Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Xml / System / Xml / Dom / XmlDocumentFragment.cs / 1 / XmlDocumentFragment.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- //DocumentFragment
is a "lightweight" or "minimal" //Document
object. It is very common to want to be able to // extract a portion of a document's tree or to create a new fragment of a // document. Imagine implementing a user command like cut or rearranging a // document by moving fragments around. It is desirable to have an object // which can hold such fragments and it is quite natural to use a Node for // this purpose. While it is true that aDocument
object could // fulfil this role, aDocument
object can potentially be a // heavyweight object, depending on the underlying implementation. What is // really needed for this is a very lightweight object. //DocumentFragment
is such an object. //Furthermore, various operations -- such as inserting nodes as children // of another
Node
-- may takeDocumentFragment
// objects as arguments; this results in all the child nodes of the //DocumentFragment
being moved to the child list of this node. //The children of a
DocumentFragment
node are zero or more // nodes representing the tops of any sub-trees defining the structure of the // document.DocumentFragment
nodes do not need to be // well-formed XML documents (although they do need to follow the rules // imposed upon well-formed XML parsed entities, which can have multiple top // nodes). For example, aDocumentFragment
might have only one // child and that child node could be aText
node. Such a // structure model represents neither an HTML document nor a well-formed XML // document. //When a
DocumentFragment
is inserted into a //Document
(or indeed any otherNode
that may take // children) the children of theDocumentFragment
and not the //DocumentFragment
itself are inserted into the //Node
. This makes theDocumentFragment
very // useful when the user wishes to create nodes that are siblings; the //DocumentFragment
acts as the parent of these nodes so that the // user can use the standard methods from theNode
interface, // such asinsertBefore()
andappendChild()
. namespace System.Xml { using System.Diagnostics; using System.Xml.XPath; // Represents a lightweight object that is useful for tree insert // operations. public class XmlDocumentFragment : XmlNode { XmlLinkedNode lastChild; protected internal XmlDocumentFragment( XmlDocument ownerDocument ): base( ) { if ( ownerDocument == null ) throw new ArgumentException(Res.GetString(Res.Xdom_Node_Null_Doc)); parentNode= ownerDocument; } // Gets the name of the node. public override String Name { get { return OwnerDocument.strDocumentFragmentName;} } // Gets the name of the current node without the namespace prefix. public override String LocalName { get { return OwnerDocument.strDocumentFragmentName;} } // Gets the type of the current node. public override XmlNodeType NodeType { get { return XmlNodeType.DocumentFragment;} } // Gets the parent of this node (for nodes that can have parents). public override XmlNode ParentNode { get { return null;} } // Gets the XmlDocument that contains this node. public override XmlDocument OwnerDocument { get { return (XmlDocument)parentNode; } } // Gets or sets the markup representing just // the children of this node. public override string InnerXml { get { return base.InnerXml; } set { RemoveAll(); XmlLoader loader = new XmlLoader(); //Hack that the content is the same element loader.ParsePartialContent( this, value, XmlNodeType.Element ); } } // Creates a duplicate of this node. public override XmlNode CloneNode(bool deep) { Debug.Assert( OwnerDocument != null ); XmlDocument doc = OwnerDocument; XmlDocumentFragment clone = doc.CreateDocumentFragment(); if (deep) clone.CopyChildren( doc, this, deep ); return clone; } internal override bool IsContainer { get { return true;} } internal override XmlLinkedNode LastNode { get { return lastChild;} set { lastChild = value;} } internal override bool IsValidChildType( XmlNodeType type ) { switch (type) { case XmlNodeType.Element: case XmlNodeType.Text: case XmlNodeType.EntityReference: case XmlNodeType.Comment: case XmlNodeType.Whitespace: case XmlNodeType.SignificantWhitespace: case XmlNodeType.ProcessingInstruction: case XmlNodeType.CDATA: return true; case XmlNodeType.XmlDeclaration: //if there is an XmlDeclaration node, it has to be the first node; XmlNode firstNode = FirstChild; if (firstNode == null || firstNode.NodeType != XmlNodeType.XmlDeclaration) return