Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Data / System / Data / DataRowView.cs / 4 / DataRowView.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data { using System.Diagnostics; using System.ComponentModel; #if WINFSInternalOnly internal #else public #endif class DataRowView : ICustomTypeDescriptor, System.ComponentModel.IEditableObject, System.ComponentModel.IDataErrorInfo, System.ComponentModel.INotifyPropertyChanged { private readonly DataView dataView; private readonly DataRow _row; private bool delayBeginEdit; private static PropertyDescriptorCollection zeroPropertyDescriptorCollection = new PropertyDescriptorCollection(null); ////// When the PropertyChanged event happens, it must happen on the same DataRowView reference. /// This is so a generic event handler like Windows Presentation Foundation can redirect as appropriate. /// Having DataView.Equals is not sufficient for WPF, because two different instances may be equal but not equivalent. /// For DataRowView, if two instances are equal then they are equivalent. /// private System.ComponentModel.PropertyChangedEventHandler onPropertyChanged; internal DataRowView(DataView dataView, DataRow row) { this.dataView = dataView; this._row = row; } ////// Checks for same reference instead of equivalent ///or . /// /// Necessary for ListChanged event handlers to use data structures that use the default to /// instead of /// to understand if they need to add a event handler. /// public override bool Equals(object other) { return Object.ReferenceEquals(this, other); } /// Hashcode of public override Int32 GetHashCode() { // Everett compatability, must return hashcode for DataRow // this does prevent using this object in collections like Hashtable // which use the hashcode as an immutable value to identify this object // user could/should have used the DataRow property instead of the hashcode return Row.GetHashCode(); } public DataView DataView { get { return dataView; } } internal int ObjectID { get { return _row.ObjectID; } } ///Gets or sets a value in specified column. /// Specified column index. ///Uses either ///or to access /// when setting a value. public object this[int ndx] { get { return Row[ndx, RowVersionDefault]; } set { if (!dataView.AllowEdit && !IsNew) { throw ExceptionBuilder.CanNotEdit(); } SetColumnValue(dataView.Table.Columns[ndx], value); } } /// Gets the specified column value or related child view or sets a value in specified column. /// Specified column or relation name when getting. Specified column name when setting. ////// when is ambigous. Unmatched ///when getting a value. Unmatched ///when setting a value. public object this[string property] { get { DataColumn column = dataView.Table.Columns[property]; if (null != column) { return Row[column, RowVersionDefault]; } else if (dataView.Table.DataSet != null && dataView.Table.DataSet.Relations.Contains(property)) { return CreateChildView(property); } throw ExceptionBuilder.PropertyNotFound(property, dataView.Table.TableName); } set { DataColumn column = dataView.Table.Columns[property]; if (null == column) { throw ExceptionBuilder.SetFailed(property); } if (!dataView.AllowEdit && !IsNew) { throw ExceptionBuilder.CanNotEdit(); } SetColumnValue(column, value); } } // IDataErrorInfo stuff string System.ComponentModel.IDataErrorInfo.this[string colName] { get { return Row.GetColumnError(colName); } } string System.ComponentModel.IDataErrorInfo.Error { get { return Row.RowError; } } /// when setting a value. /// Gets the current version description of the ////// in relation to /// Either public DataRowVersion RowVersion { get { return (RowVersionDefault & ~DataRowVersion.Proposed); } } ///or Either private DataRowVersion RowVersionDefault { get { return Row.GetDefaultRowVersion(dataView.RowStateFilter); } } internal int GetRecord() { return Row.GetRecordFromVersion(RowVersionDefault); } internal object GetColumnValue(DataColumn column) { return Row[column, RowVersionDefault]; } internal void SetColumnValue(DataColumn column, object value) { if (delayBeginEdit) { delayBeginEdit = false; Row.BeginEdit(); } if (DataRowVersion.Original == RowVersionDefault) { throw ExceptionBuilder.SetFailed(column.ColumnName); } Row[column] = value; } ///or /// Returns a /// Specified/// for the child /// with the specified . /// . /// null or mismatch between public DataView CreateChildView(DataRelation relation) { if (relation == null || relation.ParentKey.Table != DataView.Table) { throw ExceptionBuilder.CreateChildView(); } int record = GetRecord(); object[] values = relation.ParentKey.GetKeyValues(record); RelatedView childView = new RelatedView(relation.ChildColumnsReference, values); childView.SetIndex("", DataViewRowState.CurrentRows, null); // finish construction via RelatedView.SetIndex childView.SetDataViewManager(DataView.DataViewManager); return childView; } ///and . /// Specified name. /// Unmatched public DataView CreateChildView(string relationName) { return CreateChildView(DataView.Table.ChildRelations[relationName]); } public DataRow Row { get { return _row; } } public void BeginEdit() { delayBeginEdit = true; } public void CancelEdit() { DataRow tmpRow = Row; if (IsNew) { dataView.FinishAddNew(false); } else { tmpRow.CancelEdit(); } delayBeginEdit = false; } public void EndEdit() { if (IsNew) { dataView.FinishAddNew(true); } else { Row.EndEdit(); } delayBeginEdit = false; } public bool IsNew { get { return (_row == dataView.addNewRow); } } public bool IsEdit { get { return ( Row.HasVersion(DataRowVersion.Proposed) || // It was edited or delayBeginEdit); // DataRowView.BegingEdit() was called, but not edited yet. } } public void Delete() { dataView.Delete(Row); } #region ICustomTypeDescriptor AttributeCollection ICustomTypeDescriptor.GetAttributes() { return new AttributeCollection((Attribute[])null); } string ICustomTypeDescriptor.GetClassName() { return null; } string ICustomTypeDescriptor.GetComponentName() { return null; } TypeConverter ICustomTypeDescriptor.GetConverter() { return null; } EventDescriptor ICustomTypeDescriptor.GetDefaultEvent() { return null; } PropertyDescriptor ICustomTypeDescriptor.GetDefaultProperty() { return null; } object ICustomTypeDescriptor.GetEditor(Type editorBaseType) { return null; } EventDescriptorCollection ICustomTypeDescriptor.GetEvents() { return new EventDescriptorCollection(null); } EventDescriptorCollection ICustomTypeDescriptor.GetEvents(Attribute[] attributes) { return new EventDescriptorCollection(null); } PropertyDescriptorCollection ICustomTypeDescriptor.GetProperties() { return((ICustomTypeDescriptor)this).GetProperties(null); } PropertyDescriptorCollection ICustomTypeDescriptor.GetProperties(Attribute[] attributes) { return (dataView.Table != null ? dataView.Table.GetPropertyDescriptorCollection(attributes) : zeroPropertyDescriptorCollection); } object ICustomTypeDescriptor.GetPropertyOwner(PropertyDescriptor pd) { return this; } #endregion public event PropertyChangedEventHandler PropertyChanged { add { onPropertyChanged += value; } remove { onPropertyChanged -= value; } } internal void RaisePropertyChangedEvent (string propName){ // Do not try catch, we would mask users bugs. if they throw we would catch if (onPropertyChanged != null) { onPropertyChanged (this, new PropertyChangedEventArgs(propName)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved..
