Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Automation / Peers / GridViewAutomationPeer.cs / 1305600 / GridViewAutomationPeer.cs
using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Windows; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Interop; using System.Windows.Media; using MS.Internal; using MS.Internal.Automation; using MS.Win32; namespace System.Windows.Automation.Peers { ////// GridView automation peer /// ////// Basically, the idea is to add a virtual method called CreateAutomationPeer on ViewBase /// Any view can override this method to create its own automation peer. /// ListView will use this method to get an automation peer for a given view and default to /// the properties/methods/patterns implemented by the view before going to default fall-backs on it /// These view automation peer must implement IViewAutomationPeer interface /// public class GridViewAutomationPeer : IViewAutomationPeer, ITableProvider { /// public GridViewAutomationPeer(GridView owner, ListView listview) : base() { Invariant.Assert(owner != null); Invariant.Assert(listview != null); _owner = owner; _listview = listview; //Remember the items/columns count when GVAP is created, this is used for firing RowCount/ColumnCount changed event _oldItemsCount = _listview.Items.Count; _oldColumnsCount = _owner.Columns.Count; ((INotifyCollectionChanged)_owner.Columns).CollectionChanged += new NotifyCollectionChangedEventHandler(OnColumnCollectionChanged); } /// AutomationControlType IViewAutomationPeer.GetAutomationControlType() { return AutomationControlType.DataGrid; } /// object IViewAutomationPeer.GetPattern(PatternInterface patternInterface) { object ret = null; switch (patternInterface) { case PatternInterface.Grid: case PatternInterface.Table: ret = this; break; } return ret; } /// ListIViewAutomationPeer.GetChildren(List children) { //Add GridViewHeaderRowPresenter as the first child of ListView if (_owner.HeaderRowPresenter != null) { AutomationPeer peer = UIElementAutomationPeer.CreatePeerForElement(_owner.HeaderRowPresenter); if (peer != null) { //If children is null, we still need to create an empty list to insert HeaderRowPresenter if (children == null) { children = new List (); } children.Insert(0, peer); } } return children; } ItemAutomationPeer IViewAutomationPeer.CreateItemAutomationPeer(object item) { ListViewAutomationPeer lvAP = UIElementAutomationPeer.FromElement(_listview) as ListViewAutomationPeer; return new GridViewItemAutomationPeer(item, lvAP); } /// void IViewAutomationPeer.ItemsChanged(NotifyCollectionChangedEventArgs e) { ListViewAutomationPeer peer = UIElementAutomationPeer.FromElement(_listview) as ListViewAutomationPeer; if (peer != null) { if (_oldItemsCount != _listview.Items.Count) { peer.RaisePropertyChangedEvent(GridPatternIdentifiers.RowCountProperty, _oldItemsCount, _listview.Items.Count); } _oldItemsCount = _listview.Items.Count; } } //Called when the view is detached from the listview // Note: see bug 1555137 for details. // Never inline, as we don't want to unnecessarily link the // automation DLL via the ITableProvider, IGridProvider interface type initialization. [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] void IViewAutomationPeer.ViewDetached() { ((INotifyCollectionChanged)_owner.Columns).CollectionChanged -= new NotifyCollectionChangedEventHandler(OnColumnCollectionChanged); } #region ITableProvider /// /// Indicates if the data is best presented by row or column /// RowOrColumnMajor ITableProvider.RowOrColumnMajor { get { return RowOrColumnMajor.RowMajor; } } ////// Collection of column headers /// IRawElementProviderSimple[] ITableProvider.GetColumnHeaders() { if (_owner.HeaderRowPresenter != null) { Listarray = new List (_owner.HeaderRowPresenter.ActualColumnHeaders.Count); ListViewAutomationPeer lvpeer = UIElementAutomationPeer.FromElement(_listview) as ListViewAutomationPeer; if(lvpeer != null) { foreach (UIElement e in _owner.HeaderRowPresenter.ActualColumnHeaders) { AutomationPeer peer = UIElementAutomationPeer.CreatePeerForElement(e); if (peer != null) { array.Add(ElementProxy.StaticWrap(peer, lvpeer)); } } } return array.ToArray(); } return new IRawElementProviderSimple[0] ; } /// /// Collection of row headers /// IRawElementProviderSimple[] ITableProvider.GetRowHeaders() { //If there are no row headers, return an empty array return new IRawElementProviderSimple[0]; } #endregion #region IGridProvider ////// number of columns in the grid /// int IGridProvider.ColumnCount { get { if (_owner.HeaderRowPresenter != null) { return _owner.HeaderRowPresenter.ActualColumnHeaders.Count; } return _owner.Columns.Count; } } ////// number of rows in the grid /// int IGridProvider.RowCount { get { return _listview.Items.Count; } } ////// Obtain the IRawElementProviderSimple at an absolute position /// IRawElementProviderSimple IGridProvider.GetItem(int row, int column) { if (row < 0 || row >= ((IGridProvider)this).RowCount) { throw new ArgumentOutOfRangeException("row"); } if (column < 0 || column >= ((IGridProvider)this).ColumnCount) { throw new ArgumentOutOfRangeException("column"); } ListViewItem lvi = _listview.ItemContainerGenerator.ContainerFromIndex(row) as ListViewItem; //If item is virtualized, try to de-virtualize it if (lvi == null && !_listview.IsGrouping) { VirtualizingPanel itemsHost = _listview.ItemsHost as VirtualizingPanel; if (itemsHost != null) { itemsHost.BringIndexIntoView(row); } lvi = _listview.ItemContainerGenerator.ContainerFromIndex(row) as ListViewItem; if (lvi != null) { //Must call Invoke here to force run the render process _listview.Dispatcher.Invoke( System.Windows.Threading.DispatcherPriority.Loaded, (System.Windows.Threading.DispatcherOperationCallback)delegate(object arg) { return null; }, null); } } //lvi is null, it is virtualized, so we can't return its cell if (lvi != null) { AutomationPeer lvpeer = UIElementAutomationPeer.FromElement(_listview); if(lvpeer != null) { Listrows = lvpeer.GetChildren(); //Headers is the first child of GridView, so we need to skip it here if (rows.Count-1 > row) { AutomationPeer peer = rows[row+1]; List columns = peer.GetChildren(); if (columns.Count > column) { return ElementProxy.StaticWrap(columns[column], lvpeer); } } } } return null; } /// /// Fire ColumnCount changed event for GridPattern /// private void OnColumnCollectionChanged(object sender, NotifyCollectionChangedEventArgs e) { if (_oldColumnsCount != _owner.Columns.Count) { ListViewAutomationPeer peer = UIElementAutomationPeer.FromElement(_listview) as ListViewAutomationPeer; Invariant.Assert(peer != null); if (peer != null) { peer.RaisePropertyChangedEvent(GridPatternIdentifiers.ColumnCountProperty, _oldColumnsCount, _owner.Columns.Count); } } _oldColumnsCount = _owner.Columns.Count; AutomationPeer lvPeer = UIElementAutomationPeer.FromElement(_listview); if (lvPeer != null) { Listlist = lvPeer.GetChildren(); if (list != null) { foreach (AutomationPeer peer in list) { peer.InvalidatePeer(); } } } } #endregion #region Helper internal