Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / textformatting / NumberSubstitution.cs / 1 / NumberSubstitution.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation // // File: NumberSubstituion.cs // // Contents: Number substitution related types // // Spec: http://team/sites/Avalon/Specs/Cultural%20digit%20substitution.htm // // Created: 1-7-2005 Niklas Borson (niklasb) // //----------------------------------------------------------------------- using System; using System.Globalization; using System.ComponentModel; using System.Windows; using MS.Internal.FontCache; // for HashFn using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; // Allow suppression of presharp warnings #pragma warning disable 1634, 1691 namespace System.Windows.Media { ////// The NumberSubstitution class specifies how numbers in text /// are to be displayed. /// public class NumberSubstitution { ////// Initializes a NumberSubstitution object with default values. /// public NumberSubstitution() { _source = NumberCultureSource.Text; _cultureOverride = null; _substitution = NumberSubstitutionMethod.AsCulture; } ////// Initializes a NumberSubstitution object with explicit values. /// /// Specifies how the number culture is determined. /// Number culture if NumberCultureSource.Override is specified. /// Type of number substitution to perform. public NumberSubstitution( NumberCultureSource source, CultureInfo cultureOverride, NumberSubstitutionMethod substitution) { _source = source; _cultureOverride = ThrowIfInvalidCultureOverride(cultureOverride); _substitution = substitution; } ////// The CultureSource property specifies how the culture for numbers /// is determined. The default value is NumberCultureSource.Text, /// which means the number culture is the culture of the text run. /// public NumberCultureSource CultureSource { get { return _source; } set { if ((uint)value > (uint)NumberCultureSource.Override) throw new InvalidEnumArgumentException("CultureSource", (int)value, typeof(NumberCultureSource)); _source = value; } } ////// If the CultureSource == NumberCultureSource.Override, this /// property specifies the number culture. A value of null is interpreted /// as US-English. The default value is null. If CultureSource != /// NumberCultureSource.Override, this property is ignored. /// [TypeConverter(typeof(System.Windows.CultureInfoIetfLanguageTagConverter))] public CultureInfo CultureOverride { get { return _cultureOverride; } set { _cultureOverride = ThrowIfInvalidCultureOverride(value); } } ////// Helper function to throw an exception if invalid value is specified for /// CultureOverride property. /// /// Culture to validate. ///The value of the culture parameter. private static CultureInfo ThrowIfInvalidCultureOverride(CultureInfo culture) { if (!IsValidCultureOverride(culture)) { throw new ArgumentException(SR.Get(SRID.SpecificNumberCultureRequired)); } return culture; } ////// Determines whether the specific culture is a valid value for the /// CultureOverride property. /// /// Culture to validate. ///Returns true if it's a valid CultureOverride, false if not. private static bool IsValidCultureOverride(CultureInfo culture) { // Null culture override is OK, but otherwise it must be a specific culture. return (culture == null) || !(culture.IsNeutralCulture || culture.Equals(CultureInfo.InvariantCulture)); } ////// Weakly typed validation callback for CultureOverride dependency property. /// /// CultureInfo object to validate; the type is assumed /// to be CultureInfo as the type is validated by the property engine. ///Returns true if it's a valid culture, false if not. private static bool IsValidCultureOverrideValue(object value) { return IsValidCultureOverride((CultureInfo)value); } ////// Specifies the type of number substitution to perform, if any. /// public NumberSubstitutionMethod Substitution { get { return _substitution; } set { if ((uint)value > (uint)NumberSubstitutionMethod.Traditional) throw new InvalidEnumArgumentException("Substitution", (int)value, typeof(NumberSubstitutionMethod)); _substitution = value; } } ////// DP For CultureSource /// public static readonly DependencyProperty CultureSourceProperty = DependencyProperty.RegisterAttached( "CultureSource", typeof(NumberCultureSource), typeof(NumberSubstitution)); ////// Setter for NumberSubstitution