Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / ControlPropertyNameConverter.cs / 1 / ControlPropertyNameConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Globalization; using System.Reflection; using System.Web.Util; using System.Security.Permissions; ////// TypeConverter for ControlParameter's PropertyName property. /// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class ControlPropertyNameConverter : StringConverter { ////// Returns a list of all the propery names for a given control. /// private string[] GetPropertyNames(Control control) { ArrayList array = new ArrayList(); PropertyDescriptorCollection pdc = TypeDescriptor.GetProperties(control.GetType()); foreach (PropertyDescriptor desc in pdc) { array.Add(desc.Name); } array.Sort(Comparer.Default); return (string[])array.ToArray(typeof(string)); } ////// Returns a collection of standard values retrieved from the context specified /// by the specified type descriptor. /// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { if (context == null) { return null; } // Get ControlID ControlParameter param = (ControlParameter)context.Instance; string controlID = param.ControlID; // Check that we actually have a control ID if (String.IsNullOrEmpty(controlID)) return null; // Get designer host IDesignerHost host = (IDesignerHost)context.GetService(typeof(IDesignerHost)); Debug.Assert(host != null, "Unable to get IDesignerHost in ControlPropertyNameConverter"); if (host == null) return null; // Get control ComponentCollection allComponents = host.Container.Components; Control control = allComponents[controlID] as Control; if (control == null) return null; string[] propertyNames = GetPropertyNames(control); return new StandardValuesCollection(propertyNames); } ////// Gets whether or not the context specified contains exclusive standard values. /// public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return false; } ////// Gets whether or not the specified context contains supported standard values. /// public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { return (context != null); } } }
Link Menu
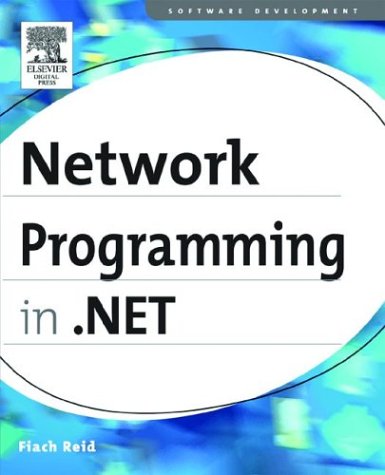
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HMACSHA384.cs
- MetadataArtifactLoaderCompositeFile.cs
- ListViewGroupItemCollection.cs
- WindowsToolbar.cs
- SqlProviderServices.cs
- SqlError.cs
- DeferredSelectedIndexReference.cs
- SqlFunctions.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- WindowPattern.cs
- PointCollection.cs
- graph.cs
- DataGridViewCellParsingEventArgs.cs
- HtmlShim.cs
- TickBar.cs
- DataTransferEventArgs.cs
- XmlElementAttribute.cs
- UpdatePanel.cs
- SizeChangedInfo.cs
- TraceContextEventArgs.cs
- SecurityTokenResolver.cs
- PaintEvent.cs
- TargetFrameworkUtil.cs
- CancellationHandler.cs
- TextLineBreak.cs
- HitTestFilterBehavior.cs
- counter.cs
- ReachUIElementCollectionSerializer.cs
- ConfigViewGenerator.cs
- PingReply.cs
- SqlDataSourceDesigner.cs
- EmissiveMaterial.cs
- WSHttpBindingBase.cs
- WorkflowInstanceExtensionManager.cs
- RequestCachePolicyConverter.cs
- GenericUI.cs
- DataSet.cs
- AsnEncodedData.cs
- DbSource.cs
- ValueConversionAttribute.cs
- ConfigXmlText.cs
- OdbcErrorCollection.cs
- MetadataItemEmitter.cs
- BinaryMethodMessage.cs
- Evaluator.cs
- CookieParameter.cs
- DependencyPropertyChangedEventArgs.cs
- Comparer.cs
- ListenerConnectionDemuxer.cs
- XamlWriter.cs
- SqlUserDefinedTypeAttribute.cs
- AmbientLight.cs
- Point3D.cs
- LogEntryHeaderSerializer.cs
- MemoryStream.cs
- UpDownBaseDesigner.cs
- JournalNavigationScope.cs
- BroadcastEventHelper.cs
- EngineSiteSapi.cs
- ErrorItem.cs
- FloatUtil.cs
- ActivityExecutor.cs
- _NetworkingPerfCounters.cs
- RelationshipType.cs
- FileDialog_Vista.cs
- SqlDataSource.cs
- DataGridViewHeaderCell.cs
- cryptoapiTransform.cs
- OleAutBinder.cs
- SelectManyQueryOperator.cs
- WmlCommandAdapter.cs
- Floater.cs
- FtpCachePolicyElement.cs
- HttpPostProtocolReflector.cs
- UnsafeNativeMethods.cs
- Utilities.cs
- ValueTypeFixupInfo.cs
- DataGridViewAutoSizeColumnsModeEventArgs.cs
- RegexMatchCollection.cs
- CmsUtils.cs
- XmlChildNodes.cs
- GridToolTip.cs
- CompositeFontFamily.cs
- ToolBar.cs
- Canonicalizers.cs
- DescendantQuery.cs
- DataBindingList.cs
- SqlDataSourceStatusEventArgs.cs
- FileDialogCustomPlaces.cs
- COMException.cs
- AudioBase.cs
- SystemUdpStatistics.cs
- Vector3DCollection.cs
- CheckBox.cs
- FormViewPagerRow.cs
- ImageMapEventArgs.cs
- FloaterBaseParaClient.cs
- RowSpanVector.cs
- SafeNativeMethods.cs
- SqlDuplicator.cs