Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / WebControls / ContentPlaceHolder.cs / 1 / ContentPlaceHolder.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Security.Permissions; using System.Web.UI; using System.Web.Util; internal class ContentPlaceHolderBuilder : ControlBuilder { private string _contentPlaceHolderID; private string _templateName; internal string Name { get { return _templateName; } } public override void Init(TemplateParser parser, ControlBuilder parentBuilder, Type type, string tagName, string ID, IDictionary attribs) { // Copy the ID so that it will be available when BuildObject is called _contentPlaceHolderID = ID; if (parser.FInDesigner) { // shortcut for designer base.Init(parser, parentBuilder, type, tagName, ID, attribs); return; } if (String.IsNullOrEmpty(ID)) { throw new HttpException(SR.GetString(SR.Control_Missing_Attribute, "ID", type.Name)); } _templateName = ID; MasterPageParser masterPageParser = parser as MasterPageParser; if (masterPageParser == null) { throw new HttpException(SR.GetString(SR.ContentPlaceHolder_only_in_master)); } base.Init(parser, parentBuilder, type, tagName, ID, attribs); if (masterPageParser.PlaceHolderList.Contains(Name)) throw new HttpException(SR.GetString(SR.ContentPlaceHolder_duplicate_contentPlaceHolderID, Name)); masterPageParser.PlaceHolderList.Add(Name); } public override object BuildObject() { MasterPage masterPage = TemplateControl as MasterPage; Debug.Assert(masterPage != null || InDesigner); // Instantiate the ContentPlaceHolder ContentPlaceHolder cph = (ContentPlaceHolder) base.BuildObject(); // If the page is providing content, instantiate it in the holder if (PageProvidesMatchingContent(masterPage)) { ITemplate tpl = ((System.Web.UI.ITemplate)(masterPage.ContentTemplates[_contentPlaceHolderID])); HttpContext context = HttpContext.Current; // Remember the old TemplateControl TemplateControl oldControl = context.TemplateControl; // Storing the template control into the context // since each thread needs to set it differently. context.TemplateControl = masterPage._ownerControl; try { // Instantiate the template using the correct TemplateControl tpl.InstantiateIn(cph); } finally { // Revert back to the old templateControl context.TemplateControl = oldControl; } } return cph; } internal override void BuildChildren(object parentObj) { MasterPage masterPage = TemplateControl as MasterPage; // If the page is providing content, don't call the base, which would // instantiate the default content (which we don't want) if (PageProvidesMatchingContent(masterPage)) return; base.BuildChildren(parentObj); } private bool PageProvidesMatchingContent(MasterPage masterPage) { if (masterPage != null && masterPage.ContentTemplates != null && masterPage.ContentTemplates.Contains(_contentPlaceHolderID)) { return true; } return false; } } // Factory used to efficiently create builder instances internal class ContentPlaceHolderBuilderFactory: IWebObjectFactory { object IWebObjectFactory.CreateInstance() { return new ContentPlaceHolderBuilder(); } } [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [ControlBuilderAttribute(typeof(ContentPlaceHolderBuilder))] [Designer("System.Web.UI.Design.WebControls.ContentPlaceHolderDesigner, " + AssemblyRef.SystemDesign)] [ToolboxItemFilter("System.Web.UI")] [ToolboxItemFilter("Microsoft.VisualStudio.Web.WebForms.MasterPageWebFormDesigner", ToolboxItemFilterType.Require)] [ToolboxData("<{0}:ContentPlaceHolder runat=\"server\">{0}:ContentPlaceHolder>")] public class ContentPlaceHolder : Control, INonBindingContainer { } }
Link Menu
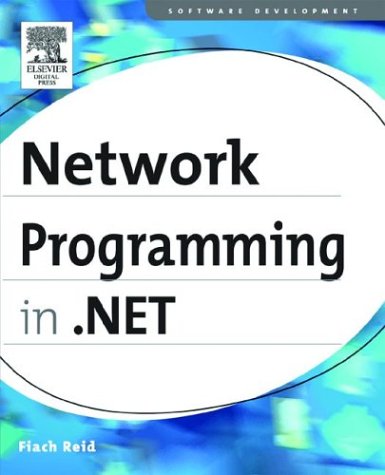
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DesignSurfaceCollection.cs
- StringHandle.cs
- GenericTypeParameterConverter.cs
- XmlSchemaType.cs
- ReferenceConverter.cs
- _PooledStream.cs
- Encoder.cs
- TreeView.cs
- DataRowComparer.cs
- ConfigXmlCDataSection.cs
- CollectionChangeEventArgs.cs
- Panel.cs
- DesignerGenericWebPart.cs
- DefaultPrintController.cs
- ResourceDescriptionAttribute.cs
- CursorConverter.cs
- EntityContainer.cs
- IndicShape.cs
- StrokeNodeData.cs
- StreamGeometryContext.cs
- COM2FontConverter.cs
- WindowsListViewItemCheckBox.cs
- SpotLight.cs
- MimeTypeMapper.cs
- DetailsViewAutoFormat.cs
- dbenumerator.cs
- GregorianCalendar.cs
- EntityType.cs
- EntityConnectionStringBuilder.cs
- Action.cs
- ListItem.cs
- QilParameter.cs
- SmiConnection.cs
- NavigationHelper.cs
- LogFlushAsyncResult.cs
- InputScopeAttribute.cs
- StylusDownEventArgs.cs
- CompressionTracing.cs
- PropertyOverridesTypeEditor.cs
- ResourceExpression.cs
- OAVariantLib.cs
- PagerSettings.cs
- DataExpression.cs
- TypedElement.cs
- ControlPersister.cs
- RuleInfoComparer.cs
- SystemBrushes.cs
- ContentElement.cs
- DataBoundLiteralControl.cs
- PathSegment.cs
- ImplicitInputBrush.cs
- GeneratedView.cs
- XComponentModel.cs
- XmlAttributeProperties.cs
- AssemblyAttributes.cs
- DynamicField.cs
- RectValueSerializer.cs
- RegexRunner.cs
- CodeChecksumPragma.cs
- ProcessModule.cs
- CompositeActivityMarkupSerializer.cs
- OperationCanceledException.cs
- SoapDocumentMethodAttribute.cs
- IIS7WorkerRequest.cs
- LocatorPartList.cs
- CompoundFileStorageReference.cs
- ExtendedPropertyDescriptor.cs
- Relationship.cs
- InlineObject.cs
- XmlSortKeyAccumulator.cs
- HMACSHA512.cs
- PolygonHotSpot.cs
- Scripts.cs
- PortCache.cs
- FormViewPageEventArgs.cs
- TaskFormBase.cs
- TextEditor.cs
- HttpListenerPrefixCollection.cs
- DataTable.cs
- LocalizabilityAttribute.cs
- InfoCardSchemas.cs
- LineSegment.cs
- SortFieldComparer.cs
- ExpressionBuilder.cs
- EdgeProfileValidation.cs
- SafeHGlobalHandleCritical.cs
- ByteFacetDescriptionElement.cs
- Internal.cs
- HandleTable.cs
- CharKeyFrameCollection.cs
- StylusPointPropertyInfo.cs
- Module.cs
- Timeline.cs
- IDReferencePropertyAttribute.cs
- BaseValidator.cs
- BitmapEffectCollection.cs
- CreateUserWizardStep.cs
- MimeFormatter.cs
- Atom10FormatterFactory.cs
- SafeEventHandle.cs