Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Data / System / Data / ProviderBase / WrappedIUnknown.cs / 1 / WrappedIUnknown.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.ProviderBase { using System; using System.Data.Common; using System.Runtime.CompilerServices; using System.Runtime.ConstrainedExecution; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using System.Threading; // We wrap the interface as a native IUnknown IntPtr so that every // thread that creates a connection will fake the correct context when // in transactions, otherwise everything is marshalled. We do this // for two reasons: first for the connection pooler, this is a significant // performance gain, second for the OLE DB provider, it doesn't marshal. internal class WrappedIUnknown : SafeHandle { internal WrappedIUnknown() : base(IntPtr.Zero, true) { } internal WrappedIUnknown(object unknown) : this() { if (null != unknown) { RuntimeHelpers.PrepareConstrainedRegions(); try {} finally { base.handle = Marshal.GetIUnknownForObject(unknown); // } } } public override bool IsInvalid { get { return (IntPtr.Zero == base.handle); } } internal object ComWrapper() { // NOTE: Method, instead of property, to avoid being evaluated at // runtime in the debugger. object value = null; bool mustRelease = false; RuntimeHelpers.PrepareConstrainedRegions(); try { DangerousAddRef(ref mustRelease); IntPtr handle = DangerousGetHandle(); value = System.Runtime.Remoting.Services.EnterpriseServicesHelper.WrapIUnknownWithComObject(handle); } finally { if (mustRelease) { DangerousRelease(); } } return value; } override protected bool ReleaseHandle() { // NOTE: The SafeHandle class guarantees this will be called exactly once. IntPtr ptr = base.handle; base.handle = IntPtr.Zero; if (IntPtr.Zero != ptr) { Marshal.Release(ptr); } return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
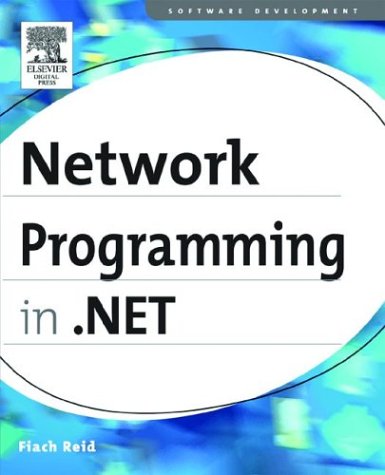
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WindowsListViewSubItem.cs
- URLAttribute.cs
- FixedDocumentPaginator.cs
- InputLanguage.cs
- PersistenceContextEnlistment.cs
- InputBindingCollection.cs
- EventsTab.cs
- OutKeywords.cs
- CancellableEnumerable.cs
- AsyncContentLoadedEventArgs.cs
- XmlSchemaElement.cs
- IApplicationTrustManager.cs
- HyperLinkStyle.cs
- XmlSecureResolver.cs
- DependencyObject.cs
- XamlSerializationHelper.cs
- XPathNode.cs
- BindingValueChangedEventArgs.cs
- DockPatternIdentifiers.cs
- BitmapEffectGeneralTransform.cs
- ServerIdentity.cs
- PathData.cs
- AutomationElementIdentifiers.cs
- PageStatePersister.cs
- Stack.cs
- PageTrueTypeFont.cs
- NodeFunctions.cs
- FrameworkContextData.cs
- LassoHelper.cs
- SqlTriggerContext.cs
- SchemaCreator.cs
- TablePattern.cs
- Point4D.cs
- NameSpaceExtractor.cs
- BaseTemplateParser.cs
- UidPropertyAttribute.cs
- SystemWebCachingSectionGroup.cs
- RectKeyFrameCollection.cs
- RowCache.cs
- StringUtil.cs
- FunctionImportElement.cs
- TableCellsCollectionEditor.cs
- XmlComplianceUtil.cs
- PropertyOrder.cs
- CuspData.cs
- XmlILAnnotation.cs
- SqlUdtInfo.cs
- WebControlsSection.cs
- HttpDebugHandler.cs
- NetworkStream.cs
- ForwardPositionQuery.cs
- InfocardInteractiveChannelInitializer.cs
- EntityDataSourceConfigureObjectContextPanel.cs
- WindowsFormsSectionHandler.cs
- COMException.cs
- XmlSchemaSimpleTypeRestriction.cs
- TrustManager.cs
- OperandQuery.cs
- StorageMappingItemCollection.cs
- NetSectionGroup.cs
- DragDeltaEventArgs.cs
- odbcmetadatacollectionnames.cs
- Debug.cs
- TranslateTransform3D.cs
- IfAction.cs
- _DisconnectOverlappedAsyncResult.cs
- DoWorkEventArgs.cs
- Schema.cs
- XmlAttribute.cs
- SBCSCodePageEncoding.cs
- VisualTarget.cs
- XamlStream.cs
- WebPartZoneCollection.cs
- AggregateException.cs
- AnimationClockResource.cs
- PackageProperties.cs
- Header.cs
- Aes.cs
- versioninfo.cs
- PrivilegeNotHeldException.cs
- Point3DConverter.cs
- TraceData.cs
- CodeExpressionRuleDeclaration.cs
- TextDecorationLocationValidation.cs
- KeyManager.cs
- _SslStream.cs
- PhysicalOps.cs
- ParameterToken.cs
- XmlJsonReader.cs
- NativeMethods.cs
- IntellisenseTextBox.cs
- MenuAutomationPeer.cs
- QueryTreeBuilder.cs
- SubclassTypeValidatorAttribute.cs
- RecordsAffectedEventArgs.cs
- XmlUnspecifiedAttribute.cs
- InputChannelAcceptor.cs
- TextTreeTextElementNode.cs
- TableItemProviderWrapper.cs
- HostDesigntimeLicenseContext.cs