Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / MS / Internal / Annotations / Component / AnnotationAdorner.cs / 1 / AnnotationAdorner.cs
//---------------------------------------------------------------------------- //// Copyright(C) Microsoft Corporation. All rights reserved. // // // Description: // AnnotationAdorner wraps an IAnnotationComponent. Its the bridge // between the component and the adorner layer. // // History: // 04/01/2004: axelk: Created AnnotationAdorner.cs // 10/20/2004: rruiz: Moved class to MS.Internal. // // Copyright(C) 2002 by Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Windows; using System.Windows.Documents; using System.Windows.Media; namespace MS.Internal.Annotations.Component { ////// An adorner which wraps one IAnnotationComponent. The wrapped component must be at least a UIElement. /// Adorners are UIElements. /// Note:-This class is sealed because it calls OnVisualChildrenChanged virtual in the /// constructor and it does not override it, but derived classes could. /// internal sealed class AnnotationAdorner : Adorner { #region Constructors ////// Return an initialized annotation adorner /// /// The annotation component to wrap in the annotation adorner /// element being annotated public AnnotationAdorner(IAnnotationComponent component, UIElement annotatedElement): base(annotatedElement) { //The summary on top of the file says:- //The wrapped component must be at least a UIElement if(component is UIElement) { _annotationComponent = component; // wrapped annotation component is added as visual child this.AddVisualChild((UIElement)_annotationComponent); } else { throw new ArgumentException(SR.Get(SRID.AnnotationAdorner_NotUIElement), "component"); } } #endregion Constructors #region Public Methods ////// Forwarded to the annotation component to get desired transform relative to the annotated element /// /// Transform to adorned element ///Transform to annotation component public override GeneralTransform GetDesiredTransform(GeneralTransform transform) { //if the component is not visual we do not need this if (!(_annotationComponent is UIElement)) return null; // Give the superclass a chance to modify the transform transform = base.GetDesiredTransform(transform); GeneralTransform compTransform = _annotationComponent.GetDesiredTransform(transform); //ToDo. if the annotated element is null this component must be unloaded. //Temporary return null until the PageViewer Load/Unload bug is fixed //Convert it to an exception after that. if (_annotationComponent.AnnotatedElement == null) return null; if (compTransform == null) { // We need to store the element we are registering on. It may not // be available from the annotation component later. _annotatedElement = _annotationComponent.AnnotatedElement; // Wait for valid text view _annotatedElement.LayoutUpdated += OnLayoutUpdated; return transform; } return compTransform; } #endregion Public Methods #region Protected Methods ////// Derived class must implement to support Visual children. The method must return /// the child at the specified index. Index must be between 0 and GetVisualChildrenCount-1. /// /// By default a Visual does not have any children. /// /// Remark: /// During this virtual call it is not valid to modify the Visual tree. /// protected override Visual GetVisualChild(int index) { if(index != 0 || _annotationComponent == null) { throw new ArgumentOutOfRangeException("index", index, SR.Get(SRID.Visual_ArgumentOutOfRange)); } return (UIElement)_annotationComponent; } ////// Derived classes override this property to enable the Visual code to enumerate /// the Visual children. Derived classes need to return the number of children /// from this method. /// /// By default a Visual does not have any children. /// /// Remark: During this virtual method the Visual tree must not be modified. /// protected override int VisualChildrenCount { get { return _annotationComponent != null ? 