Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / MS / Internal / AppModel / SiteOfOriginContainer.cs / 1 / SiteOfOriginContainer.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // SiteOfOriginContainer is an implementation of the abstract Package class. // It contains nontrivial overrides for GetPartCore and Exists. // Many of the methods on Package are not applicable to loading application // resources, so the SiteOfOriginContainer implementations of these methods throw // the NotSupportedException. // // History: // 06/16/2005: Erichar - Initial creation. //----------------------------------------------------------------------------- using System; using System.IO.Packaging; using System.IO; using System.Collections.Generic; using System.Windows.Resources; using System.Resources; using System.Reflection; using System.Globalization; using System.Windows; using System.Windows.Navigation; using System.Deployment.Application; using System.Security; using System.Security.Permissions; using MS.Internal.PresentationCore; namespace MS.Internal.AppModel { ////// SiteOfOriginContainer is an implementation of the abstract Package class. /// It contains nontrivial overrides for GetPartCore and Exists. /// Many of the methods on Package are not applicable to loading files /// so the SiteOfOriginContainer implementations of these methods throw /// the NotSupportedException. /// internal class SiteOfOriginContainer : System.IO.Packaging.Package { //----------------------------------------------------- // // Static Methods // //----------------------------------------------------- #region Static Methods internal static Uri SiteOfOrigin { [FriendAccessAllowed] get { Uri siteOfOrigin = SiteOfOriginForBrowserApplications; if (siteOfOrigin == null) { // Calling FixFileUri because BaseDirectory will be a c:\\ style path siteOfOrigin = BaseUriHelper.FixFileUri(new Uri(System.AppDomain.CurrentDomain.BaseDirectory)); } #if DEBUG if (_traceSwitch.Enabled) System.Diagnostics.Trace.TraceInformation( DateTime.Now.ToLongTimeString() + " " + DateTime.Now.Millisecond + " " + System.Threading.Thread.CurrentThread.ManagedThreadId + ": SiteOfOriginContainer: returning site of origin " + siteOfOrigin); #endif return siteOfOrigin; } } // we separated this from the rest of the code because this code is used for media permission // tests in partial trust but we want to do this without hitting the code path for regular exe's // as in the code above. This will get hit for click once apps, xbaps, xaml and xps internal static Uri SiteOfOriginForBrowserApplications { [FriendAccessAllowed] get { Uri siteOfOrigin = null; if (_debugSecurityZoneURL.Value != null) { siteOfOrigin = _debugSecurityZoneURL.Value; } else if (_browserSource.Value != null) { siteOfOrigin = _browserSource.Value; } else if (ApplicationDeployment.IsNetworkDeployed) { siteOfOrigin = GetDeploymentUri(); } return siteOfOrigin; } } ////// Critical - Whatever is set here will be treated as site of origin. /// internal static Uri BrowserSource { get { return _browserSource.Value; } [SecurityCritical, FriendAccessAllowed] set { _browserSource.Value = value; } } // This is set when -debugsecurityzoneurl parameter is used. ////// Critical - Whatever is set here will be treated as site of origin. /// internal static Uri DebugSecurityZoneURL { get { return _debugSecurityZoneURL.Value; } [SecurityCritical, FriendAccessAllowed] set { _debugSecurityZoneURL.Value = value; } } #endregion //------------------------------------------------------ // // Public Constructors // //----------------------------------------------------- #region Public Constructors ////// Default Constructor /// internal SiteOfOriginContainer() : base(FileAccess.Read) { } #endregion //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ // None //----------------------------------------------------- // // Public Methods // //------------------------------------------------------ #region Public Methods ////// public override bool PartExists(Uri uri) { return true; } #endregion //----------------------------------------------------- // // Public Events // //----------------------------------------------------- // None //----------------------------------------------------- // // Internal Constructors // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties /// /// Critical: sets BooleanSwitch.Enabled which LinkDemands /// TreatAsSafe: ok to enable tracing /// internal static bool TraceSwitchEnabled { get { return _traceSwitch.Enabled; } [SecurityCritical, SecurityTreatAsSafe] set { _traceSwitch.Enabled = value; } } internal static System.Diagnostics.BooleanSwitch _traceSwitch = new System.Diagnostics.BooleanSwitch("SiteOfOrigin", "SiteOfOriginContainer and SiteOfOriginPart trace messages"); #endregion //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- // None //------------------------------------------------------ // // Internal Events // //----------------------------------------------------- // None //----------------------------------------------------- // // Protected Constructors // //----------------------------------------------------- // None //------------------------------------------------------ // // Protected Methods // //----------------------------------------------------- #region Protected Methods ////// This method creates a SiteOfOriginPart which will create a WebRequest /// to access files at the site of origin. /// /// ///protected override PackagePart GetPartCore(Uri uri) { #if DEBUG if (_traceSwitch.Enabled) System.Diagnostics.Trace.TraceInformation( DateTime.Now.ToLongTimeString() + " " + DateTime.Now.Millisecond + " " + System.Threading.Thread.CurrentThread.ManagedThreadId + ": SiteOfOriginContainer: Creating SiteOfOriginPart for Uri " + uri); #endif return new SiteOfOriginPart(this, uri); } #endregion //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ #region Private Methods /// /// Critical - Performs an elevation to access ApplicationIdentity /// TreatAsSafe - Returns the Uri the .application/.xapp was launched from if the application was network deployed. /// This will not work for a standalone application due to the invariant assert. This information is /// not considered critical because an application is allowed to know where it was deployed from just /// not the local path it is running from. /// This information is also typically available from ApplicationDeployment.CurrentDeployment.ActivationUri /// or ApplicationDeployment.CurrentDeployment.UpdateLocation but those properties are null for applications /// that do not take Uri parameters and do not receive updates from the web. Neither of those properties /// requires an assert to access and are available in partial trust. We are not using them because the need /// for a site of origin is not dependent on update or uri parameters. /// [SecurityCritical, SecurityTreatAsSafe] private static Uri GetDeploymentUri() { Invariant.Assert(ApplicationDeployment.IsNetworkDeployed); AppDomain currentDomain = System.AppDomain.CurrentDomain; ApplicationIdentity ident = null; string codeBase = null; SecurityPermission p1 = new SecurityPermission(SecurityPermissionFlag.ControlDomainPolicy); p1.Assert(); try { ident = currentDomain.ApplicationIdentity; // ControlDomainPolicy } finally { CodeAccessPermission.RevertAssert(); } SecurityPermission p2 = new SecurityPermission(SecurityPermissionFlag.UnmanagedCode); p2.Assert(); try { codeBase = ident.CodeBase; // Unmanaged Code permission } finally { CodeAccessPermission.RevertAssert(); } return new Uri(new Uri(codeBase), new Uri(".", UriKind.Relative)); } #endregion //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Members private static SecurityCriticalDataForSet_browserSource; private static SecurityCriticalDataForSet _debugSecurityZoneURL; #endregion Private Members //----------------------------------------------------- // // Uninteresting (but required) overrides // //----------------------------------------------------- #region Uninteresting (but required) overrides protected override PackagePart CreatePartCore(Uri uri, string contentType, CompressionOption compressionOption) { return null; } protected override void DeletePartCore(Uri uri) { throw new NotSupportedException(); } protected override PackagePart[] GetPartsCore() { throw new NotSupportedException(); } protected override void FlushCore() { throw new NotSupportedException(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // SiteOfOriginContainer is an implementation of the abstract Package class. // It contains nontrivial overrides for GetPartCore and Exists. // Many of the methods on Package are not applicable to loading application // resources, so the SiteOfOriginContainer implementations of these methods throw // the NotSupportedException. // // History: // 06/16/2005: Erichar - Initial creation. //----------------------------------------------------------------------------- using System; using System.IO.Packaging; using System.IO; using System.Collections.Generic; using System.Windows.Resources; using System.Resources; using System.Reflection; using System.Globalization; using System.Windows; using System.Windows.Navigation; using System.Deployment.Application; using System.Security; using System.Security.Permissions; using MS.Internal.PresentationCore; namespace MS.Internal.AppModel { ////// SiteOfOriginContainer is an implementation of the abstract Package class. /// It contains nontrivial overrides for GetPartCore and Exists. /// Many of the methods on Package are not applicable to loading files /// so the SiteOfOriginContainer implementations