Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Net / System / Net / HttpListenerResponse.cs / 2 / HttpListenerResponse.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System; using System.Collections.Generic; using System.Globalization; using System.IO; using System.Net.Sockets; using System.Runtime.InteropServices; using System.Text; using System.Threading; using System.ComponentModel; public sealed unsafe class HttpListenerResponse : /* BaseHttpResponse, */ IDisposable { enum ResponseState { Created, ComputedHeaders, SentHeaders, Closed, } // from ndp\fx\src\xsp\system\web\workerrequest.cs static readonly string[][] s_HTTPStatusDescriptions = new string[][] { null, new string[] { /* 100 */ "Continue", /* 101 */ "Switching Protocols", /* 102 */ "Processing" }, new string[] { /* 200 */ "OK", /* 201 */ "Created", /* 202 */ "Accepted", /* 203 */ "Non-Authoritative Information", /* 204 */ "No Content", /* 205 */ "Reset Content", /* 206 */ "Partial Content", /* 207 */ "Multi-Status" }, new string[] { /* 300 */ "Multiple Choices", /* 301 */ "Moved Permanently", /* 302 */ "Found", /* 303 */ "See Other", /* 304 */ "Not Modified", /* 305 */ "Use Proxy", /* 306 */ null, /* 307 */ "Temporary Redirect" }, new string[] { /* 400 */ "Bad Request", /* 401 */ "Unauthorized", /* 402 */ "Payment Required", /* 403 */ "Forbidden", /* 404 */ "Not Found", /* 405 */ "Method Not Allowed", /* 406 */ "Not Acceptable", /* 407 */ "Proxy Authentication Required", /* 408 */ "Request Timeout", /* 409 */ "Conflict", /* 410 */ "Gone", /* 411 */ "Length Required", /* 412 */ "Precondition Failed", /* 413 */ "Request Entity Too Large", /* 414 */ "Request-Uri Too Long", /* 415 */ "Unsupported Media Type", /* 416 */ "Requested Range Not Satisfiable", /* 417 */ "Expectation Failed", /* 418 */ null, /* 419 */ null, /* 420 */ null, /* 421 */ null, /* 422 */ "Unprocessable Entity", /* 423 */ "Locked", /* 424 */ "Failed Dependency" }, new string[] { /* 500 */ "Internal Server Error", /* 501 */ "Not Implemented", /* 502 */ "Bad Gateway", /* 503 */ "Service Unavailable", /* 504 */ "Gateway Timeout", /* 505 */ "Http Version Not Supported", /* 506 */ null, /* 507 */ "Insufficient Storage" } }; internal static string GetStatusDescription(int code) { if (code >= 100 && code < 600) { int i = code / 100; int j = code % 100; if (j < s_HTTPStatusDescriptions[i].Length) { return s_HTTPStatusDescriptions[i][j]; } } return null; } // unsafe class HttpListenerResponse : IDisposable { private Encoding m_ContentEncoding; private CookieCollection m_Cookies; private string m_StatusDescription; private bool m_KeepAlive; private ResponseState m_ResponseState; private WebHeaderCollection m_WebHeaders; private HttpResponseStream m_ResponseStream; private long m_ContentLength; private BoundaryType m_BoundaryType; private UnsafeNclNativeMethods.HttpApi.HTTP_RESPONSE m_NativeResponse; private HttpListenerContext m_HttpContext; internal HttpListenerResponse() { if(Logging.On)Logging.PrintInfo(Logging.HttpListener, this, ".ctor", ""); m_NativeResponse = new UnsafeNclNativeMethods.HttpApi.HTTP_RESPONSE(); m_WebHeaders = new WebHeaderCollection(WebHeaderCollectionType.HttpListenerResponse); m_BoundaryType = BoundaryType.None; m_NativeResponse.StatusCode = (ushort)HttpStatusCode.OK; m_NativeResponse.Version.MajorVersion = 1; m_NativeResponse.Version.MinorVersion = 