Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / documents / DocumentPageHost.cs / 1305600 / DocumentPageHost.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: DocumentPageHost.cs // // Description: Provides a view port for a page of content for a DocumentPage. // //--------------------------------------------------------------------------- using System; using System.Windows; // UIElement using System.Windows.Media; // Visual namespace MS.Internal.Documents { ////// Provides a view port for a page of content for a DocumentPage. /// internal class DocumentPageHost : FrameworkElement { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Create an instance of a DocumentPageHost. /// internal DocumentPageHost() : base() { } #endregion Constructors //-------------------------------------------------------------------- // // Internal Methods // //------------------------------------------------------------------- #region Internal Methods internal static void DisconnectPageVisual(Visual pageVisual) { // There might be a case where a visual associated with a page was // inserted to a visual tree before. It got removed later, but GC did not // destroy its parent yet. To workaround this case always check for the parent // of page visual and disconnect it, when necessary. Visual currentParent = VisualTreeHelper.GetParent(pageVisual) as Visual; if (currentParent != null) { ContainerVisual pageVisualHost = currentParent as ContainerVisual; if (pageVisualHost == null) throw new ArgumentException(SR.Get(SRID.DocumentPageView_ParentNotDocumentPageHost), "pageVisual"); DocumentPageHost docPageHost = VisualTreeHelper.GetParent(pageVisualHost) as DocumentPageHost; if (docPageHost == null) throw new ArgumentException(SR.Get(SRID.DocumentPageView_ParentNotDocumentPageHost), "pageVisual"); docPageHost.PageVisual = null; } } #endregion Internal Methods //-------------------------------------------------------------------- // // Internal Properties // //-------------------------------------------------------------------- #region Internal Properties ////// Root of visual subtree hosted by this DocumentPageHost. /// internal Visual PageVisual { get { return _pageVisual; } set { ContainerVisual pageVisualHost; if (_pageVisual != null) { pageVisualHost = VisualTreeHelper.GetParent(_pageVisual) as ContainerVisual; Invariant.Assert(pageVisualHost != null); pageVisualHost.Children.Clear(); this.RemoveVisualChild(pageVisualHost); } _pageVisual = value; if (_pageVisual != null) { pageVisualHost = new ContainerVisual(); this.AddVisualChild(pageVisualHost); pageVisualHost.Children.Add(_pageVisual); pageVisualHost.SetValue(FlowDirectionProperty, FlowDirection.LeftToRight); } } } ////// Internal cached offset. /// internal Point CachedOffset; #endregion Internal Properties #region VisualChildren ////// Derived class must implement to support Visual children. The method must return /// the child at the specified index. Index must be between 0 and GetVisualChildrenCount-1. /// /// By default a Visual does not have any children. /// /// Remark: /// During this virtual call it is not valid to modify the Visual tree. /// protected override Visual GetVisualChild(int index) { if (index != 0 || _pageVisual == null) { throw new ArgumentOutOfRangeException("index", index, SR.Get(SRID.Visual_ArgumentOutOfRange)); } return VisualTreeHelper.GetParent(_pageVisual) as Visual; } ////// Derived classes override this property to enable the Visual code to enumerate /// the Visual children. Derived classes need to return the number of children /// from this method. /// /// By default a Visual does not have any children. /// /// Remark: During this virtual method the Visual tree must not be modified. /// protected override int VisualChildrenCount { get { return _pageVisual != null ? 1 : 0; } } #endregion VisualChildren private Visual _pageVisual; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: DocumentPageHost.cs // // Description: Provides a view port for a page of content for a DocumentPage. // //--------------------------------------------------------------------------- using System; using System.Windows; // UIElement using System.Windows.Media; // Visual namespace MS.Internal.Documents { ////// Provides a view port for a page of content for a DocumentPage. /// internal class DocumentPageHost : FrameworkElement { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Create an instance of a DocumentPageHost. /// internal DocumentPageHost() : base() { } #endregion Constructors //-------------------------------------------------------------------- // // Internal Methods // //------------------------------------------------------------------- #region Internal Methods internal static void DisconnectPageVisual(Visual pageVisual) { // There might be a case where a visual associated with a page was // inserted to a visual tree before. It got removed later, but GC did not // destroy its parent yet. To workaround this case always check for the parent // of page visual and disconnect it, when necessary. Visual currentParent = VisualTreeHelper.GetParent(pageVisual) as