Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / MS / Internal / Data / BindingWorker.cs / 1 / BindingWorker.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Defines BindingWorker base class. // //--------------------------------------------------------------------------- using System; using System.Reflection; using System.ComponentModel; using System.Windows; using System.Windows.Controls; using System.Windows.Data; using System.Windows.Threading; namespace MS.Internal.Data { // Base class for binding workers. // Derived classes implement binding functionality depending on the // type of source, e.g. ClrBindingWorker, XmlBindingWorker internal abstract class BindingWorker { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- protected BindingWorker(BindingExpression b) { _bindingExpression = b; } //------------------------------------------------------ // // Internal properties - used by parent BindingExpression // //----------------------------------------------------- internal virtual Type SourcePropertyType { get { return null; } } internal virtual bool CanUpdate { get { return false; } } internal BindingExpression ParentBindingExpression { get { return _bindingExpression; } } internal Type TargetPropertyType { get { return TargetProperty.PropertyType; } } internal virtual bool IsDBNullValidForUpdate { get { return false; } } internal virtual object SourceItem { get { return null; } } internal virtual string SourcePropertyName { get { return null; } } //------------------------------------------------------ // // Internal methods - used by parent BindingExpression // //------------------------------------------------------ internal virtual void AttachDataItem() {} internal virtual void DetachDataItem() {} internal virtual void OnCurrentChanged(ICollectionView collectionView, EventArgs args) {} internal virtual object RawValue() { return null; } internal virtual void UpdateValue(object value) {} internal virtual void RefreshValue() {} internal virtual bool UsesDependencyProperty(DependencyObject d, DependencyProperty dp) { return false; } internal virtual void OnSourceInvalidation(DependencyObject d, DependencyProperty dp, bool isASubPropertyChange) {} internal virtual ValidationError ValidateDataError(BindingExpressionBase bindingExpressionBase) { return null; } //----------------------------------------------------- // // Protected Properties // //------------------------------------------------------ protected Binding ParentBinding { get { return ParentBindingExpression.ParentBinding; } } protected bool IsDynamic { get { return ParentBindingExpression.IsDynamic; } } internal bool IsReflective { get { return ParentBindingExpression.IsReflective; } } protected bool IgnoreSourcePropertyChange { get { return ParentBindingExpression.IgnoreSourcePropertyChange; } } protected object DataItem { get { return ParentBindingExpression.DataItem; } } protected DependencyObject TargetElement { get { return ParentBindingExpression.TargetElement; } } protected DependencyProperty TargetProperty { get { return ParentBindingExpression.TargetProperty; } } protected DataBindEngine Engine { get { return ParentBindingExpression.Engine; } } protected Dispatcher Dispatcher { get { return ParentBindingExpression.Dispatcher; } } protected BindingStatus Status { get { return ParentBindingExpression.Status; } set { ParentBindingExpression.SetStatus(value); } } //----------------------------------------------------- // // Protected Methods // //----------------------------------------------------- protected void SetTransferIsPending(bool value) { ParentBindingExpression.IsTransferPending = value; } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ BindingExpression _bindingExpression; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Defines BindingWorker base class. // //--------------------------------------------------------------------------- using System; using System.Reflection; using System.ComponentModel; using System.Windows; using System.Windows.Controls; using System.Windows.Data; using System.Windows.Threading; namespace MS.Internal.Data { // Base class for binding workers. // Derived classes implement binding functionality depending on the // type of source, e.g. ClrBindingWorker, XmlBindingWorker internal abstract class BindingWorker { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- protected BindingWorker(BindingExpression b) { _bindingExpression = b; } //------------------------------------------------------ // // Internal properties - used by parent BindingExpression // //----------------------------------------------------- internal virtual Type SourcePropertyType { get { return null; } } internal virtual bool CanUpdate { get { return false; } } internal BindingExpression ParentBindingExpression { get { return _bindingExpression; } } internal Type TargetPropertyType { get { return