Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Automation / Peers / GridViewCellAutomationPeer.cs / 1305600 / GridViewCellAutomationPeer.cs
using System; using System.Collections; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Windows; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Interop; using System.Windows.Media; using MS.Internal; using MS.Win32; namespace System.Windows.Automation.Peers { /// public class GridViewCellAutomationPeer : FrameworkElementAutomationPeer, ITableItemProvider { /// internal GridViewCellAutomationPeer(ContentPresenter owner, ListViewAutomationPeer parent) : base(owner) { Invariant.Assert(parent != null); _listviewAP = parent; } /// internal GridViewCellAutomationPeer(TextBlock owner, ListViewAutomationPeer parent) : base(owner) { Invariant.Assert(parent != null); _listviewAP = parent; } /// protected override string GetClassNameCore() { return Owner.GetType().Name; } /// override protected AutomationControlType GetAutomationControlTypeCore() { if (Owner is TextBlock) { return AutomationControlType.Text; } else { return AutomationControlType.Custom; } } /// override public object GetPattern(PatternInterface patternInterface) { if (patternInterface == PatternInterface.GridItem || patternInterface == PatternInterface.TableItem) { return this; } return base.GetPattern(patternInterface); } /// protected override bool IsControlElementCore() { if (Owner is TextBlock) { return true; } else { Listchildren = GetChildrenAutomationPeer(Owner); return children != null && children.Count >= 1; } } internal int Column { get { return _column; } set { _column = value; } } internal int Row { get { return _row; } set { _row = value; } } #region ITableItem IRawElementProviderSimple[] ITableItemProvider.GetRowHeaderItems() { //If there are no row headers, return an empty array return new IRawElementProviderSimple[0]; } IRawElementProviderSimple[] ITableItemProvider.GetColumnHeaderItems() { ListView listview = _listviewAP.Owner as ListView; if (listview != null && listview.View is GridView) { GridView gridview = listview.View as GridView; if (gridview.HeaderRowPresenter != null && gridview.HeaderRowPresenter.ActualColumnHeaders.Count > Column) { GridViewColumnHeader header = gridview.HeaderRowPresenter.ActualColumnHeaders[Column]; AutomationPeer peer = UIElementAutomationPeer.FromElement(header); if (peer != null) { return new IRawElementProviderSimple[] { ProviderFromPeer(peer) }; } } } return new IRawElementProviderSimple[0]; } #endregion #region IGridItem int IGridItemProvider.Row { get { return Row; } } int IGridItemProvider.Column { get { return Column; } } int IGridItemProvider.RowSpan { get { return 1; } } int IGridItemProvider.ColumnSpan { get { return 1; } } IRawElementProviderSimple IGridItemProvider.ContainingGrid { get { return ProviderFromPeer(_listviewAP); } } #endregion #region Private Methods /// /// Get the children of the parent which has automation peer /// private ListGetChildrenAutomationPeer(Visual parent) { Invariant.Assert(parent != null); List children = null; iterate(parent, (IteratorCallback)delegate(AutomationPeer peer) { if (children == null) children = new List (); children.Add(peer); return (false); }); return children; } private delegate bool IteratorCallback(AutomationPeer peer); // private static bool iterate(Visual parent, IteratorCallback callback) { bool done = false; AutomationPeer peer = null; int count = parent.InternalVisualChildrenCount; for (int i = 0; i < count && !done; i++) { Visual child = parent.InternalGetVisualChild(i); if (child != null && child.CheckFlagsAnd(VisualFlags.IsUIElement) && (peer = CreatePeerForElement((UIElement)child)) != null) { done = callback(peer); } else { done = iterate(child, callback); } } return (done); } #endregion #region Private Fields private ListViewAutomationPeer _listviewAP; private int _column; private int _row; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Windows; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Interop; using System.Windows.Media; using MS.Internal; using MS.Win32; namespace System.Windows.Automation.Peers { /// public class GridViewCellAutomationPeer : FrameworkElementAutomationPeer, ITableItemProvider { /// internal GridViewCellAutomationPeer(ContentPresenter owner, ListViewAutomationPeer parent) : base(owner) { Invariant.Assert(parent != null); _listviewAP = parent; } /// internal GridViewCellAutomationPeer(TextBlock owner, ListViewAutomationPeer parent) : base(owner) { Invariant.Assert(parent != null); _listviewAP = parent; } /// protected override string GetClassNameCore() { return Owner.GetType().Name; } /// override protected AutomationControlType GetAutomationControlTypeCore() { if (Owner is TextBlock) { return AutomationControlType.Text; } else { return AutomationControlType.Custom; } } /// override public object GetPattern(PatternInterface patternInterface) { if (patternInterface == PatternInterface.GridItem || patternInterface == PatternInterface.TableItem) { return this; } return base.GetPattern(patternInterface); } /// protected