Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Markup / Localizer / BamlLocalizabilityResolver.cs / 1 / BamlLocalizabilityResolver.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: BamlLocalizabilityResolver class // // History: // 11/29/2004: Garyyang Created the file // 03/24/2005 Garyyang Move to System.Windows.Markup.Localizer namespace //--------------------------------------------------------------------------- namespace System.Windows.Markup.Localizer { ////// BamlLocalizabilityResolver class. It is implemented by Baml localization API client to provide /// Localizability settings to Baml content /// public abstract class BamlLocalizabilityResolver { ////// Obtain the localizability of an element and /// the whether the element can be formatted inline. /// The method is called when extracting localizable resources from baml /// /// Full assembly name /// Full class name ///ElementLocalizability public abstract ElementLocalizability GetElementLocalizability( string assembly, string className ); ////// Obtain the localizability of a property /// The method is called when extracting localizable resources from baml /// /// Full assembly name /// Full class name that contains the property defintion /// property name ///LocalizabilityAttribute for the property public abstract LocalizabilityAttribute GetPropertyLocalizability( string assembly, string className, string property ); ////// Return full class name of a formatting tag that hasn't been encountered in Baml /// The method is called when applying translations to the localized baml /// /// formatting tag name ///Full name of the class that is formatted inline public abstract string ResolveFormattingTagToClass( string formattingTag ); ////// Return full name of the assembly that contains the class definition /// /// Full class name ///Full name of the assembly containing the class public abstract string ResolveAssemblyFromClass( string className ); } ////// The localizability information for an element /// public class ElementLocalizability { private string _formattingTag; private LocalizabilityAttribute _attribute; ////// Constructor /// public ElementLocalizability() { } ////// Constructor /// /// formatting tag, give a non-empty value to indicate that the class is formatted inline /// LocalizabilityAttribute for the class public ElementLocalizability(string formattingTag, LocalizabilityAttribute attribute) { _formattingTag = formattingTag; _attribute = attribute; } ////// Set or Get the formatting tag /// public string FormattingTag { get { return _formattingTag; } set { _formattingTag = value; } } ////// Set or get the LocalizabilityAttribute /// public LocalizabilityAttribute Attribute { get { return _attribute; } set { _attribute = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: BamlLocalizabilityResolver class // // History: // 11/29/2004: Garyyang Created the file // 03/24/2005 Garyyang Move to System.Windows.Markup.Localizer namespace //--------------------------------------------------------------------------- namespace System.Windows.Markup.Localizer { ////// BamlLocalizabilityResolver class. It is implemented by Baml localization API client to provide /// Localizability settings to Baml content /// public abstract class BamlLocalizabilityResolver { ////// Obtain the localizability of an element and /// the whether the element can be formatted inline. /// The method is called when extracting localizable resources from baml /// /// Full assembly name /// Full class name ///ElementLocalizability public abstract ElementLocalizability GetElementLocalizability( string assembly, string className ); ////// Obtain the localizability of a property /// The method is called when extracting localizable resources from baml /// /// Full assembly name /// Full class name that contains the property defintion /// property name ///LocalizabilityAttribute for the property public abstract