TargetProperty.PropertyType; } } internal virtual bool IsDBNullValidForUpdate { get { return false; } } internal virtual object SourceItem { get { return null; } } internal virtual string SourcePropertyName { get { return null; } } //------------------------------------------------------ // // Internal methods - used by parent BindingExpression // //------------------------------------------------------ internal virtual void AttachDataItem() {} internal virtual void DetachDataItem() {} internal virtual void OnCurrentChanged(ICollectionView collectionView, EventArgs args) {} internal virtual object RawValue() { return null; } internal virtual void UpdateValue(object value) {} internal virtual void RefreshValue() {} internal virtual bool UsesDependencyProperty(DependencyObject d, DependencyProperty dp) { return false; } internal virtual void OnSourceInvalidation(DependencyObject d, DependencyProperty dp, bool isASubPropertyChange) {} internal virtual ValidationError ValidateDataError(BindingExpressionBase bindingExpressionBase) { return null; } //----------------------------------------------------- // // Protected Properties // //------------------------------------------------------ protected Binding ParentBinding { get { return ParentBindingExpression.ParentBinding; } } protected bool IsDynamic { get { return ParentBindingExpression.IsDynamic; } } internal bool IsReflective { get { return ParentBindingExpression.IsReflective; } } protected bool IgnoreSourcePropertyChange { get { return ParentBindingExpression.IgnoreSourcePropertyChange; } } protected object DataItem { get { return ParentBindingExpression.DataItem; } } protected DependencyObject TargetElement { get { return ParentBindingExpression.TargetElement; } } protected DependencyProperty TargetProperty { get { return ParentBindingExpression.TargetProperty; } } protected DataBindEngine Engine { get { return ParentBindingExpression.Engine; } } protected Dispatcher Dispatcher { get { return ParentBindingExpression.Dispatcher; } } protected BindingStatus Status { get { return ParentBindingExpression.Status; } set { ParentBindingExpression.SetStatus(value); } } //----------------------------------------------------- // // Protected Methods // //----------------------------------------------------- protected void SetTransferIsPending(bool value) { ParentBindingExpression.IsTransferPending = value; } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ BindingExpression _bindingExpression; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
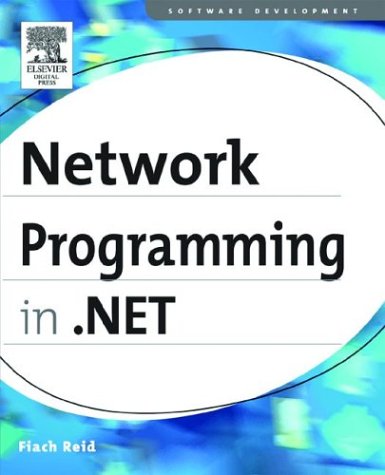
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GeneratedView.cs
- WizardStepBase.cs
- CompensatableSequenceActivity.cs
- SystemDropShadowChrome.cs
- Missing.cs
- ContractsBCL.cs
- CallId.cs
- PasswordRecoveryAutoFormat.cs
- Dictionary.cs
- TransformerInfoCollection.cs
- NameSpaceEvent.cs
- ResourceDescriptionAttribute.cs
- CompleteWizardStep.cs
- SubpageParagraph.cs
- DelegateInArgument.cs
- StateBag.cs
- Documentation.cs
- DoubleAnimationClockResource.cs
- WS2007HttpBindingCollectionElement.cs
- XPathNavigatorKeyComparer.cs
- DataKeyArray.cs
- FileDialogPermission.cs
- CallSiteOps.cs
- SelectedDatesCollection.cs
- ReturnType.cs
- SocketElement.cs
- ShadowGlyph.cs
- CheckBoxField.cs
- UdpRetransmissionSettings.cs
- RegexTree.cs
- ExtendedPropertyDescriptor.cs
- _SafeNetHandles.cs
- NamespaceTable.cs
- SerTrace.cs
- Constants.cs
- SafeHandle.cs
- WorkflowDesignerColors.cs
- UndoEngine.cs
- DataTableNameHandler.cs
- TransformFinalBlockRequest.cs
- ClassicBorderDecorator.cs
- MessageEnumerator.cs
- BaseUriHelper.cs
- FormParameter.cs
- UrlMappingsModule.cs
- Util.cs
- AuthenticodeSignatureInformation.cs
- CngUIPolicy.cs
- ResourceProviderFactory.cs
- XmlNamedNodeMap.cs
- DBParameter.cs
- ApplicationContext.cs
- dbdatarecord.cs
- WebServicesInteroperability.cs
- AppSettingsReader.cs
- FamilyCollection.cs
- BinaryUtilClasses.cs
- SmiEventStream.cs
- SelectionListDesigner.cs
- PropertyValueChangedEvent.cs
- DataGridRowAutomationPeer.cs
- TextureBrush.cs
- SqlPersistenceProviderFactory.cs
- sqlcontext.cs
- Vertex.cs
- CurrentChangingEventManager.cs
- _HeaderInfoTable.cs
- LazyTextWriterCreator.cs
- HtmlInputImage.cs
- MatrixStack.cs
- DbConnectionOptions.cs
- Application.cs
- MethodRental.cs
- FaultConverter.cs
- HttpHostedTransportConfiguration.cs
- CustomLineCap.cs
- AnimationClock.cs
- XhtmlTextWriter.cs
- XmlDataSource.cs
- DispatchWrapper.cs
- RecordConverter.cs
- XmlDocumentViewSchema.cs
- X509SecurityToken.cs
- DataFormat.cs
- SocketElement.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- AnimationClock.cs
- DataServiceBehavior.cs
- CrossSiteScriptingValidation.cs
- Comparer.cs
- OledbConnectionStringbuilder.cs
- CellIdBoolean.cs
- wgx_sdk_version.cs
- XmlSchemaSequence.cs
- LabelLiteral.cs
- XmlTextReaderImpl.cs
- CqlIdentifiers.cs
- CodeTypeDelegate.cs
- TextServicesManager.cs
- CompModSwitches.cs