Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Controls / Label.cs / 1 / Label.cs
/****************************************************************************\ * * File: Label.cs * * Description: * Implements label control. * * Copyright (C) 2003-2006 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Windows.Threading; using System.Windows; using System.Windows.Input; using System.Windows.Markup; namespace System.Windows.Controls { ////// The Label control provides two basic pieces of functionality. It provides the /// ability to label a control -- called the "target" herein -- thus providing information /// to the application user about the target. The labeling is done through both /// the actual text of the Label control and through information surfaced through /// the UIAutomation API. The second function of the Label control is to provide /// mnemonics support -- both functional and visual -- the target. For example, the /// TextBox has no way of displaying additional information outside of its content. /// A Label control could help solve this problem. Note, the Label control is /// frequently used in dialogs to allow quick keyboard access to controls in the dialog. /// ///[Localizability(LocalizationCategory.Label)] public class Label : ContentControl { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors /// /// Static constructor /// static Label() { EventManager.RegisterClassHandler(typeof(Label), AccessKeyManager.AccessKeyPressedEvent, new AccessKeyPressedEventHandler(OnAccessKeyPressed)); DefaultStyleKeyProperty.OverrideMetadata(typeof(Label), new FrameworkPropertyMetadata(typeof(Label))); _dType = DependencyObjectType.FromSystemTypeInternal(typeof(Label)); // prevent label from being a tab stop and focusable IsTabStopProperty.OverrideMetadata(typeof(Label), new FrameworkPropertyMetadata(MS.Internal.KnownBoxes.BooleanBoxes.FalseBox)); FocusableProperty.OverrideMetadata(typeof(Label), new FrameworkPropertyMetadata(MS.Internal.KnownBoxes.BooleanBoxes.FalseBox)); } ////// Default Label constructor /// ////// Automatic determination of current Dispatcher. Use alternative constructor /// that accepts a Dispatcher for best performance. /// public Label() : base() { } #endregion //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// DependencyProperty for Target property. /// public static readonly DependencyProperty TargetProperty = DependencyProperty.Register( "Target", typeof(UIElement), typeof(Label), new FrameworkPropertyMetadata( (UIElement) null, new PropertyChangedCallback(OnTargetChanged))); ////// The target of this label. /// public UIElement Target { get { return (UIElement) GetValue(TargetProperty); } set { SetValue(TargetProperty, value); } } private static void OnTargetChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { Label label = (Label) d; UIElement oldElement = (UIElement) e.OldValue; UIElement newElement = (UIElement) e.NewValue; // If the Target property has changed, set the LabeledByProperty on // the new Target and clear the LabeledByProperty on the old Target. if (oldElement != null) { object oldValueLabeledBy = oldElement.GetValue(LabeledByProperty); // If this Label was actually labelling the oldValue // then clear the LabeledByProperty if (oldValueLabeledBy == label) { oldElement.ClearValue(LabeledByProperty); } } if (newElement != null) { newElement.SetValue(LabeledByProperty, label); } } ////// Attached DependencyProperty which indicates the element that is labeling /// another element. /// private static readonly DependencyProperty LabeledByProperty = DependencyProperty.RegisterAttached( "LabeledBy", typeof(Label), typeof(Label), new FrameworkPropertyMetadata((Label)null)); ////// Returns the Label that an element might have been labeled by. /// /// The element that might have been labeled. ///The Label that