Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / xsp / System / Web / Extensions / ui / WebResourceUtil.cs / 1 / WebResourceUtil.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Reflection; using System.Diagnostics; using System.Collections; using System.Globalization; using System.Web.UI; using System.Web.Resources; using System.Web.Util; internal static class WebResourceUtil { // Maps Pair(resourceName, assembly) to bool private static readonly Hashtable _assemblyContainsWebResourceCache = Hashtable.Synchronized(new Hashtable()); // Returns true if the assembly contains a Web resource and an embedded resource with // the sepecified name. Throws exception if assembly contains Web resource but no // embedded resource, since this is always an error. public static bool AssemblyContainsWebResource(Assembly assembly, string resourceName) { // PERF: Special-case known resources in our own assembly if (assembly == AssemblyCache.SystemWebExtensions) { return SystemWebExtensionsContainsWebResource(resourceName); } // Getting and checking the custom attributes is expensive, so we cache the result // of the lookup. Pair key = new Pair (resourceName, assembly); object assemblyContainsWebResource = _assemblyContainsWebResourceCache[key]; if (assemblyContainsWebResource == null) { assemblyContainsWebResource = false; object[] attrs = assembly.GetCustomAttributes(typeof(WebResourceAttribute), false); foreach (WebResourceAttribute attr in attrs) { // Resource names are always case-sensitive if (String.Equals(attr.WebResource, resourceName, StringComparison.Ordinal)) { if (assembly.GetManifestResourceStream(resourceName) != null) { assemblyContainsWebResource = true; break; } else { // Always an error to contain Web resource but not embedded resource. throw new InvalidOperationException(String.Format( CultureInfo.CurrentUICulture, AtlasWeb.WebResourceUtil_AssemblyDoesNotContainEmbeddedResource, assembly, resourceName)); } } } _assemblyContainsWebResourceCache[key] = assemblyContainsWebResource; } return (bool)assemblyContainsWebResource; } private static bool SystemWebExtensionsContainsWebResource(string resourceName) { // PERF: Switching over the length is more performant than switching over the string itself // or checking equality against each string. When switching over the string itself, the switch // is compiled to a lookup in a static Dictionary , which is 5-10 times slower than // switching over the length. Checking equality against each string ranges from equal performance // to 10 times slower, depending on how early a match is found. switch (resourceName.Length) { case 16: return resourceName == "MicrosoftAjax.js"; case 24: return resourceName == "MicrosoftAjaxWebForms.js"; case 21: return resourceName == "MicrosoftAjaxTimer.js"; case 22: return resourceName == "MicrosoftAjax.debug.js"; case 30: return resourceName == "MicrosoftAjaxWebForms.debug.js"; case 33: return resourceName == "MicrosoftAjaxDataService.debug.js"; case 27: return resourceName == "MicrosoftAjaxTimer.debug.js" || resourceName == "MicrosoftAjaxDataService.js"; default: return false; } } // Throws exception if the assembly does not contain a Web resource and an embedded resource // with the specified name. public static void VerifyAssemblyContainsReleaseWebResource(Assembly assembly, string releaseResourceName) { if (!AssemblyContainsWebResource(assembly, releaseResourceName)) { throw new InvalidOperationException(String.Format( CultureInfo.CurrentUICulture, AtlasWeb.WebResourceUtil_AssemblyDoesNotContainReleaseWebResource, assembly, releaseResourceName)); } } // Throws exception if the assembly does not contain a Web resource and an embedded resource // with the specified name. public static void VerifyAssemblyContainsDebugWebResource(Assembly assembly, string debugResourceName) { if (!AssemblyContainsWebResource(assembly, debugResourceName)) { throw new InvalidOperationException(String.Format( CultureInfo.CurrentUICulture, AtlasWeb.WebResourceUtil_AssemblyDoesNotContainDebugWebResource, assembly, debugResourceName)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Reflection; using System.Diagnostics; using System.Collections; using System.Globalization; using System.Web.UI; using System.Web.Resources; using System.Web.Util; internal static class WebResourceUtil { // Maps Pair(resourceName, assembly) to bool private static readonly Hashtable _assemblyContainsWebResourceCache = Hashtable.Synchronized(new Hashtable()); // Returns true if the assembly contains a Web resource and an embedded resource with // the sepecified name. Throws exception if assembly contains Web resource but no // embedded resource, since this is always an error. public static bool AssemblyContainsWebResource(Assembly assembly, string resourceName) { // PERF: Special-case known resources in our own assembly if (assembly == AssemblyCache.SystemWebExtensions) { return SystemWebExtensionsContainsWebResource(resourceName); } // Getting and checking the custom attributes is expensive, so we cache the result // of the lookup. Pair key = new Pair (resourceName, assembly); object assemblyContainsWebResource = _assemblyContainsWebResourceCache[key]; if (assemblyContainsWebResource == null) { assemblyContainsWebResource = false; object[] attrs = assembly.GetCustomAttributes(typeof(WebResourceAttribute), false); foreach (WebResourceAttribute attr in attrs) { // Resource names are always case-sensitive if (String.Equals(attr.WebResource, resourceName, StringComparison.Ordinal)) { if (assembly.GetManifestResourceStream(resourceName) != null) { assemblyContainsWebResource = true; break; } else { // Always an error to contain Web resource but not embedded resource. throw new InvalidOperationException(String.Format( CultureInfo.CurrentUICulture, AtlasWeb.WebResourceUtil_AssemblyDoesNotContainEmbeddedResource, assembly, resourceName)); } } } _assemblyContainsWebResourceCache[key] = assemblyContainsWebResource; } return (bool)assemblyContainsWebResource; } private static bool SystemWebExtensionsContainsWebResource(string resourceName) { // PERF: Switching over the length is more performant than switching over the string itself // or checking equality against each string. When switching over the string itself, the switch // is compiled to a lookup in a static Dictionary , which is 5-10 times slower than // switching over the length. Checking equality against each string ranges from equal performance // to 10 times slower, depending on how early a match is found. switch (resourceName.Length) { case 16: return resourceName == "MicrosoftAjax.js"; case 24: return resourceName == "MicrosoftAjaxWebForms.js"; case 21: return resourceName == "MicrosoftAjaxTimer.js"; case 22: return resourceName == "MicrosoftAjax.debug.js"; case 30: return resourceName == "MicrosoftAjaxWebForms.debug.js"; case 33: return resourceName == "MicrosoftAjaxDataService.debug.js"; case 27: return resourceName == "MicrosoftAjaxTimer.debug.js" || resourceName == "MicrosoftAjaxDataService.js"; default: return false; } } // Throws exception if the assembly does not contain a Web resource and an embedded resource // with the specified name. public static void VerifyAssemblyContainsReleaseWebResource(Assembly assembly, string releaseResourceName) { if (!AssemblyContainsWebResource(assembly, releaseResourceName)) { throw new InvalidOperationException(String.Format( CultureInfo.CurrentUICulture, AtlasWeb.WebResourceUtil_AssemblyDoesNotContainReleaseWebResource, assembly, releaseResourceName)); } } // Throws exception if the assembly does not contain a Web resource and an embedded resource // with the specified name. public static void VerifyAssemblyContainsDebugWebResource(Assembly assembly, string debugResourceName) { if (!AssemblyContainsWebResource(assembly, debugResourceName)) { throw new InvalidOperationException(String.Format( CultureInfo.CurrentUICulture, AtlasWeb.WebResourceUtil_AssemblyDoesNotContainDebugWebResource, assembly, debugResourceName)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
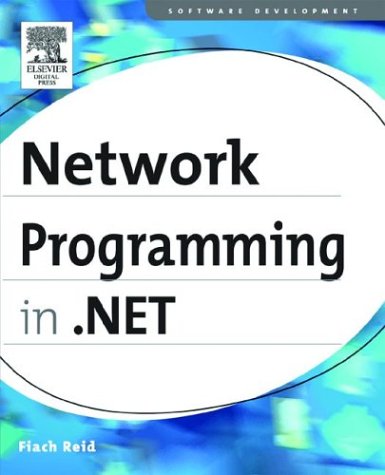
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextFindEngine.cs
- ListItemCollection.cs
- ConfigurationStrings.cs
- _PooledStream.cs
- XmlnsDefinitionAttribute.cs
- SByte.cs
- Internal.cs
- TextBoxBase.cs
- PersonalizationDictionary.cs
- ConfigXmlSignificantWhitespace.cs
- NonClientArea.cs
- WorkflowMarkupSerializationException.cs
- GlobalDataBindingHandler.cs
- Point4D.cs
- BinaryQueryOperator.cs
- Regex.cs
- X509SecurityToken.cs
- ProtocolViolationException.cs
- Compress.cs
- BevelBitmapEffect.cs
- ExtensionQuery.cs
- Query.cs
- DateTimeConverter.cs
- Misc.cs
- XmlSortKey.cs
- ApplyImportsAction.cs
- SqlIdentifier.cs
- MutexSecurity.cs
- SoapObjectWriter.cs
- ToolBarPanel.cs
- StateChangeEvent.cs
- BasePropertyDescriptor.cs
- DoubleAnimationUsingPath.cs
- returneventsaver.cs
- OleDbParameterCollection.cs
- StylusPointPropertyInfoDefaults.cs
- ComPlusContractBehavior.cs
- LocatorPartList.cs
- Compiler.cs
- RegexStringValidator.cs
- KeyInfo.cs
- StylusDevice.cs
- uribuilder.cs
- TextLineResult.cs
- DbDataReader.cs
- StructuredTypeInfo.cs
- Variable.cs
- StreamInfo.cs
- SerializerWriterEventHandlers.cs
- AssertFilter.cs
- BindingListCollectionView.cs
- HttpStaticObjectsCollectionBase.cs
- NamespaceEmitter.cs
- LinearGradientBrush.cs
- XmlSchemaType.cs
- UTF7Encoding.cs
- FlowDocumentReader.cs
- externdll.cs
- List.cs
- UpdatePanelTriggerCollection.cs
- PeerNameRecordCollection.cs
- OutputCacheProfileCollection.cs
- EventsTab.cs
- GenericEnumerator.cs
- MouseGestureValueSerializer.cs
- ChangeInterceptorAttribute.cs
- DesignerTransaction.cs
- loginstatus.cs
- ReadOnlyAttribute.cs
- XmlSerializerNamespaces.cs
- PreProcessor.cs
- ListBox.cs
- InternalCache.cs
- QilInvoke.cs
- CasesDictionary.cs
- HealthMonitoringSection.cs
- ListViewAutomationPeer.cs
- CompleteWizardStep.cs
- StrokeIntersection.cs
- CodeGen.cs
- SqlDataSourceCommandEventArgs.cs
- DiffuseMaterial.cs
- NestPullup.cs
- MailWebEventProvider.cs
- initElementDictionary.cs
- Gdiplus.cs
- CodeAttributeDeclaration.cs
- EntityClassGenerator.cs
- RijndaelManaged.cs
- ToggleProviderWrapper.cs
- Axis.cs
- ErrorFormatter.cs
- DateTimeFormat.cs
- CommandBinding.cs
- SafeThemeHandle.cs
- DataErrorValidationRule.cs
- InitializingNewItemEventArgs.cs
- control.ime.cs
- TriggerActionCollection.cs
- CompressEmulationStream.cs