Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / MS / Internal / IO / Packaging / ContainerActivationHelper.cs / 1 / ContainerActivationHelper.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Helper functions to be used for activating/hosting a container // // History: // 07/19/04: [....] Refacted out from Application.cs and DocobjHost.cs // 07/19/04: [....] Added side-by-side support for Metro Package // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.IO; using System.IO.Packaging; using System.Reflection; using System.Security; using System.Security.Permissions; using System.Windows; using System.Windows.Navigation; using System.Windows.Markup; using MS.Internal.IO.Packaging.CompoundFile; using MS.Internal.Utility; using MS.Internal.PresentationFramework; using MS.Utility; // MimeType using MS.Internal.Documents; // DocumentApplication namespace MS.Internal.IO.Packaging { ////// Helper class to be used for activating or hosting a container /// static internal class ContainerActivationHelper { //----------------------------------------------------- // // Public Constructors // //----------------------------------------------------- // NONE //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- // NONE //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ // NONE //----------------------------------------------------- // // Public Events // //------------------------------------------------------ // NONE //----------------------------------------------------- // // Internal Constructors // //----------------------------------------------------- // None //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Load an app from a container /// ////// ////// Critical: This sets the container path, which is critical, and handles other critical data, such as the /// uri, rootStorage, container, and ServiceProvider /// [SecurityCritical] static internal Application LoadFromContainer(Uri containerUri, #if CF_Envelope_Activation_Enabled StorageRoot rootStorage, #endif Package container, IServiceProvider isp) { #if CF_Envelope_Activation_Enabled CheckAndAssertForContainer(rootStorage, container); #else CheckAndAssertForContainer(container); #endif #if CF_Envelope_Activation_Enabled if (rootStorage != null) { // // This is RM Initialization event. for Compound File case DataSpaceManager dataSpaceManager = rootStorage.GetDataSpaceManager(); dataSpaceManager.OnTransformInitialization += new DataSpaceManager.TransformInitializeEventHandler(Application.HandleCompoundFileRmTransformInitConsumption); } BindUriHelper.Container = rootStorage; #endif // CF_Envelope_Activation_Enabled #if CF_Envelope_Activation_Enabled if (rootStorage != null) { // ToDo ([....]: CF Envelope): We need this when we implement CF Envelope // this includes the container and is in the ssres scheme // BindUriHelper.BaseUri = CompoundFileUri.CreateFromAbsoluteUri(containerUri); } else { #endif Debug.Assert(container != null); // Case sensitive is fine here because Uri.Scheme contract is to return in lower case only. if (!SecurityHelper.AreStringTypesEqual(containerUri.Scheme, PackUriHelper.UriSchemePack)) { // this includes the container and is in the pack scheme BindUriHelper.BaseUri = PackUriHelper.Create(containerUri); } #if CF_Envelope_Activation_Enabled } return GetContainerEntryPoint(rootStorage, container, isp); #else return GetContainerEntryPoint(container, isp); #endif } #endregion Internal Methods //------------------------------------------------------ // // Internal Events // //----------------------------------------------------- // None //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods #if CF_Envelope_Activation_Enabled // ToDo ([....]: CF Envelope): We need this when we implement CF Envelope ////// Get the application from a container /// ////// /// ToDo ([....]): PS# 25616 Remove the parameter, rootStorage, once we make full transition to Metro ////// Critical because it sets the IServiceProvider (critical data) based on input