Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Shared / MS / Internal / SafeSecurityHelper.cs / 1 / SafeSecurityHelper.cs
/****************************************************************************\ * * File: SafeSecurityHelper.cs * * Purpose: Helper functions that require elevation but are safe to use. * * History: * 10/15/04: [....] Created * * Copyright (C) 2004 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Globalization; using System.Security; using System.Security.Permissions; using System.Reflection; using MS.Win32; using System.Runtime.InteropServices; using Microsoft.Win32; #if PRESENTATIONFRAMEWORK using System.Windows; using System.Windows.Media; #endif // The SafeSecurityHelper class differs between assemblies and could not actually be // shared, so it is duplicated across namespaces to prevent name collision. #if WINDOWS_BASE namespace MS.Internal.WindowsBase #elif PRESENTATION_CORE namespace MS.Internal.PresentationCore #elif PRESENTATIONFRAMEWORK namespace MS.Internal.PresentationFramework #elif REACHFRAMEWORK namespace MS.Internal.ReachFramework #elif DRT namespace MS.Internal.Drt #else #error Class is being used from an unknown assembly. #endif { internal static partial class SafeSecurityHelper { #if PRESENTATION_CORE ////// Given a rectangle with coords in local screen coordinates. /// Return the rectangle in global screen coordinates. /// ////// Critical - calls a critical function. /// TreatAsSafe - handing out a transformed rect is considered safe. /// /// Although we are calling a function that passes an array, and no. of elements. /// We limit this call to only call this function with a 2 as the count of elements. /// Thereby containing any potential for the call to Win32 to cause a buffer overrun. /// [SecurityCritical, SecurityTreatAsSafe ] internal static void TransformLocalRectToScreen(HandleRef hwnd, ref NativeMethods.RECT rcWindowCoords) { int retval = MapWindowPoints(hwnd , new HandleRef(null, IntPtr.Zero), ref rcWindowCoords, 2); int win32Err = Marshal.GetLastWin32Error(); if(retval == 0 && win32Err != 0) { throw new System.ComponentModel.Win32Exception(win32Err); } } #endif #if PRESENTATION_CORE || PRESENTATIONFRAMEWORK ||REACHFRAMEWORK || DEBUG #if !WINDOWS_BASE ////// Given an assembly, returns the partial name of the assembly. /// ////// This code used to perform an elevation but no longer needs to. /// The method is being kept in this class to ease auditing and /// should have a security review if changed. /// The string returned does not contain path information. /// This code is duplicated in SafeSecurityHelperPBT.cs. /// internal static string GetAssemblyPartialName(Assembly assembly) { AssemblyName name = new AssemblyName(assembly.FullName); string partialName = name.Name; return (partialName != null) ? partialName : String.Empty; } #endif #endif #if PRESENTATIONFRAMEWORK ////// Get the full assembly name by combining the partial name passed in /// with everything else from proto assembly. /// ////// This code used to perform an elevation but no longer needs to. /// The method is being kept in this class to ease auditing and /// should have a security review if changed. /// The string returned does not contain path information. /// internal static string GetFullAssemblyNameFromPartialName( Assembly protoAssembly, string partialName) { AssemblyName name = new AssemblyName(protoAssembly.FullName); name.Name = partialName; return name.FullName; } ////// Critical: This code accesses PresentationSource which is critical but does not /// expose it. /// TreatAsSafe: PresentationSource is not exposed and Client to Screen co-ordinates is /// safe to expose /// [SecurityCritical,SecurityTreatAsSafe] internal static Point ClientToScreen(UIElement relativeTo, Point point) { GeneralTransform transform; PresentationSource source = PresentationSource.CriticalFromVisual(relativeTo); if (source == null) { return new Point(double.NaN, double.NaN); } transform = relativeTo.TransformToAncestor(source.RootVisual); Point ptRoot; transform.TryTransform(point, out ptRoot); Point ptClient = PointUtil.RootToClient(ptRoot, source); Point ptScreen = PointUtil.ClientToScreen(ptClient, source); return ptScreen; } #endif // PRESENTATIONFRAMEWORK #if WINDOWS_BASE ////// This function iterates through the assemblies loaded in the current /// AppDomain and finds one that has the same assemblyName passed in. /// ////// This code used to perform an elevation but no longer needs to. /// The method is being kept in this class to ease auditing and /// should have a security review if changed. /// This code is duplicated in SafeSecurityHelperPBT.cs. /// internal static Assembly GetLoadedAssembly(string assemblyName, bool ignoreCase) { Assembly returnAssembly = null; Assembly[] assemblies = AppDomain.CurrentDomain.GetAssemblies(); for (int i = assemblies.Length -1; i >= 0; i--) { string fullName = assemblies[i].FullName; bool found = String.Compare(fullName, assemblyName, ignoreCase, CultureInfo.GetCultureInfo(DOTNETCULTURE)) == 0; if (!found) { int indexComma = fullName.IndexOf(",", StringComparison.Ordinal); if (indexComma > 0) { string shortName = fullName.Substring(0, indexComma); found = String.Compare(shortName, assemblyName, ignoreCase, CultureInfo.GetCultureInfo(DOTNETCULTURE)) == 0; } } if (found) { returnAssembly = assemblies[i]; break; } } return returnAssembly; } #endif // WINDOWS_BASE #if PRESENTATION_CORE // // Determine if two Public Key Tokens are the same. // private static bool IsSameKeyToken(byte[] reqKeyToken, byte[] curKeyToken) { bool isSame = false; if (reqKeyToken == null && curKeyToken == null) { // Both Key Tokens are not set, treat them as same. isSame = true; } else if (reqKeyToken != null && curKeyToken != null) { // Both KeyTokens are set. if (reqKeyToken.Length == curKeyToken.Length) { isSame = true; for (int i=0; i/// This function iterates through the assemblies loaded in the current /// AppDomain and finds one that has the same assemblyName, version and public /// key token which are passed in. /// /// /// This code used to perform an elevation but no longer needs to. /// The method is being kept in this class to ease auditing and /// should have a security review if changed. /// internal static Assembly GetLoadedAssembly(AssemblyName assemblyName, bool ignoreCase) { Assembly returnAssembly = null; Assembly[] assemblies = AppDomain.CurrentDomain.GetAssemblies(); for (int i = assemblies.Length -1; i >= 0; i--) { AssemblyName curAsmName = new AssemblyName(assemblies[i].FullName); CultureInfo curCulture, reqCulture; byte[] curKeyToken, reqKeyToken; curCulture = curAsmName.CultureInfo; reqCulture = assemblyName.CultureInfo; curKeyToken = curAsmName.GetPublicKeyToken( ); reqKeyToken = assemblyName.GetPublicKeyToken( ); if ( (String.Compare(curAsmName.Name, assemblyName.Name, ignoreCase, CultureInfo.GetCultureInfo(DOTNETCULTURE) ) == 