LocalizabilityAttribute GetPropertyLocalizability( string assembly, string className, string property ); ////// Return full class name of a formatting tag that hasn't been encountered in Baml /// The method is called when applying translations to the localized baml /// /// formatting tag name ///Full name of the class that is formatted inline public abstract string ResolveFormattingTagToClass( string formattingTag ); ////// Return full name of the assembly that contains the class definition /// /// Full class name ///Full name of the assembly containing the class public abstract string ResolveAssemblyFromClass( string className ); } ////// The localizability information for an element /// public class ElementLocalizability { private string _formattingTag; private LocalizabilityAttribute _attribute; ////// Constructor /// public ElementLocalizability() { } ////// Constructor /// /// formatting tag, give a non-empty value to indicate that the class is formatted inline /// LocalizabilityAttribute for the class public ElementLocalizability(string formattingTag, LocalizabilityAttribute attribute) { _formattingTag = formattingTag; _attribute = attribute; } ////// Set or Get the formatting tag /// public string FormattingTag { get { return _formattingTag; } set { _formattingTag = value; } } ////// Set or get the LocalizabilityAttribute /// public LocalizabilityAttribute Attribute { get { return _attribute; } set { _attribute = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
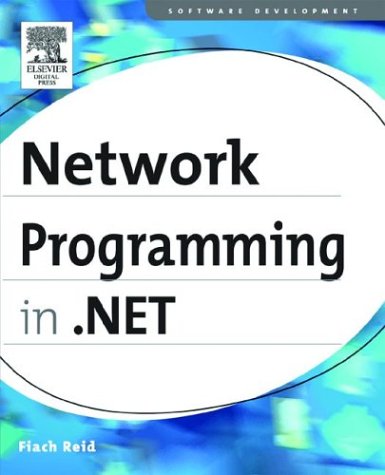
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MimeObjectFactory.cs
- WindowsAuthenticationEventArgs.cs
- ViewValidator.cs
- UTF32Encoding.cs
- InvokeProviderWrapper.cs
- AnnotationDocumentPaginator.cs
- OpenTypeLayout.cs
- RC2.cs
- AssemblyBuilder.cs
- TablePattern.cs
- Model3DCollection.cs
- RangeEnumerable.cs
- WhitespaceRule.cs
- DetailsViewModeEventArgs.cs
- SqlPersonalizationProvider.cs
- HttpListenerResponse.cs
- TraceUtility.cs
- SqlProfileProvider.cs
- InsufficientMemoryException.cs
- ConfigurationStrings.cs
- ApplicationSecurityManager.cs
- XmlSchemaCollection.cs
- Duration.cs
- ResolveMatchesApril2005.cs
- ObjectDataSourceEventArgs.cs
- Int32.cs
- InternalException.cs
- DateTimeStorage.cs
- ExceptionNotification.cs
- ChildChangedEventArgs.cs
- HtmlTernaryTree.cs
- AdornerPresentationContext.cs
- OdbcCommand.cs
- DetailsViewDeleteEventArgs.cs
- WebSysDescriptionAttribute.cs
- BitmapFrame.cs
- SqlColumnizer.cs
- BitmapMetadataEnumerator.cs
- ObjectQueryState.cs
- DrawingContextWalker.cs
- NameValueFileSectionHandler.cs
- ReliabilityContractAttribute.cs
- WebPartsSection.cs
- RowParagraph.cs
- HtmlButton.cs
- ConfigXmlElement.cs
- CompilerTypeWithParams.cs
- MemoryFailPoint.cs
- Double.cs
- COM2ComponentEditor.cs
- ApplicationContext.cs
- ToolStripLabel.cs
- XmlEntityReference.cs
- ZipIOLocalFileDataDescriptor.cs
- FrameworkTemplate.cs
- FormattedText.cs
- AttributeEmitter.cs
- AsymmetricSignatureDeformatter.cs
- EarlyBoundInfo.cs
- commandenforcer.cs
- XmlDocumentType.cs
- remotingproxy.cs
- HorizontalAlignConverter.cs
- FormatterConverter.cs
- NavigateEvent.cs
- TitleStyle.cs
- RuleSettings.cs
- CapabilitiesRule.cs
- DataMemberConverter.cs
- ListItemCollection.cs
- Decimal.cs
- ResumeStoryboard.cs
- Matrix3D.cs
- DataBoundControl.cs
- OleCmdHelper.cs
- DtrList.cs
- HttpRequestCacheValidator.cs
- XPathNodeInfoAtom.cs
- DecoderReplacementFallback.cs
- SynchronizedInputProviderWrapper.cs
- CodeEntryPointMethod.cs
- TextPenaltyModule.cs
- DbMetaDataFactory.cs
- CommandHelper.cs
- Parameter.cs
- CompositeFontFamily.cs
- DiscardableAttribute.cs
- WindowsFormsSynchronizationContext.cs
- PngBitmapDecoder.cs
- Avt.cs
- CreateInstanceBinder.cs
- JsonQNameDataContract.cs
- XamlDesignerSerializationManager.cs
- X509Logo.cs
- CommandID.cs
- StatusStrip.cs
- SHA512.cs
- SafeFileMappingHandle.cs
- InstalledFontCollection.cs
- ButtonColumn.cs