static Visual FindVisualByType(Visual parent, Type type) { if (parent != null) { int count = parent.InternalVisualChildrenCount; for (int i = 0; i < count; i++) { Visual visual = parent.InternalGetVisualChild(i); if (!type.IsInstanceOfType(visual)) { visual = FindVisualByType(visual, type); } if (visual != null) { return visual; } } } return null; } #endregion #region Private Fields private GridView _owner; private ListView _listview; //Store the old items/columns count, this is used for firing RowCount changed event in IGridProvider private int _oldItemsCount = 0; private int _oldColumnsCount = 0; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Windows; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Interop; using System.Windows.Media; using MS.Internal; using MS.Internal.Automation; using MS.Win32; namespace System.Windows.Automation.Peers { /// /// GridView automation peer /// ////// Basically, the idea is to add a virtual method called CreateAutomationPeer on ViewBase /// Any view can override this method to create its own automation peer. /// ListView will use this method to get an automation peer for a given view and default to /// the properties/methods/patterns implemented by the view before going to default fall-backs on it /// These view automation peer must implement IViewAutomationPeer interface /// public class GridViewAutomationPeer : IViewAutomationPeer, ITableProvider { /// public GridViewAutomationPeer(GridView owner, ListView listview) : base() { Invariant.Assert(owner != null); Invariant.Assert(listview != null); _owner = owner; _listview = listview; //Remember the items/columns count when GVAP is created, this is used for firing RowCount/ColumnCount changed event _oldItemsCount = _listview.Items.Count; _oldColumnsCount = _owner.Columns.Count; ((INotifyCollectionChanged)_owner.Columns).CollectionChanged += new NotifyCollectionChangedEventHandler(OnColumnCollectionChanged); } /// AutomationControlType IViewAutomationPeer.GetAutomationControlType() { return AutomationControlType.DataGrid; } /// object IViewAutomationPeer.GetPattern(PatternInterface patternInterface) { object ret = null; switch (patternInterface) { case PatternInterface.Grid: case PatternInterface.Table: ret = this; break; } return ret; } /// ListIViewAutomationPeer.GetChildren(List children) { //Add GridViewHeaderRowPresenter as the first child of ListView if (_owner.HeaderRowPresenter != null) { AutomationPeer peer = UIElementAutomationPeer.CreatePeerForElement(_owner.HeaderRowPresenter); if (peer != null) { //If children is null, we still need to create an empty list to insert HeaderRowPresenter if (children == null) { children = new List (); } children.Insert(0, peer); } } return children; } ItemAutomationPeer IViewAutomationPeer.CreateItemAutomationPeer(object item) { ListViewAutomationPeer lvAP = UIElementAutomationPeer.FromElement(_listview) as ListViewAutomationPeer; return new GridViewItemAutomationPeer(item, lvAP); } /// void IViewAutomationPeer.ItemsChanged(NotifyCollectionChangedEventArgs e) { ListViewAutomationPeer peer = UIElementAutomationPeer.FromElement(_listview) as ListViewAutomationPeer; if (peer != null) { if (_oldItemsCount != _listview.Items.Count) { peer.RaisePropertyChangedEvent(GridPatternIdentifiers.RowCountProperty, _oldItemsCount, _listview.Items.Count); } _oldItemsCount = _listview.Items.Count; } } //Called when the view is detached from the listview // Note: see bug 1555137 for details. // Never inline, as we don't want to