Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Input / Stylus / StylusPointDescription.cs / 1 / StylusPointDescription.cs
//------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Input; using System.Windows.Media; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows.Input { ////// StylusPointDescription describes the properties that a StylusPoint supports. /// public class StylusPointDescription { ////// Internal statics for our magic numbers /// internal static readonly int RequiredCountOfProperties = 3; internal static readonly int RequiredXIndex = 0; internal static readonly int RequiredYIndex = 1; internal static readonly int RequiredPressureIndex = 2; internal static readonly int MaximumButtonCount = 31; private int _buttonCount = 0; private int _originalPressureIndex = RequiredPressureIndex; private StylusPointPropertyInfo[] _stylusPointPropertyInfos; ////// StylusPointDescription /// public StylusPointDescription() { //implement the default packet description _stylusPointPropertyInfos = new StylusPointPropertyInfo[] { StylusPointPropertyInfoDefaults.X, StylusPointPropertyInfoDefaults.Y, StylusPointPropertyInfoDefaults.NormalPressure }; } ////// StylusPointDescription /// public StylusPointDescription(IEnumerablestylusPointPropertyInfos) { if (null == stylusPointPropertyInfos) { throw new ArgumentNullException("stylusPointPropertyInfos"); } List infos = new List (stylusPointPropertyInfos); if (infos.Count < RequiredCountOfProperties || infos[RequiredXIndex].Id != StylusPointPropertyIds.X || infos[RequiredYIndex].Id != StylusPointPropertyIds.Y || infos[RequiredPressureIndex].Id != StylusPointPropertyIds.NormalPressure) { throw new ArgumentException(SR.Get(SRID.InvalidStylusPointDescription), "stylusPointPropertyInfos"); } // // look for duplicates, validate that buttons are last // List seenIds = new List (); seenIds.Add(StylusPointPropertyIds.X); seenIds.Add(StylusPointPropertyIds.Y); seenIds.Add(StylusPointPropertyIds.NormalPressure); int buttonCount = 0; for (int x = RequiredCountOfProperties; x < infos.Count; x++) { if (seenIds.Contains(infos[x].Id)) { throw new ArgumentException(SR.Get(SRID.InvalidStylusPointDescriptionDuplicatesFound), "stylusPointPropertyInfos"); } if (infos[x].IsButton) { buttonCount++; } else { //this is not a button, make sure we haven't seen one before if (buttonCount > 0) { throw new ArgumentException(SR.Get(SRID.InvalidStylusPointDescriptionButtonsMustBeLast), "stylusPointPropertyInfos"); } } seenIds.Add(infos[x].Id); } if (buttonCount > MaximumButtonCount) { throw new ArgumentException(SR.Get(SRID.InvalidStylusPointDescriptionTooManyButtons), "stylusPointPropertyInfos"); } _buttonCount = buttonCount; _stylusPointPropertyInfos = infos.ToArray(); } /// /// StylusPointDescription /// /// stylusPointPropertyInfos /// originalPressureIndex - does the digitizer really support pressure? If so, the index this was at internal StylusPointDescription(IEnumerablestylusPointPropertyInfos, int originalPressureIndex) : this (stylusPointPropertyInfos) { _originalPressureIndex = originalPressureIndex; } /// /// HasProperty /// /// stylusPointProperty public bool HasProperty(StylusPointProperty stylusPointProperty) { if (null == stylusPointProperty) { throw new ArgumentNullException("stylusPointProperty"); } int index = IndexOf(stylusPointProperty.Id); if (-1 == index) { return false; } return true; } ////// The count of properties this StylusPointDescription contains /// public int PropertyCount { get { return _stylusPointPropertyInfos.Length; } } ////// GetProperty /// /// stylusPointProperty public StylusPointPropertyInfo GetPropertyInfo(StylusPointProperty stylusPointProperty) { if (null == stylusPointProperty) { throw new ArgumentNullException("stylusPointProperty"); } return GetPropertyInfo(stylusPointProperty.Id); } ////// GetPropertyInfo /// /// guid internal StylusPointPropertyInfo GetPropertyInfo(Guid guid) { int index = IndexOf(guid); if (-1 == index) { //we didn't find it throw new ArgumentException("stylusPointProperty"); } return _stylusPointPropertyInfos[index]; } ////// Returns the index of the given StylusPointProperty by ID, or -1 if none is found /// internal int GetPropertyIndex(Guid guid) { return IndexOf(guid); } ////// GetStylusPointProperties /// public ReadOnlyCollectionGetStylusPointProperties() { return new ReadOnlyCollection (_stylusPointPropertyInfos); } /// /// GetStylusPointPropertyIdss /// internal Guid[] GetStylusPointPropertyIds() { Guid[] ret = new Guid[_stylusPointPropertyInfos.Length]; for (int x = 0; x < ret.Length; x++) { ret[x] = _stylusPointPropertyInfos[x].Id; } return ret; } ////// Internal helper for determining how many ints in a raw int array /// correspond to one point we get from the input system /// internal int GetInputArrayLengthPerPoint() { int buttonLength = _buttonCount > 0 ? 1 : 0; int propertyLength = (_stylusPointPropertyInfos.Length - _buttonCount) + buttonLength; if (!this.ContainsTruePressure) { propertyLength--; } return propertyLength; } ////// Internal helper for determining how many members a StylusPoint's /// internal int[] should be for additional data /// internal int GetExpectedAdditionalDataCount() { int buttonLength = _buttonCount > 0 ? 1 : 0; int expectedLength = ((_stylusPointPropertyInfos.Length - _buttonCount) + buttonLength) - 3 /*x, y, p*/; return expectedLength; } ////// Internal helper for determining how many ints in a raw int array /// correspond to one point when saving to himetric /// ///internal int GetOutputArrayLengthPerPoint() { int length = GetInputArrayLengthPerPoint(); if (!this.ContainsTruePressure) { length++; } return length; } /// /// Internal helper for determining how many buttons are present /// internal int ButtonCount { get { return _buttonCount; } } ////// Internal helper for determining what bit position the button is at /// internal int GetButtonBitPosition(StylusPointProperty buttonProperty) { if (!buttonProperty.IsButton) { throw new InvalidOperationException(); } int buttonIndex = 0; for (int x = _stylusPointPropertyInfos.Length - _buttonCount; //start of the buttons x < _stylusPointPropertyInfos.Length; x++) { if (_stylusPointPropertyInfos[x].Id == buttonProperty.Id) { return buttonIndex; } if (_stylusPointPropertyInfos[x].IsButton) { // we're in the buttons, but this isn't the right one, // bump the button index and keep looking buttonIndex++; } } return -1; } ////// ContainsTruePressure - true if this StylusPointDescription was instanced /// by a TabletDevice or by ISF serialization that contains NormalPressure /// internal bool ContainsTruePressure { get { return (_originalPressureIndex != -1); } } ////// Internal helper to determine the original pressure index /// internal int OriginalPressureIndex { get { return _originalPressureIndex; } } ////// Returns true if the two StylusPointDescriptions have the same StylusPointProperties. Metrics are ignored. /// /// stylusPointDescription1 /// stylusPointDescription2 public static bool AreCompatible(StylusPointDescription stylusPointDescription1, StylusPointDescription stylusPointDescription2) { if (stylusPointDescription1 == null || stylusPointDescription2 == null) { throw new ArgumentNullException("stylusPointDescription"); } #pragma warning disable 6506 // if a StylusPointDescription is not null, then _stylusPointPropertyInfos is not null. // // ignore X, Y, Pressure - they