override bool IsControlElementCore() { if (Owner is TextBlock) { return true; } else { List children = GetChildrenAutomationPeer(Owner); return children != null && children.Count >= 1; } } internal int Column { get { return _column; } set { _column = value; } } internal int Row { get { return _row; } set { _row = value; } } #region ITableItem IRawElementProviderSimple[] ITableItemProvider.GetRowHeaderItems() { //If there are no row headers, return an empty array return new IRawElementProviderSimple[0]; } IRawElementProviderSimple[] ITableItemProvider.GetColumnHeaderItems() { ListView listview = _listviewAP.Owner as ListView; if (listview != null && listview.View is GridView) { GridView gridview = listview.View as GridView; if (gridview.HeaderRowPresenter != null && gridview.HeaderRowPresenter.ActualColumnHeaders.Count > Column) { GridViewColumnHeader header = gridview.HeaderRowPresenter.ActualColumnHeaders[Column]; AutomationPeer peer = UIElementAutomationPeer.FromElement(header); if (peer != null) { return new IRawElementProviderSimple[] { ProviderFromPeer(peer) }; } } } return new IRawElementProviderSimple[0]; } #endregion #region IGridItem int IGridItemProvider.Row { get { return Row; } } int IGridItemProvider.Column { get { return Column; } } int IGridItemProvider.RowSpan { get { return 1; } } int IGridItemProvider.ColumnSpan { get { return 1; } } IRawElementProviderSimple IGridItemProvider.ContainingGrid { get { return ProviderFromPeer(_listviewAP); } } #endregion #region Private Methods /// /// Get the children of the parent which has automation peer /// private ListGetChildrenAutomationPeer(Visual parent) { Invariant.Assert(parent != null); List children = null; iterate(parent, (IteratorCallback)delegate(AutomationPeer peer) { if (children == null) children = new List (); children.Add(peer); return (false); }); return children; } private delegate bool IteratorCallback(AutomationPeer peer); // private static bool iterate(Visual parent, IteratorCallback callback) { bool done = false; AutomationPeer peer = null; int count = parent.InternalVisualChildrenCount; for (int i = 0; i < count && !done; i++) { Visual child = parent.InternalGetVisualChild(i); if (child != null && child.CheckFlagsAnd(VisualFlags.IsUIElement) && (peer = CreatePeerForElement((UIElement)child)) != null) { done = callback(peer); } else { done = iterate(child, callback); } } return (done); } #endregion #region Private Fields private ListViewAutomationPeer _listviewAP; private int _column; private int _row; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
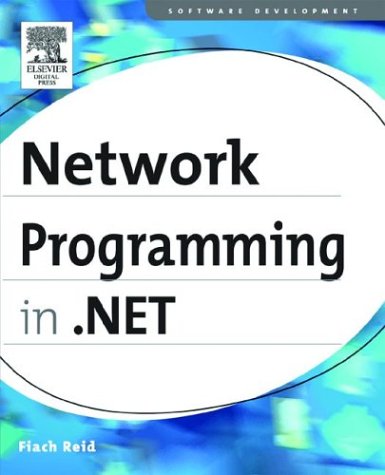
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebGetAttribute.cs
- XmlQueryRuntime.cs
- DynamicAttribute.cs
- ThicknessAnimationBase.cs
- CurrencyWrapper.cs
- BufferModeSettings.cs
- HttpResponseInternalWrapper.cs
- AliasedExpr.cs
- log.cs
- IISMapPath.cs
- XmlWrappingWriter.cs
- COM2IDispatchConverter.cs
- ObjectItemNoOpAssemblyLoader.cs
- HttpCapabilitiesBase.cs
- UrlPath.cs
- Animatable.cs
- CornerRadius.cs
- BoolExpr.cs
- XmlnsPrefixAttribute.cs
- MobileListItemCollection.cs
- DispatcherExceptionFilterEventArgs.cs
- SiteMapNodeItem.cs
- Pair.cs
- ToolStrip.cs
- ToolStripSplitButton.cs
- CodeTypeConstructor.cs
- DllNotFoundException.cs
- ScopelessEnumAttribute.cs
- Border.cs
- SimpleType.cs
- ActivitySurrogate.cs
- AnimatedTypeHelpers.cs
- WebPartEventArgs.cs
- DeflateStream.cs
- GlobalizationSection.cs
- ExchangeUtilities.cs
- AxHost.cs
- CacheSection.cs
- WorkerRequest.cs
- ProtocolsConfigurationHandler.cs
- translator.cs
- TableRow.cs
- CallInfo.cs
- CounterSampleCalculator.cs
- DESCryptoServiceProvider.cs
- EntityCommandExecutionException.cs
- InputBindingCollection.cs
- D3DImage.cs
- FontNamesConverter.cs
- ThumbAutomationPeer.cs
- EntityKey.cs
- LinkLabel.cs
- PointConverter.cs
- ModelPropertyCollectionImpl.cs
- ResizeGrip.cs
- DataSourceCacheDurationConverter.cs
- WCFModelStrings.Designer.cs
- HandlerWithFactory.cs
- ParserOptions.cs
- DataTemplateKey.cs
- DateTimeFormat.cs
- ProfileBuildProvider.cs
- Line.cs
- NetworkCredential.cs
- ObjectItemAttributeAssemblyLoader.cs
- AppModelKnownContentFactory.cs
- DataGridViewComboBoxColumn.cs
- HttpGetClientProtocol.cs
- ISessionStateStore.cs
- MissingMemberException.cs
- PropertyGridCommands.cs
- Propagator.JoinPropagator.JoinPredicateVisitor.cs
- CellRelation.cs
- NetCodeGroup.cs
- HitTestWithPointDrawingContextWalker.cs
- CodeTypeReference.cs
- ErrorRuntimeConfig.cs
- ToolStripItemTextRenderEventArgs.cs
- DragCompletedEventArgs.cs
- TriggerActionCollection.cs
- DesignerFrame.cs
- PropertyItemInternal.cs
- UnSafeCharBuffer.cs
- parserscommon.cs
- SystemColorTracker.cs
- UserControlCodeDomTreeGenerator.cs
- ColumnMapProcessor.cs
- IPAddressCollection.cs
- EntityConnection.cs
- TemplateNameScope.cs
- AdPostCacheSubstitution.cs
- __Filters.cs
- RootAction.cs
- mediaeventargs.cs
- Models.cs
- PropertyStore.cs
- TypeUtils.cs
- RegexGroupCollection.cs
- WSFederationHttpSecurityElement.cs
- OrderByQueryOptionExpression.cs