Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / Configuration / SqlCacheDependencyDatabase.cs / 1 / SqlCacheDependencyDatabase.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.Globalization; using System.IO; using System.Text; using System.Diagnostics; using System.Security.Permissions; // class SqlCacheDependencySection [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class SqlCacheDependencyDatabase : ConfigurationElement { private static readonly ConfigurationElementProperty s_elemProperty = new ConfigurationElementProperty(new CallbackValidator(typeof(SqlCacheDependencyDatabase), Validate)); private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propName; private static readonly ConfigurationProperty _propConnectionStringName; private static readonly ConfigurationProperty _propPollTime; static SqlCacheDependencyDatabase() { // Property initialization _properties = new ConfigurationPropertyCollection(); _propName = new ConfigurationProperty("name", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired | ConfigurationPropertyOptions.IsKey); _propConnectionStringName = new ConfigurationProperty("connectionStringName", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired); _propPollTime = new ConfigurationProperty("pollTime", typeof(int), 60000, ConfigurationPropertyOptions.None); _properties.Add(_propName); _properties.Add(_propConnectionStringName); _properties.Add(_propPollTime); } private int defaultPollTime; // This may be set by the outer node to specify the default poll time (i.e. not specified on this node) public SqlCacheDependencyDatabase(string name, string connectionStringName, int pollTime) { Name = name; ConnectionStringName = connectionStringName; PollTime = pollTime; } public SqlCacheDependencyDatabase(string name, string connectionStringName) { Name = name; ConnectionStringName = connectionStringName; } internal SqlCacheDependencyDatabase() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } protected override ConfigurationElementProperty ElementProperty { get { return s_elemProperty; } } private static void Validate(object value) { if (value == null) { throw new ArgumentNullException("sqlCacheDependencyDatabase"); } Debug.Assert(value is SqlCacheDependencyDatabase); SqlCacheDependencyDatabase elem = (SqlCacheDependencyDatabase)value; if (elem.PollTime != 0 && elem.PollTime < 500) { throw new ConfigurationErrorsException( SR.GetString(SR.Invalid_sql_cache_dep_polltime), elem.ElementInformation.Properties["pollTime"].Source, elem.ElementInformation.Properties["pollTime"].LineNumber); } } internal void CheckDefaultPollTime(int value) { // This method will be called by the outer node. // If the poolTime property is not specified in the node, then grab the one // from above. if (ElementInformation.Properties["pollTime"].ValueOrigin == PropertyValueOrigin.Default) { defaultPollTime = value; } } [ConfigurationProperty("name", IsRequired = true, IsKey = true)] [StringValidator(MinLength = 1)] public string Name { get { return (string)base[_propName]; } set { base[_propName] = value; } } [ConfigurationProperty("connectionStringName", IsRequired = true)] [StringValidator(MinLength = 1)] public string ConnectionStringName { get { return (string)base[_propConnectionStringName]; } set { base[_propConnectionStringName] = value; } } [ConfigurationProperty("pollTime", DefaultValue = 60000)] public int PollTime { get { if (ElementInformation.Properties["pollTime"].ValueOrigin == PropertyValueOrigin.Default) { return defaultPollTime; // Return the default value from outer node } else { return (int)base[_propPollTime]; } } set { base[_propPollTime] = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.Globalization; using System.IO; using System.Text; using System.Diagnostics; using System.Security.Permissions; // class SqlCacheDependencySection [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class SqlCacheDependencyDatabase : ConfigurationElement { private static readonly ConfigurationElementProperty s_elemProperty = new ConfigurationElementProperty(new CallbackValidator(typeof(SqlCacheDependencyDatabase), Validate)); private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propName; private static readonly ConfigurationProperty _propConnectionStringName; private static readonly ConfigurationProperty _propPollTime; static SqlCacheDependencyDatabase() { // Property initialization _properties = new ConfigurationPropertyCollection(); _propName = new ConfigurationProperty("name", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired | ConfigurationPropertyOptions.IsKey); _propConnectionStringName = new ConfigurationProperty("connectionStringName", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired); _propPollTime = new ConfigurationProperty("pollTime", typeof(int), 