Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / infocard / Client / System / IdentityModel / Selectors / InternalPolicyElement.cs / 1305376 / InternalPolicyElement.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IdentityModel.Selectors { using System; using System.Collections.Generic; using System.IO; using System.Runtime.InteropServices; using System.Text; using System.Xml; using Microsoft.InfoCards.Diagnostics; using IDT=Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary: // This is the managed representation of the native POLICY_ELEMENT struct. This internal version // knows how to Marshal itself and manages the native memory associated with a marshaled POLICY_ELEMENT. // internal class InternalPolicyElement : IDisposable { CardSpacePolicyElement m_element; NativePolicyElement m_nativeElement; IntPtr m_nativePtr; // // Parameters: // target - The target of the token being described. // parameters - describes the type of token required by the target. // public InternalPolicyElement( CardSpacePolicyElement element ) { m_nativePtr = IntPtr.Zero; if( null == element.Target ) { throw IDT.ThrowHelperArgumentNull( "PolicyElement.Target" ); } m_element = element; } public static int Size { get { return Marshal.SizeOf( typeof( NativePolicyElement ) ); } } // // Summary: // Marshals the PolicyElement to it's native format. // // Parameters: // ptr - A pointer to native memory in which to place the native format of the PolicyElement. Must be // a buffer atleast as large as this.Size. // public void DoMarshal( IntPtr ptr ) { string target = m_element.Target.OuterXml; string issuer = ""; IDT.DebugAssert( IntPtr.Zero == m_nativePtr, "Pointer already assigned" ); m_nativePtr = ptr; if( m_element.Issuer != null ) { issuer = m_element.Issuer.OuterXml; } string tokenParameters = string.Empty; if( null != m_element.Parameters ) { tokenParameters = CardSpaceSelector.XmlToString( m_element.Parameters ); } m_nativeElement.targetEndpointAddress = target; m_nativeElement.issuerEndpointAddress = issuer; m_nativeElement.issuedTokenParameters = tokenParameters; m_nativeElement.policyNoticeLink = null != m_element.PolicyNoticeLink ? m_element.PolicyNoticeLink.ToString() : null ; m_nativeElement.policyNoticeVersion = m_element.PolicyNoticeVersion; m_nativeElement.isManagedCardProvider = m_element.IsManagedIssuer; Marshal.StructureToPtr( m_nativeElement, ptr, false ); return; } public void Dispose() { Dispose( true ); } ~InternalPolicyElement() { Dispose( false ); } private void Dispose( bool disposing ) { if( IntPtr.Zero != m_nativePtr ) { Marshal.DestroyStructure( m_nativePtr, typeof( NativePolicyElement ) ); m_nativePtr = IntPtr.Zero; } if( disposing ) { GC.SuppressFinalize( this ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IdentityModel.Selectors { using System; using System.Collections.Generic; using System.IO; using System.Runtime.InteropServices; using System.Text; using System.Xml; using Microsoft.InfoCards.Diagnostics; using IDT=Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary: // This is the managed representation of the native POLICY_ELEMENT struct. This internal version // knows how to Marshal itself and manages the native memory associated with a marshaled POLICY_ELEMENT. // internal class InternalPolicyElement : IDisposable { CardSpacePolicyElement m_element; NativePolicyElement m_nativeElement; IntPtr m_nativePtr; // // Parameters: // target - The target of the token being described. // parameters - describes the type of token required by the target. // public InternalPolicyElement( CardSpacePolicyElement element ) { m_nativePtr = IntPtr.Zero; if( null == element.Target ) { throw IDT.ThrowHelperArgumentNull( "PolicyElement.Target" ); } m_element = element; } public static int Size { get { return Marshal.SizeOf( typeof( NativePolicyElement ) ); } } // // Summary: // Marshals the PolicyElement to it's native format. // // Parameters: // ptr - A pointer to native memory in which to place the native format of the PolicyElement. Must be // a buffer atleast as large as this.Size. // public void DoMarshal( IntPtr ptr ) { string target = m_element.Target.OuterXml; string issuer = ""; IDT.DebugAssert( IntPtr.Zero == m_nativePtr, "Pointer already assigned" ); m_nativePtr = ptr; if( m_element.Issuer != null ) { issuer = m_element.Issuer.OuterXml; } string tokenParameters = string.Empty; if( null != m_element.Parameters ) { tokenParameters = CardSpaceSelector.XmlToString( m_element.Parameters ); } m_nativeElement.targetEndpointAddress = target; m_nativeElement.issuerEndpointAddress = issuer; m_nativeElement.issuedTokenParameters = tokenParameters; m_nativeElement.policyNoticeLink = null != m_element.PolicyNoticeLink ? m_element.PolicyNoticeLink.ToString() : null ; m_nativeElement.policyNoticeVersion = m_element.PolicyNoticeVersion; m_nativeElement.isManagedCardProvider = m_element.IsManagedIssuer; Marshal.StructureToPtr( m_nativeElement, ptr, false ); return; } public void Dispose() { Dispose( true ); } ~InternalPolicyElement() { Dispose( false ); } private void Dispose( bool disposing ) { if( IntPtr.Zero != m_nativePtr ) { Marshal.DestroyStructure( m_nativePtr, typeof( NativePolicyElement ) ); m_nativePtr = IntPtr.Zero; } if( disposing ) { GC.SuppressFinalize( this ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
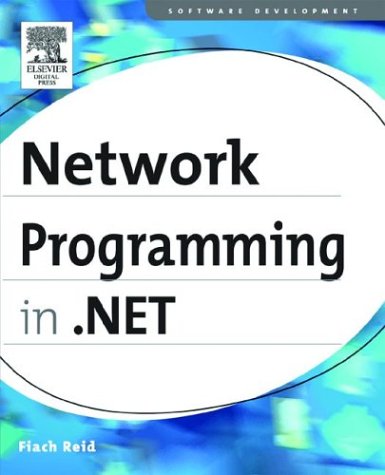
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ThreadStateException.cs
- FormViewPageEventArgs.cs
- AxisAngleRotation3D.cs
- ChineseLunisolarCalendar.cs
- ResourceCodeDomSerializer.cs
- ItemList.cs
- ListBindableAttribute.cs
- QuadraticBezierSegment.cs
- IxmlLineInfo.cs
- AttachInfo.cs
- CommandEventArgs.cs
- SqlRewriteScalarSubqueries.cs
- TypeCollectionPropertyEditor.cs
- Select.cs
- StreamGeometry.cs
- PriorityQueue.cs
- SynchronizedInputPattern.cs
- _DisconnectOverlappedAsyncResult.cs
- XmlEncoding.cs
- UserControlParser.cs
- ProfileSettingsCollection.cs
- DrawingState.cs
- Stack.cs
- ConfigXmlElement.cs
- ScalarConstant.cs
- Socket.cs
- RtType.cs
- SQLBinaryStorage.cs
- CodeNamespaceImportCollection.cs
- MouseWheelEventArgs.cs
- ImplicitInputBrush.cs
- Message.cs
- CacheMemory.cs
- ResXBuildProvider.cs
- StyleModeStack.cs
- CodeAccessSecurityEngine.cs
- ContentHostHelper.cs
- RtfNavigator.cs
- InputProviderSite.cs
- precedingquery.cs
- SafeTokenHandle.cs
- ISessionStateStore.cs
- HttpModuleCollection.cs
- OrthographicCamera.cs
- SyndicationElementExtension.cs
- GeneralTransform3DTo2D.cs
- ScriptControlManager.cs
- SingleConverter.cs
- _SecureChannel.cs
- TabControlAutomationPeer.cs
- XmlWrappingWriter.cs
- SQLResource.cs
- NativeMethodsOther.cs
- Console.cs
- DocumentEventArgs.cs
- RotationValidation.cs
- ExtenderControl.cs
- FontStyles.cs
- ItemList.cs
- PassportAuthentication.cs
- RegisteredHiddenField.cs
- RoutedEventHandlerInfo.cs
- Int64Animation.cs
- PrimitiveXmlSerializers.cs
- RecognizedAudio.cs
- ExpressionNode.cs
- ParseNumbers.cs
- CodeNamespaceImportCollection.cs
- AttachedPropertyDescriptor.cs
- TextParagraphView.cs
- ViewStateException.cs
- SqlBuilder.cs
- SyntaxCheck.cs
- DataGridViewSelectedCellCollection.cs
- SystemInfo.cs
- MimeObjectFactory.cs
- Gdiplus.cs
- PropertyExpression.cs
- StringConverter.cs
- ContextMenu.cs
- ExclusiveCanonicalizationTransform.cs
- ConnectionStringsExpressionBuilder.cs
- IDQuery.cs
- DiscoveryMessageSequence11.cs
- ValidationSummary.cs
- CodeChecksumPragma.cs
- SpeakInfo.cs
- CommentEmitter.cs
- FormsAuthenticationModule.cs
- ListControl.cs
- NotifyIcon.cs
- SqlNotificationRequest.cs
- IntPtr.cs
- InkCanvasFeedbackAdorner.cs
- ShapeTypeface.cs
- DBNull.cs
- FixedHyperLink.cs
- KeyInfo.cs
- SQLUtility.cs
- BufferBuilder.cs