parameters. /// [SecurityCritical] static private Application GetContainerEntryPoint(StorageRoot rootStorage, Package container, IServiceProvider isp) { if (container != null) { return GetContainerEntryPoint(container, isp); } if (rootStorage != null) { return GetContainerEntryPoint(rootStorage, isp); } return null; } ////// Get the application from a container (CF) /// ////// /// ToDo ([....]): PS# 25616 Remove this method once we make full transition to Metro ////// Critical because it sets the apps IServiceProvider (critical data) based on an input parameter /// [SecurityCritical] static private Application GetContainerEntryPoint(StorageRoot root, IServiceProvider isp) { StorageInfo rootStorage = (StorageInfo) root; StreamInfo startupPageStreamInfo = new StreamInfo(rootStorage, StartupPageStreamName); if (!startupPageStreamInfo.Exists) { throw new NotSupportedException(SR.Get(SRID.UnknownContainerFormat)); } string startupPage = GetStringFromStreamInfo(startupPageStreamInfo); if (startupPage == null || startupPage.Length == 0) { throw new NotSupportedException(SR.Get(SRID.UnknownContainerFormat)); } Application appObject = new Application(); appObject.StartupUri = new Uri(startupPage, UriKind.RelativeOrAbsolute); if (isp != null) { appObject.ServiceProvider = isp; } return appObject; } #endif ////// Get the application from a container /// ////// /// ToDo ([....]): PS# 25616 Make this one internal and remove other overloads /// once we make full transition to Metro ////// Critical because it sets the apps IServiceProvider (critical data) based on an input parameter /// [SecurityCritical] static private Application GetContainerEntryPoint(Package container, IServiceProvider isp) { // check to make sure that StartingPart is xaml PackagePart startingPart = GetReachPackageStartingPart(container); // the only supported mime types in a container if ((startingPart == null) || (!startingPart.ValidatedContentType.AreTypeAndSubTypeEqual( MimeTypeMapper.FixedDocumentSequenceMime ))) { throw new NotSupportedException(SR.Get(SRID.UnknownContainerFormat)); } // load DocumentApplication DocumentApplication appObject = new DocumentApplication(); // assign StartPart as Startup Uri appObject.StartupUri = startingPart.Uri; if (isp != null) { appObject.ServiceProvider = isp; } return appObject; } #if CF_Envelope_Activation_Enabled // ToDo ([....]: CF Envelope): We need this when we implement CF Envelope // ToDo ([....]: CF Envelope): We need this when we implement CF Envelope ////// Get a string from the stream /// ////// /// ToDo ([....]): PS# 25616 Remove this method once we make full transition to Metro private static string GetStringFromStreamInfo(StreamInfo streamInfo) { string streamString; using(Stream stream = streamInfo.Open(FileMode.Open, FileAccess.Read)) { using(BinaryReader binaryReader = new BinaryReader(stream)) { streamString = binaryReader.ReadString(); } } return streamString; } ////// Get the Uri of the stream given base Uri /// ////// /// ToDo ([....]): PS# 25616 Remove this method once we make full transition to Metro ////// Critical as it access the base uri through GetResolvedUri /// TreatAsSafe since it demands unrestricted permission /// [SecurityCritical, SecurityTreatAsSafe] private static Uri GetUriForStream(Uri baseBpu, String streamFullName) { SecurityHelper.DemandUnmanagedCode(); Debug.Assert(streamFullName != null && streamFullName.Length > 0); if (streamFullName == null || streamFullName.Length < 1) return null; // // Create Uri for the given stream // Uri bpu = new Uri(streamFullName.Replace('\\', '/'), UriKind.RelativeOrAbsolute); if (bpu.IsAbsoluteUri == false) bpu = BindUriHelper.GetResolvedUri(CompoundFileUri.CreateFromAbsoluteUri(baseBpu), bpu); return bpu; } #endif static private void CheckAndAssertForContainer( #if CF_Envelope_Activation_Enabled StorageRoot root, #endif Package container) { #if CF_Envelope_Activation_Enabled // ToDo ([....]: CF Envelope): We need this when we implement CF Envelope // ToDo ([....]: CF Envelope): We need this when we implement CF Envelope Debug.Assert((root != null && container == null) || (root == null && container != null), "Only one of CF-specific or Metro container should be opened"); #else Debug.Assert(container != null, "No container is opened"); #endif } ////// Gets StartingPart of the Reach Package. /// ///PackagePart static private PackagePart GetReachPackageStartingPart(Package package) { Debug.Assert(package != null, "package cannot be null"); PackageRelationship startingPartRelationship = null; foreach (PackageRelationship rel in package.GetRelationshipsByType(ReachPackageStartingPartRelationshipType)) { if (startingPartRelationship != null) { throw new InvalidDataException(SR.Get(SRID.MoreThanOneStartingParts)); } startingPartRelationship = rel; } if (startingPartRelationship != null) { Uri startPartUri = PackUriHelper.ResolvePartUri(startingPartRelationship.SourceUri, startingPartRelationship.TargetUri); if(package.PartExists(startPartUri)) return (package.GetPart(startPartUri)); else return null; } return null; } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Members //we use this dummy URI to resolve relative URIs treating the container as the authority. private static readonly Uri _defaultUri = new Uri("http://defaultcontainer/"); private static readonly string ReachPackageStartingPartRelationshipType = "http://schemas.microsoft.com/xps/2005/06/fixedrepresentation"; #endregion Private Members } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
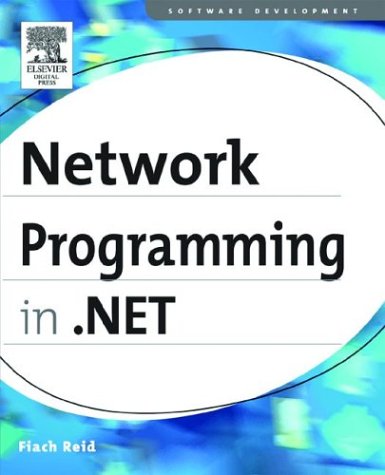
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AutomationPatternInfo.cs
- WebPartConnection.cs
- DependencyProperty.cs
- EntityUtil.cs
- SqlUdtInfo.cs
- DisposableCollectionWrapper.cs
- designeractionbehavior.cs
- DataGrid.cs
- AnnotationService.cs
- TimerEventSubscriptionCollection.cs
- SmtpTransport.cs
- DataGridViewCellEventArgs.cs
- RedirectionProxy.cs
- SemanticResultValue.cs
- PersonalizationProvider.cs
- DelegateTypeInfo.cs
- ConfigPathUtility.cs
- BamlWriter.cs
- xmlglyphRunInfo.cs
- LocalizationParserHooks.cs
- LabelLiteral.cs
- ListViewHitTestInfo.cs
- OleDbDataReader.cs
- SingleBodyParameterMessageFormatter.cs
- FolderBrowserDialog.cs
- IPAddressCollection.cs
- WbmpConverter.cs
- httpserverutility.cs
- TemplateAction.cs
- NegotiateStream.cs
- CodeTypeMember.cs
- List.cs
- SourceFilter.cs
- SocketElement.cs
- ParseNumbers.cs
- MemberAccessException.cs
- ProviderMetadata.cs
- DependencyPropertyKind.cs
- ToolStripItemImageRenderEventArgs.cs
- MsmqIntegrationSecurityElement.cs
- XamlSerializerUtil.cs
- SystemPens.cs
- _ConnectOverlappedAsyncResult.cs
- DebuggerAttributes.cs
- DataGridViewCellCancelEventArgs.cs
- WebPartZoneDesigner.cs
- ApplicationException.cs
- PageContentCollection.cs
- InvokePattern.cs
- SizeChangedInfo.cs
- MenuItemBinding.cs
- GrabHandleGlyph.cs
- Int64.cs
- Convert.cs
- MeasurementDCInfo.cs
- XmlFormatExtensionPrefixAttribute.cs
- CmsUtils.cs
- SafeRightsManagementQueryHandle.cs
- embossbitmapeffect.cs
- AnnotationHighlightLayer.cs
- WebPartConnectionsConfigureVerb.cs
- TextBoxRenderer.cs
- FactoryRecord.cs
- DbBuffer.cs
- XPathParser.cs
- MobileDeviceCapabilitiesSectionHandler.cs
- DataSourceProvider.cs
- FontDriver.cs
- DispatchChannelSink.cs
- NestedContainer.cs
- ProcessManager.cs
- OdbcError.cs
- TableCellAutomationPeer.cs
- CachedFontFamily.cs
- SettingsPropertyNotFoundException.cs
- ErrorEventArgs.cs
- AnnotationResource.cs
- CapabilitiesSection.cs
- InternalEnumValidatorAttribute.cs
- DateTimeConstantAttribute.cs
- ArrayExtension.cs
- ObjectSet.cs
- WindowsRebar.cs
- KeyFrames.cs
- HandleCollector.cs
- ContentElement.cs
- SchemaCollectionPreprocessor.cs
- Scripts.cs
- DataAccessException.cs
- TakeQueryOptionExpression.cs
- Point3DCollection.cs
- DocumentViewerBaseAutomationPeer.cs
- SqlCacheDependencyDatabase.cs
- RoleService.cs
- ExtensionSimplifierMarkupObject.cs
- TextBoxRenderer.cs
- XmlNullResolver.cs
- FormView.cs
- DataRowView.cs
- CommentEmitter.cs