1; m_KeepAlive = true; m_ResponseState = ResponseState.Created; } internal HttpListenerResponse(HttpListenerContext httpContext) : this() { if(Logging.On)Logging.Associate(Logging.HttpListener, this, httpContext); m_HttpContext = httpContext; } private HttpListenerContext HttpListenerContext { get { return m_HttpContext; } } private HttpListenerRequest HttpListenerRequest { get { return HttpListenerContext.Request; } } public /* override */ Encoding ContentEncoding { get { return m_ContentEncoding; } set { m_ContentEncoding = value; } } public /* override */ string ContentType { get { return Headers[HttpResponseHeader.ContentType]; } set { CheckDisposed(); if (string.IsNullOrEmpty(value)) { Headers.Remove(HttpResponseHeader.ContentType); } else { Headers.Set(HttpResponseHeader.ContentType, value); } } } public /* override */ Stream OutputStream { get { CheckDisposed(); EnsureResponseStream(); return m_ResponseStream; } } public /* override */ string RedirectLocation { get { return Headers[HttpResponseHeader.Location]; } set { // note that this doesn't set the status code to a redirect one CheckDisposed(); if (string.IsNullOrEmpty(value)) { Headers.Remove(HttpResponseHeader.Location); } else { Headers.Set(HttpResponseHeader.Location, value); } } } public /* override */ int StatusCode { get { return (int)m_NativeResponse.StatusCode; } set { CheckDisposed(); if (value<100 || value>999) { throw new ProtocolViolationException(SR.GetString(SR.net_invalidstatus)); } m_NativeResponse.StatusCode = (ushort)value; } } public /* override */ string StatusDescription { get { if (m_StatusDescription==null) { // if the user hasn't set this, generated on the fly, if possible. // We know this one is safe, no need to verify it as in the setter. m_StatusDescription = GetStatusDescription((int)StatusCode); } if (m_StatusDescription==null) { m_StatusDescription = string.Empty; } return m_StatusDescription; } set { CheckDisposed(); if (value==null) { throw new ArgumentNullException("value"); } // Need to verify the status description doesn't contain any control characters except HT. We mask off the high // byte since that's how it's encoded. for (int i = 0; i < value.Length; i++) { char c = (char) (0x000000ff & (uint) value[i]); if ((c <= 31 && c != (byte) '\t') || c == 127) { throw new ArgumentException(SR.GetString(SR.net_WebHeaderInvalidControlChars), "name"); } } m_StatusDescription = value; } } public CookieCollection Cookies { get { if (m_Cookies==null) { m_Cookies = new CookieCollection(false); } return m_Cookies; } set { m_Cookies = value; } } public void CopyFrom(HttpListenerResponse templateResponse) { if(Logging.On)Logging.PrintInfo(Logging.HttpListener, this, "CopyFrom", "templateResponse#"+ValidationHelper.HashString(templateResponse)); m_NativeResponse = new UnsafeNclNativeMethods.HttpApi.HTTP_RESPONSE(); m_ResponseState = ResponseState.Created; m_WebHeaders = templateResponse.m_WebHeaders; m_BoundaryType = templateResponse.m_BoundaryType; m_ContentLength = templateResponse.m_ContentLength; m_NativeResponse.StatusCode = templateResponse.m_NativeResponse.StatusCode; m_NativeResponse.Version.MajorVersion = templateResponse.m_NativeResponse.Version.MajorVersion; m_NativeResponse.Version.MinorVersion = templateResponse.m_NativeResponse.Version.MinorVersion; m_StatusDescription = templateResponse.m_StatusDescription; m_KeepAlive = templateResponse.m_KeepAlive; } public bool SendChunked{ get{ return (EntitySendFormat == EntitySendFormat.Chunked); } set{ if(value) { EntitySendFormat = EntitySendFormat.Chunked; } else{ EntitySendFormat = EntitySendFormat.ContentLength; } } } internal EntitySendFormat EntitySendFormat { get { return (EntitySendFormat)m_BoundaryType; } set { CheckDisposed(); if (m_ResponseState>=ResponseState.SentHeaders) { throw new InvalidOperationException(SR.GetString(SR.net_rspsubmitted)); } if (value==EntitySendFormat.Chunked && HttpListenerRequest.ProtocolVersion.Minor==0) { throw new ProtocolViolationException(SR.GetString(SR.net_nochunkuploadonhttp10)); } m_BoundaryType = (BoundaryType)value; if (value!=EntitySendFormat.ContentLength) { m_ContentLength = -1; } } } public /* override */ bool KeepAlive { get { return m_KeepAlive; } set { CheckDisposed(); m_KeepAlive = value; } } public WebHeaderCollection Headers { get { return m_WebHeaders; } set { m_WebHeaders.Clear(); foreach (string headerName in value.AllKeys) { m_WebHeaders.Add(headerName, value[headerName]); } } } public /* override */ void AddHeader(string name, string value) { if(Logging.On)Logging.PrintInfo(Logging.HttpListener, this, "AddHeader", " name="+name+" value="+value); Headers.SetInternal(name, value); } public /* override */ void AppendHeader(string name, string value) { if(Logging.On)Logging.PrintInfo(Logging.HttpListener, this, "AppendHeader", " name="+name+" value="+value); Headers.Add(name, value); } public /* override */ void Redirect(string url) { if(Logging.On)Logging.PrintInfo(Logging.HttpListener, this, "Redirect", " url="+url); Headers.SetInternal(HttpResponseHeader.Location, url); StatusCode = (int)HttpStatusCode.Redirect; StatusDescription = GetStatusDescription((int)StatusCode); } public void AppendCookie(Cookie cookie) { if (cookie==null) { throw new ArgumentNullException("cookie"); } if(Logging.On)Logging.PrintInfo(Logging.HttpListener, this, "AppendCookie", " cookie#"+ValidationHelper.HashString(cookie)); Cookies.Add(cookie); } public void SetCookie(Cookie cookie) { if (cookie==null) { throw new ArgumentNullException("cookie"); } int added = Cookies.InternalAdd(cookie, true); if(Logging.On)Logging.PrintInfo(Logging.HttpListener, this, "SetCookie", " cookie#"+ValidationHelper.HashString(cookie)); if (added!=1) { // cookie already existed and couldn't be replaced throw new ArgumentException(SR.GetString(SR.net_cookie_exists), "cookie"); } } public long ContentLength64 { get { return m_ContentLength; } set { CheckDisposed(); if (m_ResponseState>=ResponseState.SentHeaders) { throw new InvalidOperationException(SR.GetString(SR.net_rspsubmitted)); } if (value>=0) { m_ContentLength = value; m_BoundaryType = BoundaryType.ContentLength; } else { throw new ArgumentOutOfRangeException(SR.GetString(SR.net_clsmall)); } } } public Version ProtocolVersion { get { return new Version(m_NativeResponse.Version.MajorVersion, m_NativeResponse.Version.MinorVersion); } set { CheckDisposed(); if (value==null) { throw new ArgumentNullException("value"); } if (value.Major!=1 || (value.Minor!=0 && value.Minor!