Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Core.Presentation / System / Activities / Presentation / TypeCollectionPropertyEditor.cs / 1407647 / TypeCollectionPropertyEditor.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation { using System.Activities.Core.Presentation.Themes; using System.Activities.Presentation.Model; using System.Activities.Presentation.Converters; using System.Activities.Presentation.PropertyEditing; using System.Activities.Presentation.View; using System.Windows; using System.Runtime; using System.Collections.Generic; using System.Reflection; using System.Diagnostics.CodeAnalysis; using System.ComponentModel; using System.Collections; sealed class TypeCollectionPropertyEditor : DialogPropertyValueEditor { public const string AllowDuplicate = "AllowDuplicate"; public TypeCollectionPropertyEditor() { this.InlineEditorTemplate = EditorCategoryTemplateDictionary.Instance.GetCategoryTemplate("TypeCollection_InlineTemplate"); } public override void ShowDialog(PropertyValue propertyValue, IInputElement commandSource) { ModelPropertyEntryToOwnerActivityConverter propertyEntryConverter = new ModelPropertyEntryToOwnerActivityConverter(); ModelItem activityModelItem = (ModelItem)propertyEntryConverter.Convert(propertyValue.ParentProperty, typeof(ModelItem), false, null); ModelItem parentModelItem = (ModelItem)propertyEntryConverter.Convert(propertyValue.ParentProperty, typeof(ModelItem), true, null); EditingContext context = ((IModelTreeItem)activityModelItem).ModelTreeManager.Context; ModelItemCollection inputData = parentModelItem.Properties[propertyValue.ParentProperty.PropertyName].Collection; IEnumerablerawInputData = inputData.GetCurrentValue() as IEnumerable ; Fx.Assert(rawInputData != null, "rawInputData is null or is not IEnumerable ."); ModelProperty editingProperty = activityModelItem.Properties[propertyValue.ParentProperty.PropertyName]; bool allowDuplication = EditorOptionsAttribute.GetOptionValue(editingProperty.Attributes, TypeCollectionPropertyEditor.AllowDuplicate, true); EditorWindow editorWindow = new EditorWindow(activityModelItem, rawInputData, context, activityModelItem.View, allowDuplication); if (editorWindow.ShowOkCancel()) { using (var commitEditingScope = inputData.BeginEdit(System.Activities.Core.Presentation.SR.ChangeTypeCollectionEditingScopeDesc)) { inputData.Clear(); foreach (Type i in ((TypeCollectionDesigner)editorWindow.Content).UpdatedTypeCollection) { inputData.Add(i); } commitEditingScope.Complete(); } } } sealed class EditorWindow : WorkflowElementDialog { public EditorWindow(ModelItem activity, IEnumerable data, EditingContext context, DependencyObject owner, bool allowDuplicate) { this.ModelItem = activity; this.Context = context; this.Owner = owner; this.EnableMaximizeButton = false; this.EnableMinimizeButton = false; this.MinWidth = 450; this.MinHeight = 260; this.WindowResizeMode = ResizeMode.CanResize; this.WindowSizeToContent = SizeToContent.Manual; TypeCollectionDesigner content = new TypeCollectionDesigner() { Context = context, InitialTypeCollection = data, AllowDuplicate = allowDuplicate, ParentDialog = this, }; this.Title = (string)content.Resources["controlTitle"]; this.Content = content; this.OnOk = content.OnOK; this.HelpKeyword = HelpKeywords.TypeCollectionEditor; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
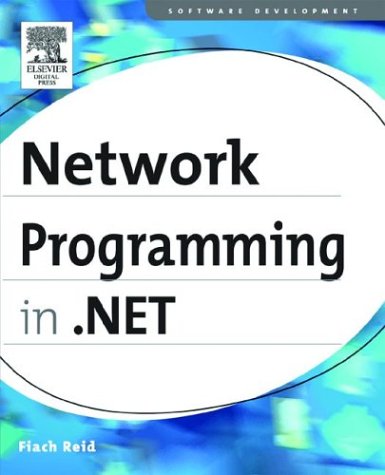
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ISAPIRuntime.cs
- DataGridViewRowsAddedEventArgs.cs
- BaseComponentEditor.cs
- InvalidDataContractException.cs
- NameTable.cs
- DataGridViewAccessibleObject.cs
- ObjectDataSourceChooseTypePanel.cs
- PatternMatcher.cs
- SafeFileMappingHandle.cs
- HashHelper.cs
- NavigationPropertyEmitter.cs
- JapaneseCalendar.cs
- SystemWebCachingSectionGroup.cs
- RootBrowserWindowProxy.cs
- GenericAuthenticationEventArgs.cs
- ScriptResourceAttribute.cs
- DropDownButton.cs
- TextSelectionProcessor.cs
- RefreshEventArgs.cs
- Repeater.cs
- DesignerVerbToolStripMenuItem.cs
- UTF32Encoding.cs
- Parameter.cs
- CollectionViewGroupInternal.cs
- ObjectStorage.cs
- ImmutablePropertyDescriptorGridEntry.cs
- HttpResponseBase.cs
- UnmanagedMemoryStream.cs
- SingleConverter.cs
- AspCompat.cs
- X509Utils.cs
- PointAnimation.cs
- DateTimeOffsetStorage.cs
- ConfigurationSectionCollection.cs
- ErrorProvider.cs
- SortedList.cs
- ConfigurationErrorsException.cs
- XmlNode.cs
- DynamicILGenerator.cs
- CryptographicAttribute.cs
- StringUtil.cs
- DataSourceCacheDurationConverter.cs
- SizeAnimationBase.cs
- WindowsIdentity.cs
- TemplateBamlTreeBuilder.cs
- ObjectAnimationBase.cs
- QuadraticBezierSegment.cs
- DrawingState.cs
- EventMappingSettings.cs
- ProfilePropertySettings.cs
- MiniAssembly.cs
- XmlUrlResolver.cs
- DataComponentMethodGenerator.cs
- CodeRemoveEventStatement.cs
- DataIdProcessor.cs
- FloatUtil.cs
- AddInBase.cs
- Common.cs
- SharedPerformanceCounter.cs
- IndexedGlyphRun.cs
- ObjectSet.cs
- TextParagraphProperties.cs
- VBCodeProvider.cs
- ListenDesigner.cs
- ImageMap.cs
- PropertyReferenceSerializer.cs
- Utils.cs
- PropertyTab.cs
- Latin1Encoding.cs
- RichTextBox.cs
- ChangeInterceptorAttribute.cs
- Listbox.cs
- DataGridViewImageColumn.cs
- QueryStoreStatusRequest.cs
- WinInetCache.cs
- RequestResizeEvent.cs
- ProfileEventArgs.cs
- OrthographicCamera.cs
- HttpRuntime.cs
- BamlLocalizabilityResolver.cs
- CompilationUnit.cs
- Speller.cs
- GAC.cs
- PropagationProtocolsTracing.cs
- FileSystemInfo.cs
- CompiledELinqQueryState.cs
- SafeBuffer.cs
- UpdateRecord.cs
- DockProviderWrapper.cs
- WbmpConverter.cs
- SystemSounds.cs
- ToolTip.cs
- OletxDependentTransaction.cs
- Lease.cs
- ValidationPropertyAttribute.cs
- FixedSOMSemanticBox.cs
- ContentTextAutomationPeer.cs
- ContainerControl.cs
- WindowsStatusBar.cs
- LinkDescriptor.cs