Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / Configuration / TagPrefixInfo.cs / 2 / TagPrefixInfo.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.IO; using System.Text; using System.Web.Util; using System.Web.UI; using System.Web.Compilation; using System.Threading; using System.Web.Configuration; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class TagPrefixInfo : ConfigurationElement { private static readonly ConfigurationElementProperty s_elemProperty = new ConfigurationElementProperty(new CallbackValidator(typeof(TagPrefixInfo), Validate)); private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propTagPrefix = new ConfigurationProperty("tagPrefix", typeof(string), "/", null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired); private static readonly ConfigurationProperty _propTagName = new ConfigurationProperty("tagName", typeof(string), String.Empty, null, null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propNamespace = new ConfigurationProperty("namespace", typeof(string), String.Empty, null, null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propAssembly = new ConfigurationProperty("assembly", typeof(string), String.Empty, null, null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propSource = new ConfigurationProperty("src", typeof(string), String.Empty, null, null, ConfigurationPropertyOptions.None); static TagPrefixInfo() { _properties = new ConfigurationPropertyCollection(); _properties.Add(_propTagPrefix); _properties.Add(_propTagName); _properties.Add(_propNamespace); _properties.Add(_propAssembly); _properties.Add(_propSource); } internal TagPrefixInfo() { } public TagPrefixInfo(String tagPrefix, String nameSpace, String assembly, String tagName, String source) : this() { TagPrefix = tagPrefix; Namespace = nameSpace; Assembly = assembly; TagName = tagName; Source = source; } public override bool Equals(object prefix) { TagPrefixInfo ns = prefix as TagPrefixInfo; return StringUtil.Equals(TagPrefix, ns.TagPrefix) && StringUtil.Equals(TagName, ns.TagName) && StringUtil.Equals(Namespace, ns.Namespace) && StringUtil.Equals(Assembly, ns.Assembly) && StringUtil.Equals(Source, ns.Source); } public override int GetHashCode() { return TagPrefix.GetHashCode() ^ TagName.GetHashCode() ^ Namespace.GetHashCode() ^ Assembly.GetHashCode() ^ Source.GetHashCode(); } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("tagPrefix", IsRequired = true, DefaultValue = "/")] [StringValidator(MinLength = 1)] public string TagPrefix { get { return (string)base[_propTagPrefix]; } set { base[_propTagPrefix] = value; } } [ConfigurationProperty("tagName")] public string TagName { get { return (string)base[_propTagName]; } set { base[_propTagName] = value; } } [ConfigurationProperty("namespace")] public string Namespace { get { return (string)base[_propNamespace]; } set { base[_propNamespace] = value; } } [ConfigurationProperty("assembly")] public string Assembly { get { return (string)base[_propAssembly]; } set { base[_propAssembly] = value; } } [ConfigurationProperty("src")] public string Source { get { return (string)base[_propSource]; } set { if (!String.IsNullOrEmpty(value)) { base[_propSource] = value; } else { base[_propSource] = null; } } } protected override ConfigurationElementProperty ElementProperty { get { return s_elemProperty; } } private static void Validate(object value) { if (value == null) { throw new ArgumentNullException("control"); } TagPrefixInfo elem = (TagPrefixInfo)value; if (System.Web.UI.Util.ContainsWhiteSpace(elem.TagPrefix)) { throw new ConfigurationErrorsException( SR.GetString(SR.Space_attribute, "tagPrefix")); } bool invalid = false; if (!String.IsNullOrEmpty(elem.Namespace)) { // It is a custom control if (!