=1)) { throw new ArgumentException(SR.GetString(SR.net_wrongversion), "value"); } m_NativeResponse.Version.MajorVersion = (ushort)value.Major; m_NativeResponse.Version.MinorVersion = (ushort)value.Minor; } } public void Abort() { if(Logging.On)Logging.Enter(Logging.HttpListener, this, "abort", ""); try { if (m_ResponseState>=ResponseState.Closed) { return; } m_ResponseState = ResponseState.Closed; HttpListenerContext.Abort(); } finally { if(Logging.On)Logging.Exit(Logging.HttpListener, this, "abort", ""); } } public void Close(byte[] responseEntity, bool willBlock) { if(Logging.On)Logging.Enter(Logging.HttpListener, this, "Close", " responseEntity="+ValidationHelper.HashString(responseEntity)+" willBlock="+willBlock); try { CheckDisposed(); if (responseEntity==null) { throw new ArgumentNullException("responseEntity"); } GlobalLog.Print("HttpListenerResponse#" + ValidationHelper.HashString(this) + "::Close() ResponseState:" + m_ResponseState + " BoundaryType:" + m_BoundaryType + " ContentLength:" + m_ContentLength); if (m_ResponseState=ResponseState.Closed) { return; } EnsureResponseStream(); m_ResponseStream.Close(); m_ResponseState = ResponseState.Closed; HttpListenerContext.Close(); } void IDisposable.Dispose() { Dispose(true); GC.SuppressFinalize(this); } // old API, now private, and helper methods internal BoundaryType BoundaryType { get { return m_BoundaryType; } } internal bool SentHeaders { get { return m_ResponseState>=ResponseState.SentHeaders; } } internal bool ComputedHeaders { get { return m_ResponseState>=ResponseState.ComputedHeaders; } } private void EnsureResponseStream() { if (m_ResponseStream==null) { m_ResponseStream = new HttpResponseStream(HttpListenerContext); } } private void NonBlockingCloseCallback(IAsyncResult asyncResult) { try { m_ResponseStream.EndWrite(asyncResult); } catch (Win32Exception) { } finally { m_ResponseStream.Close(); HttpListenerContext.Close(); m_ResponseState = ResponseState.Closed; } } /* 12.3 HttpSendHttpResponse() and HttpSendResponseEntityBody() Flag Values. The following flags can be used on calls to HttpSendHttpResponse() and HttpSendResponseEntityBody() API calls: #define HTTP_SEND_RESPONSE_FLAG_DISCONNECT 0x00000001 #define HTTP_SEND_RESPONSE_FLAG_MORE_DATA 0x00000002 #define HTTP_SEND_RESPONSE_FLAG_RAW_HEADER 0x00000004 #define HTTP_SEND_RESPONSE_FLAG_VALID 0x00000007 HTTP_SEND_RESPONSE_FLAG_DISCONNECT: specifies that the network connection should be disconnected immediately after sending the response, overriding the HTTP protocol's persistent connection features. HTTP_SEND_RESPONSE_FLAG_MORE_DATA: specifies that additional entity body data will be sent by the caller. Thus, the last call HttpSendResponseEntityBody for a RequestId, will have this flag reset. HTTP_SEND_RESPONSE_RAW_HEADER: specifies that a caller of HttpSendResponseEntityBody() is intentionally omitting a call to HttpSendHttpResponse() in order to bypass normal header processing. The actual HTTP header will be generated by the application and sent as entity body. This flag should be passed on the first call to HttpSendResponseEntityBody, and not after. Thus, flag is not applicable to HttpSendHttpResponse. */ internal unsafe uint SendHeaders(UnsafeNclNativeMethods.HttpApi.HTTP_DATA_CHUNK* pDataChunk, HttpResponseStreamAsyncResult asyncResult, UnsafeNclNativeMethods.HttpApi.HTTP_FLAGS flags) { GlobalLog.Print("HttpListenerResponse#" + ValidationHelper.HashString(this) + "::SendHeaders() pDataChunk:" + ValidationHelper.ToString((IntPtr)pDataChunk) + " asyncResult:" + ValidationHelper.ToString(asyncResult)); GlobalLog.Assert(!SentHeaders, "HttpListenerResponse#{0}::SendHeaders()|SentHeaders is true.", ValidationHelper.HashString(this)); // Log headers if(Logging.On) { StringBuilder sb = new StringBuilder("HttpListenerResponse Headers:\n"); for (int i=0; i pinnedHeaders = SerializeHeaders(ref m_NativeResponse.Headers); try { if (pDataChunk!