Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / MS / Internal / Automation / TableProviderWrapper.cs / 1 / TableProviderWrapper.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Table pattern provider wrapper for WCP // // History: // 07/21/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Threading; using System.Windows.Media; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Windows.Automation.Peers; namespace MS.Internal.Automation { // Automation/WCP Wrapper class: Implements that UIAutomation I...Provider // interface, and calls through to a WCP AutomationPeer which implements the corresponding // I...Provider inteface. Marshalls the call from the RPC thread onto the // target AutomationPeer's context. // // Class has two major parts to it: // * Implementation of the I...Provider, which uses Dispatcher.Invoke // to call a private method (lives in second half of the class) via a delegate, // if necessary, packages any params into an object param. Return type of Invoke // must be cast from object to appropriate type. // * private methods - one for each interface entry point - which get called back // on the right context. These call through to the peer that's actually // implenting the I...Provider version of the interface. internal class TableProviderWrapper: MarshalByRefObject, ITableProvider { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private TableProviderWrapper( AutomationPeer peer, ITableProvider iface ) { _peer = peer; _iface = iface; } #endregion Constructors //------------------------------------------------------ // // Interface ITableProvider // //----------------------------------------------------- #region Interface ITableProvider public IRawElementProviderSimple GetItem(int row, int column) { return (IRawElementProviderSimple) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetItem ), new int [ ] { row, column } ); } public int RowCount { get { return (int) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetRowCount ), null ); } } public int ColumnCount { get { return (int) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetColumnCount ), null ); } } public IRawElementProviderSimple [] GetRowHeaders() { return (IRawElementProviderSimple []) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetRowHeaders ), null ); } public IRawElementProviderSimple [] GetColumnHeaders() { return (IRawElementProviderSimple []) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetColumnHeaders ), null ); } public RowOrColumnMajor RowOrColumnMajor { get { return (RowOrColumnMajor) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetRowOrColumnMajor ), null ); } } #endregion Interface ITableProvider //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods internal static object Wrap( AutomationPeer peer, object iface ) { return new TableProviderWrapper( peer, (ITableProvider) iface ); } #endregion Internal Methods //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods private object GetItem( object arg ) { int [ ] coords = (int [ ]) arg; return _iface.GetItem( coords[ 0 ], coords[ 1 ] ); } private object GetRowCount( object unused ) { return _iface.RowCount; } private object GetColumnCount( object unused ) { return _iface.ColumnCount; } private object GetRowHeaders( object unused ) { return _iface.GetRowHeaders(); } private object GetColumnHeaders( object unused ) { return _iface.GetColumnHeaders(); } private object GetRowOrColumnMajor( object unused ) { return _iface.RowOrColumnMajor; } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationPeer _peer; private ITableProvider _iface; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Table pattern provider wrapper for WCP // // History: // 07/21/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Threading; using System.Windows.Media; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Windows.Automation.Peers; namespace MS.Internal.Automation { // Automation/WCP Wrapper class: Implements that UIAutomation I...Provider // interface, and calls through to a WCP AutomationPeer which implements the corresponding // I...Provider inteface. Marshalls the call from the RPC thread onto the // target AutomationPeer's context. // // Class has two major parts to it: // * Implementation of the I...Provider, which uses Dispatcher.Invoke // to call a private method (lives in second half of the class) via a delegate, // if necessary, packages any params into an object param. Return type of Invoke // must be cast from object to appropriate type. // * private methods - one for each interface entry point - which get called back // on the right context. These call through to the peer that's actually // implenting the I...Provider version of the interface. internal class TableProviderWrapper: MarshalByRefObject, ITableProvider { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private