unnecessarily link the // automation DLL via the ITableProvider, IGridProvider interface type initialization. [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] void IViewAutomationPeer.ViewDetached() { ((INotifyCollectionChanged)_owner.Columns).CollectionChanged -= new NotifyCollectionChangedEventHandler(OnColumnCollectionChanged); } #region ITableProvider /// /// Indicates if the data is best presented by row or column /// RowOrColumnMajor ITableProvider.RowOrColumnMajor { get { return RowOrColumnMajor.RowMajor; } } ////// Collection of column headers /// IRawElementProviderSimple[] ITableProvider.GetColumnHeaders() { if (_owner.HeaderRowPresenter != null) { Listarray = new List (_owner.HeaderRowPresenter.ActualColumnHeaders.Count); ListViewAutomationPeer lvpeer = UIElementAutomationPeer.FromElement(_listview) as ListViewAutomationPeer; if(lvpeer != null) { foreach (UIElement e in _owner.HeaderRowPresenter.ActualColumnHeaders) { AutomationPeer peer = UIElementAutomationPeer.CreatePeerForElement(e); if (peer != null) { array.Add(ElementProxy.StaticWrap(peer, lvpeer)); } } } return array.ToArray(); } return new IRawElementProviderSimple[0] ; } /// /// Collection of row headers /// IRawElementProviderSimple[] ITableProvider.GetRowHeaders() { //If there are no row headers, return an empty array return new IRawElementProviderSimple[0]; } #endregion #region IGridProvider ////// number of columns in the grid /// int IGridProvider.ColumnCount { get { if (_owner.HeaderRowPresenter != null) { return _owner.HeaderRowPresenter.ActualColumnHeaders.Count; } return _owner.Columns.Count; } } ////// number of rows in the grid /// int IGridProvider.RowCount { get { return _listview.Items.Count; } } ////// Obtain the IRawElementProviderSimple at an absolute position /// IRawElementProviderSimple IGridProvider.GetItem(int row, int column) { if (row < 0 || row >= ((IGridProvider)this).RowCount) { throw new ArgumentOutOfRangeException("row"); } if (column < 0 || column >= ((IGridProvider)this).ColumnCount) { throw new ArgumentOutOfRangeException("column"); } ListViewItem lvi = _listview.ItemContainerGenerator.ContainerFromIndex(row) as ListViewItem; //If item is virtualized, try to de-virtualize it if (lvi == null && !_listview.IsGrouping) { VirtualizingPanel itemsHost = _listview.ItemsHost as VirtualizingPanel; if (itemsHost != null) { itemsHost.BringIndexIntoView(row); } lvi = _listview.ItemContainerGenerator.ContainerFromIndex(row) as ListViewItem; if (lvi != null) { //Must call Invoke here to force run the render process _listview.Dispatcher.Invoke( System.Windows.Threading.DispatcherPriority.Loaded, (System.Windows.Threading.DispatcherOperationCallback)delegate(object arg) { return null; }, null); } } //lvi is null, it is virtualized, so we can't return its cell if (lvi != null) { AutomationPeer lvpeer = UIElementAutomationPeer.FromElement(_listview); if(lvpeer != null) { Listrows = lvpeer.GetChildren(); //Headers is the first child of GridView, so we need to skip it here if (rows.Count-1 > row) { AutomationPeer peer = rows[row+1]; List columns = peer.GetChildren(); if (columns.Count > column) { return ElementProxy.StaticWrap(columns[column], lvpeer); } } } } return null; } /// /// Fire ColumnCount changed event for GridPattern /// private void OnColumnCollectionChanged(object sender, NotifyCollectionChangedEventArgs e) { if (_oldColumnsCount != _owner.Columns.Count) { ListViewAutomationPeer peer = UIElementAutomationPeer.FromElement(_listview) as