Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / SplitterPanel.cs / 1305376 / SplitterPanel.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using Microsoft.Win32; using System; using System.ComponentModel; using System.Diagnostics; using System.Security; using System.Security.Permissions; using System.Windows.Forms; using System.Drawing; using System.Drawing.Design; using System.Drawing.Imaging; using System.Runtime.InteropServices; using System.ComponentModel.Design; using System.ComponentModel.Design.Serialization; ////// /// TBD. /// [ ComVisible(true), ClassInterface(ClassInterfaceType.AutoDispatch), Docking(DockingBehavior.Never), Designer("System.Windows.Forms.Design.SplitterPanelDesigner, " + AssemblyRef.SystemDesign), ToolboxItem(false) ] public sealed class SplitterPanel : Panel { SplitContainer owner = null; private bool collapsed = false; ///public SplitterPanel(SplitContainer owner) : base() { this.owner = owner; SetStyle(ControlStyles.ResizeRedraw, true); } internal bool Collapsed { get { return collapsed; } set { collapsed = value; } } /// /// /// Override AutoSize to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new bool AutoSize { get { return base.AutoSize; } set { base.AutoSize = value; } } ///[Browsable(false), EditorBrowsable(EditorBrowsableState.Never)] new public event EventHandler AutoSizeChanged { add { base.AutoSizeChanged += value; } remove { base.AutoSizeChanged -= value; } } /// /// Allows the control to optionally shrink when AutoSize is true. /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false), Localizable(false) ] public override AutoSizeMode AutoSizeMode { get { return AutoSizeMode.GrowOnly; } set { } } ////// /// Override Anchor to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new AnchorStyles Anchor { get { return base.Anchor; } set { base.Anchor = value; } } ////// /// Indicates what type of border the Splitter control has. This value /// comes from the System.Windows.Forms.BorderStyle enumeration. /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new BorderStyle BorderStyle { get { return base.BorderStyle; } set { base.BorderStyle = value; } } ////// /// The dock property. The dock property controls to which edge /// of the container this control is docked to. For example, when docked to /// the top of the container, the control will be displayed flush at the /// top of the container, extending the length of the container. /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new DockStyle Dock { get { return base.Dock; } set { base.Dock = value; } } ////// /// Override DockPadding to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] new public DockPaddingEdges DockPadding { get { return base.DockPadding; } } ////// /// The height of this SplitterPanel /// [ SRCategory(SR.CatLayout), Browsable(false), EditorBrowsable(EditorBrowsableState.Always), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ControlHeightDescr) ] public new int Height { get { if (Collapsed) { return 0; } return base.Height; } set { throw new NotSupportedException(SR.GetString(SR.SplitContainerPanelHeight)); } } internal int HeightInternal { get { return ((Panel)this).Height; } set { ((Panel)this).Height = value; } } ////// /// Override Location to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new Point Location { get { return base.Location; } set { base.Location = value; } } ////// /// Deriving classes can override this to configure a default size for their control. /// This is more efficient than setting the size in the control's constructor. /// protected override Padding DefaultMargin { get { return new Padding(0, 0, 0, 0); } } ////// /// Override AutoSize to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new Size MinimumSize { get { return base.MinimumSize; } set { base.MinimumSize = value; } } ////// /// Override AutoSize to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new Size MaximumSize { get { return base.MaximumSize; } set { base.MaximumSize = value; } } ////// /// Name of this control. The designer will set this to the same /// as the programatic Id "(name)" of the control. The name can be /// used as a key into the ControlCollection. /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new string Name { get { return base.Name; } set { base.Name = value; } } ////// /// The parent of this control. /// internal SplitContainer Owner { get { return owner; } } ////// /// The parent of this control. /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new Control Parent { get { return base.Parent; } set { base.Parent = value; } } ////// /// Override Size to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new Size Size { get { if (Collapsed) { return Size.Empty; } return base.Size; } set { base.Size = value; } } ////// /// Override TabIndex to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new int TabIndex { get { return base.TabIndex; } set { base.TabIndex = value; } } ////// /// Override TabStop to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new bool TabStop { get { return base.TabStop; } set { base.TabStop = value; } } ////// /// Override Visible to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new bool Visible { get { return base.Visible; } set { base.Visible = value; } } ////// /// The width of this control. /// [ SRCategory(SR.CatLayout), Browsable(false), EditorBrowsable(EditorBrowsableState.Always), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ControlWidthDescr) ] public new int Width { get { if (Collapsed) { return 0; } return base.Width; } set { throw new NotSupportedException(SR.GetString(SR.SplitContainerPanelWidth)); } } internal int WidthInternal { get { return ((Panel)this).Width; } set { ((Panel)this).Width = value; } } ////// /// Override VisibleChanged to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new event EventHandler VisibleChanged { add { base.VisibleChanged += value; } remove { base.VisibleChanged -= value; } } ////// /// Override DockChanged to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new event EventHandler DockChanged { add { base.DockChanged += value; } remove { base.DockChanged -= value; } } ////// /// Override LocationChanged to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new event EventHandler LocationChanged { add { base.LocationChanged += value; } remove { base.LocationChanged -= value; } } ////// /// Override TabIndexChanged to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new event EventHandler TabIndexChanged { add { base.TabIndexChanged += value; } remove { base.TabIndexChanged -= value; } } ////// /// Override TabStopChanged to make it hidden from the user in the designer /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false) ] public new event EventHandler TabStopChanged { add { base.TabStopChanged += value; } remove { base.TabStopChanged -= value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
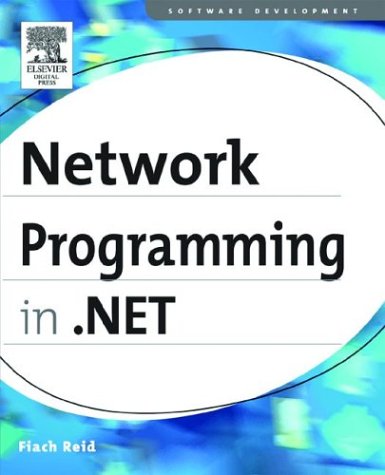
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IProvider.cs
- Hashtable.cs
- ProtocolsConfigurationHandler.cs
- DbUpdateCommandTree.cs
- Setter.cs
- AttributeTable.cs
- EdmError.cs
- HtmlElement.cs
- String.cs
- StorageEndPropertyMapping.cs
- _Events.cs
- ArrayItemValue.cs
- DropShadowEffect.cs
- FormViewUpdatedEventArgs.cs
- WindowsContainer.cs
- EndEvent.cs
- CatalogPart.cs
- RuntimeHelpers.cs
- RefType.cs
- IItemProperties.cs
- PipeStream.cs
- BooleanAnimationBase.cs
- XmlNotation.cs
- EntityDataSourceWrapperPropertyDescriptor.cs
- TextServicesManager.cs
- ReadOnlyNameValueCollection.cs
- MimeXmlReflector.cs
- bindurihelper.cs
- PersistChildrenAttribute.cs
- MultiView.cs
- PersonalizableAttribute.cs
- XdrBuilder.cs
- RequestSecurityToken.cs
- SystemColorTracker.cs
- DiagnosticTraceSource.cs
- DynamicDataRouteHandler.cs
- TripleDESCryptoServiceProvider.cs
- MouseWheelEventArgs.cs
- GenericEnumConverter.cs
- Stroke.cs
- ResourceIDHelper.cs
- EncryptedReference.cs
- UserControlAutomationPeer.cs
- MsmqIntegrationProcessProtocolHandler.cs
- VoiceObjectToken.cs
- StylusPointPropertyInfo.cs
- XmlDataSource.cs
- SqlClientWrapperSmiStreamChars.cs
- ChannelDispatcherBase.cs
- EndPoint.cs
- ADMembershipUser.cs
- InstanceNameConverter.cs
- DataGridColumnHeaderAutomationPeer.cs
- ReturnValue.cs
- TextSearch.cs
- StorageInfo.cs
- Mapping.cs
- XmlSerializableServices.cs
- ExpressionEditorAttribute.cs
- NativeWindow.cs
- LocatorPart.cs
- XamlSerializationHelper.cs
- DragStartedEventArgs.cs
- PropertyGridEditorPart.cs
- ContentPlaceHolder.cs
- FacetDescription.cs
- UnsafeNativeMethodsCLR.cs
- IgnorePropertiesAttribute.cs
- DbDataRecord.cs
- RichTextBoxAutomationPeer.cs
- TemporaryBitmapFile.cs
- ToolboxComponentsCreatingEventArgs.cs
- ExtenderControl.cs
- ConstraintStruct.cs
- Vector3DValueSerializer.cs
- Vector3dCollection.cs
- WindowProviderWrapper.cs
- RepeatBehavior.cs
- MediaContext.cs
- TimeZone.cs
- WebBrowserBase.cs
- PersonalizationStateInfoCollection.cs
- GeneralTransformGroup.cs
- SoapSchemaMember.cs
- RewritingSimplifier.cs
- UTF7Encoding.cs
- GridViewRowEventArgs.cs
- FrameworkElement.cs
- EventProviderWriter.cs
- SemanticResolver.cs
- DictionaryItemsCollection.cs
- InvokeProviderWrapper.cs
- TransformerInfo.cs
- ConfigurationValidatorBase.cs
- RootAction.cs
- CssStyleCollection.cs
- ConfigurationSchemaErrors.cs
- LocalBuilder.cs
- Merger.cs
- SqlDataSourceCache.cs