Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CompMod / System / Configuration / AppSettingsReader.cs / 1 / AppSettingsReader.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Reflection; using System.Configuration; using System.Collections.Specialized; using System.Globalization; namespace System.Configuration { ////// The AppSettingsReader class provides a wrapper for System.Configuration.ConfigurationManager.AppSettings /// which provides a single method for reading values from the config file of a particular type. /// public class AppSettingsReader { private NameValueCollection map; static Type stringType = typeof(string); static Type[] paramsArray = new Type[] { stringType }; static string NullString = "None"; ////// Constructor /// public AppSettingsReader() { map = System.Configuration.ConfigurationManager.AppSettings; } ////// Gets the value for specified key from ConfigurationManager.AppSettings, and returns /// an object of the specified type containing the value from the config file. If the key /// isn't in the config file, or if it is not a valid value for the given type, it will /// throw an exception with a descriptive message so the user can make the appropriate /// change /// public object GetValue(string key, Type type) { if (key == null) throw new ArgumentNullException("key"); if (type == null) throw new ArgumentNullException("type"); string val = map[key]; if (val == null) throw new InvalidOperationException(SR.GetString(SR.AppSettingsReaderNoKey, key)); if (type == stringType) { // It's a string, so we can ALMOST just return the value. The only // tricky point is that if it's the string "(None)", then we want to // return null. And of course we need a way to represent the string // (None), so we use ((None)), and so on... so it's a little complicated. int NoneNesting = GetNoneNesting(val); if (NoneNesting == 0) { // val is not of the form ((..((None))..)) return val; } else if (NoneNesting == 1) { // val is (None) return null; } else { // val is of the form ((..((None))..)) return val.Substring(1, val.Length - 2); } } else { try { return Convert.ChangeType(val, type, CultureInfo.InvariantCulture); } catch (Exception) { string displayString = (val.Length == 0) ? SR.AppSettingsReaderEmptyString : val; throw new InvalidOperationException(SR.GetString(SR.AppSettingsReaderCantParse, displayString, key, type.ToString())); } } } private int GetNoneNesting(string val) { int count = 0; int len = val.Length; if (len > 1) { while (val[count] == '(' && val[len - count - 1] == ')') { count++; } if (count > 0 && string.Compare(NullString, 0, val, count, len - 2 * count, StringComparison.Ordinal) != 0) { // the stuff between the parens is not "None" count = 0; } } return count; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Reflection; using System.Configuration; using System.Collections.Specialized; using System.Globalization; namespace System.Configuration { ////// The AppSettingsReader class provides a wrapper for System.Configuration.ConfigurationManager.AppSettings /// which provides a single method for reading values from the config file of a particular type. /// public class AppSettingsReader { private NameValueCollection map; static Type stringType = typeof(string); static Type[] paramsArray = new Type[] { stringType }; static string NullString = "None"; ////// Constructor /// public AppSettingsReader() { map = System.Configuration.ConfigurationManager.AppSettings; } ////// Gets the value for specified key from ConfigurationManager.AppSettings, and returns /// an object of the specified type containing the value from the config file. If the key /// isn't in the config file, or if it is not a valid value for the given type, it will /// throw an exception with a descriptive message so the user can make the appropriate /// change /// public object GetValue(string key, Type type) { if (key == null) throw new ArgumentNullException("key"); if (type == null) throw new ArgumentNullException("type"); string val = map[key]; if (val == null) throw new InvalidOperationException(SR.GetString(SR.AppSettingsReaderNoKey, key)); if (type == stringType) { // It's a string, so we can ALMOST just return the value. The only // tricky point is that if it's the string "(None)", then we want to // return null. And of course we need a way to represent the string // (None), so we use ((None)), and so on... so it's a little complicated. int NoneNesting = GetNoneNesting(val); if (NoneNesting == 0) { // val is not of the form ((..((None))..)) return val; } else if (NoneNesting == 1) { // val is (None) return null; } else { // val is of the form ((..((None))..)) return val.Substring(1, val.Length - 2); } } else { try { return Convert.ChangeType(val, type, CultureInfo.InvariantCulture); } catch (Exception) { string displayString = (val.Length == 0) ? SR.AppSettingsReaderEmptyString : val; throw new InvalidOperationException(SR.GetString(SR.AppSettingsReaderCantParse, displayString, key, type.ToString())); } } } private int GetNoneNesting(string val) { int count = 0; int len = val.Length; if (len > 1) { while (val[count] == '(' && val[len - count - 1] == ')') { count++; } if (count > 0 && string.Compare(NullString, 0, val, count, len - 2 * count, StringComparison.Ordinal) != 0) { // the stuff between the parens is not "None" count = 0; } } return count; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
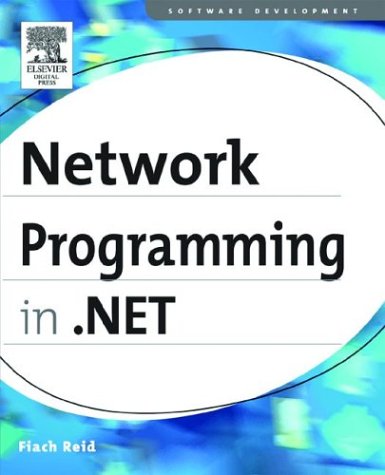
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextTreeFixupNode.cs
- ContentFileHelper.cs
- TypeName.cs
- DoubleKeyFrameCollection.cs
- DecimalAnimationBase.cs
- TextMetrics.cs
- DbSourceCommand.cs
- CorrelationService.cs
- PropertyManager.cs
- Metadata.cs
- SecurityHeaderTokenResolver.cs
- FormViewUpdateEventArgs.cs
- WebDescriptionAttribute.cs
- Win32SafeHandles.cs
- EditorZone.cs
- FocusManager.cs
- StructuredProperty.cs
- XmlTextWriter.cs
- WindowClosedEventArgs.cs
- Icon.cs
- NavigationWindowAutomationPeer.cs
- Int32AnimationBase.cs
- HttpWebRequest.cs
- URLBuilder.cs
- CompoundFileDeflateTransform.cs
- XmlExtensionFunction.cs
- XmlAttributeProperties.cs
- SafeNativeMethods.cs
- Soap.cs
- CatalogZone.cs
- sqlnorm.cs
- ApplicationProxyInternal.cs
- DispatcherExceptionEventArgs.cs
- MbpInfo.cs
- WebPartTransformer.cs
- ToolStripSystemRenderer.cs
- NumberSubstitution.cs
- BitmapDownload.cs
- TableSectionStyle.cs
- Grammar.cs
- MaterialCollection.cs
- ConstraintManager.cs
- RegisteredDisposeScript.cs
- FixedDocument.cs
- hresults.cs
- JsonByteArrayDataContract.cs
- TemplatePagerField.cs
- TreePrinter.cs
- LogStore.cs
- Int16KeyFrameCollection.cs
- WizardStepBase.cs
- SerialErrors.cs
- ConditionedDesigner.cs
- UnauthorizedAccessException.cs
- ScrollChrome.cs
- DataObject.cs
- SettingsBindableAttribute.cs
- HtmlInputButton.cs
- QilLiteral.cs
- ValidationResult.cs
- PersonalizableAttribute.cs
- DataReceivedEventArgs.cs
- PathNode.cs
- UnionExpr.cs
- LiteralTextParser.cs
- GenericTypeParameterBuilder.cs
- EventLogPermission.cs
- TextEditorThreadLocalStore.cs
- FormatException.cs
- Int16AnimationUsingKeyFrames.cs
- UnmanagedMemoryStream.cs
- BrowserCapabilitiesCodeGenerator.cs
- PrintPreviewDialog.cs
- WmpBitmapDecoder.cs
- CodePropertyReferenceExpression.cs
- DbSetClause.cs
- ButtonPopupAdapter.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- SafeProcessHandle.cs
- RegexMatch.cs
- DataGridViewColumnCollection.cs
- XD.cs
- EventDescriptor.cs
- ScaleTransform.cs
- Util.cs
- EventRoute.cs
- UnionExpr.cs
- EdmItemCollection.cs
- SocketException.cs
- HitTestParameters3D.cs
- XPathChildIterator.cs
- SqlProviderServices.cs
- NativeMethods.cs
- HttpValueCollection.cs
- DataGridViewCell.cs
- PolicyManager.cs
- CssClassPropertyAttribute.cs
- ThrowHelper.cs
- InvokeBinder.cs
- LoginName.cs