DependencyProperty /// public static void SetCultureSource(DependencyObject target, NumberCultureSource value) { if (target == null) { throw new ArgumentNullException("target"); } target.SetValue(CultureSourceProperty, value); } ////// Getter for NumberSubstitution DependencyProperty /// [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static NumberCultureSource GetCultureSource(DependencyObject target) { if (target == null) { throw new ArgumentNullException("target"); } return (NumberCultureSource)(target.GetValue(CultureSourceProperty)); } ////// DP For CultureOverride /// public static readonly DependencyProperty CultureOverrideProperty = DependencyProperty.RegisterAttached( "CultureOverride", typeof(CultureInfo), typeof(NumberSubstitution), null, // default property metadata new ValidateValueCallback(IsValidCultureOverrideValue) ); ////// Setter for NumberSubstitution DependencyProperty /// public static void SetCultureOverride(DependencyObject target, CultureInfo value) { if (target == null) { throw new ArgumentNullException("target"); } target.SetValue(CultureOverrideProperty, value); } ////// Getter for NumberSubstitution DependencyProperty /// [AttachedPropertyBrowsableForType(typeof(DependencyObject))] [TypeConverter(typeof(System.Windows.CultureInfoIetfLanguageTagConverter))] public static CultureInfo GetCultureOverride(DependencyObject target) { if (target == null) { throw new ArgumentNullException("target"); } return (CultureInfo)(target.GetValue(CultureOverrideProperty)); } ////// DP For Substitution /// public static readonly DependencyProperty SubstitutionProperty = DependencyProperty.RegisterAttached( "Substitution", typeof(NumberSubstitutionMethod), typeof(NumberSubstitution)); ////// Setter for NumberSubstitution DependencyProperty /// public static void SetSubstitution(DependencyObject target, NumberSubstitutionMethod value) { if (target == null) { throw new ArgumentNullException("target"); } target.SetValue(SubstitutionProperty, value); } ////// Getter for NumberSubstitution DependencyProperty /// [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static NumberSubstitutionMethod GetSubstitution(DependencyObject target) { if (target == null) { throw new ArgumentNullException("target"); } return (NumberSubstitutionMethod)(target.GetValue(SubstitutionProperty)); } ////// Computes hash code for this object. /// ///A 32-bit signed integer hash code. public override int GetHashCode() { int hash = HashFn.HashMultiply((int)_source) + (int)_substitution; if (_cultureOverride != null) hash = HashFn.HashMultiply(hash) + _cultureOverride.GetHashCode(); return HashFn.HashScramble(hash); } ////// Checks whether this object is equal to another NumberSubstitution object. /// /// Object to compare with. ///Returns true if the specified object is a NumberSubstitution object with the /// same properties as this object, and false otherwise. public override bool Equals(object obj) { NumberSubstitution sub = obj as NumberSubstitution; // Suppress PRESharp warning that sub can be null; apparently PRESharp // doesn't understand short circuit evaluation of operator &&. #pragma warning disable 6506 return sub != null && _source == sub._source && _substitution == sub._substitution && (_cultureOverride == null ? (sub._cultureOverride == null) : (_cultureOverride.Equals(sub._cultureOverride))); #pragma warning restore 6506 } private NumberCultureSource _source; private CultureInfo _cultureOverride; private NumberSubstitutionMethod _substitution; } ////// Used with the NumberSubstitution class, specifies how the culture for /// numbers in a text run is determined. /// public enum NumberCultureSource { ////// Number culture is TextRunProperties.CultureInfo, i.e., the culture /// of the text run. In markup, this is the xml:lang attribute. /// Text = 0, ////// Number culture is the culture of the current thread, which by default /// is the user default culture. /// User = 1, ////// Number culture is NumberSubstitution.CultureOverride. /// Override = 2 } ////// Value of the NumberSubstitution.Substitituion property, specifies /// the type of number substitution to perform, if any. /// public enum NumberSubstitutionMethod { ////// Specifies that the substitution method should be determined based /// on the number culture's NumberFormat.DigitSubstitution property. /// This