Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Documents / Span.cs / 1305600 / Span.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Span class - inline element grouping several other inline elements // //--------------------------------------------------------------------------- using MS.Internal; // Invariant.Assert using System.Windows.Controls; // TextBlock using System.Windows.Markup; // ContentProperty using System.ComponentModel; // DesignerSerializationVisibility namespace System.Windows.Documents { ////// Span element used for grouping other Inline elements. /// [ContentProperty("Inlines")] public class Span : Inline { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Initializes a new instance of a Span element. /// public Span() { } ////// Initializes a new instance of a Span element. /// /// /// An Inline element added to this Span as its first child. /// public Span(Inline childInline) : this(childInline, null) { } ////// Creates a new Span instance. /// /// /// Optional child Inline for the new Span. May be null. /// /// /// Optional position at which to insert the new Span. May be null. /// public Span(Inline childInline, TextPointer insertionPosition) { if (insertionPosition != null) { insertionPosition.TextContainer.BeginChange(); } try { if (insertionPosition != null) { // This will throw InvalidOperationException if schema validity is violated. insertionPosition.InsertInline(this); } if (childInline != null) { this.Inlines.Add(childInline); } } finally { if (insertionPosition != null) { insertionPosition.TextContainer.EndChange(); } } } ////// Creates a new Span instance covering existing content. /// /// /// Start position of the new Span. /// /// /// End position of the new Span. /// ////// start and end must both be parented by the same Paragraph, otherwise /// the method will raise an ArgumentException. /// public Span(TextPointer start, TextPointer end) { if (start == null) { throw new ArgumentNullException("start"); } if (end == null) { throw new ArgumentNullException("start"); } if (start.TextContainer != end.TextContainer) { throw new ArgumentException(SR.Get(SRID.InDifferentTextContainers, "start", "end")); } if (start.CompareTo(end) > 0) { throw new ArgumentException(SR.Get(SRID.BadTextPositionOrder, "start", "end")); } start.TextContainer.BeginChange(); try { start = TextRangeEditTables.EnsureInsertionPosition(start); Invariant.Assert(start.Parent is Run); end = TextRangeEditTables.EnsureInsertionPosition(end); Invariant.Assert(end.Parent is Run); if (start.Paragraph != end.Paragraph) { throw new ArgumentException(SR.Get(SRID.InDifferentParagraphs, "start", "end")); } // If start or end positions have a Hyperlink ancestor, we cannot split them. Inline nonMergeableAncestor; if ((nonMergeableAncestor = start.GetNonMergeableInlineAncestor()) != null) { throw new InvalidOperationException(SR.Get(SRID.TextSchema_CannotSplitElement, nonMergeableAncestor.GetType().Name)); } if ((nonMergeableAncestor = end.GetNonMergeableInlineAncestor()) != null) { throw new InvalidOperationException(SR.Get(SRID.TextSchema_CannotSplitElement, nonMergeableAncestor.GetType().Name)); } TextElement commonAncestor = TextElement.GetCommonAncestor((TextElement)start.Parent, (TextElement)end.Parent); while (start.Parent != commonAncestor) { start = SplitElement(start); } while (end.Parent != commonAncestor) { end = SplitElement(end); } if (start.Parent is Run) { start = SplitElement(start); } if (end.Parent is Run) { end = SplitElement(end); } Invariant.Assert(start.Parent == end.Parent); Invariant.Assert(TextSchema.IsValidChild(/*position*/start, /*childType*/typeof(Span))); this.Reposition(start, end); } finally { start.TextContainer.EndChange(); } } #endregion Constructors //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// Collection of Inline items contained in this Section. /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public InlineCollection Inlines { get { return new InlineCollection(this, /*isOwnerParent*/true); } } #endregion Public Properties //-------------------------------------------------------------------- // // Internal Methods // //-------------------------------------------------------------------- #region Internal Methods ////// This method is used by TypeDescriptor to determine if this property should /// be serialized. /// [EditorBrowsable(EditorBrowsableState.Never)] public