=null) { m_NativeResponse.EntityChunkCount = 1; m_NativeResponse.pEntityChunks = pDataChunk; } else if (asyncResult!=null && asyncResult.pDataChunks!=null) { m_NativeResponse.EntityChunkCount = asyncResult.dataChunkCount; m_NativeResponse.pEntityChunks = asyncResult.pDataChunks; } GlobalLog.Print("HttpListenerResponse#" + ValidationHelper.HashString(this) + "::SendHeaders() calling UnsafeNclNativeMethods.HttpApi.HttpSendHttpResponse flags:" + flags); if (StatusDescription.Length>0) { byte[] statusDescriptionBytes = new byte[WebHeaderCollection.HeaderEncoding.GetByteCount(StatusDescription)]; fixed (byte* pStatusDescription = statusDescriptionBytes) { m_NativeResponse.ReasonLength = (ushort)statusDescriptionBytes.Length; WebHeaderCollection.HeaderEncoding.GetBytes(StatusDescription, 0, statusDescriptionBytes.Length, statusDescriptionBytes, 0); m_NativeResponse.pReason = (sbyte*)pStatusDescription; fixed (UnsafeNclNativeMethods.HttpApi.HTTP_RESPONSE* pResponse = &m_NativeResponse) { if (asyncResult != null) { HttpListenerContext.EnsureBoundHandle(); } statusCode = UnsafeNclNativeMethods.HttpApi.HttpSendHttpResponse( HttpListenerContext.RequestQueueHandle, HttpListenerRequest.RequestId, (uint)flags, pResponse, null, null, SafeLocalFree.Zero, 0, asyncResult==null ? null : asyncResult.m_pOverlapped, null ); } } } else { fixed (UnsafeNclNativeMethods.HttpApi.HTTP_RESPONSE* pResponse = &m_NativeResponse) { if (asyncResult != null) { HttpListenerContext.EnsureBoundHandle(); } statusCode = UnsafeNclNativeMethods.HttpApi.HttpSendHttpResponse( HttpListenerContext.RequestQueueHandle, HttpListenerRequest.RequestId, (uint)flags, pResponse, null, null, SafeLocalFree.Zero, 0, asyncResult==null ? null : asyncResult.m_pOverlapped, null ); } } GlobalLog.Print("HttpListenerResponse#" + ValidationHelper.HashString(this) + "::SendHeaders() call to UnsafeNclNativeMethods.HttpApi.HttpSendHttpResponse returned:" + statusCode); } finally { FreePinnedHeaders(pinnedHeaders); } return statusCode; } internal void ComputeCookies() { GlobalLog.Print("HttpListenerResponse#" + ValidationHelper.HashString(this) + "::ComputeCookies() entering Set-Cookie: " + ValidationHelper.ToString(Headers[HttpResponseHeader.SetCookie]) +" Set-Cookie2: " + ValidationHelper.ToString(Headers[HttpKnownHeaderNames.SetCookie2])); if (m_Cookies!=null) { // now go through the collection, and concatenate all the cookies in per-variant strings string setCookie2 = null; string setCookie = null; for (int index=0; index 0) { Headers.SetInternal(HttpResponseHeader.WwwAuthenticate, HttpListenerContext.MutualAuthentication); } ComputeCookies(); GlobalLog.Print("HttpListenerResponse#" + ValidationHelper.HashString(this) + "::ComputeHeaders() flags:" + flags + " m_BoundaryType:" + m_BoundaryType + " m_ContentLength:" + m_ContentLength + " m_KeepAlive:" + m_KeepAlive); if (m_BoundaryType==BoundaryType.None) { m_ContentLength = -1; if (HttpListenerRequest.ProtocolVersion.Minor==0) { // m_KeepAlive = false; } else { m_BoundaryType = BoundaryType.Chunked; } } GlobalLog.Print("HttpListenerResponse#" + ValidationHelper.HashString(this) + "::ComputeHeaders() flags:" + flags + " m_BoundaryType:" + m_BoundaryType + " m_ContentLength:" + m_ContentLength + " m_KeepAlive:" + m_KeepAlive); if (m_BoundaryType==BoundaryType.ContentLength) { Headers.SetInternal(HttpResponseHeader.ContentLength, m_ContentLength.ToString("D", NumberFormatInfo.InvariantInfo)); if (m_ContentLength==0) { flags = UnsafeNclNativeMethods.HttpApi.HTTP_FLAGS.NONE; } } else if (m_BoundaryType==BoundaryType.Chunked) { Headers.SetInternal(HttpResponseHeader.TransferEncoding, HttpWebRequest.ChunkedHeader); } else if (m_BoundaryType==BoundaryType.None) { flags = UnsafeNclNativeMethods.HttpApi.HTTP_FLAGS.NONE; // seems like HTTP_SEND_RESPONSE_FLAG_MORE_DATA but this hangs the app; } else { m_KeepAlive = false; } if (!m_KeepAlive) { Headers.Add(HttpResponseHeader.Connection, "close"); if (flags==UnsafeNclNativeMethods.HttpApi.HTTP_FLAGS.NONE) { flags = UnsafeNclNativeMethods.HttpApi.HTTP_FLAGS.HTTP_SEND_RESPONSE_FLAG_DISCONNECT; } } else { if (HttpListenerRequest.ProtocolVersion.Minor == 0) { Headers.SetInternal(HttpResponseHeader.KeepAlive, "true"); } } GlobalLog.Print("HttpListenerResponse#" + ValidationHelper.HashString(this) + "::ComputeHeaders() flags:" + flags + " m_BoundaryType:" + m_BoundaryType + " m_ContentLength:" + m_ContentLength + " m_KeepAlive:" + m_KeepAlive); return flags; } private List SerializeHeaders(ref UnsafeNclNativeMethods.HttpApi.HTTP_RESPONSE_HEADERS headers) { UnsafeNclNativeMethods.HttpApi.HTTP_UNKNOWN_HEADER[] unknownHeaders = null; List pinnedHeaders; GCHandle gcHandle; /* // here we would check for BoundaryType.Raw, in this case we wouldn't need to do anything if (m_BoundaryType==BoundaryType.Raw) { return null; } */ GlobalLog.Print("HttpListenerResponse#" + ValidationHelper.HashString(this) + "::SerializeHeaders(HTTP_RESPONSE_HEADERS)"); if (Headers.Count==0) { return null; } string headerName; string headerValue; int lookup; byte[] bytes = null; pinnedHeaders = new List (); //--------------------------------------------------- // DTS Issue: 609383: // The Set-Cookie headers are being merged into one. // There are two issues here. // 1. When Set-Cookie headers are set through SetCookie method on the ListenerResponse, // there is code in the SetCookie method and the methods it calls to flatten the Set-Cookie // values. This blindly concatenates the cookies with a comma delimiter. There could be // a cookie value that contains comma, but we don't escape it with %XX value // // As an alternative users can add the Set-Cookie header through the AddHeader method // like ListenerResponse.Headers.Add("name", "value") // That way they can add multiple headers - AND They can format the value like they want it. // // 2. Now that the header collection contains multiple Set-Cookie name, value pairs // you would think the problem would go away. However here is an interesting thing. // For NameValueCollection, when you add // "Set-Cookie", "value1" // "Set-Cookie", "value2" // The NameValueCollection.Count == 1. Because there is only one key // NameValueCollection.Get("Set-Cookie") would conviniently take these two valuess // concatenate them with a comma like // value1,value2. // In order to get individual values, you need to use // string[] values = NameValueCollection.GetValues("Set-Cookie"); // // ------------------------------------------------------------- // So