is the default value. /// AsCulture = 0, ////// If the number culture is an Arabic or ---- culture, specifies that /// the digits depend on the context. Either traditional or Latin digits /// are used depending on the nearest preceding strong character or (if /// there is none) the text direction of the paragraph. /// Context = 1, ////// Specifies that code points 0x30-0x39 are always rendered as European /// digits, i.e., no substitution is performed. /// European = 2, ////// Specifies that numbers are rendered using the national digits for /// the number culture, as specified by the culture's NumberFormat /// property. /// NativeNational = 3, ////// Specifies that numbers are rendered using the traditional digits /// for the number culture. For most cultures, this is the same as /// NativeNational. However, NativeNational results in Latin digits /// for some Arabic cultures, whereas this value results in Arabic /// digits for all Arabic cultures. /// Traditional = 4 } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation // // File: NumberSubstituion.cs // // Contents: Number substitution related types // // Spec: http://team/sites/Avalon/Specs/Cultural%20digit%20substitution.htm // // Created: 1-7-2005 Niklas Borson (niklasb) // //----------------------------------------------------------------------- using System; using System.Globalization; using System.ComponentModel; using System.Windows; using MS.Internal.FontCache; // for HashFn using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; // Allow suppression of presharp warnings #pragma warning disable 1634, 1691 namespace System.Windows.Media { ////// The NumberSubstitution class specifies how numbers in text /// are to be displayed. /// public class NumberSubstitution { ////// Initializes a NumberSubstitution object with default values. /// public NumberSubstitution() { _source = NumberCultureSource.Text; _cultureOverride = null; _substitution = NumberSubstitutionMethod.AsCulture; } ////// Initializes a NumberSubstitution object with explicit values. /// /// Specifies how the number culture is determined. /// Number culture if NumberCultureSource.Override is specified. /// Type of number substitution to perform. public NumberSubstitution( NumberCultureSource source, CultureInfo cultureOverride, NumberSubstitutionMethod substitution) { _source = source; _cultureOverride = ThrowIfInvalidCultureOverride(cultureOverride); _substitution = substitution; } ////// The CultureSource property specifies how the culture for numbers /// is determined. The default value is NumberCultureSource.Text, /// which means the number culture is the culture of the text run. /// public NumberCultureSource CultureSource { get { return _source; } set { if ((uint)value > (uint)NumberCultureSource.Override) throw new InvalidEnumArgumentException("CultureSource", (int)value, typeof(NumberCultureSource)); _source = value; } } ////// If the CultureSource == NumberCultureSource.Override, this /// property specifies the number culture. A value of null is interpreted /// as US-English. The default value is null. If CultureSource != /// NumberCultureSource.Override, this property is ignored. /// [TypeConverter(typeof(System.Windows.CultureInfoIetfLanguageTagConverter))] public CultureInfo CultureOverride { get { return _cultureOverride; } set { _cultureOverride = ThrowIfInvalidCultureOverride(value); } } ////// Helper function to throw an exception if invalid value is specified for /// CultureOverride property. /// /// Culture to validate. ///The value of the culture parameter. private static CultureInfo ThrowIfInvalidCultureOverride(CultureInfo culture) { if (!IsValidCultureOverride(culture)) { throw new ArgumentException(SR.Get(SRID.SpecificNumberCultureRequired)); } return culture; } ////// Determines whether the specific culture is a valid value for the /// CultureOverride property. /// /// Culture to validate. ///Returns true if it's a valid CultureOverride, false if not. private static bool IsValidCultureOverride(CultureInfo culture) { // Null culture override is OK, but otherwise it must be a specific culture. return (culture == null) || !