Link Menu
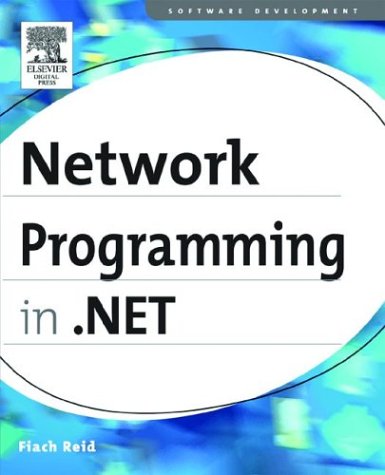
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EditorAttribute.cs
- DBCommand.cs
- SafeThemeHandle.cs
- ColumnMapProcessor.cs
- FrameworkElementFactoryMarkupObject.cs
- ObjectSelectorEditor.cs
- CounterSample.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- EventProxy.cs
- InputBuffer.cs
- ImageFormatConverter.cs
- DesignerDataTableBase.cs
- PropertyValueUIItem.cs
- DesignBindingEditor.cs
- Assert.cs
- TextOutput.cs
- DelegateArgument.cs
- WebPartChrome.cs
- XamlTypeMapperSchemaContext.cs
- ColorInterpolationModeValidation.cs
- PenCursorManager.cs
- TemplateInstanceAttribute.cs
- SymbolResolver.cs
- GetWinFXPath.cs
- ConditionChanges.cs
- MetaModel.cs
- BlurBitmapEffect.cs
- DesignerDataRelationship.cs
- InputMethodStateTypeInfo.cs
- XmlSchemaInclude.cs
- ItemDragEvent.cs
- Contracts.cs
- WinEventWrap.cs
- QueueProcessor.cs
- XmlAnyAttributeAttribute.cs
- ConfigurationManagerInternalFactory.cs
- TraceInternal.cs
- FormViewUpdatedEventArgs.cs
- ExtenderProvidedPropertyAttribute.cs
- ConfigUtil.cs
- PreDigestedSignedInfo.cs
- PropertyValidationContext.cs
- SiteMapDataSourceView.cs
- TextRange.cs
- PageAdapter.cs
- ExpressionBindingCollection.cs
- TagMapInfo.cs
- ResponseStream.cs
- SubpageParagraph.cs
- QEncodedStream.cs
- HtmlTernaryTree.cs
- HtmlInputPassword.cs
- HttpProcessUtility.cs
- XmlValueConverter.cs
- PartialArray.cs
- DoubleSumAggregationOperator.cs
- EventToken.cs
- _ServiceNameStore.cs
- MaterializeFromAtom.cs
- PinnedBufferMemoryStream.cs
- PingOptions.cs
- AppModelKnownContentFactory.cs
- StorageComplexTypeMapping.cs
- LocatorGroup.cs
- PackagePart.cs
- AttributeCollection.cs
- X509WindowsSecurityToken.cs
- WebRequestModuleElement.cs
- MemberAccessException.cs
- SizeF.cs
- List.cs
- LinkButton.cs
- HtmlWindowCollection.cs
- SystemWebExtensionsSectionGroup.cs
- WindowsToolbarItemAsMenuItem.cs
- AttributeQuery.cs
- DetailsView.cs
- MailAddressCollection.cs
- CommunicationObject.cs
- BufferModesCollection.cs
- BitmapEffectDrawingContextState.cs
- XhtmlConformanceSection.cs
- HttpRawResponse.cs
- TableCellsCollectionEditor.cs
- VisualBasicDesignerHelper.cs
- HttpApplication.cs
- InvalidPropValue.cs
- DefinitionUpdate.cs
- UndoUnit.cs
- CheckBoxBaseAdapter.cs
- FormatConvertedBitmap.cs
- Dispatcher.cs
- TracedNativeMethods.cs
- KeyValuePairs.cs
- Double.cs
- Rights.cs
- GridItem.cs
- FillBehavior.cs
- DataGridViewLayoutData.cs
- SrgsRuleRef.cs