1 : 0; } } ////// Measurement override. Delegated to children. /// /// Available size for the component ///Return the size enclosing all children protected override Size MeasureOverride(Size availableSize) { Size childConstraint = new Size(Double.PositiveInfinity, Double.PositiveInfinity); Invariant.Assert(_annotationComponent != null, "AnnotationAdorner should only have one child - the annotation component."); ((UIElement)_annotationComponent).Measure(childConstraint); return new Size(0,0); } ////// Override for /// The location reserved for this element by the parent protected override Size ArrangeOverride(Size finalSize) { Invariant.Assert(_annotationComponent != null, "AnnotationAdorner should only have one child - the annotation component."); ((UIElement)_annotationComponent).Arrange(new Rect(((UIElement)_annotationComponent).DesiredSize)); return finalSize; } #endregion ProtectedMethods #region Internal Methods ////// /// Remove all visual children of this AnnotationAdorner. /// Called by AdornerPresentationContext when an annotation adorner is removed from the adorner layer hosting it. /// internal void RemoveChildren() { this.RemoveVisualChild((UIElement)_annotationComponent); _annotationComponent = null; } ////// Call this if the visual of the annotation changes. /// This ill invalidate the AnnotationAdorner and the AdornerLayer which /// will invoke remeasuring of the annotation visual. /// internal void InvalidateTransform() { AdornerLayer adornerLayer = (AdornerLayer)VisualTreeHelper.GetParent(this); InvalidateMeasure(); adornerLayer.InvalidateVisual(); } #endregion Internal Methods #region Internal Properties ////// Return the annotation component that is being wrapped. AdornerPresentationContext needs this. /// ///Wrapped annotation component internal IAnnotationComponent AnnotationComponent { get { return _annotationComponent; } } #endregion Internal Properties #region Private Methods ////// LayoutUpdate event handler /// /// event sender (not used) /// event arguments (not used) private void OnLayoutUpdated(object sender, EventArgs args) { // Unregister for the event _annotatedElement.LayoutUpdated -= OnLayoutUpdated; _annotatedElement = null; // If there are still annotations to display, update the UI if (_annotationComponent.AttachedAnnotations.Count > 0) { _annotationComponent.PresentationContext.Host.InvalidateMeasure(); this.InvalidateMeasure(); } } #endregion Private Methods #region Private Fields ////// The wrapped annotation component /// private IAnnotationComponent _annotationComponent; ////// Used to unregister for the LayoutUpdated event - necessary because in the /// the component may have its annotated element cleared before we can unregister. /// private UIElement _annotatedElement; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- //// Copyright(C) Microsoft Corporation. All rights reserved. // // // Description: // AnnotationAdorner wraps an IAnnotationComponent. Its the bridge // between the component and the adorner layer. // // History: // 04/01/2004: axelk: Created AnnotationAdorner.cs // 10/20/2004: rruiz: Moved class to MS.Internal. // // Copyright(C) 2002 by Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Windows; using System.Windows.Documents; using System.Windows.Media; namespace MS.Internal.Annotations.Component { ////// An adorner which wraps one IAnnotationComponent. The wrapped component must be at least a UIElement. /// Adorners are UIElements. /// Note:-This class is sealed because it calls OnVisualChildrenChanged virtual in the /// constructor and it does not override it, but derived classes could. /// internal sealed class AnnotationAdorner : Adorner { #region Constructors ////// Return an initialized annotation adorner /// /// The annotation component to wrap in the annotation adorner /// element being annotated public AnnotationAdorner(IAnnotationComponent component, UIElement