Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media3D / SpotLight.cs / 1 / SpotLight.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: 3D spot light implementation. // // See spec at http://avalon/medialayer/Specifications/Avalon3D%20API%20Spec.mht // // History: // 06/18/2003 : t-gregr - Created // //--------------------------------------------------------------------------- using System; using System.Windows.Media; using System.Windows.Media.Composition; using MS.Internal; using System.ComponentModel.Design.Serialization; using System.Windows.Markup; namespace System.Windows.Media.Media3D { ////// The SpotLight derives from PointLightBase as it has a position, range, and attenuation, /// but also adds in a direction and parameters to control the "cone" of the light. /// In order to control the "cone", outerConeAngle (beyond which nothing is illuminated), /// and innerConeAngle (within which everything is fully illuminated) must be specified. /// Lighting between the outside of the inner cone and the outer cone falls off linearly. /// public sealed partial class SpotLight : PointLightBase { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor. /// /// Diffuse color of the new light. /// Position of the new light. /// Direction of the new light. /// Outer cone angle of the new light. /// Inner cone angle of the new light. public SpotLight(Color diffuseColor, Point3D position, Vector3D direction, double outerConeAngle, double innerConeAngle) : this() { // Set PointLightBase properties Color = diffuseColor; Position = position; // Set SpotLight properties Direction = direction; OuterConeAngle = outerConeAngle; InnerConeAngle = innerConeAngle; } ////// Builds a default spotlight shining onto the origin from the (0,0,-1) /// public SpotLight() {} #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: 3D spot light implementation. // // See spec at http://avalon/medialayer/Specifications/Avalon3D%20API%20Spec.mht // // History: // 06/18/2003 : t-gregr - Created // //--------------------------------------------------------------------------- using System; using System.Windows.Media; using System.Windows.Media.Composition; using MS.Internal; using System.ComponentModel.Design.Serialization; using System.Windows.Markup; namespace System.Windows.Media.Media3D { ////// The SpotLight derives from PointLightBase as it has a position, range, and attenuation, /// but also adds in a direction and parameters to control the "cone" of the light. /// In order to control the "cone", outerConeAngle (beyond which nothing is illuminated), /// and innerConeAngle (within which everything is fully illuminated) must be specified. /// Lighting between the outside of the inner cone and the outer cone falls off linearly. /// public sealed partial class SpotLight : PointLightBase { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor. /// /// Diffuse color of the new light. /// Position of the new light. /// Direction of the new light. /// Outer cone angle of the new light. /// Inner cone angle of the new light. public SpotLight(Color diffuseColor, Point3D position, Vector3D direction, double outerConeAngle, double innerConeAngle) : this() { // Set PointLightBase properties Color = diffuseColor; Position = position; // Set SpotLight properties Direction = direction; OuterConeAngle = outerConeAngle; InnerConeAngle = innerConeAngle; } ////// Builds a default spotlight shining onto the origin from the (0,0,-1) /// public SpotLight() {} #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
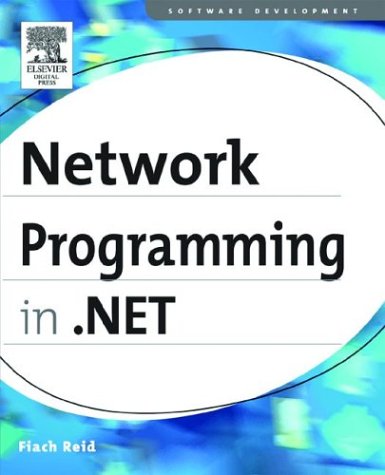
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CmsInterop.cs
- RegistrationServices.cs
- CharUnicodeInfo.cs
- OperatorExpressions.cs
- isolationinterop.cs
- Parsers.cs
- StringUtil.cs
- FormViewUpdatedEventArgs.cs
- DbParameterCollectionHelper.cs
- ValidationPropertyAttribute.cs
- ObjectStateEntryDbDataRecord.cs
- BindUriHelper.cs
- DataSetUtil.cs
- SafeNativeMethods.cs
- FormsIdentity.cs
- SelectionItemPattern.cs
- BezierSegment.cs
- ExecutionContext.cs
- SQlBooleanStorage.cs
- DiffuseMaterial.cs
- OdbcConnectionString.cs
- Parser.cs
- BindingCollection.cs
- EntityClientCacheKey.cs
- Application.cs
- TreeNode.cs
- MenuScrollingVisibilityConverter.cs
- ProtocolsConfigurationEntry.cs
- ResourceCategoryAttribute.cs
- FilterUserControlBase.cs
- HttpCapabilitiesBase.cs
- ContextMenuService.cs
- WrapPanel.cs
- NumberFormatInfo.cs
- RectValueSerializer.cs
- DiscoveryMessageSequence.cs
- HierarchicalDataBoundControlAdapter.cs
- ScriptResourceHandler.cs
- SecondaryIndexList.cs
- CanonicalXml.cs
- Int16.cs
- _PooledStream.cs
- Size3D.cs
- RuntimeArgumentHandle.cs
- SessionPageStatePersister.cs
- CqlParser.cs
- TextServicesCompartment.cs
- NetMsmqBindingElement.cs
- DecoderNLS.cs
- HttpResponseBase.cs
- FormatConvertedBitmap.cs
- DataSourceCache.cs
- PkcsUtils.cs
- BinaryEditor.cs
- SimpleWorkerRequest.cs
- FormatterConverter.cs
- SmiMetaDataProperty.cs
- TransformerConfigurationWizardBase.cs
- Int64KeyFrameCollection.cs
- CallSiteHelpers.cs
- ToolStripItemDesigner.cs
- CommandBinding.cs
- Vector3D.cs
- ClientConfigurationSystem.cs
- TableRowGroupCollection.cs
- DataList.cs
- IResourceProvider.cs
- DispatcherExceptionFilterEventArgs.cs
- ListInitExpression.cs
- RequestResponse.cs
- UInt16Converter.cs
- SendReply.cs
- NotSupportedException.cs
- OdbcConnectionFactory.cs
- HttpRequestCacheValidator.cs
- OletxTransactionManager.cs
- NullPackagingPolicy.cs
- initElementDictionary.cs
- EntityContainerEmitter.cs
- PlatformCulture.cs
- SqlInternalConnection.cs
- MouseActionConverter.cs
- TableStyle.cs
- Soap.cs
- ObjectDataSourceChooseMethodsPanel.cs
- SmtpFailedRecipientsException.cs
- AsymmetricKeyExchangeFormatter.cs
- RectConverter.cs
- StreamInfo.cs
- DataGridViewSelectedColumnCollection.cs
- ManagementScope.cs
- SessionPageStateSection.cs
- ChangeToolStripParentVerb.cs
- RectangleHotSpot.cs
- EdmMember.cs
- HttpConfigurationContext.cs
- XsdValidatingReader.cs
- DefaultTextStoreTextComposition.cs
- NamedElement.cs
- ObjectKeyFrameCollection.cs