Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Input / Cursors.cs / 1 / Cursors.cs
using System; using System.ComponentModel; using System.Text; namespace System.Windows.Input { ////// Cursors class to support stock cursors /// public static class Cursors { ////// A special value indicating that no cursor should be displayed. /// public static Cursor None { get { return EnsureCursor(CursorType.None); } } ////// Standard "no" cursor. /// public static Cursor No { get { return EnsureCursor(CursorType.No); } } ////// Standard "arrow" cursor. /// public static Cursor Arrow { get { return EnsureCursor(CursorType.Arrow); } } ////// Standard "arrow with hourglass" cursor. /// public static Cursor AppStarting { get { return EnsureCursor(CursorType.AppStarting); } } ////// Standard "crosshair arrow" cursor. /// public static Cursor Cross { get { return EnsureCursor(CursorType.Cross); } } ////// Standard "help" cursor. /// public static Cursor Help { get { return EnsureCursor(CursorType.Help); } } ////// Standard "text I-beam" cursor. /// public static Cursor IBeam { get { return EnsureCursor(CursorType.IBeam); } } ////// Standard "four-way arrow" cursor. /// public static Cursor SizeAll { get { return EnsureCursor(CursorType.SizeAll); } } ////// Standard "double arrow pointing NE and SW" cursor. /// public static Cursor SizeNESW { get { return EnsureCursor(CursorType.SizeNESW); } } ////// Standard "double arrow pointing N and S" cursor. /// public static Cursor SizeNS { get { return EnsureCursor(CursorType.SizeNS); } } ////// Standard "double arrow pointing NW and SE" cursor. /// public static Cursor SizeNWSE { get { return EnsureCursor(CursorType.SizeNWSE); } } ////// Standard "double arrow pointing W and E" cursor. /// public static Cursor SizeWE { get { return EnsureCursor(CursorType.SizeWE); } } ////// Standard "vertical up arrow" cursor. /// public static Cursor UpArrow { get { return EnsureCursor(CursorType.UpArrow); } } ////// Standard "hourglass" cursor. /// public static Cursor Wait { get { return EnsureCursor(CursorType.Wait); } } ////// Standard "hand" cursor. /// public static Cursor Hand { get { return EnsureCursor(CursorType.Hand); } } ////// Standard "pen" cursor. /// public static Cursor Pen { get { return EnsureCursor(CursorType.Pen); } } ////// Standard "scroll arrow pointing N and S" cursor. /// public static Cursor ScrollNS { get { return EnsureCursor(CursorType.ScrollNS); } } ////// Standard "scroll arrow pointing W and E" cursor. /// public static Cursor ScrollWE { get { return EnsureCursor(CursorType.ScrollWE); } } ////// Standard "scroll four-way arrow" cursor. /// public static Cursor ScrollAll { get { return EnsureCursor(CursorType.ScrollAll); } } ////// Standard "scroll arrow pointing N" cursor. /// public static Cursor ScrollN { get { return EnsureCursor(CursorType.ScrollN); } } ////// Standard "scroll arrow pointing S" cursor. /// public static Cursor ScrollS { get { return EnsureCursor(CursorType.ScrollS); } } ////// Standard "scroll arrow pointing W" cursor. /// public static Cursor ScrollW { get { return EnsureCursor(CursorType.ScrollW); } } ////// Standard "scroll arrow pointing E" cursor. /// public static Cursor ScrollE { get { return EnsureCursor(CursorType.ScrollE); } } ////// Standard "scroll arrow pointing N and W" cursor. /// public static Cursor ScrollNW { get { return EnsureCursor(CursorType.ScrollNW); } } ////// Standard "scroll arrow pointing N and E" cursor. /// public static Cursor ScrollNE { get { return EnsureCursor(CursorType.ScrollNE); } } ////// Standard "scroll arrow pointing S and W" cursor. /// public static Cursor ScrollSW { get { return EnsureCursor(CursorType.ScrollSW); } } ////// Standard "scrollSE" cursor. /// public static Cursor ScrollSE { get { return EnsureCursor(CursorType.ScrollSE); } } ////// Standard "arrow with CD" cursor. /// public static Cursor ArrowCD { get { return EnsureCursor(CursorType.ArrowCD); } } internal static Cursor EnsureCursor(CursorType cursorType) { if (_stockCursors[(int)cursorType] == null) { _stockCursors[(int)cursorType] = new Cursor(cursorType); } return _stockCursors[(int)cursorType]; } private static int _cursorTypeCount = ((int)CursorType.ArrowCD) + 1 ; private static Cursor[] _stockCursors = new Cursor[_cursorTypeCount]; //CursorType.ArrowCD = 27 } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.ComponentModel; using System.Text; namespace System.Windows.Input { ////// Cursors class to support stock cursors /// public static class Cursors { ////// A special value indicating that no cursor should be displayed. /// public static Cursor None { get { return EnsureCursor(CursorType.None); } } ////// Standard "no" cursor. /// public static Cursor No { get { return EnsureCursor(CursorType.No); } } ////// Standard "arrow" cursor. /// public static Cursor Arrow { get { return EnsureCursor(CursorType.Arrow); } } ////// Standard "arrow with hourglass" cursor. /// public static Cursor AppStarting { get { return EnsureCursor(CursorType.AppStarting); } } ////// Standard "crosshair arrow" cursor. /// public static Cursor Cross { get { return EnsureCursor(CursorType.Cross); } } ////// Standard "help" cursor. /// public static Cursor Help { get { return EnsureCursor(CursorType.Help); } } ////// Standard "text I-beam" cursor. /// public static Cursor IBeam { get { return EnsureCursor(CursorType.IBeam); } } ////// Standard "four-way arrow" cursor. /// public static Cursor SizeAll { get { return EnsureCursor(CursorType.SizeAll); } } ////// Standard "double arrow pointing NE and SW" cursor. /// public static Cursor SizeNESW { get { return EnsureCursor(CursorType.SizeNESW); } } ////// Standard "double arrow pointing N and S" cursor. /// public static Cursor SizeNS { get { return EnsureCursor(CursorType.SizeNS); } } ////// Standard "double arrow pointing NW and SE" cursor. /// public static Cursor SizeNWSE { get { return EnsureCursor(CursorType.SizeNWSE); } } ////// Standard "double arrow pointing W and E" cursor. /// public static Cursor SizeWE { get { return EnsureCursor(CursorType.SizeWE); } } ////// Standard "vertical up arrow" cursor. /// public static Cursor UpArrow { get { return EnsureCursor(CursorType.UpArrow); } } ////// Standard "hourglass" cursor. /// public static Cursor Wait { get { return EnsureCursor(CursorType.Wait); } } ////// Standard "hand" cursor. /// public static Cursor Hand { get { return EnsureCursor(CursorType.Hand); } } ////// Standard "pen" cursor. /// public static Cursor Pen { get { return EnsureCursor(CursorType.Pen); } } ////// Standard "scroll arrow pointing N and S" cursor. /// public static Cursor ScrollNS { get { return EnsureCursor(CursorType.ScrollNS); } } ////// Standard "scroll arrow pointing W and E" cursor. /// public static Cursor ScrollWE { get { return EnsureCursor(CursorType.ScrollWE); } } ////// Standard "scroll four-way arrow" cursor. /// public static Cursor ScrollAll { get { return EnsureCursor(CursorType.ScrollAll); } } ////// Standard "scroll arrow pointing N" cursor. /// public static Cursor ScrollN { get { return EnsureCursor(CursorType.ScrollN); } } ////// Standard "scroll arrow pointing S" cursor. /// public static Cursor ScrollS { get { return EnsureCursor(CursorType.ScrollS); } } ////// Standard "scroll arrow pointing W" cursor. /// public static Cursor ScrollW { get { return EnsureCursor(CursorType.ScrollW); } } ////// Standard "scroll arrow pointing E" cursor. /// public