Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CompMod / System / ComponentModel / Design / PropertyTabAttribute.cs / 1305376 / PropertyTabAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- // SECREVIEW: Remove this attribute once bug#411903 is fixed. [assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2113:SecureLateBindingMethods", Scope="member", Target="System.ComponentModel.PropertyTabAttribute.get_TabClasses():System.Type[]")] namespace System.ComponentModel { using System; using System.ComponentModel; using System.Reflection; using System.Security.Permissions; ////// [AttributeUsage(AttributeTargets.All)] public class PropertyTabAttribute : Attribute { private PropertyTabScope[] tabScopes; private Type[] tabClasses; private string[] tabClassNames; ///Identifies the property tab or tabs that should be displayed for the /// specified class or classes. ////// public PropertyTabAttribute() { tabScopes = new PropertyTabScope[0]; tabClassNames = new string[0]; } ////// Basic constructor that creates a PropertyTabAttribute. Use this ctor to derive from this /// attribute and specify multiple tab types by calling InitializeArrays. /// ////// public PropertyTabAttribute(Type tabClass) : this(tabClass, PropertyTabScope.Component) { } ////// Basic constructor that creates a property tab attribute that will create a tab /// of the specified type. /// ////// public PropertyTabAttribute(string tabClassName) : this(tabClassName, PropertyTabScope.Component) { } ////// Basic constructor that creates a property tab attribute that will create a tab /// of the specified type. /// ////// public PropertyTabAttribute(Type tabClass, PropertyTabScope tabScope) { this.tabClasses = new Type[]{ tabClass}; if (tabScope < PropertyTabScope.Document) { throw new ArgumentException(SR.GetString(SR.PropertyTabAttributeBadPropertyTabScope), "tabScope"); } this.tabScopes = new PropertyTabScope[]{tabScope}; } ////// Basic constructor that creates a property tab attribute that will create a tab /// of the specified type. /// ////// public PropertyTabAttribute(string tabClassName, PropertyTabScope tabScope) { this.tabClassNames = new string[]{ tabClassName}; if (tabScope < PropertyTabScope.Document) { throw new ArgumentException(SR.GetString(SR.PropertyTabAttributeBadPropertyTabScope), "tabScope"); } this.tabScopes = new PropertyTabScope[]{tabScope}; } ////// Basic constructor that creates a property tab attribute that will create a tab /// of the specified type. /// ////// public Type[] TabClasses { get { if (tabClasses == null && tabClassNames != null) { tabClasses = new Type[tabClassNames.Length]; for (int i=0; iGets the types of tab that this attribute specifies. ////// [To be supplied.] /// protected string[] TabClassNames{ get { if (tabClassNames != null) { return (string[])tabClassNames.Clone(); } else { return null; } } } ////// public PropertyTabScope[] TabScopes { get { return tabScopes; } } ///Gets the scopes of tabs for this System.ComponentModel.Design.PropertyTabAttribute, from System.ComponentModel.Design.PropertyTabScope. ///public override bool Equals(object other) { if (other is PropertyTabAttribute) { return Equals((PropertyTabAttribute)other); } return false; } /// public bool Equals(PropertyTabAttribute other) { if (other == (object)this) { return true; } if (other.TabClasses.Length != TabClasses.Length || other.TabScopes.Length != TabScopes.Length) { return false; } for (int i = 0; i < TabClasses.Length; i++) { if (TabClasses[i] != other.TabClasses[i] || TabScopes[i] != other.TabScopes[i]) { return false; } } return true; } /// /// public override int GetHashCode() { return base.GetHashCode(); } ////// Returns the hashcode for this object. /// ////// protected void InitializeArrays(string[] tabClassNames, PropertyTabScope[] tabScopes) { InitializeArrays(tabClassNames, null, tabScopes); } ////// Utiliity function to set the types of tab classes this PropertyTabAttribute specifies. /// ////// protected void InitializeArrays(Type[] tabClasses, PropertyTabScope[] tabScopes) { InitializeArrays(null, tabClasses, tabScopes); } private void InitializeArrays(string[] tabClassNames, Type[] tabClasses, PropertyTabScope[] tabScopes) { if (tabClasses != null) { if (tabScopes != null && tabClasses.Length != tabScopes.Length) { throw new ArgumentException(SR.GetString(SR.PropertyTabAttributeArrayLengthMismatch)); } this.tabClasses = (Type[])tabClasses.Clone(); } else if (tabClassNames != null) { if (tabScopes != null && tabClasses.Length != tabScopes.Length) { throw new ArgumentException(SR.GetString(SR.PropertyTabAttributeArrayLengthMismatch)); } this.tabClassNames = (string[])tabClassNames.Clone(); this.tabClasses = null; } else if (this.tabClasses == null && this.tabClassNames == null) { throw new ArgumentException(SR.GetString(SR.PropertyTabAttributeParamsBothNull)); } if (tabScopes != null) { for (int i = 0; i < tabScopes.Length; i++) { if (tabScopes[i] < PropertyTabScope.Document) { throw new ArgumentException(SR.GetString(SR.PropertyTabAttributeBadPropertyTabScope)); } } this.tabScopes = (PropertyTabScope[])tabScopes.Clone(); } else { this.tabScopes = new PropertyTabScope[tabClasses.Length]; for (int i = 0; i < TabScopes.Length; i++) { this.tabScopes[i] = PropertyTabScope.Component; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Utiliity function to set the types of tab classes this PropertyTabAttribute specifies. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- // SECREVIEW: Remove this attribute once bug#411903 is fixed. [assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2113:SecureLateBindingMethods", Scope="member", Target="System.ComponentModel.PropertyTabAttribute.get_TabClasses():System.Type[]")] namespace System.ComponentModel { using System; using System.ComponentModel; using System.Reflection; using System.Security.Permissions; ////// [AttributeUsage(AttributeTargets.All)] public class PropertyTabAttribute : Attribute { private PropertyTabScope[] tabScopes; private Type[] tabClasses; private string[] tabClassNames; ///Identifies the property tab or tabs that should be displayed for the /// specified class or classes. ////// public PropertyTabAttribute() { tabScopes = new PropertyTabScope[0]; tabClassNames = new string[0]; } ////// Basic constructor that creates a PropertyTabAttribute. Use this ctor to derive from this /// attribute and specify multiple tab types by calling InitializeArrays. /// ////// public PropertyTabAttribute(Type tabClass) : this(tabClass, PropertyTabScope.Component) { } ////// Basic constructor that creates a property tab attribute that will create a tab /// of the specified type. /// ////// public PropertyTabAttribute(string tabClassName) : this(tabClassName, PropertyTabScope.Component) { } ////// Basic constructor that creates a property tab attribute that will create a tab /// of the specified type. /// ////// public PropertyTabAttribute(Type tabClass, PropertyTabScope tabScope) { this.tabClasses = new Type[]{ tabClass}; if (tabScope < PropertyTabScope.Document) { throw new ArgumentException(SR.GetString(SR.PropertyTabAttributeBadPropertyTabScope), "tabScope"); } this.tabScopes = new PropertyTabScope[]{tabScope}; } ////// Basic constructor that creates a property tab attribute that will create a tab /// of the specified type. /// ////// public PropertyTabAttribute(string tabClassName, PropertyTabScope tabScope) { this.tabClassNames = new string[]{ tabClassName}; if (tabScope < PropertyTabScope.Document) { throw new ArgumentException(SR.GetString(SR.PropertyTabAttributeBadPropertyTabScope), "tabScope"); } this.tabScopes = new PropertyTabScope[]{tabScope}; } ////// Basic constructor that creates a property tab attribute that will create a tab /// of the specified type. /// ////// public Type[] TabClasses { get { if (tabClasses == null && tabClassNames != null) { tabClasses = new Type[tabClassNames.Length]; for (int i=0; iGets the types of tab that this attribute specifies. ////// [To be supplied.] /// protected string[] TabClassNames{ get { if (tabClassNames != null) { return (string[])tabClassNames.Clone(); } else { return null; } } } ////// public PropertyTabScope[] TabScopes { get { return tabScopes; } } ///Gets the scopes of tabs for this System.ComponentModel.Design.PropertyTabAttribute, from System.ComponentModel.Design.PropertyTabScope. ///public override bool Equals(object other) { if (other is PropertyTabAttribute) { return Equals((PropertyTabAttribute)other); } return false; } /// public bool Equals(PropertyTabAttribute other) { if (other == (object)this) { return true; } if (other.TabClasses.Length != TabClasses.Length || other.TabScopes.Length != TabScopes.Length) { return false; } for (int i = 0; i < TabClasses.Length; i++) { if (TabClasses[i] != other.TabClasses[i] || TabScopes[i] != other.TabScopes[i]) { return false; } } return true; } /// /// public override int GetHashCode() { return base.GetHashCode(); } ////// Returns the hashcode for this object. /// ////// protected void InitializeArrays(string[] tabClassNames, PropertyTabScope[] tabScopes) { InitializeArrays(tabClassNames, null, tabScopes); } ////// Utiliity function to set the types of tab classes this PropertyTabAttribute specifies. /// ////// protected void InitializeArrays(Type[] tabClasses, PropertyTabScope[] tabScopes) { InitializeArrays(null, tabClasses, tabScopes); } private void InitializeArrays(string[] tabClassNames, Type[] tabClasses, PropertyTabScope[] tabScopes) { if (tabClasses != null) { if (tabScopes != null && tabClasses.Length != tabScopes.Length) { throw new ArgumentException(SR.GetString(SR.PropertyTabAttributeArrayLengthMismatch)); } this.tabClasses = (Type[])tabClasses.Clone(); } else if (tabClassNames != null) { if (tabScopes != null && tabClasses.Length != tabScopes.Length) { throw new ArgumentException(SR.GetString(SR.PropertyTabAttributeArrayLengthMismatch)); } this.tabClassNames = (string[])tabClassNames.Clone(); this.tabClasses = null; } else if (this.tabClasses == null && this.tabClassNames == null) { throw new ArgumentException(SR.GetString(SR.PropertyTabAttributeParamsBothNull)); } if (tabScopes != null) { for (int i = 0; i < tabScopes.Length; i++) { if (tabScopes[i] < PropertyTabScope.Document) { throw new ArgumentException(SR.GetString(SR.PropertyTabAttributeBadPropertyTabScope)); } } this.tabScopes = (PropertyTabScope[])tabScopes.Clone(); } else { this.tabScopes = new PropertyTabScope[tabClasses.Length]; for (int i = 0; i < TabScopes.Length; i++) { this.tabScopes[i] = PropertyTabScope.Component; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Utiliity function to set the types of tab classes this PropertyTabAttribute specifies. /// ///
Link Menu
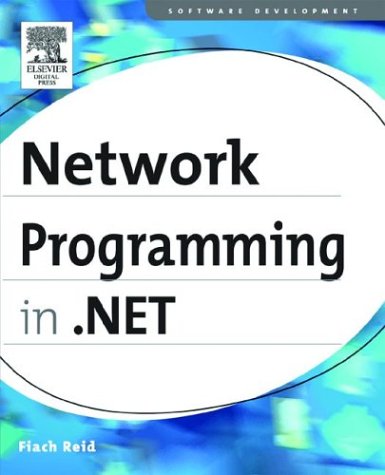
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _ConnectStream.cs
- CodeIdentifiers.cs
- WindowsEditBox.cs
- Splitter.cs
- UDPClient.cs
- ToolStripItemImageRenderEventArgs.cs
- ProfileModule.cs
- InitializationEventAttribute.cs
- DiscardableAttribute.cs
- DataRecordInfo.cs
- BuilderElements.cs
- EntityProviderFactory.cs
- BoolLiteral.cs
- CompilerCollection.cs
- FontStyle.cs
- UnsafeNativeMethods.cs
- DbConnectionPool.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- WarningException.cs
- EndpointInfoCollection.cs
- ConfigViewGenerator.cs
- RegexParser.cs
- SaveFileDialog.cs
- DataServiceSaveChangesEventArgs.cs
- BinHexEncoder.cs
- Attributes.cs
- UrlAuthFailedErrorFormatter.cs
- ObjectDataSourceDisposingEventArgs.cs
- PropertyDescriptorCollection.cs
- OLEDB_Enum.cs
- NumericUpDownAccelerationCollection.cs
- TextTreeInsertElementUndoUnit.cs
- SrgsGrammarCompiler.cs
- CompiledAction.cs
- XamlClipboardData.cs
- CacheOutputQuery.cs
- SafeRegistryHandle.cs
- InheritanceAttribute.cs
- TraceLevelStore.cs
- ResourceDisplayNameAttribute.cs
- Page.cs
- BaseDataList.cs
- DbgUtil.cs
- PropertyCondition.cs
- OverrideMode.cs
- VisualStyleRenderer.cs
- XmlSerializationReader.cs
- AutoCompleteStringCollection.cs
- IntPtr.cs
- HtmlMeta.cs
- PointCollection.cs
- recordstate.cs
- RequestStatusBarUpdateEventArgs.cs
- Tablet.cs
- SerializableAttribute.cs
- FileLevelControlBuilderAttribute.cs
- DataRow.cs
- BatchServiceHost.cs
- CorrelationManager.cs
- SecurityValidationBehavior.cs
- DataQuery.cs
- IconConverter.cs
- PeerCollaboration.cs
- XPathDocument.cs
- IndependentAnimationStorage.cs
- CollectionsUtil.cs
- RootDesignerSerializerAttribute.cs
- DataSourceExpressionCollection.cs
- IntSecurity.cs
- HijriCalendar.cs
- TypedDatasetGenerator.cs
- TextPatternIdentifiers.cs
- FilterableAttribute.cs
- UpDownBase.cs
- XsltSettings.cs
- FactoryRecord.cs
- TouchEventArgs.cs
- MouseGestureConverter.cs
- ArgIterator.cs
- PictureBox.cs
- NativeMethodsCLR.cs
- BoundPropertyEntry.cs
- SelectionProcessor.cs
- SspiNegotiationTokenAuthenticator.cs
- ToolStripContainer.cs
- ClrProviderManifest.cs
- MembershipPasswordException.cs
- SqlProfileProvider.cs
- _ConnectionGroup.cs
- OdbcTransaction.cs
- ToolStripSeparator.cs
- ConfigurationConverterBase.cs
- ProcessHostMapPath.cs
- Literal.cs
- SmtpMail.cs
- InvokeMethod.cs
- FamilyTypeface.cs
- ApplicationHost.cs
- GetPageCompletedEventArgs.cs
- EventListener.cs