Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / Tools / xws_reg / System / ServiceModel / Install / ServiceHandle.cs / 1 / ServiceHandle.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Install { using Microsoft.Win32.SafeHandles; using System; using System.ComponentModel; using System.Diagnostics; using System.Runtime.ConstrainedExecution; using System.Runtime.InteropServices; using System.Security.AccessControl; using System.Text; internal class ServiceHandle : SafeHandleZeroOrMinusOneIsInvalid { internal ServiceHandle() : base(true) { } internal void ChangeServiceConfig(int serviceType, string binaryPathName, string dependencies, string displayName) { #pragma warning suppress 56523 // [....]; Win32Exception default constructor calls Marshal.GetLastWin32Error() internally. if (!NativeMethods.ChangeServiceConfig(this, serviceType, NativeMethods.SERVICE_NO_CHANGE, NativeMethods.SERVICE_NO_CHANGE, binaryPathName, null, IntPtr.Zero, dependencies, null, null, displayName)) { throw new Win32Exception(); } } internal void Delete() { #pragma warning suppress 56523 // [....]; Win32Exception default constructor calls Marshal.GetLastWin32Error() internally. if (!NativeMethods.DeleteService(this)) { throw new Win32Exception(); } } internal QUERY_SERVICE_CONFIG QueryServiceConfig() { QUERY_SERVICE_CONFIG serviceConfig = new QUERY_SERVICE_CONFIG(); int win32Error = ErrorCodes.NoError; int bufferSize = 0; using (QueryServiceConfigHandle serviceConfigHandle = new QueryServiceConfigHandle(IntPtr.Zero)) { NativeMethods.QueryServiceConfig(this, serviceConfigHandle, 0, out bufferSize); win32Error = Marshal.GetLastWin32Error(); if (ErrorCodes.ErrorInsufficientBuffer != win32Error) { throw new Win32Exception(win32Error); } } using (QueryServiceConfigHandle serviceConfigHandle = new QueryServiceConfigHandle(bufferSize)) { if (NativeMethods.QueryServiceConfig(this, serviceConfigHandle, bufferSize, out bufferSize)) { serviceConfig = serviceConfigHandle.ServiceConfig; } else { win32Error = Marshal.GetLastWin32Error(); throw new Win32Exception(win32Error); } } return serviceConfig; } protected override bool ReleaseHandle() { #pragma warning suppress 56523 // [....]; Win32Exception default constructor calls Marshal.GetLastWin32Error() internally. bool retVal = NativeMethods.CloseServiceHandle(handle); if (!retVal) { throw new Win32Exception(); } return retVal; } internal void SetDescription(string description) { SERVICE_DESCRIPTION serviceDescription; serviceDescription.lpDescription = description; #pragma warning suppress 56523 // [....]; Win32Exception default constructor calls Marshal.GetLastWin32Error() internally. if (!NativeMethods.ChangeServiceDescription(this, NativeMethods.SERVICE_CONFIG_DESCRIPTION, ref serviceDescription)) { throw new Win32Exception(); } } internal unsafe void SetFailureActions(TimeSpan resetPeriod, TimeSpan[] restartPeriods) { SERVICE_FAILURE_ACTIONS failureActions = new SERVICE_FAILURE_ACTIONS(); Debug.Assert(resetPeriod.TotalSeconds <= uint.MaxValue); Debug.Assert(resetPeriod.TotalSeconds >= 0); failureActions.dwResetPeriod = (uint) resetPeriod.TotalSeconds; SC_ACTION[] actions = null; if (restartPeriods != null) { actions = new SC_ACTION[restartPeriods.Length + 1]; for (int i = 0; i < restartPeriods.Length; ++i) { Debug.Assert(restartPeriods[i].TotalMilliseconds <= uint.MaxValue); Debug.Assert(restartPeriods[i].TotalMilliseconds >= 0); actions[i].Type = NativeMethods.SC_ACTION_RESTART; actions[i].Delay = (uint) restartPeriods[i].TotalMilliseconds; } actions[restartPeriods.Length].Type = NativeMethods.SC_ACTION_NONE; failureActions.cActions = (uint) actions.Length; } fixed (SC_ACTION *actionsPtr = actions) { failureActions.lpsaActions = actionsPtr; #pragma warning suppress 56523 // [....]; Win32Exception default constructor calls Marshal.GetLastWin32Error() internally. if (!NativeMethods.ChangeFailureActions(this, NativeMethods.SERVICE_CONFIG_FAILURE_ACTIONS, ref failureActions)) { throw new Win32Exception(); } } } internal void SetSecurityDescriptor(string descriptor) { RawSecurityDescriptor rawDescriptor = new RawSecurityDescriptor(descriptor); Debug.Assert(rawDescriptor.Group == null); Debug.Assert(rawDescriptor.Owner == null); Debug.Assert(rawDescriptor.DiscretionaryAcl != null); Debug.Assert(rawDescriptor.SystemAcl != null); byte[] binaryDescriptor = new byte[rawDescriptor.BinaryLength]; rawDescriptor.GetBinaryForm(binaryDescriptor, 0); #pragma warning suppress 56523 // [....]; Win32Exception default constructor calls Marshal.GetLastWin32Error() internally. if (!NativeMethods.SetServiceObjectSecurity(this, NativeMethods.DACL_SECURITY_INFORMATION | NativeMethods.SACL_SECURITY_INFORMATION, binaryDescriptor)) { throw new Win32Exception(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
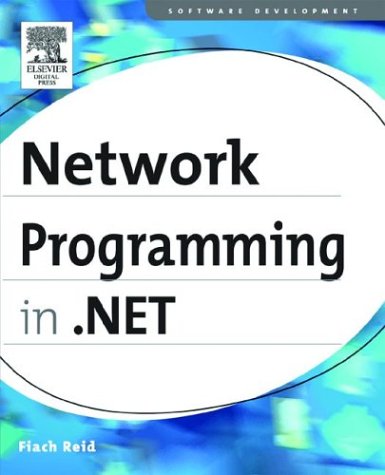
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GatewayIPAddressInformationCollection.cs
- CodeTypeDeclaration.cs
- ConfigXmlSignificantWhitespace.cs
- BuildResult.cs
- TextBoxAutoCompleteSourceConverter.cs
- ShapeTypeface.cs
- FigureHelper.cs
- ViewKeyConstraint.cs
- BasicHttpBindingElement.cs
- DefaultClaimSet.cs
- BaseProcessProtocolHandler.cs
- PresentationAppDomainManager.cs
- TableLayout.cs
- hresults.cs
- ToolStripSystemRenderer.cs
- EventManager.cs
- CodeTypeDeclarationCollection.cs
- OleServicesContext.cs
- PropertyEntry.cs
- ExtentKey.cs
- CacheChildrenQuery.cs
- EntityDesignerUtils.cs
- TransactionTable.cs
- SqlDataRecord.cs
- EdmItemCollection.cs
- PlanCompiler.cs
- LateBoundBitmapDecoder.cs
- XamlTreeBuilder.cs
- DefaultWorkflowSchedulerService.cs
- Comparer.cs
- Relationship.cs
- SamlAuthorizationDecisionStatement.cs
- SynchronizedMessageSource.cs
- Visual.cs
- SBCSCodePageEncoding.cs
- CommandBinding.cs
- StreamAsIStream.cs
- DbConvert.cs
- DetailsViewPageEventArgs.cs
- BatchParser.cs
- BmpBitmapEncoder.cs
- Encoder.cs
- EasingFunctionBase.cs
- DataSourceXmlElementAttribute.cs
- HttpStaticObjectsCollectionWrapper.cs
- DatatypeImplementation.cs
- DisplayInformation.cs
- TextDecorationCollection.cs
- FacetChecker.cs
- DecimalAnimation.cs
- GenericWebPart.cs
- EntitySqlQueryBuilder.cs
- JoinCqlBlock.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- EntitySqlQueryCacheKey.cs
- RoutedEventHandlerInfo.cs
- ExeContext.cs
- DateTimeFormat.cs
- Italic.cs
- TextProperties.cs
- StateWorkerRequest.cs
- WinCategoryAttribute.cs
- Compiler.cs
- _HelperAsyncResults.cs
- ColorTranslator.cs
- DiagnosticStrings.cs
- VisualTreeUtils.cs
- ThumbAutomationPeer.cs
- CellPartitioner.cs
- SplineKeyFrames.cs
- AttributeCollection.cs
- PeekCompletedEventArgs.cs
- LabelAutomationPeer.cs
- BreadCrumbTextConverter.cs
- HtmlElementErrorEventArgs.cs
- PiiTraceSource.cs
- WebPartDisplayMode.cs
- DataRelationCollection.cs
- AggregationMinMaxHelpers.cs
- SessionParameter.cs
- DefaultAuthorizationContext.cs
- FileRegion.cs
- ConfigurationValues.cs
- InstallerTypeAttribute.cs
- BufferedStream.cs
- TypedTableBaseExtensions.cs
- XmlNodeList.cs
- DbConnectionPool.cs
- AdornerDecorator.cs
- HTMLTagNameToTypeMapper.cs
- ByteConverter.cs
- ListParagraph.cs
- NavigationEventArgs.cs
- CategoryAttribute.cs
- IdentifierCollection.cs
- XmlSchemaFacet.cs
- RepeatBehavior.cs
- TextInfo.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- _ConnectOverlappedAsyncResult.cs