static Cursor ScrollE { get { return EnsureCursor(CursorType.ScrollE); } } ////// Standard "scroll arrow pointing N and W" cursor. /// public static Cursor ScrollNW { get { return EnsureCursor(CursorType.ScrollNW); } } ////// Standard "scroll arrow pointing N and E" cursor. /// public static Cursor ScrollNE { get { return EnsureCursor(CursorType.ScrollNE); } } ////// Standard "scroll arrow pointing S and W" cursor. /// public static Cursor ScrollSW { get { return EnsureCursor(CursorType.ScrollSW); } } ////// Standard "scrollSE" cursor. /// public static Cursor ScrollSE { get { return EnsureCursor(CursorType.ScrollSE); } } ////// Standard "arrow with CD" cursor. /// public static Cursor ArrowCD { get { return EnsureCursor(CursorType.ArrowCD); } } internal static Cursor EnsureCursor(CursorType cursorType) { if (_stockCursors[(int)cursorType] == null) { _stockCursors[(int)cursorType] = new Cursor(cursorType); } return _stockCursors[(int)cursorType]; } private static int _cursorTypeCount = ((int)CursorType.ArrowCD) + 1 ; private static Cursor[] _stockCursors = new Cursor[_cursorTypeCount]; //CursorType.ArrowCD = 27 } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
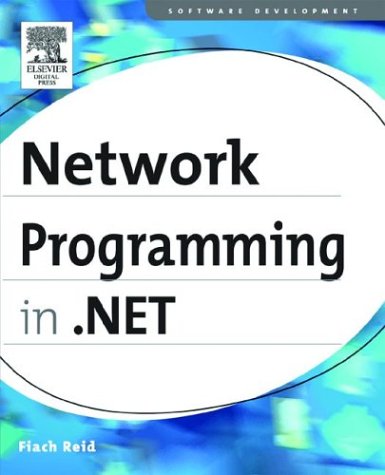
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DelegateTypeInfo.cs
- ContentIterators.cs
- TextFormatterImp.cs
- BindingWorker.cs
- SafeCoTaskMem.cs
- DesignerSerializationVisibilityAttribute.cs
- Compilation.cs
- ChooseAction.cs
- TerminatorSinks.cs
- ImageDrawing.cs
- Image.cs
- Vector3DAnimationUsingKeyFrames.cs
- AlphabetConverter.cs
- XmlWriter.cs
- XsdBuildProvider.cs
- MD5HashHelper.cs
- EncoderReplacementFallback.cs
- EventItfInfo.cs
- HelpInfo.cs
- MasterPageCodeDomTreeGenerator.cs
- RelationshipEndMember.cs
- ContainsSearchOperator.cs
- TimeSpanConverter.cs
- CharacterString.cs
- JournalEntryListConverter.cs
- RSAPKCS1SignatureFormatter.cs
- CuspData.cs
- ContentPresenter.cs
- ArrayElementGridEntry.cs
- fixedPageContentExtractor.cs
- LinqDataSourceSelectEventArgs.cs
- InheritanceContextChangedEventManager.cs
- RemotingService.cs
- CaseStatementSlot.cs
- ContextBase.cs
- FrameworkName.cs
- ResourceCategoryAttribute.cs
- SiteMapHierarchicalDataSourceView.cs
- CharAnimationBase.cs
- FixedSOMPageElement.cs
- SecurityState.cs
- WebEventTraceProvider.cs
- PreservationFileWriter.cs
- CompareValidator.cs
- TraceListeners.cs
- GridItemCollection.cs
- CqlIdentifiers.cs
- GenerateTemporaryTargetAssembly.cs
- MappingModelBuildProvider.cs
- TagPrefixAttribute.cs
- ColorMatrix.cs
- StrokeCollectionConverter.cs
- MatrixAnimationBase.cs
- WebPartDisplayModeCollection.cs
- CodeSpit.cs
- _ScatterGatherBuffers.cs
- RelationshipWrapper.cs
- CustomAttributeFormatException.cs
- RawStylusSystemGestureInputReport.cs
- FilterUserControlBase.cs
- GlyphsSerializer.cs
- ErrorRuntimeConfig.cs
- LongValidator.cs
- EventlogProvider.cs
- AsmxEndpointPickerExtension.cs
- CodeValidator.cs
- FrameworkContentElement.cs
- Label.cs
- ListViewDeletedEventArgs.cs
- SelectionWordBreaker.cs
- XmlSignatureManifest.cs
- BinaryFormatter.cs
- SqlParameterizer.cs
- Array.cs
- TemplateBindingExtensionConverter.cs
- XsdBuilder.cs
- XmlDataImplementation.cs
- BaseValidatorDesigner.cs
- RootDesignerSerializerAttribute.cs
- DiagnosticTrace.cs
- ClientTargetCollection.cs
- Types.cs
- OdbcError.cs
- ScriptResourceAttribute.cs
- Object.cs
- FormatConvertedBitmap.cs
- HttpResponseHeader.cs
- DbSetClause.cs
- HMACSHA256.cs
- QueryCoreOp.cs
- SimpleBitVector32.cs
- WindowsListViewGroupHelper.cs
- CubicEase.cs
- HttpHandlersInstallComponent.cs
- Resources.Designer.cs
- TimerElapsedEvenArgs.cs
- LinqDataSourceDeleteEventArgs.cs
- PathSegmentCollection.cs
- ImageInfo.cs
- CategoryGridEntry.cs