Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / ScrollBarRenderer.cs / 1 / ScrollBarRenderer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Windows.Forms.VisualStyles; using System.Diagnostics.CodeAnalysis; using Microsoft.Win32; ////// /// public sealed class ScrollBarRenderer { //Make this per-thread, so that different threads can safely use these methods. [ThreadStatic] private static VisualStyleRenderer visualStyleRenderer = null; //cannot instantiate private ScrollBarRenderer() { } ////// This is a rendering class for the ScrollBar control. /// ////// /// public static bool IsSupported { get { return VisualStyleRenderer.IsSupported; // no downlevel support } } ////// Returns true if this class is supported for the current OS and user/application settings, /// otherwise returns false. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawArrowButton(Graphics g, Rectangle bounds, ScrollBarArrowButtonState state) { InitializeRenderer(VisualStyleElement.ScrollBar.ArrowButton.LeftNormal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a ScrollBar arrow button. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawHorizontalThumb(Graphics g, Rectangle bounds, ScrollBarState state) { InitializeRenderer(VisualStyleElement.ScrollBar.ThumbButtonHorizontal.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a horizontal ScrollBar thumb. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawVerticalThumb(Graphics g, Rectangle bounds, ScrollBarState state) { InitializeRenderer(VisualStyleElement.ScrollBar.ThumbButtonVertical.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a vertical ScrollBar thumb. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawHorizontalThumbGrip(Graphics g, Rectangle bounds, ScrollBarState state) { InitializeRenderer(VisualStyleElement.ScrollBar.GripperHorizontal.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a horizontal ScrollBar thumb grip. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawVerticalThumbGrip(Graphics g, Rectangle bounds, ScrollBarState state) { InitializeRenderer(VisualStyleElement.ScrollBar.GripperVertical.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a vertical ScrollBar thumb grip. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawRightHorizontalTrack(Graphics g, Rectangle bounds, ScrollBarState state) { InitializeRenderer(VisualStyleElement.ScrollBar.RightTrackHorizontal.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a horizontal ScrollBar thumb. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawLeftHorizontalTrack(Graphics g, Rectangle bounds, ScrollBarState state) { InitializeRenderer(VisualStyleElement.ScrollBar.LeftTrackHorizontal.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a horizontal ScrollBar thumb. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawUpperVerticalTrack(Graphics g, Rectangle bounds, ScrollBarState state) { InitializeRenderer(VisualStyleElement.ScrollBar.UpperTrackVertical.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a vertical ScrollBar thumb in the center of the given bounds. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawLowerVerticalTrack(Graphics g, Rectangle bounds, ScrollBarState state) { InitializeRenderer(VisualStyleElement.ScrollBar.LowerTrackVertical.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a vertical ScrollBar thumb in the center of the given bounds. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawSizeBox(Graphics g, Rectangle bounds, ScrollBarSizeBoxState state) { InitializeRenderer(VisualStyleElement.ScrollBar.SizeBox.LeftAlign, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a ScrollBar size box in the center of the given bounds. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static Size GetThumbGripSize(Graphics g, ScrollBarState state) { InitializeRenderer(VisualStyleElement.ScrollBar.GripperHorizontal.Normal, (int)state); return visualStyleRenderer.GetPartSize(g, ThemeSizeType.True); } ////// Returns the size of the ScrollBar thumb grip. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static Size GetSizeBoxSize(Graphics g, ScrollBarState state) { InitializeRenderer(VisualStyleElement.ScrollBar.SizeBox.LeftAlign, (int)state); return visualStyleRenderer.GetPartSize(g, ThemeSizeType.True); } private static void InitializeRenderer(VisualStyleElement element, int state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(element.ClassName, element.Part, state); } else { visualStyleRenderer.SetParameters(element.ClassName, element.Part, state); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved./// Returns the size of the ScrollBar size box. /// ///
Link Menu
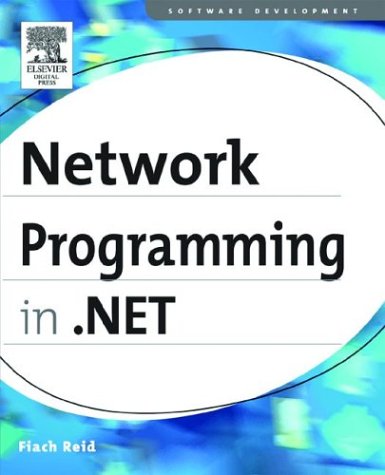
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Point.cs
- WebPartCancelEventArgs.cs
- HttpServerVarsCollection.cs
- TextShapeableCharacters.cs
- ShaperBuffers.cs
- HebrewNumber.cs
- TraceListener.cs
- ConstantSlot.cs
- SchemaName.cs
- EndpointDiscoveryMetadata11.cs
- RtfToXamlLexer.cs
- CustomErrorsSection.cs
- FreeFormDragDropManager.cs
- DelayedRegex.cs
- LinqDataSourceInsertEventArgs.cs
- TypeValidationEventArgs.cs
- TablePattern.cs
- DeviceContext.cs
- WindowsSlider.cs
- ServiceParser.cs
- ClientSession.cs
- ReflectEventDescriptor.cs
- NamedPermissionSet.cs
- MsmqInputSessionChannelListener.cs
- StorageSetMapping.cs
- VSWCFServiceContractGenerator.cs
- RelationalExpressions.cs
- JsonFaultDetail.cs
- UrlSyndicationContent.cs
- InstanceLockTracking.cs
- DataSpaceManager.cs
- NativeMethods.cs
- Size.cs
- HostElement.cs
- DocumentPageHost.cs
- EntityDataSourceContainerNameConverter.cs
- PipeSecurity.cs
- EventItfInfo.cs
- DataGrid.cs
- TransformGroup.cs
- SafeSecurityHandles.cs
- COM2ComponentEditor.cs
- DataGridViewRowEventArgs.cs
- DocComment.cs
- MinMaxParagraphWidth.cs
- XmlSerializationWriter.cs
- XmlSchemaSequence.cs
- ItemDragEvent.cs
- FileLevelControlBuilderAttribute.cs
- BitStream.cs
- XamlFilter.cs
- AdapterDictionary.cs
- NameValueCollection.cs
- ToolStripRenderEventArgs.cs
- TextHidden.cs
- RuntimeWrappedException.cs
- EntityCollection.cs
- RC2CryptoServiceProvider.cs
- WmlCalendarAdapter.cs
- BrowserDefinition.cs
- UrlMappingsSection.cs
- SiteMapHierarchicalDataSourceView.cs
- FunctionImportElement.cs
- HelpPage.cs
- Stylesheet.cs
- SoundPlayerAction.cs
- messageonlyhwndwrapper.cs
- EndpointDiscoveryBehavior.cs
- Focus.cs
- VisualTarget.cs
- XomlCompiler.cs
- TemplateControlBuildProvider.cs
- SettingsBase.cs
- Inline.cs
- TextEditorSelection.cs
- ProgramPublisher.cs
- SplineKeyFrames.cs
- StreamWriter.cs
- ChangeProcessor.cs
- ValidationPropertyAttribute.cs
- EntityDataSourceDataSelectionPanel.cs
- DesignerObject.cs
- DataGridLength.cs
- DynamicControl.cs
- TableLayoutPanel.cs
- ECDsa.cs
- XamlInterfaces.cs
- AttributeParameterInfo.cs
- PersonalizationProviderHelper.cs
- StronglyTypedResourceBuilder.cs
- DefinitionBase.cs
- FieldInfo.cs
- AttributeUsageAttribute.cs
- ViewManager.cs
- MD5CryptoServiceProvider.cs
- DictionaryContent.cs
- _LoggingObject.cs
- DataGridViewRowHeaderCell.cs
- ProcessHostFactoryHelper.cs
- ToolStripEditorManager.cs