(culture.IsNeutralCulture || culture.Equals(CultureInfo.InvariantCulture)); } ////// Weakly typed validation callback for CultureOverride dependency property. /// /// CultureInfo object to validate; the type is assumed /// to be CultureInfo as the type is validated by the property engine. ///Returns true if it's a valid culture, false if not. private static bool IsValidCultureOverrideValue(object value) { return IsValidCultureOverride((CultureInfo)value); } ////// Specifies the type of number substitution to perform, if any. /// public NumberSubstitutionMethod Substitution { get { return _substitution; } set { if ((uint)value > (uint)NumberSubstitutionMethod.Traditional) throw new InvalidEnumArgumentException("Substitution", (int)value, typeof(NumberSubstitutionMethod)); _substitution = value; } } ////// DP For CultureSource /// public static readonly DependencyProperty CultureSourceProperty = DependencyProperty.RegisterAttached( "CultureSource", typeof(NumberCultureSource), typeof(NumberSubstitution)); ////// Setter for NumberSubstitution DependencyProperty /// public static void SetCultureSource(DependencyObject target, NumberCultureSource value) { if (target == null) { throw new ArgumentNullException("target"); } target.SetValue(CultureSourceProperty, value); } ////// Getter for NumberSubstitution DependencyProperty /// [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static NumberCultureSource GetCultureSource(DependencyObject target) { if (target == null) { throw new ArgumentNullException("target"); } return (NumberCultureSource)(target.GetValue(CultureSourceProperty)); } ////// DP For CultureOverride /// public static readonly DependencyProperty CultureOverrideProperty = DependencyProperty.RegisterAttached( "CultureOverride", typeof(CultureInfo), typeof(NumberSubstitution), null, // default property metadata new ValidateValueCallback(IsValidCultureOverrideValue) ); ////// Setter for NumberSubstitution DependencyProperty /// public static void SetCultureOverride(DependencyObject target, CultureInfo value) { if (target == null) { throw new ArgumentNullException("target"); } target.SetValue(CultureOverrideProperty, value); } ////// Getter for NumberSubstitution DependencyProperty /// [AttachedPropertyBrowsableForType(typeof(DependencyObject))] [TypeConverter(typeof(System.Windows.CultureInfoIetfLanguageTagConverter))] public static CultureInfo GetCultureOverride(DependencyObject target) { if (target == null) { throw new ArgumentNullException("target"); } return (CultureInfo)(target.GetValue(CultureOverrideProperty)); } ////// DP For Substitution /// public static readonly DependencyProperty SubstitutionProperty = DependencyProperty.RegisterAttached( "Substitution", typeof(NumberSubstitutionMethod), typeof(NumberSubstitution)); ////// Setter for NumberSubstitution DependencyProperty /// public static void SetSubstitution(DependencyObject target, NumberSubstitutionMethod value) { if (target == null) { throw new ArgumentNullException("target"); } target.SetValue(SubstitutionProperty, value); } ////// Getter for NumberSubstitution DependencyProperty /// [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static NumberSubstitutionMethod GetSubstitution(DependencyObject target) { if (target == null) { throw new ArgumentNullException("target"); } return (NumberSubstitutionMethod)(target.GetValue(SubstitutionProperty)); } ////// Computes hash code for this object. /// ///A 32-bit signed integer hash code. public override int GetHashCode() { int hash = HashFn.HashMultiply((int)_source) + (int)_substitution; if (_cultureOverride != null) hash = HashFn.HashMultiply(hash) + _cultureOverride.GetHashCode(); return HashFn.HashScramble(hash); } ////// Checks whether this object is equal to another NumberSubstitution object. /// /// Object to compare with. ///Returns true if the specified object is a NumberSubstitution object with the /// same properties as this object, and false otherwise. public override bool Equals(object obj) { NumberSubstitution sub = obj as NumberSubstitution; // Suppress PRESharp warning that sub can be null; apparently PRESharp // doesn't understand short circuit evaluation of operator &&. #pragma warning disable 6506 return sub != null && _source == sub._source && _substitution == sub._substitution && (_cultureOverride == null ? (sub._cultureOverride == null) : (_cultureOverride.Equals(sub._cultureOverride))); #pragma warning restore 6506 } private NumberCultureSource _source; private CultureInfo _cultureOverride; private