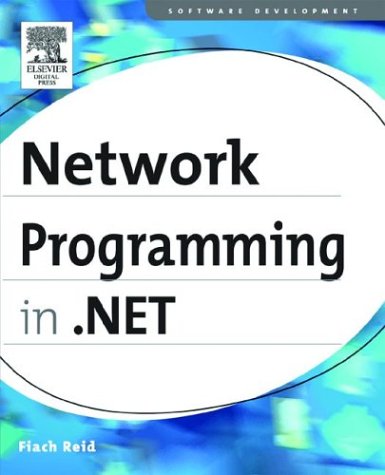
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RectangleConverter.cs
- PersonalizationStateQuery.cs
- SymmetricKey.cs
- TrackingServices.cs
- Point4D.cs
- ChangePasswordDesigner.cs
- MethodAccessException.cs
- StructuredTypeEmitter.cs
- HttpRuntime.cs
- ApplicationCommands.cs
- LineSegment.cs
- Pen.cs
- ManagementQuery.cs
- CustomCategoryAttribute.cs
- EntityClientCacheKey.cs
- remotingproxy.cs
- HttpsTransportBindingElement.cs
- SortExpressionBuilder.cs
- TimeZone.cs
- SamlConstants.cs
- ItemContainerPattern.cs
- ClientProxyGenerator.cs
- MethodToken.cs
- SystemPens.cs
- BoundField.cs
- FtpWebResponse.cs
- ObjectSecurity.cs
- _KerberosClient.cs
- BrowserCapabilitiesFactoryBase.cs
- ClassDataContract.cs
- InkPresenter.cs
- TypeValidationEventArgs.cs
- NameObjectCollectionBase.cs
- ResourceSetExpression.cs
- MailDefinition.cs
- Selection.cs
- SafeArchiveContext.cs
- BStrWrapper.cs
- TextRangeAdaptor.cs
- CustomValidator.cs
- BuildProviderUtils.cs
- ParentQuery.cs
- ListItem.cs
- OrderedDictionary.cs
- WebPartExportVerb.cs
- AuthenticationManager.cs
- TimelineCollection.cs
- ProfilePropertySettings.cs
- NamespaceMapping.cs
- UserInitiatedRoutedEventPermission.cs
- DynamicValidatorEventArgs.cs
- ValidatedControlConverter.cs
- StateRuntime.cs
- NetworkAddressChange.cs
- CodePropertyReferenceExpression.cs
- DataGridViewTextBoxColumn.cs
- CapiSafeHandles.cs
- InternalTypeHelper.cs
- TableRowCollection.cs
- MessageQueueCriteria.cs
- FormatterServices.cs
- HelpProvider.cs
- MsmqIntegrationBinding.cs
- Deserializer.cs
- WebConvert.cs
- HebrewCalendar.cs
- OdbcParameter.cs
- CryptoKeySecurity.cs
- SqlCacheDependencySection.cs
- validationstate.cs
- EventRoute.cs
- PassportPrincipal.cs
- TreeNode.cs
- HandlerFactoryWrapper.cs
- SqlProcedureAttribute.cs
- MemberInfoSerializationHolder.cs
- TdsValueSetter.cs
- TextEditorDragDrop.cs
- DesignTimeHTMLTextWriter.cs
- TimeSpanStorage.cs
- BufferedGraphicsContext.cs
- DefaultValidator.cs
- WebEventTraceProvider.cs
- ProfileService.cs
- TimeSpanValidatorAttribute.cs
- OptionUsage.cs
- ResourceProviderFactory.cs
- MailHeaderInfo.cs
- InOutArgumentConverter.cs
- OracleLob.cs
- ColorMatrix.cs
- CodeTypeMemberCollection.cs
- ScrollChangedEventArgs.cs
- SwitchLevelAttribute.cs
- NotifyParentPropertyAttribute.cs
- PresentationSource.cs
- MenuItemCollectionEditorDialog.cs
- QilTernary.cs
- MaskedTextBoxTextEditorDropDown.cs
- JsonEnumDataContract.cs