of these methods throw /// the NotSupportedException. /// internal class SiteOfOriginContainer : System.IO.Packaging.Package { //----------------------------------------------------- // // Static Methods // //----------------------------------------------------- #region Static Methods internal static Uri SiteOfOrigin { [FriendAccessAllowed] get { Uri siteOfOrigin = SiteOfOriginForBrowserApplications; if (siteOfOrigin == null) { // Calling FixFileUri because BaseDirectory will be a c:\\ style path siteOfOrigin = BaseUriHelper.FixFileUri(new Uri(System.AppDomain.CurrentDomain.BaseDirectory)); } #if DEBUG if (_traceSwitch.Enabled) System.Diagnostics.Trace.TraceInformation( DateTime.Now.ToLongTimeString() + " " + DateTime.Now.Millisecond + " " + System.Threading.Thread.CurrentThread.ManagedThreadId + ": SiteOfOriginContainer: returning site of origin " + siteOfOrigin); #endif return siteOfOrigin; } } // we separated this from the rest of the code because this code is used for media permission // tests in partial trust but we want to do this without hitting the code path for regular exe's // as in the code above. This will get hit for click once apps, xbaps, xaml and xps internal static Uri SiteOfOriginForBrowserApplications { [FriendAccessAllowed] get { Uri siteOfOrigin = null; if (_debugSecurityZoneURL.Value != null) { siteOfOrigin = _debugSecurityZoneURL.Value; } else if (_browserSource.Value != null) { siteOfOrigin = _browserSource.Value; } else if (ApplicationDeployment.IsNetworkDeployed) { siteOfOrigin = GetDeploymentUri(); } return siteOfOrigin; } } ////// Critical - Whatever is set here will be treated as site of origin. /// internal static Uri BrowserSource { get { return _browserSource.Value; } [SecurityCritical, FriendAccessAllowed] set { _browserSource.Value = value; } } // This is set when -debugsecurityzoneurl parameter is used. ////// Critical - Whatever is set here will be treated as site of origin. /// internal static Uri DebugSecurityZoneURL { get { return _debugSecurityZoneURL.Value; } [SecurityCritical, FriendAccessAllowed] set { _debugSecurityZoneURL.Value = value; } } #endregion //------------------------------------------------------ // // Public Constructors // //----------------------------------------------------- #region Public Constructors ////// Default Constructor /// internal SiteOfOriginContainer() : base(FileAccess.Read) { } #endregion //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ // None //----------------------------------------------------- // // Public Methods // //------------------------------------------------------ #region Public Methods ////// public override bool PartExists(Uri uri) { return true; } #endregion //----------------------------------------------------- // // Public Events // //----------------------------------------------------- // None //----------------------------------------------------- // // Internal Constructors // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties /// /// Critical: sets BooleanSwitch.Enabled which LinkDemands /// TreatAsSafe: ok to enable tracing /// internal static bool TraceSwitchEnabled { get { return _traceSwitch.Enabled; } [SecurityCritical, SecurityTreatAsSafe] set { _traceSwitch.Enabled = value; } } internal static System.Diagnostics.BooleanSwitch _traceSwitch = new System.Diagnostics.BooleanSwitch("SiteOfOrigin", "SiteOfOriginContainer and SiteOfOriginPart trace messages"); #endregion //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- // None //------------------------------------------------------ // // Internal Events // //----------------------------------------------------- // None //----------------------------------------------------- // // Protected Constructors // //----------------------------------------------------- // None //------------------------------------------------------ // // Protected Methods // //----------------------------------------------------- #region Protected Methods ////// This method creates a SiteOfOriginPart which will create a WebRequest /// to access files at the site of origin. /// /// ///protected override PackagePart GetPartCore(Uri uri) { #if DEBUG if (_traceSwitch.Enabled) System.Diagnostics.Trace.TraceInformation( DateTime.Now.ToLongTimeString() + " " + DateTime.Now.Millisecond + " " + System.Threading.Thread.CurrentThread.ManagedThreadId + ": SiteOfOriginContainer: Creating SiteOfOriginPart for Uri " + uri); #endif return new SiteOfOriginPart(this, uri); } #endregion //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ #region Private Methods /// /// Critical - Performs an elevation to access ApplicationIdentity /// TreatAsSafe - Returns the Uri the .application/.xapp was launched from if the application was network deployed. /// This will not work for a standalone application