are guaranteed to be the first3 members // Debug.Assert( stylusPointDescription1._stylusPointPropertyInfos.Length >= RequiredCountOfProperties && stylusPointDescription1._stylusPointPropertyInfos[0].Id == StylusPointPropertyIds.X && stylusPointDescription1._stylusPointPropertyInfos[1].Id == StylusPointPropertyIds.Y && stylusPointDescription1._stylusPointPropertyInfos[2].Id == StylusPointPropertyIds.NormalPressure); Debug.Assert( stylusPointDescription2._stylusPointPropertyInfos.Length >= RequiredCountOfProperties && stylusPointDescription2._stylusPointPropertyInfos[0].Id == StylusPointPropertyIds.X && stylusPointDescription2._stylusPointPropertyInfos[1].Id == StylusPointPropertyIds.Y && stylusPointDescription2._stylusPointPropertyInfos[2].Id == StylusPointPropertyIds.NormalPressure); if (stylusPointDescription1._stylusPointPropertyInfos.Length != stylusPointDescription2._stylusPointPropertyInfos.Length) { return false; } for (int x = RequiredCountOfProperties; x < stylusPointDescription1._stylusPointPropertyInfos.Length; x++) { if (!StylusPointPropertyInfo.AreCompatible(stylusPointDescription1._stylusPointPropertyInfos[x], stylusPointDescription2._stylusPointPropertyInfos[x])) { return false; } } #pragma warning restore 6506 return true; } ////// Returns a new StylusPointDescription with the common StylusPointProperties from both /// /// stylusPointDescription /// stylusPointDescriptionPreserveInfo ///The StylusPointProperties from stylusPointDescriptionPreserveInfo will be returned in the new StylusPointDescription public static StylusPointDescription GetCommonDescription(StylusPointDescription stylusPointDescription, StylusPointDescription stylusPointDescriptionPreserveInfo) { if (stylusPointDescription == null) { throw new ArgumentNullException("stylusPointDescription"); } if (stylusPointDescriptionPreserveInfo == null) { throw new ArgumentNullException("stylusPointDescriptionPreserveInfo"); } #pragma warning disable 6506 // if a StylusPointDescription is not null, then _stylusPointPropertyInfos is not null. // // ignore X, Y, Pressure - they are guaranteed to be the first3 members // Debug.Assert(stylusPointDescription._stylusPointPropertyInfos.Length >= 3 && stylusPointDescription._stylusPointPropertyInfos[0].Id == StylusPointPropertyIds.X && stylusPointDescription._stylusPointPropertyInfos[1].Id == StylusPointPropertyIds.Y && stylusPointDescription._stylusPointPropertyInfos[2].Id == StylusPointPropertyIds.NormalPressure); Debug.Assert(stylusPointDescriptionPreserveInfo._stylusPointPropertyInfos.Length >= 3 && stylusPointDescriptionPreserveInfo._stylusPointPropertyInfos[0].Id == StylusPointPropertyIds.X && stylusPointDescriptionPreserveInfo._stylusPointPropertyInfos[1].Id == StylusPointPropertyIds.Y && stylusPointDescriptionPreserveInfo._stylusPointPropertyInfos[2].Id == StylusPointPropertyIds.NormalPressure); //add x, y, p ListcommonProperties = new List (); commonProperties.Add(stylusPointDescriptionPreserveInfo._stylusPointPropertyInfos[0]); commonProperties.Add(stylusPointDescriptionPreserveInfo._stylusPointPropertyInfos[1]); commonProperties.Add(stylusPointDescriptionPreserveInfo._stylusPointPropertyInfos[2]); //add common properties for (int x = RequiredCountOfProperties; x < stylusPointDescription._stylusPointPropertyInfos.Length; x++) { for (int y = RequiredCountOfProperties; y < stylusPointDescriptionPreserveInfo._stylusPointPropertyInfos.Length; y++) { if (StylusPointPropertyInfo.AreCompatible( stylusPointDescription._stylusPointPropertyInfos[x], stylusPointDescriptionPreserveInfo._stylusPointPropertyInfos[y])) { commonProperties.Add(stylusPointDescriptionPreserveInfo._stylusPointPropertyInfos[y]); } } } #pragma