Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Shared / MS / Win32 / SafeSystemMetrics.cs / 1305600 / SafeSystemMetrics.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2004 // // File: SafeSystemMetrics.cs // This class is copied from the system metrics class in frameworks. The // reason it exists is to consolidate all system metric calls through one layer // so that maintenance from a security stand point gets easier. We will add // mertrics on a need basis. The caching code is removed since the original calls // that were moved here do not rely on caching. If there is a percieved perf. problem // we can work on enabling this. //----------------------------------------------------------------------------- using System; using System.Collections; using System.Runtime.InteropServices; using System.Windows.Media; using Microsoft.Win32; using System.Security; using System.Security.Permissions; using MS.Win32; using MS.Internal; using MS.Internal.Interop; using MS.Internal.PresentationCore; namespace MS.Win32 { ////// Contains properties that are queries into the system's various settings. /// [FriendAccessAllowed] // Built into Core, also used by Framework. internal sealed class SafeSystemMetrics { private SafeSystemMetrics() { } #if !PRESENTATION_CORE ////// Maps to SM_CXVIRTUALSCREEN /// ////// TreatAsSafe --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// internal static int VirtualScreenWidth { [SecurityCritical,SecurityTreatAsSafe] get { SecurityHelper.DemandUnmanagedCode(); return UnsafeNativeMethods.GetSystemMetrics(SM.CXVIRTUALSCREEN); } } ////// Maps to SM_CYVIRTUALSCREEN /// ////// TreatAsSafe --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// internal static int VirtualScreenHeight { [SecurityCritical,SecurityTreatAsSafe] get { SecurityHelper.DemandUnmanagedCode(); return UnsafeNativeMethods.GetSystemMetrics(SM.CYVIRTUALSCREEN); } } #endif //end !PRESENTATIONCORE ////// Maps to SM_CXDOUBLECLK /// ////// TreatAsSafe --This data is safe to expose /// Security Critical -- Calling UnsafeNativeMethods /// internal static int DoubleClickDeltaX { [SecurityCritical, SecurityTreatAsSafe] get { return UnsafeNativeMethods.GetSystemMetrics(SM.CXDOUBLECLK); } } ////// Maps to SM_CYDOUBLECLK /// ////// TreatAsSafe --This data is safe to expose /// Security Critical -- Calling UnsafeNativeMethods /// internal static int DoubleClickDeltaY { [SecurityCritical, SecurityTreatAsSafe] get { return UnsafeNativeMethods.GetSystemMetrics(SM.CYDOUBLECLK); } } ////// Maps to SM_CXDRAG /// ////// TreatAsSafe --This data is safe to expose /// Security Critical -- Calling UnsafeNativeMethods /// internal static int DragDeltaX { [SecurityCritical, SecurityTreatAsSafe] get { return UnsafeNativeMethods.GetSystemMetrics(SM.CXDRAG); } } ////// Maps to SM_CYDRAG /// ////// TreatAsSafe --This data is safe to expose /// Security Critical -- Calling UnsafeNativeMethods /// internal static int DragDeltaY { [SecurityCritical, SecurityTreatAsSafe] get { return UnsafeNativeMethods.GetSystemMetrics(SM.CYDRAG); } } ////// Is an IMM enabled ? Maps to SM_IMMENABLED /// //////Critical - calls a method that performs an elevation. /// TreatAsSafe - data is considered safe to expose. /// internal static bool IsImmEnabled { [SecurityCritical, SecurityTreatAsSafe] get { return (UnsafeNativeMethods.GetSystemMetrics(SM.IMMENABLED) != 0); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2004 // // File: SafeSystemMetrics.cs // This class is copied from the system metrics class in frameworks. The // reason it exists is to consolidate all system metric calls through one layer // so that maintenance from a security stand point gets easier. We will add // mertrics on a need basis. The caching code is removed since the original calls // that were moved here do not rely on caching. If there is a percieved perf. problem // we can work on enabling this. //----------------------------------------------------------------------------- using System; using System.Collections; using System.Runtime.InteropServices; using System.Windows.Media; using Microsoft.Win32; using System.Security; using System.Security.Permissions; using MS.Win32; using MS.Internal; using MS.Internal.Interop; using MS.Internal.PresentationCore; namespace MS.Win32 { ////// Contains properties that are queries into the system's various settings. /// [FriendAccessAllowed] // Built into Core, also used by Framework. internal sealed class SafeSystemMetrics { private SafeSystemMetrics() { } #if !PRESENTATION_CORE ////// Maps to SM_CXVIRTUALSCREEN /// ////// TreatAsSafe --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// internal static int VirtualScreenWidth { [SecurityCritical,SecurityTreatAsSafe] get { SecurityHelper.DemandUnmanagedCode(); return UnsafeNativeMethods.GetSystemMetrics(SM.CXVIRTUALSCREEN); } } ////// Maps to SM_CYVIRTUALSCREEN /// ////// TreatAsSafe --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// internal static int VirtualScreenHeight { [SecurityCritical,SecurityTreatAsSafe] get { SecurityHelper.DemandUnmanagedCode(); return UnsafeNativeMethods.GetSystemMetrics(SM.CYVIRTUALSCREEN); } } #endif //end !PRESENTATIONCORE ////// Maps to SM_CXDOUBLECLK /// ////// TreatAsSafe --This data is safe to expose /// Security Critical -- Calling UnsafeNativeMethods /// internal static int DoubleClickDeltaX { [SecurityCritical, SecurityTreatAsSafe] get { return UnsafeNativeMethods.GetSystemMetrics(SM.CXDOUBLECLK); } } ////// Maps to SM_CYDOUBLECLK /// ////// TreatAsSafe --This data is safe to expose /// Security Critical -- Calling UnsafeNativeMethods /// internal static int DoubleClickDeltaY { [SecurityCritical, SecurityTreatAsSafe] get { return UnsafeNativeMethods.GetSystemMetrics(SM.CYDOUBLECLK); } } ////// Maps to SM_CXDRAG /// ////// TreatAsSafe --This data is safe to expose /// Security Critical -- Calling UnsafeNativeMethods /// internal static int DragDeltaX { [SecurityCritical, SecurityTreatAsSafe] get { return UnsafeNativeMethods.GetSystemMetrics(SM.CXDRAG); } } ////// Maps to SM_CYDRAG /// ////// TreatAsSafe --This data is safe to expose /// Security Critical -- Calling UnsafeNativeMethods /// internal static int DragDeltaY { [SecurityCritical, SecurityTreatAsSafe] get { return UnsafeNativeMethods.GetSystemMetrics(SM.CYDRAG); } } ////// Is an IMM enabled ? Maps to SM_IMMENABLED /// //////Critical - calls a method that performs an elevation. /// TreatAsSafe - data is considered safe to expose. /// internal static bool IsImmEnabled { [SecurityCritical, SecurityTreatAsSafe] get { return (UnsafeNativeMethods.GetSystemMetrics(SM.IMMENABLED) != 0); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
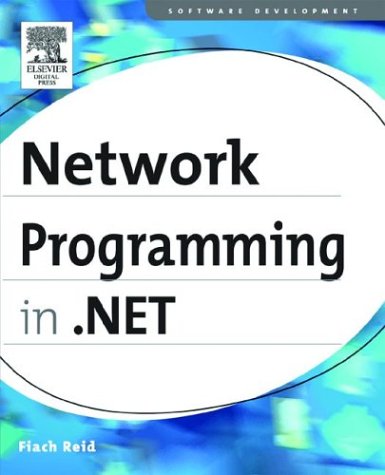
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MessageFault.cs
- EnumUnknown.cs
- ConfigurationElementCollection.cs
- ManipulationCompletedEventArgs.cs
- DoubleAnimation.cs
- InternalPolicyElement.cs
- Token.cs
- ButtonFieldBase.cs
- EventPrivateKey.cs
- RegexGroupCollection.cs
- DoubleStorage.cs
- NotificationContext.cs
- StrokeFIndices.cs
- ELinqQueryState.cs
- MetadataArtifactLoaderFile.cs
- CatalogPartCollection.cs
- StandardBindingReliableSessionElement.cs
- ErrorStyle.cs
- WebConfigurationHost.cs
- GridToolTip.cs
- TransformerInfoCollection.cs
- DecimalConverter.cs
- TypeGeneratedEventArgs.cs
- Random.cs
- RecommendedAsConfigurableAttribute.cs
- FixedDSBuilder.cs
- PartManifestEntry.cs
- CompensationParticipant.cs
- CancelEventArgs.cs
- LongCountAggregationOperator.cs
- XslVisitor.cs
- SQLDecimal.cs
- PrintingPermission.cs
- ParameterModifier.cs
- List.cs
- Renderer.cs
- WebServiceTypeData.cs
- CreateInstanceBinder.cs
- EnumerationRangeValidationUtil.cs
- XmlSchemaGroup.cs
- DocumentSequenceHighlightLayer.cs
- WebPartsSection.cs
- LogFlushAsyncResult.cs
- FontResourceCache.cs
- DataTemplateKey.cs
- XPSSignatureDefinition.cs
- WebPartMovingEventArgs.cs
- View.cs
- SoapMessage.cs
- ControllableStoryboardAction.cs
- RichTextBox.cs
- XmlWhitespace.cs
- ZipIOBlockManager.cs
- TextSpan.cs
- DataListComponentEditor.cs
- BuiltInPermissionSets.cs
- CultureInfoConverter.cs
- DesignerActionVerbItem.cs
- RegexCaptureCollection.cs
- ControlPager.cs
- ProtectedConfiguration.cs
- DataGridViewRowEventArgs.cs
- SoapBinding.cs
- SimpleType.cs
- BuildProviderCollection.cs
- Border.cs
- SamlDoNotCacheCondition.cs
- GenericTextProperties.cs
- StringSorter.cs
- LinkArea.cs
- SegmentInfo.cs
- StringExpressionSet.cs
- TextEditorContextMenu.cs
- SqlDataSourceWizardForm.cs
- ProviderManager.cs
- UrlMappingsModule.cs
- DES.cs
- StreamWriter.cs
- FamilyTypeface.cs
- LongAverageAggregationOperator.cs
- PlatformNotSupportedException.cs
- DataServiceClientException.cs
- JpegBitmapDecoder.cs
- KeyProperty.cs
- ZipIOCentralDirectoryFileHeader.cs
- FileAuthorizationModule.cs
- RC2.cs
- ReferencedAssemblyResolver.cs
- RewritingProcessor.cs
- TableLayoutCellPaintEventArgs.cs
- SHA384.cs
- StringCollectionMarkupSerializer.cs
- CheckBoxAutomationPeer.cs
- TrackingProfileDeserializationException.cs
- StringResourceManager.cs
- EntityDataSourceSelectedEventArgs.cs
- MultiView.cs
- ListCollectionView.cs
- dataprotectionpermission.cs
- DTCTransactionManager.cs