annotatedElement): base(annotatedElement) { //The summary on top of the file says:- //The wrapped component must be at least a UIElement if(component is UIElement) { _annotationComponent = component; // wrapped annotation component is added as visual child this.AddVisualChild((UIElement)_annotationComponent); } else { throw new ArgumentException(SR.Get(SRID.AnnotationAdorner_NotUIElement), "component"); } } #endregion Constructors #region Public Methods ////// Forwarded to the annotation component to get desired transform relative to the annotated element /// /// Transform to adorned element ///Transform to annotation component public override GeneralTransform GetDesiredTransform(GeneralTransform transform) { //if the component is not visual we do not need this if (!(_annotationComponent is UIElement)) return null; // Give the superclass a chance to modify the transform transform = base.GetDesiredTransform(transform); GeneralTransform compTransform = _annotationComponent.GetDesiredTransform(transform); //ToDo. if the annotated element is null this component must be unloaded. //Temporary return null until the PageViewer Load/Unload bug is fixed //Convert it to an exception after that. if (_annotationComponent.AnnotatedElement == null) return null; if (compTransform == null) { // We need to store the element we are registering on. It may not // be available from the annotation component later. _annotatedElement = _annotationComponent.AnnotatedElement; // Wait for valid text view _annotatedElement.LayoutUpdated += OnLayoutUpdated; return transform; } return compTransform; } #endregion Public Methods #region Protected Methods ////// Derived class must implement to support Visual children. The method must return /// the child at the specified index. Index must be between 0 and GetVisualChildrenCount-1. /// /// By default a Visual does not have any children. /// /// Remark: /// During this virtual call it is not valid to modify the Visual tree. /// protected override Visual GetVisualChild(int index) { if(index != 0 || _annotationComponent == null) { throw new ArgumentOutOfRangeException("index", index, SR.Get(SRID.Visual_ArgumentOutOfRange)); } return (UIElement)_annotationComponent; } ////// Derived classes override this property to enable the Visual code to enumerate /// the Visual children. Derived classes need to return the number of children /// from this method. /// /// By default a Visual does not have any children. /// /// Remark: During this virtual method the Visual tree must not be modified. /// protected override int VisualChildrenCount { get { return _annotationComponent != null ? 1 : 0; } } ////// Measurement override. Delegated to children. /// /// Available size for the component ///Return the size enclosing all children protected override Size MeasureOverride(Size availableSize) { Size childConstraint = new Size(Double.PositiveInfinity, Double.PositiveInfinity); Invariant.Assert(_annotationComponent != null, "AnnotationAdorner should only have one child - the annotation component."); ((UIElement)_annotationComponent).Measure(childConstraint); return new Size(0,0); } ////// Override for /// The location reserved for this element by the parent protected override Size ArrangeOverride(Size finalSize) { Invariant.Assert(_annotationComponent != null, "AnnotationAdorner should only have one child - the annotation component."); ((UIElement)_annotationComponent).Arrange(new Rect(((UIElement)_annotationComponent).DesiredSize)); return finalSize; } #endregion ProtectedMethods #region Internal Methods ////// /// Remove all visual children of this AnnotationAdorner. /// Called by AdornerPresentationContext when an annotation adorner is removed from the adorner layer hosting it. /// internal void RemoveChildren() { this.RemoveVisualChild((UIElement)_annotationComponent); _annotationComponent = null; } ////// Call this if the visual of the annotation