Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx35 / System.ServiceModel.Web / System / ServiceModel / Dispatcher / MultiplexingDispatchMessageFormatter.cs / 1305376 / MultiplexingDispatchMessageFormatter.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System; using System.Collections.Generic; using System.Net.Mime; using System.Runtime; using System.ServiceModel; using System.ServiceModel.Channels; using System.ServiceModel.Description; using System.ServiceModel.Web; class MultiplexingDispatchMessageFormatter : IDispatchMessageFormatter { Dictionaryformatters; WebMessageFormat defaultFormat; Dictionary defaultContentTypes; public WebMessageFormat DefaultFormat { get { return this.defaultFormat; } } public Dictionary DefaultContentTypes { get { return this.defaultContentTypes; } } public MultiplexingDispatchMessageFormatter(Dictionary formatters, WebMessageFormat defaultFormat) { if (formatters == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("formatters"); } this.formatters = formatters; this.defaultFormat = defaultFormat; this.defaultContentTypes = new Dictionary (); Fx.Assert(this.formatters.ContainsKey(this.defaultFormat), "The default format should always be included in the dictionary of formatters."); } public void DeserializeRequest(Message message, object[] parameters) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException(SR2.GetString(SR2.SerializingRequestNotSupportedByFormatter, this))); } public Message SerializeReply(MessageVersion messageVersion, object[] parameters, object result) { OutgoingWebResponseContext outgoingResponse = WebOperationContext.Current.OutgoingResponse; WebMessageFormat? nullableFormat = outgoingResponse.Format; WebMessageFormat format = nullableFormat ?? this.defaultFormat; if (!this.formatters.ContainsKey(format)) { string operationName = " "; MessageProperties messageProperties = OperationContext.Current.IncomingMessageProperties; if (messageProperties.ContainsKey(WebHttpDispatchOperationSelector.HttpOperationNamePropertyName)) { operationName = messageProperties[WebHttpDispatchOperationSelector.HttpOperationNamePropertyName] as string; } throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException(SR2.GetString(SR2.OperationDoesNotSupportFormat, operationName, format.ToString()))); } if (string.IsNullOrEmpty(outgoingResponse.ContentType)) { string automatedSelectionContentType = outgoingResponse.AutomatedFormatSelectionContentType; if (!string.IsNullOrEmpty(automatedSelectionContentType)) { // Don't set the content-type if it is default xml for backwards compatiabilty if (!string.Equals(automatedSelectionContentType, defaultContentTypes[WebMessageFormat.Xml], StringComparison.OrdinalIgnoreCase)) { outgoingResponse.ContentType = automatedSelectionContentType; } } else { // Don't set the content-type if it is default xml for backwards compatiabilty if (format != WebMessageFormat.Xml) { outgoingResponse.ContentType = defaultContentTypes[format]; } } } Message message = this.formatters[format].SerializeReply(messageVersion, parameters, result); return message; } public bool SupportsMessageFormat(WebMessageFormat format) { return this.formatters.ContainsKey(format); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
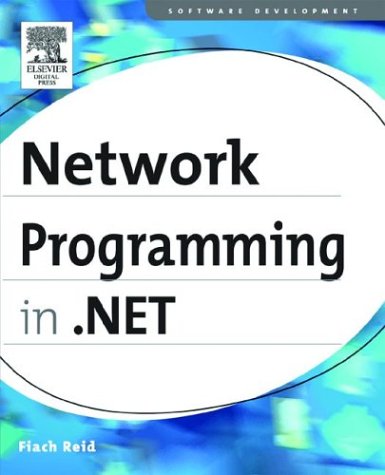
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MenuCommand.cs
- TextEditorContextMenu.cs
- ConfigurationValidatorBase.cs
- Function.cs
- NamespaceList.cs
- LayoutEditorPart.cs
- ClientProxyGenerator.cs
- LinkClickEvent.cs
- PropertyPath.cs
- AssemblyAttributes.cs
- graph.cs
- UIElementCollection.cs
- XmlSerializerFactory.cs
- SoapIgnoreAttribute.cs
- baseaxisquery.cs
- HierarchicalDataBoundControlAdapter.cs
- DebugControllerThread.cs
- NullableIntAverageAggregationOperator.cs
- LogicalCallContext.cs
- BaseResourcesBuildProvider.cs
- PersistChildrenAttribute.cs
- Memoizer.cs
- GifBitmapDecoder.cs
- XslException.cs
- COM2ComponentEditor.cs
- Win32Exception.cs
- SecureUICommand.cs
- HierarchicalDataTemplate.cs
- Documentation.cs
- UIElementParagraph.cs
- SchemaImporter.cs
- HtmlUtf8RawTextWriter.cs
- SchemaImporterExtension.cs
- NameValueSectionHandler.cs
- Sentence.cs
- GridViewAutoFormat.cs
- BooleanFunctions.cs
- RayHitTestParameters.cs
- TextServicesContext.cs
- RtfToken.cs
- BitmapEffectDrawing.cs
- ScrollChrome.cs
- TransformDescriptor.cs
- BreakRecordTable.cs
- ObjectConverter.cs
- ListViewPagedDataSource.cs
- XmlCharCheckingWriter.cs
- IntegerValidatorAttribute.cs
- BitmapData.cs
- validation.cs
- UseLicense.cs
- MailAddress.cs
- MyContact.cs
- ExceptionRoutedEventArgs.cs
- Statements.cs
- RtfNavigator.cs
- RepeatBehaviorConverter.cs
- Deflater.cs
- TypeGeneratedEventArgs.cs
- DoubleAnimationUsingKeyFrames.cs
- BezierSegment.cs
- FunctionImportElement.cs
- SafeSystemMetrics.cs
- SQLUtility.cs
- Configuration.cs
- Mappings.cs
- StreamReader.cs
- DataGridViewCellParsingEventArgs.cs
- PeerEndPoint.cs
- FontWeight.cs
- ObjectContextServiceProvider.cs
- TrackingProfile.cs
- _NtlmClient.cs
- PasswordBox.cs
- ViewBox.cs
- ValidationErrorCollection.cs
- DetailsViewDeleteEventArgs.cs
- MILUtilities.cs
- SiteMapHierarchicalDataSourceView.cs
- CommandField.cs
- VarRemapper.cs
- PeerNameRecord.cs
- XPathNodeList.cs
- BadImageFormatException.cs
- smtpconnection.cs
- WebPartZoneBase.cs
- ProcessModuleCollection.cs
- MarginCollapsingState.cs
- AccessText.cs
- StyleSheetDesigner.cs
- LinqDataView.cs
- ProfileGroupSettingsCollection.cs
- _Semaphore.cs
- BuilderElements.cs
- SslStreamSecurityElement.cs
- WebConfigurationHostFileChange.cs
- WebPartDisplayModeEventArgs.cs
- ProfilePropertySettings.cs
- ValidationHelpers.cs
- CacheDependency.cs