Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / MIT / System / Web / UI / MobileControls / ControlPager.cs / 1305376 / ControlPager.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; using System.Security.Permissions; namespace System.Web.UI.MobileControls { /* * Control pager, a class that provides state as a form is paginated. * * Copyright (c) 2000 Microsoft Corporation */ ///[AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] public class ControlPager { private Form _form; private int _pageWeight; private int _pageCount = 0; private int _remainingWeight = 0; //private int _maximumPage = -1; /// public static readonly int DefaultWeight = 100; /// public static readonly int UseDefaultWeight = -1; /// public ControlPager(Form form, int pageWeight) { _form = form; _pageWeight = pageWeight; } /// public int GetPage(int weight) { if (weight > _remainingWeight) { PageCount++; RemainingWeight = PageWeight; } if (weight > _remainingWeight) { _remainingWeight = 0; } else { _remainingWeight -= weight; } return PageCount; } /// public int PageWeight { get { return _pageWeight; } } /// public int RemainingWeight { get { return _remainingWeight; } set { _remainingWeight = value; } } /// public int PageCount { get { return _pageCount; } set { _pageCount = value; } } /* internal int MaximumPage { get { return _maximumPage; } set { _maximumPage = value; } } */ /// public ItemPager GetItemPager(MobileControl control, int itemCount, int itemsPerPage, int itemWeight) { return new ItemPager(this, control, itemCount, itemsPerPage, itemWeight); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; using System.Security.Permissions; namespace System.Web.UI.MobileControls { /* * Control pager, a class that provides state as a form is paginated. * * Copyright (c) 2000 Microsoft Corporation */ ///[AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] public class ControlPager { private Form _form; private int _pageWeight; private int _pageCount = 0; private int _remainingWeight = 0; //private int _maximumPage = -1; /// public static readonly int DefaultWeight = 100; /// public static readonly int UseDefaultWeight = -1; /// public ControlPager(Form form, int pageWeight) { _form = form; _pageWeight = pageWeight; } /// public int GetPage(int weight) { if (weight > _remainingWeight) { PageCount++; RemainingWeight = PageWeight; } if (weight > _remainingWeight) { _remainingWeight = 0; } else { _remainingWeight -= weight; } return PageCount; } /// public int PageWeight { get { return _pageWeight; } } /// public int RemainingWeight { get { return _remainingWeight; } set { _remainingWeight = value; } } /// public int PageCount { get { return _pageCount; } set { _pageCount = value; } } /* internal int MaximumPage { get { return _maximumPage; } set { _maximumPage = value; } } */ /// public ItemPager GetItemPager(MobileControl control, int itemCount, int itemsPerPage, int itemWeight) { return new ItemPager(this, control, itemCount, itemsPerPage, itemWeight); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
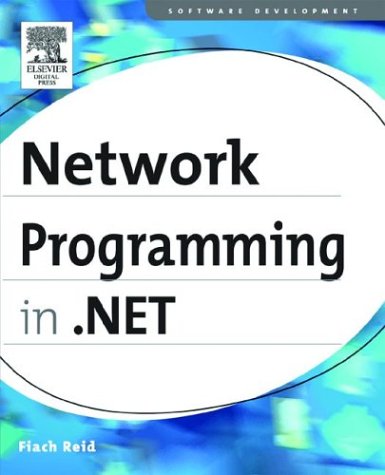
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ChainOfResponsibility.cs
- DependencyPropertyAttribute.cs
- TypeExtensionConverter.cs
- ThreadStateException.cs
- ToolBarButtonClickEvent.cs
- ComplexPropertyEntry.cs
- ReferenceConverter.cs
- smtpconnection.cs
- InfoCardAsymmetricCrypto.cs
- XmlSignificantWhitespace.cs
- InfoCardRSAPKCS1KeyExchangeDeformatter.cs
- ToolStripRenderEventArgs.cs
- WaitHandle.cs
- CollectionDataContractAttribute.cs
- IIS7UserPrincipal.cs
- LinkedList.cs
- ChangePassword.cs
- ControlPropertyNameConverter.cs
- CodeVariableDeclarationStatement.cs
- PrintingPermission.cs
- TabControlEvent.cs
- XmlEnumAttribute.cs
- DesignerDataTable.cs
- DataGridViewComboBoxColumn.cs
- InstanceDescriptor.cs
- ScalarType.cs
- RSACryptoServiceProvider.cs
- ToolStripRenderEventArgs.cs
- Subset.cs
- PathGeometry.cs
- UriSchemeKeyedCollection.cs
- QilXmlReader.cs
- Matrix.cs
- ContentWrapperAttribute.cs
- DataControlField.cs
- CollaborationHelperFunctions.cs
- Hyperlink.cs
- MonthCalendarDesigner.cs
- BridgeDataRecord.cs
- URL.cs
- DataGridViewCellStyleConverter.cs
- EntityDataSourceSelectingEventArgs.cs
- UnsafeNetInfoNativeMethods.cs
- OperationExecutionFault.cs
- SequentialUshortCollection.cs
- SafeMILHandle.cs
- ProviderConnectionPoint.cs
- DataSourceXmlSerializationAttribute.cs
- TextFormatterContext.cs
- SafeTokenHandle.cs
- WebPageTraceListener.cs
- InstallerTypeAttribute.cs
- InsufficientMemoryException.cs
- ColorTransform.cs
- Trigger.cs
- GridViewCellAutomationPeer.cs
- ListDataHelper.cs
- GridEntry.cs
- DataObjectCopyingEventArgs.cs
- Verify.cs
- ObjectContextServiceProvider.cs
- BounceEase.cs
- ScrollItemProviderWrapper.cs
- CorrelationResolver.cs
- WebBrowsableAttribute.cs
- BStrWrapper.cs
- WindowsSolidBrush.cs
- GroupBoxRenderer.cs
- TypeSource.cs
- SpnegoTokenProvider.cs
- AffineTransform3D.cs
- AppPool.cs
- DuplicateWaitObjectException.cs
- xmlNames.cs
- basevalidator.cs
- PageRanges.cs
- PrintPreviewControl.cs
- ProgressBarHighlightConverter.cs
- CssClassPropertyAttribute.cs
- TypeInformation.cs
- BypassElement.cs
- FocusChangedEventArgs.cs
- Hex.cs
- Binding.cs
- FixedTextContainer.cs
- OdbcHandle.cs
- ListControlStringCollectionEditor.cs
- ProcessStartInfo.cs
- ArgumentNullException.cs
- AutoCompleteStringCollection.cs
- FlowNode.cs
- TextReader.cs
- HyperLinkColumn.cs
- ProjectionCamera.cs
- CustomExpression.cs
- DataGridViewButtonColumn.cs
- PathFigure.cs
- LineBreakRecord.cs
- Array.cs
- ClientFormsAuthenticationMembershipProvider.cs