(String.IsNullOrEmpty(elem.TagName) && String.IsNullOrEmpty(elem.Source))) { invalid = true; } } else if (!String.IsNullOrEmpty(elem.TagName)) { // It is a user control if (!(String.IsNullOrEmpty(elem.Namespace) && String.IsNullOrEmpty(elem.Assembly) && !String.IsNullOrEmpty(elem.Source))) { invalid = true; } } else { invalid = true; } if (invalid) { throw new ConfigurationErrorsException( SR.GetString(SR.Invalid_tagprefix_entry)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.IO; using System.Text; using System.Web.Util; using System.Web.UI; using System.Web.Compilation; using System.Threading; using System.Web.Configuration; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class TagPrefixInfo : ConfigurationElement { private static readonly ConfigurationElementProperty s_elemProperty = new ConfigurationElementProperty(new CallbackValidator(typeof(TagPrefixInfo), Validate)); private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propTagPrefix = new ConfigurationProperty("tagPrefix", typeof(string), "/", null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired); private static readonly ConfigurationProperty _propTagName = new ConfigurationProperty("tagName", typeof(string), String.Empty, null, null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propNamespace = new ConfigurationProperty("namespace", typeof(string), String.Empty, null, null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propAssembly = new ConfigurationProperty("assembly", typeof(string), String.Empty, null, null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propSource = new ConfigurationProperty("src", typeof(string), String.Empty, null, null, ConfigurationPropertyOptions.None); static TagPrefixInfo() { _properties = new ConfigurationPropertyCollection(); _properties.Add(_propTagPrefix); _properties.Add(_propTagName); _properties.Add(_propNamespace); _properties.Add(_propAssembly); _properties.Add(_propSource); } internal TagPrefixInfo() { } public TagPrefixInfo(String tagPrefix, String nameSpace, String assembly, String tagName, String source) : this() { TagPrefix = tagPrefix; Namespace = nameSpace; Assembly = assembly; TagName = tagName; Source = source; } public override bool Equals(object prefix) { TagPrefixInfo ns = prefix as TagPrefixInfo; return StringUtil.Equals(TagPrefix, ns.TagPrefix) && StringUtil.Equals(TagName, ns.TagName) && StringUtil.Equals(Namespace, ns.Namespace) && StringUtil.Equals(Assembly, ns.Assembly) && StringUtil.Equals(Source, ns.Source); } public override int GetHashCode() { return TagPrefix.GetHashCode() ^ TagName.GetHashCode() ^ Namespace.GetHashCode() ^ Assembly.GetHashCode() ^ Source.GetHashCode(); } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("tagPrefix", IsRequired = true, DefaultValue = "/")] [StringValidator(MinLength = 1)] public string TagPrefix { get { return (string)base[_propTagPrefix]; } set { base[_propTagPrefix] = value; } } [ConfigurationProperty("tagName")] public string TagName { get { return (string)base[_propTagName]; } set { base[_propTagName] = value; } } [ConfigurationProperty("namespace")] public string Namespace { get { return (string)base[_propNamespace]; } set { base[_propNamespace] = value; } } [ConfigurationProperty("assembly")] public string Assembly { get { return (string)base[_propAssembly]; } set { base[_propAssembly] = value; } } [ConfigurationProperty("src")] public string Source { get { return (string)base[_propSource]; } set { if (!String.IsNullOrEmpty(value)) { base[_propSource] = value; } else { base[_propSource] = null; } } } protected override ConfigurationElementProperty ElementProperty { get { return s_elemProperty; } } private static void Validate(object value) { if (value == null) { throw new ArgumentNullException("control"); } TagPrefixInfo elem = (TagPrefixInfo)value; if (System.Web.UI.Util.ContainsWhiteSpace(elem.TagPrefix)) { throw new ConfigurationErrorsException( SR.GetString(SR.Space_attribute, "tagPrefix")); } bool invalid = false; if (!String.IsNullOrEmpty(elem.Namespace)) { // It is a custom control if (!(String.IsNullOrEmpty(elem.TagName) && String.IsNullOrEmpty(elem.Source))) { invalid = true; } } else if (!String.IsNullOrEmpty(elem.TagName)) { // It is a user control if (!(String.IsNullOrEmpty(elem.Namespace) && String.IsNullOrEmpty(elem.Assembly) && !String.IsNullOrEmpty(elem.Source))) { invalid = true; } } else { invalid = true; } if (invalid) { throw new ConfigurationErrorsException( SR.GetString(SR.Invalid_tagprefix_entry)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
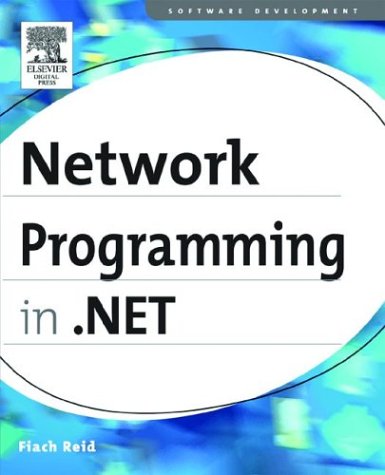
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NonSerializedAttribute.cs
- WebPartMenuStyle.cs
- TranslateTransform.cs
- CssTextWriter.cs
- ObjectConverter.cs
- oledbconnectionstring.cs
- EntityDataSourceChangingEventArgs.cs
- HatchBrush.cs
- CodePropertyReferenceExpression.cs
- SqlTypesSchemaImporter.cs
- ObjectDataSource.cs
- ClientBuildManager.cs
- ConnectionPoint.cs
- Input.cs
- ServicePointManagerElement.cs
- DispatcherHookEventArgs.cs
- NativeMethods.cs
- EarlyBoundInfo.cs
- DataRowView.cs
- UnsafeNativeMethods.cs
- PkcsUtils.cs
- ToolZone.cs
- HtmlToClrEventProxy.cs
- TransportSecurityBindingElement.cs
- InvalidComObjectException.cs
- TreeNode.cs
- Effect.cs
- SharedConnectionWorkflowTransactionService.cs
- ElementAction.cs
- storepermissionattribute.cs
- CodeNamespaceImportCollection.cs
- ImageBrush.cs
- TypedRowGenerator.cs
- DataControlButton.cs
- ColumnTypeConverter.cs
- ProfileBuildProvider.cs
- PublisherIdentityPermission.cs
- InvalidPropValue.cs
- ImportContext.cs
- Vector3DValueSerializer.cs
- baseshape.cs
- PersonalizationProviderHelper.cs
- XamlLoadErrorInfo.cs
- Processor.cs
- ECDsa.cs
- OutKeywords.cs
- Stacktrace.cs
- DataGridHeaderBorder.cs
- UdpChannelListener.cs
- UnsafeNativeMethods.cs
- OrderedDictionaryStateHelper.cs
- ADRole.cs
- SqlMultiplexer.cs
- DefaultIfEmptyQueryOperator.cs
- QilVisitor.cs
- ScopelessEnumAttribute.cs
- AnimationTimeline.cs
- ObjectSet.cs
- CodeLinePragma.cs
- ListViewUpdateEventArgs.cs
- OptimizedTemplateContent.cs
- DataColumnChangeEvent.cs
- DelegatedStream.cs
- InvokeGenerator.cs
- SetIndexBinder.cs
- OutArgumentConverter.cs
- ProviderManager.cs
- EventQueueState.cs
- ErrorLog.cs
- GC.cs
- System.Data.OracleClient_BID.cs
- HttpResponseHeader.cs
- BlurEffect.cs
- Page.cs
- EventHandlerList.cs
- MarginsConverter.cs
- DesignerActionList.cs
- PathStreamGeometryContext.cs
- ReadOnlyCollectionBase.cs
- PagesSection.cs
- EnumerableCollectionView.cs
- LOSFormatter.cs
- ListenUriMode.cs
- MultiplexingDispatchMessageFormatter.cs
- SqlFlattener.cs
- ParallelTimeline.cs
- LookupBindingPropertiesAttribute.cs
- BindableTemplateBuilder.cs
- RangeValidator.cs
- altserialization.cs
- TextSpanModifier.cs
- ContextMenu.cs
- CodeDomConfigurationHandler.cs
- Point3DCollection.cs
- SimpleHandlerBuildProvider.cs
- securestring.cs
- TextEmbeddedObject.cs
- PrefixQName.cs
- StorageEntityTypeMapping.cs
- ProxyWebPart.cs