labels the element. Null otherwise. ////// This internal method is used by UIAutomation's FrameworkElementProxy. /// internal static Label GetLabeledBy(DependencyObject o) { if (o == null) { throw new ArgumentNullException("o"); } return (Label)o.GetValue(LabeledByProperty); } #endregion //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ ////// Creates AutomationPeer ( protected override System.Windows.Automation.Peers.AutomationPeer OnCreateAutomationPeer() { return new System.Windows.Automation.Peers.LabelAutomationPeer(this); } #region Private Methods private static void OnAccessKeyPressed(object sender, AccessKeyPressedEventArgs e) { Label label = sender as Label; // if (!e.Handled && e.Scope == null && (e.Target == null || e.Target == label)) { e.Target = label.Target; } } #endregion #region DTypeThemeStyleKey // Returns the DependencyObjectType for the registered ThemeStyleKey's default // value. Controls will override this method to return approriate types. internal override DependencyObjectType DTypeThemeStyleKey { get { return _dType; } } private static DependencyObjectType _dType; #endregion DTypeThemeStyleKey } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.) ///
Link Menu
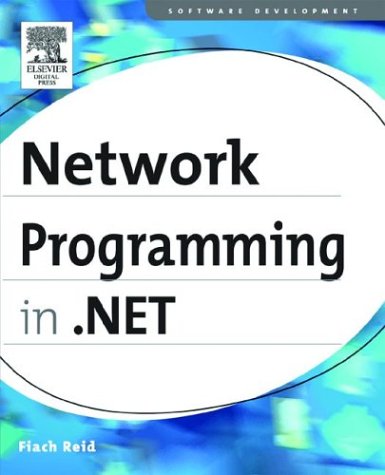
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StackSpiller.Generated.cs
- GB18030Encoding.cs
- IndexerReference.cs
- EncodedStreamFactory.cs
- IgnorePropertiesAttribute.cs
- IndexOutOfRangeException.cs
- PropertyGrid.cs
- StylusPointDescription.cs
- TextProviderWrapper.cs
- RowBinding.cs
- ProfessionalColorTable.cs
- PenThreadPool.cs
- ColorBuilder.cs
- WebPartEditVerb.cs
- XmlSchemaAnyAttribute.cs
- ConditionValidator.cs
- MsmqTransportSecurityElement.cs
- Barrier.cs
- PowerStatus.cs
- PropertyGridDesigner.cs
- NavigationWindowAutomationPeer.cs
- ActiveXHelper.cs
- WindowsEditBoxRange.cs
- ColorMatrix.cs
- GridViewRowPresenterBase.cs
- ToggleButton.cs
- DbProviderSpecificTypePropertyAttribute.cs
- DataServiceClientException.cs
- BeginCreateSecurityTokenRequest.cs
- ModuleElement.cs
- BaseCodeDomTreeGenerator.cs
- DataBindingCollection.cs
- IndentedWriter.cs
- bidPrivateBase.cs
- DocumentSchemaValidator.cs
- QueryGenerator.cs
- TableLayoutSettingsTypeConverter.cs
- DataGridViewRowsAddedEventArgs.cs
- EncoderReplacementFallback.cs
- IDictionary.cs
- MenuItemBindingCollection.cs
- XmlSchemas.cs
- XmlDownloadManager.cs
- NullableLongAverageAggregationOperator.cs
- GorillaCodec.cs
- CancellationTokenSource.cs
- InvalidWMPVersionException.cs
- RuntimeArgumentHandle.cs
- DefaultEvaluationContext.cs
- CompositeScriptReferenceEventArgs.cs
- RemotingServices.cs
- Unit.cs
- DataSourceSelectArguments.cs
- EntityDataSourceChangingEventArgs.cs
- IPAddressCollection.cs
- FileDialog_Vista.cs
- BasicHttpSecurity.cs
- EdmScalarPropertyAttribute.cs
- ADConnectionHelper.cs
- AxHost.cs
- SchemaCollectionPreprocessor.cs
- RequestCacheValidator.cs
- FontCacheUtil.cs
- MulticastNotSupportedException.cs
- AdapterSwitches.cs
- KeyEventArgs.cs
- UpnEndpointIdentity.cs
- ControlCachePolicy.cs
- NavigationWindowAutomationPeer.cs
- LinearKeyFrames.cs
- ResourceAssociationSetEnd.cs
- ActivityExecutionContext.cs
- RtfFormatStack.cs
- PageBuildProvider.cs
- SettingsPropertyIsReadOnlyException.cs
- ActivityStatusChangeEventArgs.cs
- MultiByteCodec.cs
- RequestCache.cs
- Timeline.cs
- formatter.cs
- ChannelFactoryRefCache.cs
- CreateSequenceResponse.cs
- Menu.cs
- TextBoxAutomationPeer.cs
- SiteOfOriginContainer.cs
- FontWeightConverter.cs
- SmtpMail.cs
- BamlLocalizationDictionary.cs
- TreeNodeBindingCollection.cs
- ConfigurationSection.cs
- AsyncCompletedEventArgs.cs
- ViewStateException.cs
- ExceptionHandlers.cs
- RestClientProxyHandler.cs
- BindingContext.cs
- TextTreeText.cs
- SafeCryptoHandles.cs
- Baml2006Reader.cs
- ToolBarTray.cs
- SynchronizationContext.cs