NumberSubstitutionMethod _substitution; } ////// Used with the NumberSubstitution class, specifies how the culture for /// numbers in a text run is determined. /// public enum NumberCultureSource { ////// Number culture is TextRunProperties.CultureInfo, i.e., the culture /// of the text run. In markup, this is the xml:lang attribute. /// Text = 0, ////// Number culture is the culture of the current thread, which by default /// is the user default culture. /// User = 1, ////// Number culture is NumberSubstitution.CultureOverride. /// Override = 2 } ////// Value of the NumberSubstitution.Substitituion property, specifies /// the type of number substitution to perform, if any. /// public enum NumberSubstitutionMethod { ////// Specifies that the substitution method should be determined based /// on the number culture's NumberFormat.DigitSubstitution property. /// This is the default value. /// AsCulture = 0, ////// If the number culture is an Arabic or ---- culture, specifies that /// the digits depend on the context. Either traditional or Latin digits /// are used depending on the nearest preceding strong character or (if /// there is none) the text direction of the paragraph. /// Context = 1, ////// Specifies that code points 0x30-0x39 are always rendered as European /// digits, i.e., no substitution is performed. /// European = 2, ////// Specifies that numbers are rendered using the national digits for /// the number culture, as specified by the culture's NumberFormat /// property. /// NativeNational = 3, ////// Specifies that numbers are rendered using the traditional digits /// for the number culture. For most cultures, this is the same as /// NativeNational. However, NativeNational results in Latin digits /// for some Arabic cultures, whereas this value results in Arabic /// digits for all Arabic cultures. /// Traditional = 4 } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
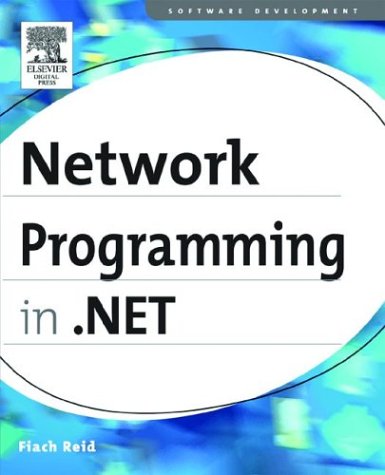
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InputManager.cs
- TdsParser.cs
- Error.cs
- OperationCanceledException.cs
- SQLInt64Storage.cs
- InvokeHandlers.cs
- CacheHelper.cs
- EventData.cs
- XmlValidatingReader.cs
- AssemblyInfo.cs
- TemplateContentLoader.cs
- XmlComplianceUtil.cs
- HttpConfigurationSystem.cs
- CultureInfo.cs
- CreateUserWizard.cs
- ModuleConfigurationInfo.cs
- BamlReader.cs
- CompiledRegexRunnerFactory.cs
- GridProviderWrapper.cs
- OciHandle.cs
- ListBoxItemAutomationPeer.cs
- DataSourceSelectArguments.cs
- StackOverflowException.cs
- ValidatingReaderNodeData.cs
- ExpandCollapseProviderWrapper.cs
- BinaryMethodMessage.cs
- DataControlFieldCell.cs
- DrawItemEvent.cs
- XmlHelper.cs
- ViewBase.cs
- TextServicesCompartmentContext.cs
- FormViewInsertedEventArgs.cs
- Matrix3DValueSerializer.cs
- XamlWriterExtensions.cs
- storepermission.cs
- XamlWrapperReaders.cs
- XDRSchema.cs
- _KerberosClient.cs
- DbTransaction.cs
- TableProviderWrapper.cs
- ByteStreamGeometryContext.cs
- ObjectDataSourceEventArgs.cs
- EntityException.cs
- StateChangeEvent.cs
- PtsHost.cs
- AssemblyInfo.cs
- XsltContext.cs
- _ScatterGatherBuffers.cs
- RuntimeEnvironment.cs
- PlanCompiler.cs
- _NegotiateClient.cs
- ErrorWebPart.cs
- WindowsPen.cs
- TransformGroup.cs
- ToolboxBitmapAttribute.cs
- TypeContext.cs
- CodeDirectionExpression.cs
- TableLayoutPanelCellPosition.cs
- CompilerWrapper.cs
- ReverseComparer.cs
- RsaKeyIdentifierClause.cs
- ConfigurationLockCollection.cs
- TimeSpan.cs
- XmlProcessingInstruction.cs
- TypefaceMetricsCache.cs
- MSAANativeProvider.cs
- PrtTicket_Public.cs
- Soap12ProtocolReflector.cs
- httpserverutility.cs
- CommonDialog.cs
- LogicalChannelCollection.cs
- IndexerNameAttribute.cs
- XD.cs
- WebPageTraceListener.cs
- ConfigXmlSignificantWhitespace.cs
- HashRepartitionEnumerator.cs
- RemotingConfigParser.cs
- TextHidden.cs
- CrossAppDomainChannel.cs
- TemplateNameScope.cs
- TextEditorTyping.cs
- ButtonBase.cs
- GroupBoxAutomationPeer.cs
- ProfileSection.cs
- CompatibleComparer.cs
- LiteralControl.cs
- SafeEventLogWriteHandle.cs
- GridViewRow.cs
- MetadataFile.cs
- DependencyObjectValidator.cs
- GetIndexBinder.cs
- PropertyEmitter.cs
- DateTimeOffsetConverter.cs
- WebHttpSecurity.cs
- AutoCompleteStringCollection.cs
- WebPartTransformerCollection.cs
- FixedStringLookup.cs
- SqlXml.cs
- TabOrder.cs
- GifBitmapEncoder.cs