Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Security / Cryptography / RijndaelManaged.cs / 1305376 / RijndaelManaged.cs
using System.Diagnostics.Contracts; // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // // // RijndaelManaged.cs // namespace System.Security.Cryptography { [System.Runtime.InteropServices.ComVisible(true)] public sealed class RijndaelManaged : Rijndael { public RijndaelManaged () { #if FEATURE_CRYPTO if (CryptoConfig.AllowOnlyFipsAlgorithms) throw new InvalidOperationException(Environment.GetResourceString("Cryptography_NonCompliantFIPSAlgorithm")); Contract.EndContractBlock(); #endif // FEATURE_CRYPTO } // #CoreCLRRijndaelModes // // On CoreCLR we limit the supported cipher modes and padding modes for the AES algorithm to a // single hard coded value. This allows us to remove a lot of code by removing support for the // uncommon cases and forcing everyone to use the same common padding and ciper modes: // // - CipherMode: CipherMode.CBC // - PaddingMode: PaddingMode.PKCS7 public override ICryptoTransform CreateEncryptor (byte[] rgbKey, byte[] rgbIV) { return NewEncryptor(rgbKey, ModeValue, rgbIV, FeedbackSizeValue, RijndaelManagedTransformMode.Encrypt); } public override ICryptoTransform CreateDecryptor (byte[] rgbKey, byte[] rgbIV) { return NewEncryptor(rgbKey, ModeValue, rgbIV, FeedbackSizeValue, RijndaelManagedTransformMode.Decrypt); } public override void GenerateKey () { KeyValue = Utils.GenerateRandom(KeySizeValue / 8); } public override void GenerateIV () { IVValue = Utils.GenerateRandom(BlockSizeValue / 8); } private ICryptoTransform NewEncryptor (byte[] rgbKey, CipherMode mode, byte[] rgbIV, int feedbackSize, RijndaelManagedTransformMode encryptMode) { // Build the key if one does not already exist if (rgbKey == null) { rgbKey = Utils.GenerateRandom(KeySizeValue / 8); } // If not ECB mode, make sure we have an IV. In CoreCLR we do not support ECB, so we must have // an IV in all cases. #if !FEATURE_CRYPTO if (mode != CipherMode.ECB) { #endif // !FEATURE_CRYPTO if (rgbIV == null) { rgbIV = Utils.GenerateRandom(BlockSizeValue / 8); } #if !FEATURE_CRYPTO } #endif // !FEATURE_CRYPTO // Create the encryptor/decryptor object return new RijndaelManagedTransform (rgbKey, mode, rgbIV, BlockSizeValue, feedbackSize, PaddingValue, encryptMode); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System.Diagnostics.Contracts; // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // // // RijndaelManaged.cs // namespace System.Security.Cryptography { [System.Runtime.InteropServices.ComVisible(true)] public sealed class RijndaelManaged : Rijndael { public RijndaelManaged () { #if FEATURE_CRYPTO if (CryptoConfig.AllowOnlyFipsAlgorithms) throw new InvalidOperationException(Environment.GetResourceString("Cryptography_NonCompliantFIPSAlgorithm")); Contract.EndContractBlock(); #endif // FEATURE_CRYPTO } // #CoreCLRRijndaelModes // // On CoreCLR we limit the supported cipher modes and padding modes for the AES algorithm to a // single hard coded value. This allows us to remove a lot of code by removing support for the // uncommon cases and forcing everyone to use the same common padding and ciper modes: // // - CipherMode: CipherMode.CBC // - PaddingMode: PaddingMode.PKCS7 public override ICryptoTransform CreateEncryptor (byte[] rgbKey, byte[] rgbIV) { return NewEncryptor(rgbKey, ModeValue, rgbIV, FeedbackSizeValue, RijndaelManagedTransformMode.Encrypt); } public override ICryptoTransform CreateDecryptor (byte[] rgbKey, byte[] rgbIV) { return