Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataWeb / Server / System / Data / Services / UpdateTracker.cs / 1 / UpdateTracker.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a class used to track updates for callbacks. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services { using System.Collections.Generic; using System.Data.Services.Providers; using System.Diagnostics; using System.Reflection; ///Provides a class used to track updates for callbacks. internal class UpdateTracker { #region Private fields. ////// A dictionary of containers mapping to the changes on those /// containers, each of which consists of an element and the /// action taken on it. /// private Dictionary> items; /// Underlying data source instance. private IDataServiceProvider provider; #endregion Private fields. ///Initializes a new /// underlying data source instance. private UpdateTracker(IDataServiceProvider provider) { this.provider = provider; this.items = new Dictionaryinstance. >(); } /// Fires the notification for a single action. /// Service on which methods should be invoked. /// Object to be tracked. /// Container in which object is changed. /// Action affecting target. internal static void FireNotification(object service, object target, ResourceContainer container, UpdateOperations action) { Debug.Assert(service != null, "service != null"); AssertActionValues(target, container); MethodInfo[] methods = container.WriteAuthorizationMethods; if (methods != null) { object[] parameters = new object[2]; parameters[0] = target; parameters[1] = action; for (int i = 0; i < methods.Length; i++) { try { methods[i].Invoke(service, parameters); } catch (TargetInvocationException exception) { ErrorHandler.HandleTargetInvocationException(exception); throw; } } } } ////// Create a new instance of update tracker /// /// description about the request uri. /// underlying data source. ////// Returns a new instance of UpdateTracker if the request is not targetted at open types; null otherwise. /// #if !ASTORIA_OPEN_OBJECT [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", MessageId = "description", Justification = "Required for open type support")] #endif internal static UpdateTracker CreateUpdateTracker(RequestDescription description, IDataServiceProvider provider) { // We don't support notifications for open types. Hence not creating a tracker for open types request. #if ASTORIA_OPEN_OBJECT if (description.TargetKind != RequestTargetKind.OpenProperty && description.TargetKind != RequestTargetKind.OpenPropertyValue) { #endif return new UpdateTracker(provider); #if ASTORIA_OPEN_OBJECT } return null; #endif } ///Fires all notifications /// Service on which methods should be invoked. internal void FireNotifications(object service) { Debug.Assert(service != null, "service != null"); object[] parameters = new object[2]; foreach (var item in this.items) { MethodInfo[] methods = item.Key.WriteAuthorizationMethods; Debug.Assert(methods != null, "methods != null - should not have been tracking changes to the container otherwise."); foreach (var element in item.Value) { parameters[0] = this.provider.ResolveResource(element.Key); parameters[1] = element.Value; for (int i = 0; i < methods.Length; i++) { try { methods[i].Invoke(service, parameters); } catch (TargetInvocationException exception) { ErrorHandler.HandleTargetInvocationException(exception); throw; } } } // Make elements elegible for garbage collection. item.Value.Clear(); } // Make dictionary elegible for garbage collection. this.items = null; } ////// Tracks the specified /// Object to be tracked. /// Container in which object is changed. /// Action affecting target. ///for a /// given on the . /// /// If internal void TrackAction(object target, ResourceContainer container, UpdateOperations action) { AssertActionValues(target, container); Debug.Assert(this.items != null, "this.items != null - otherwise FireNotification has already been called"); // If it won't be necessary for us to fire authorizatio methods, // skip tracking altogether. if (container.WriteAuthorizationMethods == null) { return; } // Get the container for which the change has taken place. Dictionarywas already being tracked, the actions are OR'ed together. ///
Link Menu
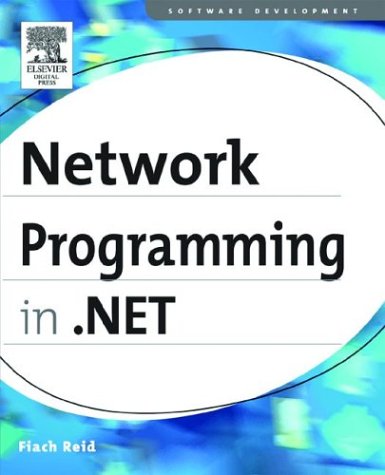
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RenderTargetBitmap.cs
- WebPageTraceListener.cs
- MaxSessionCountExceededException.cs
- FtpCachePolicyElement.cs
- VSDExceptions.cs
- UInt32.cs
- KeyboardEventArgs.cs
- TreeIterator.cs
- BuildManagerHost.cs
- Calendar.cs
- RelationshipConstraintValidator.cs
- ResolveNameEventArgs.cs
- ResourceExpressionEditorSheet.cs
- DictionaryEntry.cs
- SerializationException.cs
- DoubleAnimationBase.cs
- MessageUtil.cs
- TypeConverterAttribute.cs
- CalendarTable.cs
- XmlDataCollection.cs
- Attributes.cs
- ChooseAction.cs
- StrongNameIdentityPermission.cs
- MetadataItemSerializer.cs
- Flattener.cs
- ScriptReferenceBase.cs
- ImageAutomationPeer.cs
- DynamicFilter.cs
- CircleHotSpot.cs
- _NetRes.cs
- Label.cs
- GroupBoxRenderer.cs
- TimeBoundedCache.cs
- SafeProcessHandle.cs
- ScrollData.cs
- SamlAdvice.cs
- XmlEnumAttribute.cs
- TextUtf8RawTextWriter.cs
- RegisterResponseInfo.cs
- RoleGroupCollection.cs
- EventSinkHelperWriter.cs
- WarningException.cs
- HtmlTable.cs
- DeflateInput.cs
- DBAsyncResult.cs
- PartitionedStream.cs
- ClickablePoint.cs
- ObjectStateFormatter.cs
- ProviderUtil.cs
- ExternalFile.cs
- WinEventWrap.cs
- PersistenceTask.cs
- ColumnPropertiesGroup.cs
- ValueChangedEventManager.cs
- SharedStatics.cs
- IDQuery.cs
- StreamInfo.cs
- DataGridViewCellCollection.cs
- XmlSchemaResource.cs
- RegisteredScript.cs
- SQLString.cs
- ACL.cs
- Internal.cs
- SecurityAlgorithmSuiteConverter.cs
- XPathNavigatorReader.cs
- FrugalMap.cs
- ObjectDataProvider.cs
- DataFormats.cs
- updateconfighost.cs
- OleAutBinder.cs
- CAGDesigner.cs
- RequestUriProcessor.cs
- FrameworkContextData.cs
- RtfControls.cs
- RelationshipNavigation.cs
- UserControl.cs
- ImageMap.cs
- SerTrace.cs
- SafeMILHandle.cs
- SafeRegistryHandle.cs
- GCHandleCookieTable.cs
- SoapRpcMethodAttribute.cs
- PrimitiveXmlSerializers.cs
- DataServiceProviderMethods.cs
- Volatile.cs
- FunctionNode.cs
- x509utils.cs
- PerspectiveCamera.cs
- CryptoHandle.cs
- altserialization.cs
- FontEmbeddingManager.cs
- XPathNavigatorKeyComparer.cs
- ReadOnlyDictionary.cs
- NamespaceQuery.cs
- SafeBuffer.cs
- WebPartEditorOkVerb.cs
- counter.cs
- DateTimeConstantAttribute.cs
- TextProviderWrapper.cs
- HasActivatableWorkflowEvent.cs