warning restore 6506 return new StylusPointDescription(commonProperties); } /// /// Returns true if this StylusPointDescription is a subset /// of the StylusPointDescription passed in /// /// stylusPointDescriptionSuperset ///public bool IsSubsetOf(StylusPointDescription stylusPointDescriptionSuperset) { if (null == stylusPointDescriptionSuperset) { throw new ArgumentNullException("stylusPointDescriptionSuperset"); } if (stylusPointDescriptionSuperset._stylusPointPropertyInfos.Length < _stylusPointPropertyInfos.Length) { return false; } // // iterate through our local properties and make sure that the // superset contains them // for (int x = 0; x < _stylusPointPropertyInfos.Length; x++) { Guid id = _stylusPointPropertyInfos[x].Id; if (-1 == stylusPointDescriptionSuperset.IndexOf(id)) { return false; } } return true; } /// /// Returns the index of the given StylusPointProperty, or -1 if none is found /// /// propertyId private int IndexOf(Guid propertyId) { for (int x = 0; x < _stylusPointPropertyInfos.Length; x++) { if (_stylusPointPropertyInfos[x].Id == propertyId) { return x; } } return -1; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
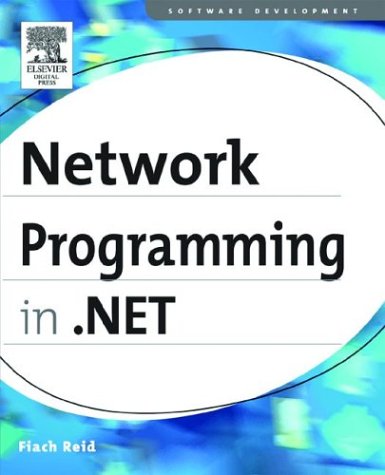
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FormsAuthenticationCredentials.cs
- ActivationArguments.cs
- WebControl.cs
- NameSpaceExtractor.cs
- ConnectionPointConverter.cs
- InputScopeConverter.cs
- GradientSpreadMethodValidation.cs
- ProcessHostConfigUtils.cs
- RegexRunnerFactory.cs
- BulletChrome.cs
- XComponentModel.cs
- ExpressionParser.cs
- GradientBrush.cs
- TypeDependencyAttribute.cs
- XmlLoader.cs
- ThreadStartException.cs
- ConfigXmlCDataSection.cs
- HoistedLocals.cs
- StaticDataManager.cs
- ContainerActivationHelper.cs
- EventLogPermissionEntry.cs
- DataControlFieldCollection.cs
- NameSpaceEvent.cs
- HostAdapter.cs
- DoubleKeyFrameCollection.cs
- KerberosTicketHashIdentifierClause.cs
- arc.cs
- ValueChangedEventManager.cs
- CheckBox.cs
- AssertSection.cs
- ToolStripDropDownClosedEventArgs.cs
- CompareValidator.cs
- MdiWindowListStrip.cs
- ModelTreeEnumerator.cs
- PermissionAttributes.cs
- WsdlContractConversionContext.cs
- Selector.cs
- IProvider.cs
- XmlImplementation.cs
- DataColumnMappingCollection.cs
- CharUnicodeInfo.cs
- _DomainName.cs
- TextParagraphProperties.cs
- BinaryFormatterWriter.cs
- EventsTab.cs
- ScriptReferenceBase.cs
- ToolZone.cs
- Pts.cs
- SaveFileDialog.cs
- _NegotiateClient.cs
- AtomEntry.cs
- SingleSelectRootGridEntry.cs
- DrawingGroup.cs
- CellQuery.cs
- TableRowCollection.cs
- IndexerNameAttribute.cs
- ProfileServiceManager.cs
- JpegBitmapDecoder.cs
- ReadOnlyDataSource.cs
- ProfileWorkflowElement.cs
- OdbcDataReader.cs
- PointAnimationUsingKeyFrames.cs
- GeometryConverter.cs
- KerberosRequestorSecurityToken.cs
- CompilerParameters.cs
- MessageFilterException.cs
- InputScopeManager.cs
- typedescriptorpermission.cs
- SiteMapHierarchicalDataSourceView.cs
- DataTableMapping.cs
- StringBlob.cs
- SqlDataSourceParameterParser.cs
- HybridObjectCache.cs
- TreeNodeCollection.cs
- FixedPageStructure.cs
- WebScriptMetadataFormatter.cs
- DetailsViewPageEventArgs.cs
- XmlFormatReaderGenerator.cs
- HiddenFieldPageStatePersister.cs
- SimpleTypeResolver.cs
- EntryIndex.cs
- FaultPropagationQuery.cs
- URLIdentityPermission.cs
- DesignerSerializationOptionsAttribute.cs
- _AutoWebProxyScriptWrapper.cs
- PageThemeBuildProvider.cs
- SessionStateSection.cs
- AlgoModule.cs
- HttpApplication.cs
- WindowsSecurityToken.cs
- PrinterSettings.cs
- DataServiceCollectionOfT.cs
- parserscommon.cs
- UserValidatedEventArgs.cs
- TextBox.cs
- WsatTransactionHeader.cs
- BitmapData.cs
- MailDefinition.cs
- RemoveStoryboard.cs
- OleDbParameter.cs