Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / Tokens / SymmetricKey.cs / 1305376 / SymmetricKey.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.IdentityModel.Tokens { using System.IdentityModel.Selectors; using System.Security.Cryptography; public class InMemorySymmetricSecurityKey : SymmetricSecurityKey { int keySize; byte[] symmetricKey; public InMemorySymmetricSecurityKey(byte[] symmetricKey) : this(symmetricKey, true) { } public InMemorySymmetricSecurityKey(byte[] symmetricKey, bool cloneBuffer) { if (symmetricKey == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("symmetricKey")); } if (symmetricKey.Length == 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentException(SR.GetString(SR.SymmetricKeyLengthTooShort, symmetricKey.Length))); } this.keySize = symmetricKey.Length * 8; if (cloneBuffer) { this.symmetricKey = new byte[symmetricKey.Length]; Buffer.BlockCopy(symmetricKey, 0, this.symmetricKey, 0, symmetricKey.Length); } else { this.symmetricKey = symmetricKey; } } public override int KeySize { get { return this.keySize; } } public override byte[] DecryptKey(string algorithm, byte[] keyData) { return CryptoHelper.UnwrapKey(this.symmetricKey, keyData, algorithm); } public override byte[] EncryptKey(string algorithm, byte[] keyData) { return CryptoHelper.WrapKey(this.symmetricKey, keyData, algorithm); } public override byte[] GenerateDerivedKey(string algorithm, byte[] label, byte[] nonce, int derivedKeyLength, int offset) { return CryptoHelper.GenerateDerivedKey(this.symmetricKey, algorithm, label, nonce, derivedKeyLength, offset); } public override ICryptoTransform GetDecryptionTransform(string algorithm, byte[] iv) { return CryptoHelper.CreateDecryptor(this.symmetricKey, iv, algorithm); } public override ICryptoTransform GetEncryptionTransform(string algorithm, byte[] iv) { return CryptoHelper.CreateEncryptor(this.symmetricKey, iv, algorithm); } public override int GetIVSize(string algorithm) { return CryptoHelper.GetIVSize(algorithm); } public override KeyedHashAlgorithm GetKeyedHashAlgorithm(string algorithm) { return CryptoHelper.CreateKeyedHashAlgorithm(this.symmetricKey, algorithm); } public override SymmetricAlgorithm GetSymmetricAlgorithm(string algorithm) { return CryptoHelper.GetSymmetricAlgorithm(this.symmetricKey, algorithm); } public override byte[] GetSymmetricKey() { byte[] local = new byte[this.symmetricKey.Length]; Buffer.BlockCopy(this.symmetricKey, 0, local, 0, this.symmetricKey.Length); return local; } public override bool IsAsymmetricAlgorithm(string algorithm) { return (CryptoHelper.IsAsymmetricAlgorithm(algorithm)); } public override bool IsSupportedAlgorithm(string algorithm) { return (CryptoHelper.IsSymmetricSupportedAlgorithm(algorithm, this.KeySize)); } public override bool IsSymmetricAlgorithm(string algorithm) { return CryptoHelper.IsSymmetricAlgorithm(algorithm); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
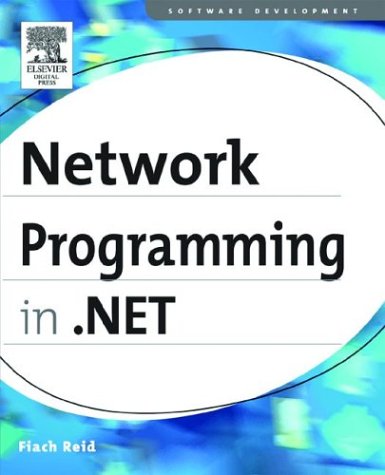
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CultureSpecificCharacterBufferRange.cs
- ObjectDisposedException.cs
- FloaterParagraph.cs
- FreezableCollection.cs
- TransformGroup.cs
- TextLineBreak.cs
- TraceInternal.cs
- ConnectionManagementElementCollection.cs
- _AutoWebProxyScriptHelper.cs
- SimpleType.cs
- DataFieldConverter.cs
- ThemeDirectoryCompiler.cs
- MergeFilterQuery.cs
- ActivationArguments.cs
- PermissionSetEnumerator.cs
- TrustLevel.cs
- MetafileHeaderWmf.cs
- ReadOnlyHierarchicalDataSourceView.cs
- DataSourceCacheDurationConverter.cs
- MaxValueConverter.cs
- EntityCollection.cs
- TextTreeFixupNode.cs
- Aes.cs
- HtmlElement.cs
- SchemaSetCompiler.cs
- DtdParser.cs
- ObjectComplexPropertyMapping.cs
- HttpCookieCollection.cs
- TaskHelper.cs
- LogLogRecordHeader.cs
- AppDomainInfo.cs
- TdsParserStaticMethods.cs
- DateTimeFormatInfo.cs
- PolicyManager.cs
- SystemFonts.cs
- EmissiveMaterial.cs
- ComponentEvent.cs
- cookiecollection.cs
- ConfigurationManager.cs
- ValidationEventArgs.cs
- ValidationEventArgs.cs
- Item.cs
- QueryCacheEntry.cs
- PEFileReader.cs
- ValidatorCompatibilityHelper.cs
- DragCompletedEventArgs.cs
- SqlAliaser.cs
- SortAction.cs
- CodeNamespaceCollection.cs
- _NtlmClient.cs
- DelegateBodyWriter.cs
- HotSpotCollection.cs
- __Filters.cs
- RijndaelManaged.cs
- DataBoundControlAdapter.cs
- ThicknessKeyFrameCollection.cs
- RC2CryptoServiceProvider.cs
- TableParaClient.cs
- DesignerResources.cs
- StateDesignerConnector.cs
- AssertFilter.cs
- SmtpClient.cs
- StringComparer.cs
- SimpleWorkerRequest.cs
- EncoderParameters.cs
- IgnoreFileBuildProvider.cs
- DataGridViewRowErrorTextNeededEventArgs.cs
- RegistryExceptionHelper.cs
- XhtmlCssHandler.cs
- FormatVersion.cs
- RichTextBoxConstants.cs
- OutputCacheModule.cs
- RequestBringIntoViewEventArgs.cs
- indexingfiltermarshaler.cs
- BufferModeSettings.cs
- MatcherBuilder.cs
- RoleExceptions.cs
- Drawing.cs
- figurelengthconverter.cs
- QueueAccessMode.cs
- SecurityPolicyVersion.cs
- SpotLight.cs
- ControlsConfig.cs
- FacetChecker.cs
- OledbConnectionStringbuilder.cs
- ControlUtil.cs
- MessageTraceRecord.cs
- DataKeyArray.cs
- Cursor.cs
- StandardBindingReliableSessionElement.cs
- TreeNodeCollectionEditor.cs
- PersonalizationProviderCollection.cs
- documentsequencetextview.cs
- TransportSecurityProtocol.cs
- DataGridDesigner.cs
- SqlAliasesReferenced.cs
- Authorization.cs
- PermissionListSet.cs
- MyContact.cs
- Compiler.cs