ListViewAutomationPeer; Invariant.Assert(peer != null); if (peer != null) { peer.RaisePropertyChangedEvent(GridPatternIdentifiers.ColumnCountProperty, _oldColumnsCount, _owner.Columns.Count); } } _oldColumnsCount = _owner.Columns.Count; AutomationPeer lvPeer = UIElementAutomationPeer.FromElement(_listview); if (lvPeer != null) { Listlist = lvPeer.GetChildren(); if (list != null) { foreach (AutomationPeer peer in list) { peer.InvalidatePeer(); } } } } #endregion #region Helper internal static Visual FindVisualByType(Visual parent, Type type) { if (parent != null) { int count = parent.InternalVisualChildrenCount; for (int i = 0; i < count; i++) { Visual visual = parent.InternalGetVisualChild(i); if (!type.IsInstanceOfType(visual)) { visual = FindVisualByType(visual, type); } if (visual != null) { return visual; } } } return null; } #endregion #region Private Fields private GridView _owner; private ListView _listview; //Store the old items/columns count, this is used for firing RowCount changed event in IGridProvider private int _oldItemsCount = 0; private int _oldColumnsCount = 0; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
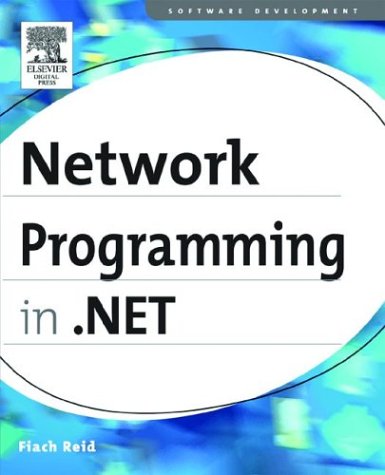
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BlockCollection.cs
- XmlQueryType.cs
- TextStore.cs
- CookielessHelper.cs
- LicenseManager.cs
- CompositeFontFamily.cs
- HelpInfo.cs
- TimelineCollection.cs
- DataViewManagerListItemTypeDescriptor.cs
- StringExpressionSet.cs
- ActivityExecutionContextCollection.cs
- EntryWrittenEventArgs.cs
- _Events.cs
- GenerateHelper.cs
- DataObject.cs
- IndexedString.cs
- XmlSchemaElement.cs
- HttpResponseInternalWrapper.cs
- SimpleApplicationHost.cs
- CodeTypeParameter.cs
- GridItem.cs
- DateTime.cs
- BatchStream.cs
- Mapping.cs
- SvcMapFile.cs
- NavigationPropertyEmitter.cs
- WrappedKeySecurityToken.cs
- EdmScalarPropertyAttribute.cs
- ObjectHandle.cs
- RangeValidator.cs
- autovalidator.cs
- FrameworkObject.cs
- WebPartZoneCollection.cs
- BamlResourceDeserializer.cs
- InlineObject.cs
- SqlParameterCollection.cs
- SortedSetDebugView.cs
- _Rfc2616CacheValidators.cs
- TextEditorParagraphs.cs
- CorrelationQuery.cs
- GetPageCompletedEventArgs.cs
- CqlErrorHelper.cs
- DataListGeneralPage.cs
- StringStorage.cs
- LifetimeServices.cs
- DataExpression.cs
- ObjectDataSourceMethodEventArgs.cs
- COM2TypeInfoProcessor.cs
- ResourceManager.cs
- PropertyValueEditor.cs
- UIElement.cs
- XmlSchemaException.cs
- Aggregates.cs
- TraceEventCache.cs
- XamlFilter.cs
- XmlSchemaInclude.cs
- AttributeXamlType.cs
- ServiceModelSectionGroup.cs
- WindowsSecurityTokenAuthenticator.cs
- XmlName.cs
- TreeNodeEventArgs.cs
- LoadWorkflowAsyncResult.cs
- Int16KeyFrameCollection.cs
- SecurityState.cs
- ImportCatalogPart.cs
- ApplicationFileParser.cs
- columnmapfactory.cs
- DocumentPageViewAutomationPeer.cs
- MarshalDirectiveException.cs
- RawStylusInputReport.cs
- RtfControlWordInfo.cs
- WorkflowOperationFault.cs
- CodeLinePragma.cs
- DynamicILGenerator.cs
- StoryFragments.cs
- RelationshipManager.cs
- wmiprovider.cs
- SessionPageStatePersister.cs
- EllipseGeometry.cs
- ConsoleKeyInfo.cs
- SoapUnknownHeader.cs
- GridErrorDlg.cs
- ConfigurationProperty.cs
- MsmqSecureHashAlgorithm.cs
- SuppressIldasmAttribute.cs
- FixedSOMTableRow.cs
- MimeObjectFactory.cs
- SafeFileHandle.cs
- Transform3DGroup.cs
- SystemResourceKey.cs
- VirtualPathProvider.cs
- Lock.cs
- PeerNameRecord.cs
- rsa.cs
- LinkedResource.cs
- EnumBuilder.cs
- LicenseProviderAttribute.cs
- ForEachDesigner.xaml.cs
- RegexRunner.cs
- XsltInput.cs