Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / HttpClientCredentialType.cs / 1 / HttpClientCredentialType.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel { using System.Net; public enum HttpClientCredentialType { None, Basic, Digest, Ntlm, Windows, Certificate } static class HttpClientCredentialTypeHelper { internal static bool IsDefined(HttpClientCredentialType value) { return (value == HttpClientCredentialType.None || value == HttpClientCredentialType.Basic || value == HttpClientCredentialType.Digest || value == HttpClientCredentialType.Ntlm || value == HttpClientCredentialType.Windows || value == HttpClientCredentialType.Certificate); } internal static AuthenticationSchemes MapToAuthenticationScheme(HttpClientCredentialType clientCredentialType) { AuthenticationSchemes result; switch (clientCredentialType) { case HttpClientCredentialType.Certificate: // fall through to None case case HttpClientCredentialType.None: result = AuthenticationSchemes.Anonymous; break; case HttpClientCredentialType.Basic: result = AuthenticationSchemes.Basic; break; case HttpClientCredentialType.Digest: result = AuthenticationSchemes.Digest; break; case HttpClientCredentialType.Ntlm: result = AuthenticationSchemes.Ntlm; break; case HttpClientCredentialType.Windows: result = AuthenticationSchemes.Negotiate; break; default: DiagnosticUtility.DebugAssert("unsupported client credential type"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } return result; } internal static HttpClientCredentialType MapToClientCredentialType(AuthenticationSchemes authenticationSchemes) { HttpClientCredentialType result; switch (authenticationSchemes) { case AuthenticationSchemes.Anonymous: result = HttpClientCredentialType.None; break; case AuthenticationSchemes.Basic: result = HttpClientCredentialType.Basic; break; case AuthenticationSchemes.Digest: result = HttpClientCredentialType.Digest; break; case AuthenticationSchemes.Ntlm: result = HttpClientCredentialType.Ntlm; break; case AuthenticationSchemes.Negotiate: result = HttpClientCredentialType.Windows; break; default: DiagnosticUtility.DebugAssert("unsupported client AuthenticationScheme"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
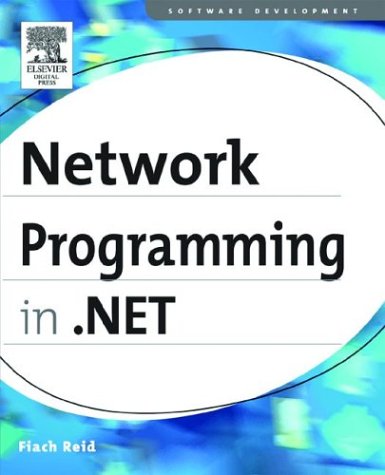
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Drawing.cs
- Privilege.cs
- FacetValues.cs
- ThreadStartException.cs
- NonSerializedAttribute.cs
- SQLBinaryStorage.cs
- GridViewColumnHeaderAutomationPeer.cs
- SystemWebSectionGroup.cs
- WebPartRestoreVerb.cs
- DataAdapter.cs
- Process.cs
- ResourceWriter.cs
- FreezableOperations.cs
- EFColumnProvider.cs
- ListSurrogate.cs
- NativeObjectSecurity.cs
- RoleGroup.cs
- ipaddressinformationcollection.cs
- FaultException.cs
- ExchangeUtilities.cs
- PackUriHelper.cs
- EntityCommandExecutionException.cs
- XmlConvert.cs
- HtmlInputRadioButton.cs
- clipboard.cs
- LayoutEditorPart.cs
- ClientApiGenerator.cs
- SourceFilter.cs
- EncodingInfo.cs
- RegexMatch.cs
- DockProviderWrapper.cs
- SqlBuffer.cs
- MappingItemCollection.cs
- ICollection.cs
- ComMethodElementCollection.cs
- SiteMapSection.cs
- TagPrefixAttribute.cs
- ToolStripStatusLabel.cs
- GregorianCalendarHelper.cs
- Color.cs
- ListMarkerSourceInfo.cs
- InputProviderSite.cs
- ChangesetResponse.cs
- TextPattern.cs
- StreamWithDictionary.cs
- FloaterParagraph.cs
- RequiredFieldValidator.cs
- BamlWriter.cs
- TranslateTransform.cs
- MsmqTransportElement.cs
- AggregateNode.cs
- DataPointer.cs
- AccessedThroughPropertyAttribute.cs
- LeafCellTreeNode.cs
- Quad.cs
- AutoSizeComboBox.cs
- ControlDesigner.cs
- TdsParserSessionPool.cs
- FormatPage.cs
- CellNormalizer.cs
- ButtonBase.cs
- UdpContractFilterBehavior.cs
- SigningDialog.cs
- TimeStampChecker.cs
- Comparer.cs
- PermissionRequestEvidence.cs
- FormsAuthenticationUser.cs
- CompilationLock.cs
- CheckBoxPopupAdapter.cs
- WebControlAdapter.cs
- EventItfInfo.cs
- BounceEase.cs
- CalendarDay.cs
- DelegatingConfigHost.cs
- DictionarySectionHandler.cs
- AssociatedControlConverter.cs
- Page.cs
- Errors.cs
- IOThreadScheduler.cs
- PageThemeBuildProvider.cs
- Utils.cs
- ScaleTransform.cs
- _ProxyChain.cs
- TextStore.cs
- VirtualizedItemPattern.cs
- AssemblySettingAttributes.cs
- DataContractSerializerElement.cs
- InternalEnumValidator.cs
- FocusManager.cs
- ActivationArguments.cs
- unsafenativemethodsother.cs
- StringResourceManager.cs
- SymDocumentType.cs
- SqlDataSourceCommandEventArgs.cs
- StorageMappingFragment.cs
- HttpCacheVary.cs
- HtmlInputImage.cs
- FaultReasonText.cs
- NullableConverter.cs
- XmlWriterTraceListener.cs