due to the invariant assert. This information is /// not considered critical because an application is allowed to know where it was deployed from just /// not the local path it is running from. /// This information is also typically available from ApplicationDeployment.CurrentDeployment.ActivationUri /// or ApplicationDeployment.CurrentDeployment.UpdateLocation but those properties are null for applications /// that do not take Uri parameters and do not receive updates from the web. Neither of those properties /// requires an assert to access and are available in partial trust. We are not using them because the need /// for a site of origin is not dependent on update or uri parameters. /// [SecurityCritical, SecurityTreatAsSafe] private static Uri GetDeploymentUri() { Invariant.Assert(ApplicationDeployment.IsNetworkDeployed); AppDomain currentDomain = System.AppDomain.CurrentDomain; ApplicationIdentity ident = null; string codeBase = null; SecurityPermission p1 = new SecurityPermission(SecurityPermissionFlag.ControlDomainPolicy); p1.Assert(); try { ident = currentDomain.ApplicationIdentity; // ControlDomainPolicy } finally { CodeAccessPermission.RevertAssert(); } SecurityPermission p2 = new SecurityPermission(SecurityPermissionFlag.UnmanagedCode); p2.Assert(); try { codeBase = ident.CodeBase; // Unmanaged Code permission } finally { CodeAccessPermission.RevertAssert(); } return new Uri(new Uri(codeBase), new Uri(".", UriKind.Relative)); } #endregion //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Members private static SecurityCriticalDataForSet_browserSource; private static SecurityCriticalDataForSet _debugSecurityZoneURL; #endregion Private Members //----------------------------------------------------- // // Uninteresting (but required) overrides // //----------------------------------------------------- #region Uninteresting (but required) overrides protected override PackagePart CreatePartCore(Uri uri, string contentType, CompressionOption compressionOption) { return null; } protected override void DeletePartCore(Uri uri) { throw new NotSupportedException(); } protected override PackagePart[] GetPartsCore() { throw new NotSupportedException(); } protected override void FlushCore() { throw new NotSupportedException(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
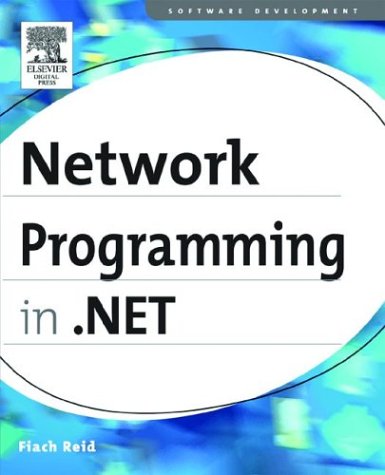
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BitStream.cs
- ServiceModelConfigurationSection.cs
- XmlSignatureManifest.cs
- Visual3D.cs
- LayoutInformation.cs
- QueryAccessibilityHelpEvent.cs
- ReflectionServiceProvider.cs
- PeerTransportElement.cs
- ReferenceEqualityComparer.cs
- PropertyPanel.cs
- CapabilitiesUse.cs
- TableCellCollection.cs
- SetterBase.cs
- UniqueConstraint.cs
- RowUpdatingEventArgs.cs
- rsa.cs
- OracleCommandBuilder.cs
- Type.cs
- MessageBox.cs
- Serializer.cs
- XmlNodeChangedEventManager.cs
- DynamicObject.cs
- HMAC.cs
- XmlSerializerSection.cs
- _DynamicWinsockMethods.cs
- LinqTreeNodeEvaluator.cs
- ListBoxChrome.cs
- HtmlMeta.cs
- DynamicDiscoSearcher.cs
- _CookieModule.cs
- AssemblyAttributes.cs
- ContextMenu.cs
- Rect.cs
- SmtpFailedRecipientsException.cs
- IgnoreDeviceFilterElementCollection.cs
- ToolboxComponentsCreatingEventArgs.cs
- RelationshipManager.cs
- DataObjectCopyingEventArgs.cs
- ConfigXmlSignificantWhitespace.cs
- AssociationTypeEmitter.cs
- PolyLineSegmentFigureLogic.cs
- FixedPageProcessor.cs
- unitconverter.cs
- ColorContextHelper.cs
- Encoder.cs
- DataIdProcessor.cs
- PerformanceCounters.cs
- StateMachineAction.cs
- WebPartRestoreVerb.cs
- SelectionProviderWrapper.cs
- URI.cs
- SqlAliaser.cs
- RelationshipWrapper.cs
- AppDomainUnloadedException.cs
- GraphicsPath.cs
- MachinePropertyVariants.cs
- TextDecorationCollectionConverter.cs
- SelectorItemAutomationPeer.cs
- ItemsPresenter.cs
- ProgressBarRenderer.cs
- ManipulationInertiaStartingEventArgs.cs
- TranslateTransform3D.cs
- KeyboardEventArgs.cs
- XmlIgnoreAttribute.cs
- SymbolEqualComparer.cs
- SHA512Managed.cs
- AssociationSet.cs
- _SSPIWrapper.cs
- DataGridViewCellFormattingEventArgs.cs
- PageAsyncTaskManager.cs
- DataGridViewRowStateChangedEventArgs.cs
- ProfilePropertySettings.cs
- FixedDocumentSequencePaginator.cs
- XsltFunctions.cs
- TypedAsyncResult.cs
- GridViewCellAutomationPeer.cs
- Rule.cs
- XsltSettings.cs
- SafeCoTaskMem.cs
- SrgsRuleRef.cs
- ChannelSinkStacks.cs
- DelayedRegex.cs
- ContentElement.cs
- WpfWebRequestHelper.cs
- dbenumerator.cs
- SqlAggregateChecker.cs
- ListViewDeletedEventArgs.cs
- NullableLongAverageAggregationOperator.cs
- MethodImplAttribute.cs
- RC2.cs
- ArgumentDesigner.xaml.cs
- FaultContractInfo.cs
- PenContexts.cs
- GroupPartitionExpr.cs
- HttpListenerRequest.cs
- _AutoWebProxyScriptWrapper.cs
- WriteFileContext.cs
- Version.cs
- Update.cs
- UrlPropertyAttribute.cs