bool ShouldSerializeInlines(XamlDesignerSerializationManager manager) { return manager != null && manager.XmlWriter == null; } #endregion Internal Methods //------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------- #region Private Methods // Splits the parent TextElement of a TextPointer, returning a TextPointer // between the two split halves. // If the TextPointer is adjacent to one of the TextElement's edges, // this method does not split the element, and instead returns a pointer // adjacent to the bordered edge, outside the TextElemetn scope. private TextPointer SplitElement(TextPointer position) { if (position.GetPointerContext(LogicalDirection.Backward) == TextPointerContext.ElementStart) { position = position.GetNextContextPosition(LogicalDirection.Backward); } else if (position.GetPointerContext(LogicalDirection.Forward) == TextPointerContext.ElementEnd) { position = position.GetNextContextPosition(LogicalDirection.Forward); } else { position = TextRangeEdit.SplitElement(position); } return position; } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Span class - inline element grouping several other inline elements // //--------------------------------------------------------------------------- using MS.Internal; // Invariant.Assert using System.Windows.Controls; // TextBlock using System.Windows.Markup; // ContentProperty using System.ComponentModel; // DesignerSerializationVisibility namespace System.Windows.Documents { ////// Span element used for grouping other Inline elements. /// [ContentProperty("Inlines")] public class Span : Inline { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Initializes a new instance of a Span element. /// public Span() { } ////// Initializes a new instance of a Span element. /// /// /// An Inline element added to this Span as its first child. /// public Span(Inline childInline) : this(childInline, null) { } ////// Creates a new Span instance. /// /// /// Optional child Inline for the new Span. May be null. /// /// /// Optional position at which to insert the new Span. May be null. /// public Span(Inline childInline, TextPointer insertionPosition) { if (insertionPosition != null) { insertionPosition.TextContainer.BeginChange(); } try { if (insertionPosition != null) { // This will throw InvalidOperationException if schema validity is violated. insertionPosition.InsertInline(this); } if (childInline != null) { this.Inlines.Add(childInline); } } finally { if (insertionPosition != null) { insertionPosition.TextContainer.EndChange(); } } } ////// Creates a new Span instance covering existing content. /// /// /// Start position of the new Span. /// /// /// End position of the new Span. /// ////// start and end must both be parented by the same Paragraph, otherwise /// the method will raise an ArgumentException. /// public Span(TextPointer start, TextPointer end) { if (start == null) { throw new ArgumentNullException("start"); } if (end == null) { throw new ArgumentNullException("start"); } if (start.TextContainer != end.TextContainer) { throw new ArgumentException(SR.Get(SRID.InDifferentTextContainers, "start", "end")); } if (start.CompareTo(end) > 0) { throw new ArgumentException(SR.Get(SRID.BadTextPositionOrder, "start", "end")); } start.TextContainer.BeginChange(); try { start = TextRangeEditTables.EnsureInsertionPosition(start); Invariant.Assert(start.Parent is Run); end = TextRangeEditTables.EnsureInsertionPosition(end); Invariant.Assert(end.Parent is Run); if (start.Paragraph != end.Paragraph) { throw new ArgumentException(SR.Get(SRID.InDifferentParagraphs, "start", "end")); } // If start or end positions have a Hyperlink ancestor, we cannot split them. Inline nonMergeableAncestor; if ((nonMergeableAncestor = start.GetNonMergeableInlineAncestor()) != null) { throw new InvalidOperationException(SR.Get(SRID.TextSchema_CannotSplitElement, nonMergeableAncestor.GetType().Name)); } if ((nonMergeableAncestor = end.GetNonMergeableInlineAncestor()) != null) { throw new InvalidOperationException(SR.Get(SRID.TextSchema_CannotSplitElement, nonMergeableAncestor.GetType().Name)); } TextElement commonAncestor = TextElement.GetCommonAncestor((TextElement)start.Parent, (TextElement)end.Parent); while (start.Parent != commonAncestor) { start = SplitElement(start); } while (end.Parent != commonAncestor) { end = SplitElement(end); } if (start.Parent is