true; else return false; //not allowed to insert a second XmlDeclaration node default: return false; } } internal override bool CanInsertAfter( XmlNode newChild, XmlNode refChild ) { Debug.Assert(newChild != null); //should be checked that newChild is not null before this function call if (newChild.NodeType == XmlNodeType.XmlDeclaration) { if (refChild == null) { //append at the end return (LastNode == null); } else return false; } return true; } internal override bool CanInsertBefore( XmlNode newChild, XmlNode refChild ) { Debug.Assert(newChild != null); //should be checked that newChild is not null before this function call if (newChild.NodeType == XmlNodeType.XmlDeclaration) { return (refChild==null || refChild==FirstChild); } return true; } // Saves the node to the specified XmlWriter. public override void WriteTo(XmlWriter w) { WriteContentTo( w ); } // Saves all the children of the node to the specified XmlWriter. public override void WriteContentTo(XmlWriter w) { foreach( XmlNode n in this ) { n.WriteTo( w ); } } internal override XPathNodeType XPNodeType { get { return XPathNodeType.Root; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- //DocumentFragment
is a "lightweight" or "minimal" //Document
object. It is very common to want to be able to // extract a portion of a document's tree or to create a new fragment of a // document. Imagine implementing a user command like cut or rearranging a // document by moving fragments around. It is desirable to have an object // which can hold such fragments and it is quite natural to use a Node for // this purpose. While it is true that aDocument
object could // fulfil this role, aDocument
object can potentially be a // heavyweight object, depending on the underlying implementation. What is // really needed for this is a very lightweight object. //DocumentFragment
is such an object. //Furthermore, various operations -- such as inserting nodes as children // of another
Node
-- may takeDocumentFragment
// objects as arguments; this results in all the child nodes of the //DocumentFragment
being moved to the child list of this node. //The children of a
DocumentFragment
node are zero or more // nodes representing the tops of any sub-trees defining the structure of the // document.DocumentFragment
nodes do not need to be // well-formed XML documents (although they do need to follow the rules // imposed upon well-formed XML parsed entities, which can have multiple top // nodes). For example, aDocumentFragment
might have only one // child and that child node could be aText
node. Such a // structure model represents neither an HTML document nor a well-formed XML // document. //When a
DocumentFragment
is inserted into a //Document
(or indeed any otherNode
that may take // children) the children of theDocumentFragment
and not the //DocumentFragment
itself are inserted into the //Node
. This makes theDocumentFragment
very // useful when the user wishes to create nodes that are siblings; the //DocumentFragment
acts as the parent of these nodes so that the // user can use the standard methods from theNode
interface, // such asinsertBefore()
andappendChild()
. namespace System.Xml { using System.Diagnostics; using System.Xml.XPath; // Represents a lightweight object that is useful for tree insert // operations. public class XmlDocumentFragment : XmlNode { XmlLinkedNode lastChild; protected internal XmlDocumentFragment( XmlDocument ownerDocument ): base( ) { if ( ownerDocument == null ) throw new ArgumentException(Res.GetString(Res.Xdom_Node_Null_Doc)); parentNode= ownerDocument; } // Gets the name of the node. public override String Name { get { return OwnerDocument.strDocumentFragmentName;} } // Gets the name of the current node without the namespace prefix. public override String LocalName { get { return OwnerDocument.strDocumentFragmentName;} } // Gets the type of the current node. public override XmlNodeType NodeType { get { return XmlNodeType.DocumentFragment;} } // Gets the parent of this node (for nodes that can have parents). public override XmlNode ParentNode { get { return null;} } // Gets the XmlDocument that contains this node. public override XmlDocument OwnerDocument { get { return (XmlDocument)parentNode; } } // Gets or sets the markup representing just // the children of this node. public override string InnerXml { get { return base.InnerXml; } set { RemoveAll(); XmlLoader loader = new XmlLoader(); //Hack that the content is the same element loader.ParsePartialContent( this, value, XmlNodeType.Element ); } } // Creates a duplicate of this node. public override XmlNode CloneNode(bool deep) { Debug.Assert( OwnerDocument != null ); XmlDocument doc = OwnerDocument; XmlDocumentFragment clone = doc.CreateDocumentFragment(); if (deep) clone.CopyChildren( doc, this, deep ); return clone; } internal override bool IsContainer { get { return true;} } internal override XmlLinkedNode LastNode { get { return lastChild;} set { lastChild = value;} } internal override bool IsValidChildType( XmlNodeType type ) { switch (type) { case XmlNodeType.Element: case XmlNodeType.Text: case XmlNodeType.EntityReference: case XmlNodeType.Comment: case XmlNodeType.Whitespace: case XmlNodeType.SignificantWhitespace: case XmlNodeType.ProcessingInstruction: case XmlNodeType.CDATA: return true; case XmlNodeType.XmlDeclaration: //if there is an XmlDeclaration node, it has to be the first node; XmlNode firstNode = FirstChild; if (firstNode == null || firstNode.NodeType != XmlNodeType.XmlDeclaration) return true; else return false; //not allowed to insert a second XmlDeclaration node default: return false; } } internal override bool CanInsertAfter( XmlNode newChild, XmlNode refChild ) { Debug.Assert(newChild != null); //should be checked that newChild is not null before this function call if (newChild.NodeType == XmlNodeType.XmlDeclaration) { if (refChild == null) { //append at the end return (LastNode == null); } else return false; } return true; } internal override bool CanInsertBefore( XmlNode newChild, XmlNode refChild ) { Debug.Assert(newChild != null); //should be checked that newChild is not null before this function call if (newChild.NodeType == XmlNodeType.XmlDeclaration) { return (refChild==null || refChild==FirstChild); } return true; } // Saves the node to the specified XmlWriter. public override void WriteTo(XmlWriter w) { WriteContentTo( w ); } // Saves all the children of the node to the specified XmlWriter. public override void WriteContentTo(XmlWriter w) { foreach( XmlNode n in this ) { n.WriteTo( w ); } } internal override XPathNodeType XPNodeType { get { return XPathNodeType.Root; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
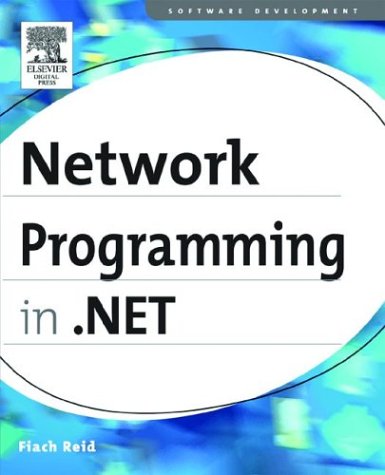
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPermission.cs
- RuleConditionDialog.Designer.cs
- Identity.cs
- MimeReflector.cs
- XhtmlTextWriter.cs
- Bezier.cs
- ComEventsInfo.cs
- XPathBinder.cs
- WindowsGrip.cs
- OrderingExpression.cs
- SqlDataSourceSelectingEventArgs.cs
- IIS7UserPrincipal.cs
- XPathBinder.cs
- ExtentCqlBlock.cs
- BitmapEffectGroup.cs
- SystemIPInterfaceStatistics.cs
- HttpCacheVary.cs
- StorageAssociationTypeMapping.cs
- DataTableCollection.cs
- ComplexLine.cs
- TimeIntervalCollection.cs
- HtmlSelect.cs
- DataGridCommandEventArgs.cs
- FontFamily.cs
- ObjectStateManager.cs
- WebControl.cs
- Array.cs
- formatstringdialog.cs
- SystemTcpStatistics.cs
- BamlTreeUpdater.cs
- SingletonChannelAcceptor.cs
- XsdBuilder.cs
- ResponseBodyWriter.cs
- CompositeScriptReferenceEventArgs.cs
- HttpCookiesSection.cs
- WebPartTransformerCollection.cs
- ColumnClickEvent.cs
- StylusSystemGestureEventArgs.cs
- ClientSettingsStore.cs
- Application.cs
- DataListItemCollection.cs
- SrgsElementList.cs
- ServiceSecurityAuditBehavior.cs
- CompensationDesigner.cs
- CodeTypeDelegate.cs
- CircleHotSpot.cs
- ExtendedPropertyInfo.cs
- XsltConvert.cs
- SemaphoreSecurity.cs
- Rights.cs
- Button.cs
- HwndTarget.cs
- SQLString.cs
- CorrelationTokenTypeConvertor.cs
- ByteStreamMessageEncoderFactory.cs
- ClientFormsIdentity.cs
- DbMetaDataCollectionNames.cs
- ReachDocumentPageSerializer.cs
- TextEffect.cs
- DataGridViewCellErrorTextNeededEventArgs.cs
- Utility.cs
- FontNamesConverter.cs
- HtmlControlPersistable.cs
- NavigationProperty.cs
- sqlnorm.cs
- MethodBuilder.cs
- ObfuscationAttribute.cs
- CommonDialog.cs
- TextParagraphProperties.cs
- FixedSOMTableRow.cs
- TransactionTraceIdentifier.cs
- View.cs
- PolygonHotSpot.cs
- ApplicationManager.cs
- CompensationTokenData.cs
- ToolStripArrowRenderEventArgs.cs
- Property.cs
- AuthenticationException.cs
- ValidatedControlConverter.cs
- FileAuthorizationModule.cs
- DetailsViewUpdatedEventArgs.cs
- TemplateContent.cs
- TreeViewItemAutomationPeer.cs
- Ipv6Element.cs
- XmlDownloadManager.cs
- CharacterHit.cs
- DashStyles.cs
- InheritedPropertyDescriptor.cs
- ErrorProvider.cs
- RC2CryptoServiceProvider.cs
- WebPart.cs
- Command.cs
- TypeToken.cs
- ProfileManager.cs
- Label.cs
- EntityDesignerUtils.cs
- EventWaitHandleSecurity.cs
- AdRotator.cs
- ModuleElement.cs
- WinEventWrap.cs