TableProviderWrapper( AutomationPeer peer, ITableProvider iface ) { _peer = peer; _iface = iface; } #endregion Constructors //------------------------------------------------------ // // Interface ITableProvider // //----------------------------------------------------- #region Interface ITableProvider public IRawElementProviderSimple GetItem(int row, int column) { return (IRawElementProviderSimple) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetItem ), new int [ ] { row, column } ); } public int RowCount { get { return (int) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetRowCount ), null ); } } public int ColumnCount { get { return (int) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetColumnCount ), null ); } } public IRawElementProviderSimple [] GetRowHeaders() { return (IRawElementProviderSimple []) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetRowHeaders ), null ); } public IRawElementProviderSimple [] GetColumnHeaders() { return (IRawElementProviderSimple []) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetColumnHeaders ), null ); } public RowOrColumnMajor RowOrColumnMajor { get { return (RowOrColumnMajor) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetRowOrColumnMajor ), null ); } } #endregion Interface ITableProvider //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods internal static object Wrap( AutomationPeer peer, object iface ) { return new TableProviderWrapper( peer, (ITableProvider) iface ); } #endregion Internal Methods //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods private object GetItem( object arg ) { int [ ] coords = (int [ ]) arg; return _iface.GetItem( coords[ 0 ], coords[ 1 ] ); } private object GetRowCount( object unused ) { return _iface.RowCount; } private object GetColumnCount( object unused ) { return _iface.ColumnCount; } private object GetRowHeaders( object unused ) { return _iface.GetRowHeaders(); } private object GetColumnHeaders( object unused ) { return _iface.GetColumnHeaders(); } private object GetRowOrColumnMajor( object unused ) { return _iface.RowOrColumnMajor; } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationPeer _peer; private ITableProvider _iface; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
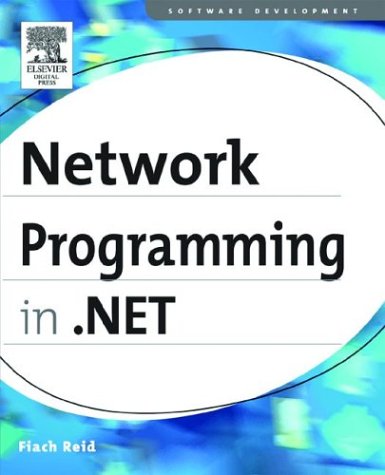
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- JpegBitmapDecoder.cs
- EntityDescriptor.cs
- ArrayElementGridEntry.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- EntitySqlQueryCacheEntry.cs
- IPipelineRuntime.cs
- DataControlFieldCollection.cs
- SafeHandle.cs
- UnsafeNativeMethods.cs
- WebPartManager.cs
- translator.cs
- ResXDataNode.cs
- FileFormatException.cs
- FontStyles.cs
- DesigntimeLicenseContextSerializer.cs
- ReferencedAssembly.cs
- WebPartsPersonalization.cs
- ClientType.cs
- AuthorizationContext.cs
- EditorBrowsableAttribute.cs
- WebConfigurationFileMap.cs
- GeneralTransform3D.cs
- TypeReference.cs
- WebExceptionStatus.cs
- EncoderParameters.cs
- SecurityTokenSerializer.cs
- Deflater.cs
- TraceSection.cs
- HitTestFilterBehavior.cs
- MapPathBasedVirtualPathProvider.cs
- DataGridRow.cs
- DragDrop.cs
- CheckBoxFlatAdapter.cs
- TextEditorTables.cs
- AsymmetricAlgorithm.cs
- PageVisual.cs
- TreeViewItemAutomationPeer.cs
- MemberAccessException.cs
- ArrayElementGridEntry.cs
- CodeTypeReferenceCollection.cs
- EntityClassGenerator.cs
- SslStream.cs
- OrderedDictionary.cs
- CapabilitiesSection.cs
- MinimizableAttributeTypeConverter.cs
- NestedContainer.cs
- InputScopeNameConverter.cs
- DataGridView.cs
- DBDataPermissionAttribute.cs
- AggregationMinMaxHelpers.cs
- GradientBrush.cs
- ConfigurationSettings.cs
- QueryAccessibilityHelpEvent.cs
- UserPreferenceChangedEventArgs.cs
- JournalEntryStack.cs
- DataGridViewColumnHeaderCell.cs
- DecoderNLS.cs
- PolicyUnit.cs
- XmlDictionaryReaderQuotas.cs
- NetworkInformationException.cs
- WorkflowApplicationTerminatedException.cs
- PriorityRange.cs
- SelectorItemAutomationPeer.cs
- ErrorTolerantObjectWriter.cs
- ClientSideQueueItem.cs
- InfoCardRSAOAEPKeyExchangeFormatter.cs
- LabelEditEvent.cs
- CatalogPartDesigner.cs
- EventHandlingScope.cs
- RepeaterItemEventArgs.cs
- ObjectViewFactory.cs
- MissingMethodException.cs
- SymbolTable.cs
- Property.cs
- Triangle.cs
- OperationContractGenerationContext.cs
- MatcherBuilder.cs
- EventBuilder.cs
- CRYPTPROTECT_PROMPTSTRUCT.cs
- URLMembershipCondition.cs
- DynamicMethod.cs
- ValueType.cs
- HebrewCalendar.cs
- ScrollData.cs
- PageParserFilter.cs
- ToggleProviderWrapper.cs
- CompilationPass2Task.cs
- RectConverter.cs
- SoapIgnoreAttribute.cs
- RolePrincipal.cs
- Tuple.cs
- TextFormatterContext.cs
- MenuItemCollection.cs
- TextBounds.cs
- BrowserDefinitionCollection.cs
- MethodBody.cs
- ApplicationContext.cs
- WebMessageEncoderFactory.cs
- HostingEnvironmentException.cs
- HttpWebResponse.cs