Visual; if (currentParent != null) { ContainerVisual pageVisualHost = currentParent as ContainerVisual; if (pageVisualHost == null) throw new ArgumentException(SR.Get(SRID.DocumentPageView_ParentNotDocumentPageHost), "pageVisual"); DocumentPageHost docPageHost = VisualTreeHelper.GetParent(pageVisualHost) as DocumentPageHost; if (docPageHost == null) throw new ArgumentException(SR.Get(SRID.DocumentPageView_ParentNotDocumentPageHost), "pageVisual"); docPageHost.PageVisual = null; } } #endregion Internal Methods //-------------------------------------------------------------------- // // Internal Properties // //-------------------------------------------------------------------- #region Internal Properties ////// Root of visual subtree hosted by this DocumentPageHost. /// internal Visual PageVisual { get { return _pageVisual; } set { ContainerVisual pageVisualHost; if (_pageVisual != null) { pageVisualHost = VisualTreeHelper.GetParent(_pageVisual) as ContainerVisual; Invariant.Assert(pageVisualHost != null); pageVisualHost.Children.Clear(); this.RemoveVisualChild(pageVisualHost); } _pageVisual = value; if (_pageVisual != null) { pageVisualHost = new ContainerVisual(); this.AddVisualChild(pageVisualHost); pageVisualHost.Children.Add(_pageVisual); pageVisualHost.SetValue(FlowDirectionProperty, FlowDirection.LeftToRight); } } } ////// Internal cached offset. /// internal Point CachedOffset; #endregion Internal Properties #region VisualChildren ////// Derived class must implement to support Visual children. The method must return /// the child at the specified index. Index must be between 0 and GetVisualChildrenCount-1. /// /// By default a Visual does not have any children. /// /// Remark: /// During this virtual call it is not valid to modify the Visual tree. /// protected override Visual GetVisualChild(int index) { if (index != 0 || _pageVisual == null) { throw new ArgumentOutOfRangeException("index", index, SR.Get(SRID.Visual_ArgumentOutOfRange)); } return VisualTreeHelper.GetParent(_pageVisual) as Visual; } ////// Derived classes override this property to enable the Visual code to enumerate /// the Visual children. Derived classes need to return the number of children /// from this method. /// /// By default a Visual does not have any children. /// /// Remark: During this virtual method the Visual tree must not be modified. /// protected override int VisualChildrenCount { get { return _pageVisual != null ? 1 : 0; } } #endregion VisualChildren private Visual _pageVisual; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
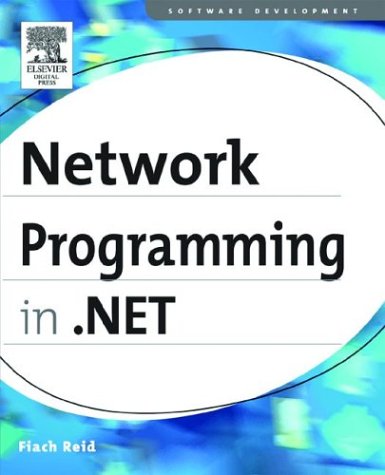
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IPPacketInformation.cs
- XmlAnyElementAttributes.cs
- TraceHandlerErrorFormatter.cs
- NavigationFailedEventArgs.cs
- Win32KeyboardDevice.cs
- GroupBoxRenderer.cs
- PathFigure.cs
- DataBindingsDialog.cs
- QuestionEventArgs.cs
- SecurityToken.cs
- XPathScanner.cs
- ExtensionFile.cs
- StringPropertyBuilder.cs
- PathFigureCollection.cs
- EntityWithKeyStrategy.cs
- FastEncoder.cs
- DataControlField.cs
- Int64AnimationUsingKeyFrames.cs
- HttpListenerException.cs
- TreeViewEvent.cs
- SystemUdpStatistics.cs
- XmlCharCheckingReader.cs
- MenuItemStyleCollection.cs
- ServicePointManagerElement.cs
- SessionStateModule.cs
- SplitterPanel.cs
- UpdatePanelControlTrigger.cs
- DelegateBodyWriter.cs
- NameValueFileSectionHandler.cs
- FileDialogPermission.cs
- SelectionHighlightInfo.cs
- Delegate.cs
- UnsettableComboBox.cs
- ThreadInterruptedException.cs
- Action.cs
- WebPartConnectionsDisconnectVerb.cs
- LinkUtilities.cs
- VirtualPathExtension.cs
- FullTextLine.cs
- SBCSCodePageEncoding.cs
- ObjectContextServiceProvider.cs
- ResourceBinder.cs
- ComboBox.cs
- BaseConfigurationRecord.cs
- WindowsFormsSynchronizationContext.cs
- ValidatedControlConverter.cs
- TransformerTypeCollection.cs
- Style.cs
- COAUTHINFO.cs
- altserialization.cs
- OpacityConverter.cs
- TransformPattern.cs
- ListBase.cs
- XmlException.cs
- SendKeys.cs
- SessionPageStateSection.cs
- XmlCharType.cs
- ExclusiveCanonicalizationTransform.cs
- FieldToken.cs
- GenericParameterDataContract.cs
- FileUpload.cs
- SizeValueSerializer.cs
- DetailsViewInsertEventArgs.cs
- OdbcConnectionPoolProviderInfo.cs
- FixedFlowMap.cs
- Tuple.cs
- CodeExpressionRuleDeclaration.cs
- ValidatorAttribute.cs
- DecoderExceptionFallback.cs
- TraceSwitch.cs
- GatewayDefinition.cs
- ValidateNames.cs
- ViewManager.cs
- PackageStore.cs
- BlockCollection.cs
- TabPage.cs
- VisualStyleInformation.cs
- TableCell.cs
- EnvironmentPermission.cs
- CriticalFinalizerObject.cs
- WindowsFormsSectionHandler.cs
- ProfileService.cs
- MediaElementAutomationPeer.cs
- AutoCompleteStringCollection.cs
- sqlser.cs
- DataMisalignedException.cs
- HtmlEmptyTagControlBuilder.cs
- ToolStripItem.cs
- HttpBindingExtension.cs
- RoleManagerEventArgs.cs
- OutputScopeManager.cs
- DbConnectionPool.cs
- UInt32Converter.cs
- DataGridViewRowErrorTextNeededEventArgs.cs
- XmlElementList.cs
- PropertyMapper.cs
- AttachInfo.cs
- ProxyElement.cs
- DataStorage.cs
- DetailsViewRowCollection.cs