changes. /// This ill invalidate the AnnotationAdorner and the AdornerLayer which /// will invoke remeasuring of the annotation visual. /// internal void InvalidateTransform() { AdornerLayer adornerLayer = (AdornerLayer)VisualTreeHelper.GetParent(this); InvalidateMeasure(); adornerLayer.InvalidateVisual(); } #endregion Internal Methods #region Internal Properties ////// Return the annotation component that is being wrapped. AdornerPresentationContext needs this. /// ///Wrapped annotation component internal IAnnotationComponent AnnotationComponent { get { return _annotationComponent; } } #endregion Internal Properties #region Private Methods ////// LayoutUpdate event handler /// /// event sender (not used) /// event arguments (not used) private void OnLayoutUpdated(object sender, EventArgs args) { // Unregister for the event _annotatedElement.LayoutUpdated -= OnLayoutUpdated; _annotatedElement = null; // If there are still annotations to display, update the UI if (_annotationComponent.AttachedAnnotations.Count > 0) { _annotationComponent.PresentationContext.Host.InvalidateMeasure(); this.InvalidateMeasure(); } } #endregion Private Methods #region Private Fields ////// The wrapped annotation component /// private IAnnotationComponent _annotationComponent; ////// Used to unregister for the LayoutUpdated event - necessary because in the /// the component may have its annotated element cleared before we can unregister. /// private UIElement _annotatedElement; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
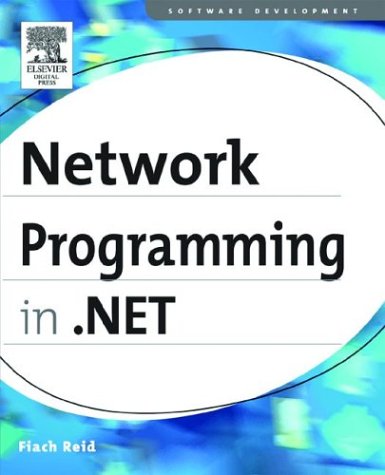
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DrawingDrawingContext.cs
- WinInet.cs
- SchemaElement.cs
- SynchronizedDispatch.cs
- HtmlMeta.cs
- SoapWriter.cs
- TextDecorationCollection.cs
- EncryptedType.cs
- SynthesizerStateChangedEventArgs.cs
- QilPatternFactory.cs
- LiteralDesigner.cs
- PeerCollaboration.cs
- HMACSHA256.cs
- HttpRuntimeSection.cs
- OracleRowUpdatedEventArgs.cs
- SqlServices.cs
- LassoSelectionBehavior.cs
- OdbcTransaction.cs
- GridView.cs
- StandardBindingElementCollection.cs
- RegionInfo.cs
- UrlAuthFailedErrorFormatter.cs
- ComplexPropertyEntry.cs
- TableLayoutStyleCollection.cs
- BuilderInfo.cs
- Propagator.cs
- Decimal.cs
- HtmlTable.cs
- SimpleRecyclingCache.cs
- RTLAwareMessageBox.cs
- OleDbFactory.cs
- ValueExpressions.cs
- SequenceDesigner.cs
- DataGridViewRowsAddedEventArgs.cs
- ToolStripDropDownMenu.cs
- StylusLogic.cs
- InputScopeAttribute.cs
- PreProcessInputEventArgs.cs
- XmlFormatExtensionAttribute.cs
- PackUriHelper.cs
- Propagator.cs
- TypeTypeConverter.cs
- GC.cs
- SafeSecurityHandles.cs
- ServiceOperationListItem.cs
- Delay.cs
- TransactionWaitAsyncResult.cs
- PolyQuadraticBezierSegment.cs
- Converter.cs
- CultureMapper.cs
- DataContract.cs
- OleStrCAMarshaler.cs
- EntityStoreSchemaGenerator.cs
- EntityCommand.cs
- safemediahandle.cs
- WindowsButton.cs
- TypeUnloadedException.cs
- SHA1Managed.cs
- DbConnectionPoolIdentity.cs
- DateTimeOffsetConverter.cs
- MsmqHostedTransportConfiguration.cs
- Completion.cs
- PropertyRecord.cs
- FormViewDeletedEventArgs.cs
- SchemaMapping.cs
- AnnotationResourceCollection.cs
- LicFileLicenseProvider.cs
- ToolStripDesignerAvailabilityAttribute.cs
- CodeEventReferenceExpression.cs
- DSASignatureDeformatter.cs
- WebPartDisplayMode.cs
- AssociationProvider.cs
- DesignerActionUI.cs
- SqlUtils.cs
- DataControlFieldHeaderCell.cs
- Pair.cs
- TransactionScope.cs
- DialogResultConverter.cs
- SqlGatherProducedAliases.cs
- VisualProxy.cs
- LinkAreaEditor.cs
- XPathExpr.cs
- XmlEncodedRawTextWriter.cs
- DBAsyncResult.cs
- webclient.cs
- KeyGesture.cs
- DataGridLinkButton.cs
- StylusPointCollection.cs
- IFormattable.cs
- KnownBoxes.cs
- TraceFilter.cs
- RubberbandSelector.cs
- RootBuilder.cs
- DataGridViewDataErrorEventArgs.cs
- MetadataUtil.cs
- ParameterModifier.cs
- QueryParameter.cs
- CancellationState.cs
- CompilerTypeWithParams.cs
- SettingsSection.cs