Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / DataRow.cs / 1 / DataRow.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections; using System.Collections.Specialized; using System.Collections.Generic; using System.IO; using System.Runtime.InteropServices; using System.Threading; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary // Class represents the portable form of a Data Row in the store. // // Remarks // The data is NOT encrypted with in this form. When done working // with this object, it is recommended that you destroy it, or at least // clear out the raw buffer. // internal class DataRow { string m_instanceId; string m_sourceId; Int32 m_localId; Int32 m_objectType; Int64 m_lastChange; Int32 m_objectSize; GlobalId m_globalId; byte[] m_buffer; DataRowIndexBuffer m_indexBuffer; public DataRow() : this( null, null ) { } private DataRow( string instanceId, string sourceId ) { m_instanceId = instanceId; m_sourceId = sourceId; m_indexBuffer = new DataRowIndexBuffer(); } // // Summary: // Gets/Sets the GlobalId for a given Row. // // Remarks: // When seting the GlobalId, the IndexObject will // automatically be created and added to the list // of values to be persisted to index. // public GlobalId GlobalId { get{ return m_globalId; } set { if( GlobalId.Empty == value ) throw IDT.ThrowHelperArgumentNull( "value" ); m_globalId = value; SetIndexValue( SecondaryIndexDefinition.GlobalIdIndex, value ); } } // // Summary: // Gets the name of the Instance that owns the DataSource. // // Remarks: // This should typically be the String version of the SID the // in normal runtime situation in the service. In the tools, // this can be any unique string. It is only used at runtime to // ensure that one data object does not get saved to another users // data file. // // This value should be NULL if the object has never been saved. // public string InstanceId { get{ return m_instanceId; } internal set{ m_instanceId = value; } } // // Summary: // Gets the string of the DataSource that owns this object. // // Remarks: // This should be the Full path of the file name of the file. // This string is not used as a file path, but this string is // a good method of ensuring a unique source id per file. // // This value should be NULL if the object has never been saved. // public string SourceId { get{ return m_sourceId; } internal set{ m_sourceId = value; } } // // Summary: // Gets the LastChange Ticks of the DataRow. // // Remarks: // This indicates the last time this object was modified. // This value can be used in the CTOR of a DateTime Struct. // public Int64 LastChange { get{ return m_lastChange; } internal set{ m_lastChange = value; } } // // Summary: // Gets the LocalId of the object. // // Remarks: // This value uniquely identifies this object in the owning // data source. It allows for fast lookups, reads, and writes // to that data source. // public Int32 LocalId { get{ return m_localId; } internal set{ m_localId = value; } } // // Summary: // Get/Sets the type of object this datarow represents. // // Remarks: // This value should be <= 0 if the row has never been saved. // When this value is Set, it will automatically update the // IndexObject associated with this row. // // // ISSUE - 2005/01/13 - [....] // Might be better to have this as an enum value of StorableObjectType, or // atleast do a check // public Int32 ObjectType { get{ return m_objectType; } set { m_objectType = value; SetIndexValue( SecondaryIndexDefinition.ObjectTypeIndex, value ); } } internal DataRowIndexBuffer IndexBuffer { get { return m_indexBuffer; } } // // Summary: // Sets the internal data buffer to be used when saving. // // Remarks: // When passing data to this method, this object does NOT // make a copy, but assumes ownership over the buffer provided. // // Paramters: // buffer: A pointer the the buffer you would like to use // as the body of the object. // public void SetDataField( byte[] buffer ) { SetDataField( buffer,buffer.Length ); } // // Summary: // Sets the internal data buffer to be used when saving. // // Remarks: // When passing data to this method, this object does NOT // make a copy, but assumes ownership over the buffer provided. // // Paramters: // buffer: A pointer the the buffer you would like to use // as the body of the object. // size: The lenght of the buffer that is used. // public void SetDataField( byte[] buffer, int