0 ) && (assemblyName.Version == null || assemblyName.Version.Equals(curAsmName.Version) ) && (reqCulture == null || reqCulture.Equals(curCulture) ) && (reqKeyToken == null || IsSameKeyToken(reqKeyToken, curKeyToken) ) ) { returnAssembly = assemblies[i]; break; } } return returnAssembly; } #endif // PRESENTATION_CORE #if PRESENTATION_CORE || PRESENTATIONFRAMEWORK // enum to choose between the various keys internal enum KeyToRead { WebBrowserDisable = 0x01 , MediaAudioDisable = 0x02 , MediaVideoDisable = 0x04 , MediaImageDisable = 0x08 , MediaAudioOrVideoDisable = KeyToRead.MediaVideoDisable | KeyToRead.MediaAudioDisable , } ////// Critical: This code elevates to access registry /// TreatAsSafe: The information it exposes is safe to give out and all it does is read a specific key /// [SecurityCritical,SecurityTreatAsSafe] internal static bool IsFeatureDisabled(KeyToRead key) { string regValue = null; bool fResult = false; switch (key) { case KeyToRead.WebBrowserDisable: regValue = RegistryKeys.value_WebBrowserDisallow; break; case KeyToRead.MediaAudioDisable: regValue = RegistryKeys.value_MediaAudioDisallow; break; case KeyToRead.MediaVideoDisable: regValue = RegistryKeys.value_MediaVideoDisallow; break; case KeyToRead.MediaImageDisable: regValue = RegistryKeys.value_MediaImageDisallow; break; case KeyToRead.MediaAudioOrVideoDisable: regValue = RegistryKeys.value_MediaAudioDisallow; break; default:// throw exception for invalid key throw(new System.ArgumentException(key.ToString())); } RegistryKey featureKey; //Assert for read access to HKLM\Software\Microsoft\Windows\Avalon RegistryPermission regPerm = new RegistryPermission(RegistryPermissionAccess.Read,"HKEY_LOCAL_MACHINE\\"+RegistryKeys.WPF_Features);//BlessedAssert regPerm.Assert();//BlessedAssert try { object obj = null; bool keyValue = false; // open the key and read the value featureKey = Registry.LocalMachine.OpenSubKey(RegistryKeys.WPF_Features); if (featureKey != null) { // If key exists and value is 1 return true else false obj = featureKey.GetValue(regValue); keyValue = obj is int && ((int)obj == 1); if (keyValue) { fResult = true; } // special case for audio and video since they can be orred // this is in the condition that audio is enabled since that is // the path that MediaAudioVideoDisable defaults to // This is purely to optimize perf on the number of calls to assert // in the media or audio scenario. if ((fResult == false) && (key == KeyToRead.MediaAudioOrVideoDisable)) { regValue = RegistryKeys.value_MediaVideoDisallow; // If key exists and value is 1 return true else false obj = featureKey.GetValue(regValue); keyValue = obj is int && ((int)obj == 1); if (keyValue) { fResult = true; } } } } finally { RegistryPermission.RevertAssert(); } return fResult; } #endif //PRESENTATIONCORE||PRESENTATIONFRAMEWORK #if PRESENTATION_CORE ////// This function is a wrapper for CultureInfo.GetCultureInfoByIetfLanguageTag(). /// The wrapper works around a bug in that routine, which causes it to throw /// a SecurityException in Partial Trust. VSWhidbey bug #572162. /// ////// Critical: This code elevates to access registry /// TreatAsSafe: The information it exposes is safe to give out and all it does is read a specific key /// [SecurityCritical,SecurityTreatAsSafe] static internal CultureInfo GetCultureInfoByIetfLanguageTag(string languageTag) { CultureInfo culture = null; RegistryPermission regPerm = new RegistryPermission(RegistryPermissionAccess.Read, RegistryKeys.HKLM_IetfLanguage);//BlessedAssert regPerm.Assert();//BlessedAssert try { culture = CultureInfo.GetCultureInfoByIetfLanguageTag(languageTag); } finally { RegistryPermission.RevertAssert(); } return culture; } #endif //PRESENTATIONCORE // // Private p-invokes. // #if !PRESENTATIONFRAMEWORK && !WINDOWS_BASE ////// Critical - this function elevates /// [SecurityCritical, SuppressUnmanagedCodeSecurity ] [DllImport(ExternDll.User32, SetLastError = true, ExactSpelling=true, CharSet=CharSet.Auto)] private static extern int MapWindowPoints(HandleRef hWndFrom, HandleRef hWndTo, [In, Out] ref NativeMethods.RECT rect, int cPoints); #endif internal const string DOTNETCULTURE = "en-us"; internal const string IMAGE = "image"; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
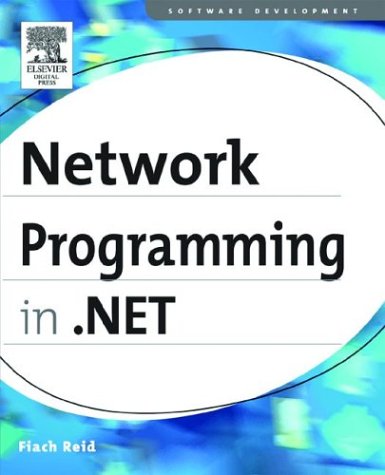
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IndexedString.cs
- EventProvider.cs
- LicenseException.cs
- SqlDataSourceConfigureFilterForm.cs
- SafeRightsManagementHandle.cs
- AttributeAction.cs
- QueryOperationResponseOfT.cs
- SystemIcmpV4Statistics.cs
- XmlnsCache.cs
- GridViewAutomationPeer.cs
- PropertyMapper.cs
- StagingAreaInputItem.cs
- UserPersonalizationStateInfo.cs
- ColumnResizeAdorner.cs
- PageScaling.cs
- PersonalizationProviderCollection.cs
- PenLineJoinValidation.cs
- OutputChannelBinder.cs
- SingleResultAttribute.cs
- CodeCatchClauseCollection.cs
- HtmlShimManager.cs
- TreeNodeCollectionEditor.cs
- ParseNumbers.cs
- NavigationService.cs
- XmlCharCheckingReader.cs
- SqlGenericUtil.cs
- ToolStripAdornerWindowService.cs
- HMACSHA384.cs
- PerformanceCounterLib.cs
- SystemParameters.cs
- ReliabilityContractAttribute.cs
- Subtree.cs
- SqlDataSource.cs
- Properties.cs
- DesignerToolStripControlHost.cs
- GridViewCommandEventArgs.cs
- SecureUICommand.cs
- ProtocolViolationException.cs
- DataGridViewColumnCollection.cs
- SmiTypedGetterSetter.cs
- SqlCommand.cs
- TransactionValidationBehavior.cs
- DateTimeStorage.cs
- FunctionNode.cs
- OpenTypeLayoutCache.cs
- TabControl.cs
- FrameworkContentElement.cs
- OdbcEnvironmentHandle.cs
- DbProviderFactory.cs
- EventData.cs
- XmlIlVisitor.cs
- Control.cs
- MergeEnumerator.cs
- ButtonFlatAdapter.cs
- TransactionValidationBehavior.cs
- Type.cs
- AssemblyBuilderData.cs
- ConnectionInterfaceCollection.cs
- SqlIdentifier.cs
- ParamArrayAttribute.cs
- FileStream.cs
- ImageKeyConverter.cs
- ListViewInsertedEventArgs.cs
- OneOfElement.cs
- UriExt.cs
- AlternateView.cs
- DataTransferEventArgs.cs
- CounterSample.cs
- VScrollProperties.cs
- SrgsElementList.cs
- SchemaImporter.cs
- Attributes.cs
- JsonReaderWriterFactory.cs
- WindowsFormsHostAutomationPeer.cs
- FixedHyperLink.cs
- OleDbInfoMessageEvent.cs
- TransactionFlowProperty.cs
- HttpClientCertificate.cs
- ProtocolsConfigurationEntry.cs
- PeerPresenceInfo.cs
- DebugTraceHelper.cs
- sqlmetadatafactory.cs
- ScriptReferenceEventArgs.cs
- _UriSyntax.cs
- PageSettings.cs
- NameObjectCollectionBase.cs
- VSWCFServiceContractGenerator.cs
- HtmlGenericControl.cs
- MessageSecurityOverTcpElement.cs
- EventWaitHandleSecurity.cs
- Vector3DConverter.cs
- DependencyProperty.cs
- ClientBuildManager.cs
- _RequestCacheProtocol.cs
- RuntimeWrappedException.cs
- Matrix.cs
- EntityProviderFactory.cs
- DesignerActionPanel.cs
- OptimizerPatterns.cs
- DataPagerFieldCommandEventArgs.cs