here is the proposed fix here. // We must first to loop through all the NameValueCollection keys // and if the name is a unknown header, we must compute the number of // values it has. Then, we should allocate that many unknown header array // elements. // // Note that a part of the fix here is to treat Set-Cookie as an unknown header // // //------------------------------------------------------------ int numUnknownHeaders = 0; for (int index=0; index pinnedHeaders) { if (pinnedHeaders!=null) { foreach (GCHandle gcHandle in pinnedHeaders) { if (gcHandle.IsAllocated) { gcHandle.Free(); } } } } private void CheckDisposed() { if (m_ResponseState>=ResponseState.Closed) { throw new ObjectDisposedException(this.GetType().FullName); } } } }
Link Menu
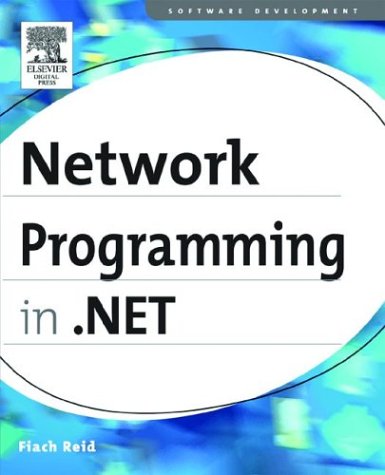
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ValidationManager.cs
- _DisconnectOverlappedAsyncResult.cs
- ScrollBarAutomationPeer.cs
- CompilerLocalReference.cs
- StrokeNodeOperations2.cs
- EDesignUtil.cs
- RC2CryptoServiceProvider.cs
- GeometryHitTestResult.cs
- WebPartCollection.cs
- HtmlTextBoxAdapter.cs
- Assert.cs
- FileSystemWatcher.cs
- WindowsStreamSecurityBindingElement.cs
- ClientConfigPaths.cs
- Gdiplus.cs
- QueryCacheEntry.cs
- BitmapMetadataBlob.cs
- ExceptionWrapper.cs
- ReflectionUtil.cs
- FunctionImportMapping.cs
- DataGridTableCollection.cs
- DiagnosticsConfigurationHandler.cs
- ApplicationSecurityManager.cs
- ProcessStartInfo.cs
- ViewLoader.cs
- XmlSchemaSimpleContent.cs
- RowToFieldTransformer.cs
- PersianCalendar.cs
- XmlNodeReader.cs
- Query.cs
- CompilerGeneratedAttribute.cs
- WindowPatternIdentifiers.cs
- ContentIterators.cs
- SafeNativeMethods.cs
- LocatorBase.cs
- RTTrackingProfile.cs
- CharacterMetrics.cs
- DurableInstanceManager.cs
- PointHitTestResult.cs
- OpCodes.cs
- NegotiationTokenAuthenticatorState.cs
- ManifestResourceInfo.cs
- BamlRecordWriter.cs
- PointConverter.cs
- SafeNativeMethods.cs
- ClientScriptManagerWrapper.cs
- TrackingLocationCollection.cs
- ApplicationHost.cs
- CodeDelegateInvokeExpression.cs
- WebExceptionStatus.cs
- EventProviderBase.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- Pair.cs
- WCFBuildProvider.cs
- DataGridViewSelectedRowCollection.cs
- SiteMapNodeItem.cs
- EntityModelSchemaGenerator.cs
- HtmlFormParameterWriter.cs
- CompressionTransform.cs
- RegexCharClass.cs
- EasingKeyFrames.cs
- AssociationSetEnd.cs
- ExceptionUtility.cs
- DisplayMemberTemplateSelector.cs
- DocumentGridContextMenu.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- TerminatorSinks.cs
- ProbeRequestResponseAsyncResult.cs
- SqlCharStream.cs
- ListViewTableCell.cs
- HtmlShim.cs
- PageStatePersister.cs
- Wildcard.cs
- DataControlImageButton.cs
- PkcsMisc.cs
- HtmlTableRowCollection.cs
- DbDeleteCommandTree.cs
- DesignerView.cs
- WebPartAddingEventArgs.cs
- SemanticBasicElement.cs
- ApplicationActivator.cs
- InitializerFacet.cs
- TrustSection.cs
- DesignerForm.cs
- AppDomainAttributes.cs
- DefaultParameterValueAttribute.cs
- CollectionBase.cs
- ArrangedElement.cs
- SelectedCellsCollection.cs
- FixedSOMFixedBlock.cs
- StringValidatorAttribute.cs
- UIElement.cs
- XPathConvert.cs
- RootBuilder.cs
- RestHandlerFactory.cs
- SqlCacheDependencyDatabase.cs
- ListViewSelectEventArgs.cs
- DateTimeHelper.cs
- Adorner.cs
- DbConnectionPoolIdentity.cs