NewEncryptor(rgbKey, ModeValue, rgbIV, FeedbackSizeValue, RijndaelManagedTransformMode.Decrypt); } public override void GenerateKey () { KeyValue = Utils.GenerateRandom(KeySizeValue / 8); } public override void GenerateIV () { IVValue = Utils.GenerateRandom(BlockSizeValue / 8); } private ICryptoTransform NewEncryptor (byte[] rgbKey, CipherMode mode, byte[] rgbIV, int feedbackSize, RijndaelManagedTransformMode encryptMode) { // Build the key if one does not already exist if (rgbKey == null) { rgbKey = Utils.GenerateRandom(KeySizeValue / 8); } // If not ECB mode, make sure we have an IV. In CoreCLR we do not support ECB, so we must have // an IV in all cases. #if !FEATURE_CRYPTO if (mode != CipherMode.ECB) { #endif // !FEATURE_CRYPTO if (rgbIV == null) { rgbIV = Utils.GenerateRandom(BlockSizeValue / 8); } #if !FEATURE_CRYPTO } #endif // !FEATURE_CRYPTO // Create the encryptor/decryptor object return new RijndaelManagedTransform (rgbKey, mode, rgbIV, BlockSizeValue, feedbackSize, PaddingValue, encryptMode); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
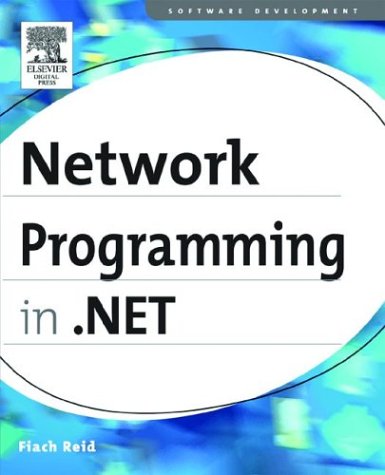
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RemoteWebConfigurationHostServer.cs
- EmptyEnumerator.cs
- HtmlTextArea.cs
- InputMethodStateChangeEventArgs.cs
- DragSelectionMessageFilter.cs
- URL.cs
- SimpleHandlerFactory.cs
- WorkflowWebHostingModule.cs
- FacetChecker.cs
- PerSessionInstanceContextProvider.cs
- Region.cs
- SqlConnectionHelper.cs
- SqlTrackingWorkflowInstance.cs
- ResXResourceWriter.cs
- QuaternionRotation3D.cs
- GetMemberBinder.cs
- MsmqHostedTransportManager.cs
- DataService.cs
- TriggerBase.cs
- UInt32.cs
- GridViewUpdatedEventArgs.cs
- DataSourceDescriptorCollection.cs
- MimeMultiPart.cs
- AdCreatedEventArgs.cs
- InfoCardBinaryReader.cs
- EpmTargetPathSegment.cs
- ProxyGenerationError.cs
- ArgumentNullException.cs
- HierarchicalDataTemplate.cs
- DecoderReplacementFallback.cs
- UserMapPath.cs
- TaiwanLunisolarCalendar.cs
- DataContractSerializerOperationBehavior.cs
- XmlValueConverter.cs
- ConstrainedDataObject.cs
- AbandonedMutexException.cs
- OdbcError.cs
- HwndAppCommandInputProvider.cs
- InitializeCorrelation.cs
- DataGridViewButtonColumn.cs
- CompModSwitches.cs
- HitTestParameters.cs
- DocumentPageHost.cs
- RadioButtonBaseAdapter.cs
- Vector3DAnimation.cs
- RequestTimeoutManager.cs
- StyleXamlParser.cs
- Executor.cs
- CompilerWrapper.cs
- ElementProxy.cs
- ListenerAdapterBase.cs
- CellCreator.cs
- LocatorPartList.cs
- PointAnimationBase.cs
- TransformerInfo.cs
- PageTheme.cs
- WebPartConnectionsCloseVerb.cs
- InheritablePropertyChangeInfo.cs
- KeyInterop.cs
- BufferedGraphicsContext.cs
- PropertyMapper.cs
- Win32Native.cs
- PageContentCollection.cs
- HtmlAnchor.cs
- PropertyAccessVisitor.cs
- HttpHostedTransportConfiguration.cs
- XmlCharType.cs
- BufferModeSettings.cs
- EnvelopedPkcs7.cs
- TreeNodeSelectionProcessor.cs
- XamlReaderHelper.cs
- BasicExpressionVisitor.cs
- ConfigXmlDocument.cs
- ValidatingReaderNodeData.cs
- BitmapEffectDrawing.cs
- ListView.cs
- DataGridViewColumnConverter.cs
- LineMetrics.cs
- ZipIOExtraFieldPaddingElement.cs
- CatalogUtil.cs
- BevelBitmapEffect.cs
- SQLMembershipProvider.cs
- WebPartActionVerb.cs
- TransactionCache.cs
- Quaternion.cs
- UnsettableComboBox.cs
- TextElementEnumerator.cs
- PathParser.cs
- CodeGeneratorOptions.cs
- RoleManagerModule.cs
- DropShadowBitmapEffect.cs
- DrawingVisual.cs
- AttachedPropertyBrowsableAttribute.cs
- HttpConfigurationContext.cs
- IntersectQueryOperator.cs
- TextModifier.cs
- graph.cs
- XmlSchemaSimpleContentExtension.cs
- Table.cs
- NativeRightsManagementAPIsStructures.cs