size ) { m_buffer = buffer; m_objectSize = size; } // // Summary: // Gets the internal buffer that contains the body of the data row. // // Remarks: // You SHOULD NOT modify the value of this return, unless you save the // row again. // // Returns: pointer to the internal buffer for the body of the row. // // public byte[] GetDataField( ) { return m_buffer; } // // Summary: // Sets All of the values for a multivalued index in one statement. // // Remarks: // Use for multivalued indexes, where you have several entries you written // to the index. // This will clear any existing values stored for the named index. // // Paramters: // name: The name of the index to set the valeus for. // multiValues: An array of objects arrays. The outer array maps to the // number of entries you want in the index, and each inner // array maps to the values you want in the index entry. // public void SetMultiIndexValue( string name, params object[][] multiValues ) { IndexObject[] objects = new IndexObject[ multiValues.Length ]; for( int i = 0; i < multiValues.Length; i++ ) { objects[ i ] = new IndexObject( multiValues[ i ] ); } m_indexBuffer.SetIndexValues( name, objects ); } // // Summary: // Sets all of the values for a single valued index in one statement. // Remarks: // // Paramters: // name: The name of the index to set the valeus for. // values: List of all the values you want to have entries in the // named index. // // Remarks: // Make sure that for value types, the second input is an array of objects instead // of the basic value type // public void SetIndexValue( string name, params object[] values ) { object[][] valueList = new object[ values.Length ][]; for( int i=0; iLastChange; row.ObjectType = pHeader->ObjectType; row.LocalId = pHeader->LocalId; row.GlobalId = pHeader->GlobalId; row.m_objectSize = pHeader->DataSize; // // The data field must set set independantly after // the data is decrypted. // return row; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
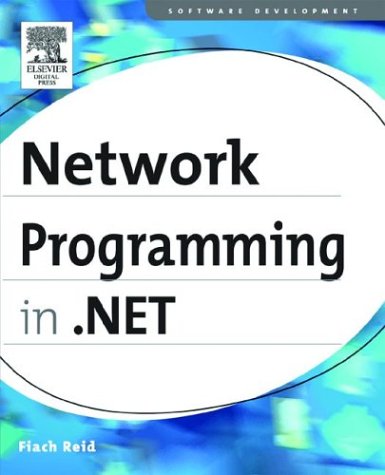
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SingleResultAttribute.cs
- HelpHtmlBuilder.cs
- Table.cs
- BackEase.cs
- WebPartConnectionsConnectVerb.cs
- TypeBuilder.cs
- ResourceContainer.cs
- ClrPerspective.cs
- DataRowComparer.cs
- PeerApplication.cs
- TemplateNodeContextMenu.cs
- StickyNoteHelper.cs
- ProcessHostMapPath.cs
- OdbcUtils.cs
- BaseHashHelper.cs
- Menu.cs
- DataGridViewRowHeaderCell.cs
- NewItemsContextMenuStrip.cs
- SortDescription.cs
- ManagedCodeMarkers.cs
- GetIndexBinder.cs
- SystemWebCachingSectionGroup.cs
- HttpCachePolicyBase.cs
- XhtmlBasicPhoneCallAdapter.cs
- NativeMethods.cs
- BufferedGraphics.cs
- OletxEnlistment.cs
- FigureParagraph.cs
- ConditionalWeakTable.cs
- OciEnlistContext.cs
- UnsafeNativeMethods.cs
- TreeView.cs
- StyleSheet.cs
- Font.cs
- DataGridAutomationPeer.cs
- SchemaType.cs
- StringValidator.cs
- MultiPropertyDescriptorGridEntry.cs
- NaturalLanguageHyphenator.cs
- XmlSerializerOperationFormatter.cs
- WebPartDisplayModeCancelEventArgs.cs
- AncillaryOps.cs
- AtlasWeb.Designer.cs
- XamlFilter.cs
- SqlError.cs
- NameValueCache.cs
- DataSourceControlBuilder.cs
- RawStylusSystemGestureInputReport.cs
- Expression.DebuggerProxy.cs
- CFStream.cs
- Thumb.cs
- MessagePropertyAttribute.cs
- TableLayoutStyle.cs
- Point3DCollection.cs
- EdmMember.cs
- ManagementException.cs
- RegistrationServices.cs
- DecoderBestFitFallback.cs
- TemplateBaseAction.cs
- SafeFileMappingHandle.cs
- EntityDescriptor.cs
- DataGridViewRowPostPaintEventArgs.cs
- CodeTypeDelegate.cs
- ClockController.cs
- LogicalExpressionEditor.cs
- SQLDateTimeStorage.cs
- LinqDataSourceHelper.cs
- DesignerUtility.cs
- SystemNetworkInterface.cs
- MarkupCompiler.cs
- GridViewItemAutomationPeer.cs
- FontStretch.cs
- ReadWriteControlDesigner.cs
- AstTree.cs
- DataGridTextColumn.cs
- BamlRecords.cs
- CheckBox.cs
- DrawingContextWalker.cs
- Properties.cs
- TagPrefixInfo.cs
- WindowsTab.cs
- ActionItem.cs
- SqlRowUpdatingEvent.cs
- StrokeNodeOperations2.cs
- SystemTcpConnection.cs
- FormsAuthenticationTicket.cs
- PropertyDescriptor.cs
- UriSectionReader.cs
- parserscommon.cs
- XmlExpressionDumper.cs
- HtmlInputSubmit.cs
- StorageComplexTypeMapping.cs
- XmlBindingWorker.cs
- RouteParametersHelper.cs
- ListView.cs
- ListBoxItemAutomationPeer.cs
- ListManagerBindingsCollection.cs
- DataGridViewColumnEventArgs.cs
- Triangle.cs
- ExtractedStateEntry.cs