60000, ConfigurationPropertyOptions.None); _properties.Add(_propName); _properties.Add(_propConnectionStringName); _properties.Add(_propPollTime); } private int defaultPollTime; // This may be set by the outer node to specify the default poll time (i.e. not specified on this node) public SqlCacheDependencyDatabase(string name, string connectionStringName, int pollTime) { Name = name; ConnectionStringName = connectionStringName; PollTime = pollTime; } public SqlCacheDependencyDatabase(string name, string connectionStringName) { Name = name; ConnectionStringName = connectionStringName; } internal SqlCacheDependencyDatabase() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } protected override ConfigurationElementProperty ElementProperty { get { return s_elemProperty; } } private static void Validate(object value) { if (value == null) { throw new ArgumentNullException("sqlCacheDependencyDatabase"); } Debug.Assert(value is SqlCacheDependencyDatabase); SqlCacheDependencyDatabase elem = (SqlCacheDependencyDatabase)value; if (elem.PollTime != 0 && elem.PollTime < 500) { throw new ConfigurationErrorsException( SR.GetString(SR.Invalid_sql_cache_dep_polltime), elem.ElementInformation.Properties["pollTime"].Source, elem.ElementInformation.Properties["pollTime"].LineNumber); } } internal void CheckDefaultPollTime(int value) { // This method will be called by the outer node. // If the poolTime property is not specified in the node, then grab the one // from above. if (ElementInformation.Properties["pollTime"].ValueOrigin == PropertyValueOrigin.Default) { defaultPollTime = value; } } [ConfigurationProperty("name", IsRequired = true, IsKey = true)] [StringValidator(MinLength = 1)] public string Name { get { return (string)base[_propName]; } set { base[_propName] = value; } } [ConfigurationProperty("connectionStringName", IsRequired = true)] [StringValidator(MinLength = 1)] public string ConnectionStringName { get { return (string)base[_propConnectionStringName]; } set { base[_propConnectionStringName] = value; } } [ConfigurationProperty("pollTime", DefaultValue = 60000)] public int PollTime { get { if (ElementInformation.Properties["pollTime"].ValueOrigin == PropertyValueOrigin.Default) { return defaultPollTime; // Return the default value from outer node } else { return (int)base[_propPollTime]; } } set { base[_propPollTime] = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
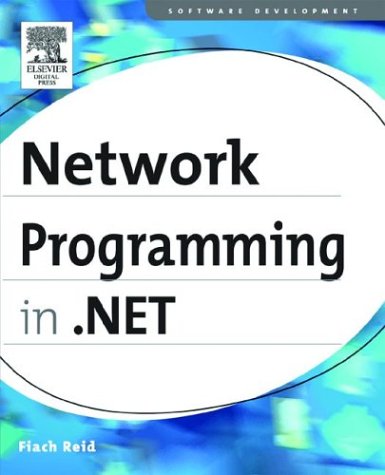
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProfessionalColors.cs
- IMembershipProvider.cs
- CompensationDesigner.cs
- LeafCellTreeNode.cs
- RealizationContext.cs
- DataSourceXmlSerializationAttribute.cs
- Binding.cs
- HideDisabledControlAdapter.cs
- DATA_BLOB.cs
- CheckBoxRenderer.cs
- ScriptResourceInfo.cs
- BuildTopDownAttribute.cs
- CodeNamespace.cs
- StyleCollection.cs
- CompilerErrorCollection.cs
- BulletedListEventArgs.cs
- EntityClientCacheEntry.cs
- GridView.cs
- DifferencingCollection.cs
- SqlDataSourceCache.cs
- HttpProtocolImporter.cs
- XmlSerializationWriter.cs
- QilValidationVisitor.cs
- Stopwatch.cs
- ExecutorLocksHeldException.cs
- DataGridParentRows.cs
- WebPartMenu.cs
- TransformConverter.cs
- CacheVirtualItemsEvent.cs
- ClientSettingsProvider.cs
- ShaperBuffers.cs
- MethodBuilder.cs
- thaishape.cs
- FlowNode.cs
- Control.cs
- GroupItemAutomationPeer.cs
- ClickablePoint.cs
- LineBreakRecord.cs
- ObjectAssociationEndMapping.cs
- CaseInsensitiveOrdinalStringComparer.cs
- SqlProvider.cs
- CheckBoxDesigner.cs
- ErrorProvider.cs
- HotSpotCollection.cs
- MimeReturn.cs
- BamlRecordReader.cs
- hebrewshape.cs
- ZoneButton.cs
- HtmlMobileTextWriter.cs
- WsdlWriter.cs
- ProfileEventArgs.cs
- CodeDelegateCreateExpression.cs
- remotingproxy.cs
- XmlSchemaObjectTable.cs
- RepeatBehavior.cs
- AutoGeneratedField.cs
- PhysicalOps.cs
- PropertyGridCommands.cs
- WebResourceUtil.cs
- CustomWebEventKey.cs
- DataColumnSelectionConverter.cs
- CompiledIdentityConstraint.cs
- Function.cs
- BamlCollectionHolder.cs
- HtmlInputSubmit.cs
- SqlCacheDependencyDatabaseCollection.cs
- SqlDataSourceSelectingEventArgs.cs
- SiteIdentityPermission.cs
- TypeReference.cs
- SystemColorTracker.cs
- Directory.cs
- ControlCommandSet.cs
- DispatcherProcessingDisabled.cs
- ClientSettingsProvider.cs
- MatrixConverter.cs
- VarRemapper.cs
- TextRange.cs
- CompilerTypeWithParams.cs
- NGCSerializerAsync.cs
- Panel.cs
- Pair.cs
- SymbolEqualComparer.cs
- SystemPens.cs
- SaveFileDialog.cs
- UserPreferenceChangedEventArgs.cs
- CodeSnippetCompileUnit.cs
- FactoryGenerator.cs
- EntityContainerEntitySet.cs
- HttpSessionStateBase.cs
- ExpressionCopier.cs
- SchemaElementDecl.cs
- MetafileHeaderEmf.cs
- XmlDataSourceView.cs
- RenderCapability.cs
- AttributeUsageAttribute.cs
- CategoryGridEntry.cs
- MetadataItemEmitter.cs
- WebRequestModuleElement.cs
- PositiveTimeSpanValidatorAttribute.cs
- KeyGestureValueSerializer.cs