Run) { start = SplitElement(start); } if (end.Parent is Run) { end = SplitElement(end); } Invariant.Assert(start.Parent == end.Parent); Invariant.Assert(TextSchema.IsValidChild(/*position*/start, /*childType*/typeof(Span))); this.Reposition(start, end); } finally { start.TextContainer.EndChange(); } } #endregion Constructors //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// Collection of Inline items contained in this Section. /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public InlineCollection Inlines { get { return new InlineCollection(this, /*isOwnerParent*/true); } } #endregion Public Properties //-------------------------------------------------------------------- // // Internal Methods // //-------------------------------------------------------------------- #region Internal Methods ////// This method is used by TypeDescriptor to determine if this property should /// be serialized. /// [EditorBrowsable(EditorBrowsableState.Never)] public bool ShouldSerializeInlines(XamlDesignerSerializationManager manager) { return manager != null && manager.XmlWriter == null; } #endregion Internal Methods //------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------- #region Private Methods // Splits the parent TextElement of a TextPointer, returning a TextPointer // between the two split halves. // If the TextPointer is adjacent to one of the TextElement's edges, // this method does not split the element, and instead returns a pointer // adjacent to the bordered edge, outside the TextElemetn scope. private TextPointer SplitElement(TextPointer position) { if (position.GetPointerContext(LogicalDirection.Backward) == TextPointerContext.ElementStart) { position = position.GetNextContextPosition(LogicalDirection.Backward); } else if (position.GetPointerContext(LogicalDirection.Forward) == TextPointerContext.ElementEnd) { position = position.GetNextContextPosition(LogicalDirection.Forward); } else { position = TextRangeEdit.SplitElement(position); } return position; } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
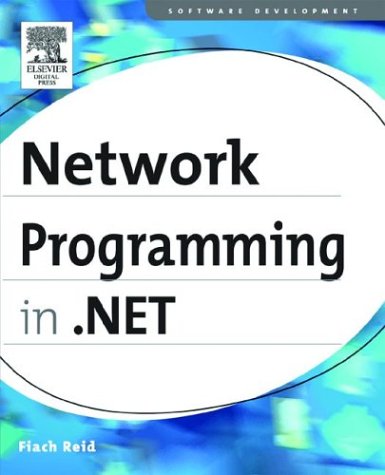
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AncillaryOps.cs
- DataControlLinkButton.cs
- MsmqBindingFilter.cs
- XmlWriterSettings.cs
- RectConverter.cs
- XPathBinder.cs
- SchemaNames.cs
- Cell.cs
- CodeCatchClauseCollection.cs
- TransformerTypeCollection.cs
- TemplateField.cs
- ConfigurationLockCollection.cs
- GB18030Encoding.cs
- DomainLiteralReader.cs
- HandlerBase.cs
- SendSecurityHeader.cs
- NetworkAddressChange.cs
- ShapingWorkspace.cs
- WebPartMenu.cs
- TdsValueSetter.cs
- StateManagedCollection.cs
- AnnotationAdorner.cs
- HatchBrush.cs
- MemoryMappedFile.cs
- SecurityTokenException.cs
- SmiEventSink_DeferedProcessing.cs
- AspNetHostingPermission.cs
- CalendarDesigner.cs
- AggregateException.cs
- HttpClientCredentialType.cs
- ConnectionConsumerAttribute.cs
- DomainUpDown.cs
- RequestCacheManager.cs
- CreateRefExpr.cs
- PtsHost.cs
- Visual3DCollection.cs
- WorkflowViewElement.cs
- PrintEvent.cs
- SAPICategories.cs
- Item.cs
- BaseCollection.cs
- PropertyTabChangedEvent.cs
- EdmEntityTypeAttribute.cs
- Window.cs
- SplitterPanel.cs
- OdbcConnectionPoolProviderInfo.cs
- TextMarkerSource.cs
- OracleConnection.cs
- MergePropertyDescriptor.cs
- Filter.cs
- SwitchExpression.cs
- SecurityStandardsManager.cs
- ScrollItemPattern.cs
- EntityEntry.cs
- Membership.cs
- CompilationSection.cs
- Pens.cs
- Wildcard.cs
- PopupEventArgs.cs
- HostAdapter.cs
- RTLAwareMessageBox.cs
- GeneralTransform3DTo2DTo3D.cs
- TypedReference.cs
- StdRegProviderWrapper.cs
- HttpProtocolImporter.cs
- DesignerVerbCollection.cs
- Win32PrintDialog.cs
- ToolStripOverflow.cs
- RNGCryptoServiceProvider.cs
- ClientSettingsSection.cs
- BooleanFunctions.cs
- XamlSerializationHelper.cs
- ZoneButton.cs
- FindCriteria11.cs
- PriorityRange.cs
- RewritingValidator.cs
- SpoolingTaskBase.cs
- RelationalExpressions.cs
- BitmapPalette.cs
- ArithmeticLiteral.cs
- webproxy.cs
- __TransparentProxy.cs
- BaseValidator.cs
- AddressUtility.cs
- EUCJPEncoding.cs
- ManagementNamedValueCollection.cs
- Image.cs
- SpellerStatusTable.cs
- DrawingImage.cs
- XmlEventCache.cs
- MailMessage.cs
- NetStream.cs
- PersistenceTypeAttribute.cs
- WindowsListView.cs
- PersistenceParticipant.cs
- LogLogRecordEnumerator.cs
- ProvidersHelper.cs
- SequentialWorkflowRootDesigner.cs
- Style.cs
- PhysicalFontFamily.cs