Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Configuration / System / Configuration / StringValidator.cs / 1305376 / StringValidator.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.IO; using System.Reflection; using System.Security.Permissions; using System.Xml; using System.Collections.Specialized; using System.Globalization; using System.ComponentModel; using System.Security; using System.Text; using System.Text.RegularExpressions; namespace System.Configuration { public class StringValidator : ConfigurationValidatorBase { private int _minLength; private int _maxLength; private string _invalidChars; public StringValidator(int minLength) : this(minLength, int.MaxValue, null) { } public StringValidator(int minLength, int maxLength) : this(minLength, maxLength, null) { } public StringValidator(int minLength, int maxLength, string invalidCharacters) { _minLength = minLength; _maxLength = maxLength; _invalidChars = invalidCharacters; } public override bool CanValidate(Type type) { return (type == typeof(string)); } public override void Validate(object value) { ValidatorUtils.HelperParamValidation(value, typeof(string)); string data = value as string; int len = (data == null ? 0 : data.Length); if (len < _minLength) { throw new ArgumentException(SR.GetString(SR.Validator_string_min_length, _minLength)); } if (len > _maxLength) { throw new ArgumentException(SR.GetString(SR.Validator_string_max_length, _maxLength)); } // Check if the string contains any invalid characters if ((len > 0) && (_invalidChars != null) && (_invalidChars.Length > 0)) { char[] array = new char[_invalidChars.Length]; _invalidChars.CopyTo(0, array, 0, _invalidChars.Length); if (data.IndexOfAny(array) != -1) { throw new ArgumentException(SR.GetString(SR.Validator_string_invalid_chars, _invalidChars)); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.IO; using System.Reflection; using System.Security.Permissions; using System.Xml; using System.Collections.Specialized; using System.Globalization; using System.ComponentModel; using System.Security; using System.Text; using System.Text.RegularExpressions; namespace System.Configuration { public class StringValidator : ConfigurationValidatorBase { private int _minLength; private int _maxLength; private string _invalidChars; public StringValidator(int minLength) : this(minLength, int.MaxValue, null) { } public StringValidator(int minLength, int maxLength) : this(minLength, maxLength, null) { } public StringValidator(int minLength, int maxLength, string invalidCharacters) { _minLength = minLength; _maxLength = maxLength; _invalidChars = invalidCharacters; } public override bool CanValidate(Type type) { return (type == typeof(string)); } public override void Validate(object value) { ValidatorUtils.HelperParamValidation(value, typeof(string)); string data = value as string; int len = (data == null ? 0 : data.Length); if (len < _minLength) { throw new ArgumentException(SR.GetString(SR.Validator_string_min_length, _minLength)); } if (len > _maxLength) { throw new ArgumentException(SR.GetString(SR.Validator_string_max_length, _maxLength)); } // Check if the string contains any invalid characters if ((len > 0) && (_invalidChars != null) && (_invalidChars.Length > 0)) { char[] array = new char[_invalidChars.Length]; _invalidChars.CopyTo(0, array, 0, _invalidChars.Length); if (data.IndexOfAny(array) != -1) { throw new ArgumentException(SR.GetString(SR.Validator_string_invalid_chars, _invalidChars)); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
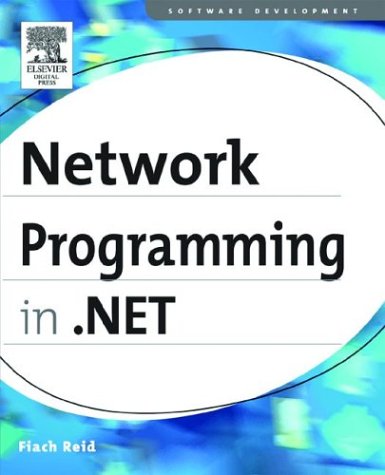
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InstanceKeyNotReadyException.cs
- MediaScriptCommandRoutedEventArgs.cs
- DataServiceHost.cs
- DataKey.cs
- GetPageCompletedEventArgs.cs
- ClientRuntime.cs
- Tool.cs
- RtfToken.cs
- CmsInterop.cs
- WebPartVerbsEventArgs.cs
- Point4D.cs
- _ChunkParse.cs
- DeferredBinaryDeserializerExtension.cs
- uribuilder.cs
- DbConnectionStringCommon.cs
- StrokeCollectionDefaultValueFactory.cs
- SchemaMerger.cs
- GetCardDetailsRequest.cs
- HtmlMobileTextWriter.cs
- XamlTypeMapper.cs
- FunctionImportMapping.cs
- AuthenticatingEventArgs.cs
- HebrewNumber.cs
- ImmutablePropertyDescriptorGridEntry.cs
- ListViewContainer.cs
- Graph.cs
- PageRouteHandler.cs
- PageThemeCodeDomTreeGenerator.cs
- Style.cs
- Nodes.cs
- IgnoreFileBuildProvider.cs
- XmlSignificantWhitespace.cs
- DetailsViewDeleteEventArgs.cs
- WindowsTitleBar.cs
- WindowsMenu.cs
- StreamUpgradeProvider.cs
- PerformanceCounterManager.cs
- CodeSnippetStatement.cs
- CalendarDateRangeChangingEventArgs.cs
- GroupItem.cs
- ModulesEntry.cs
- RotateTransform3D.cs
- ExecutedRoutedEventArgs.cs
- PrivateFontCollection.cs
- FacetValueContainer.cs
- FlowSwitchDesigner.xaml.cs
- CommentEmitter.cs
- CursorConverter.cs
- XmlLinkedNode.cs
- ImpersonateTokenRef.cs
- TCEAdapterGenerator.cs
- AmbientLight.cs
- ObfuscateAssemblyAttribute.cs
- WindowsSpinner.cs
- MembershipValidatePasswordEventArgs.cs
- DetailsViewCommandEventArgs.cs
- BatchWriter.cs
- PathNode.cs
- XmlCDATASection.cs
- ServiceThrottlingBehavior.cs
- ObjRef.cs
- NumericUpDown.cs
- FormsAuthenticationCredentials.cs
- ProfileEventArgs.cs
- OpenCollectionAsyncResult.cs
- LogicalExpr.cs
- RelationalExpressions.cs
- CheckPair.cs
- ExpressionPrefixAttribute.cs
- TrackingServices.cs
- InvalidProgramException.cs
- NTAccount.cs
- DmlSqlGenerator.cs
- MenuItemAutomationPeer.cs
- ObjectTokenCategory.cs
- ProbeMatches11.cs
- XmlUnspecifiedAttribute.cs
- SessionStateUtil.cs
- MemberProjectedSlot.cs
- SpellerInterop.cs
- TemplateControlParser.cs
- PresentationSource.cs
- RuntimeUtils.cs
- XmlSiteMapProvider.cs
- HtmlFormParameterWriter.cs
- DesignerTransaction.cs
- TaskFileService.cs
- DbConnectionFactory.cs
- TextTreeRootNode.cs
- BinaryObjectWriter.cs
- SoapAttributes.cs
- ProgressChangedEventArgs.cs
- DragEvent.cs
- MetricEntry.cs
- ListenDesigner.cs
- ProfessionalColorTable.cs
- RemoveStoryboard.cs
- SendMailErrorEventArgs.cs
- CommonGetThemePartSize.cs
- CodeAttributeArgumentCollection.cs